本节重点讲解Java中的IO流,Java中所有字节相关的输入流的基类为InputStream、输出流的基类为OutputStream,Java中所有与字符相关的输入流的基类为Reader、输出流的基类为Writer。如果操作的是字符,可以选择用字符流和字节流,如果操作的是非字符,只能用字节流。常见字符流包括:(FileReader,FileWriter)、(BufferedReader,BufferedWriter),常见的字节流包括(FileInputStream,FileOutputStream)、(BufferedInputStream,BufferedOutputStream),常见转换流包括InputStreamReader、OutputStreamWriter,Java也提供了常用的文件封装类File。下面一一介绍。
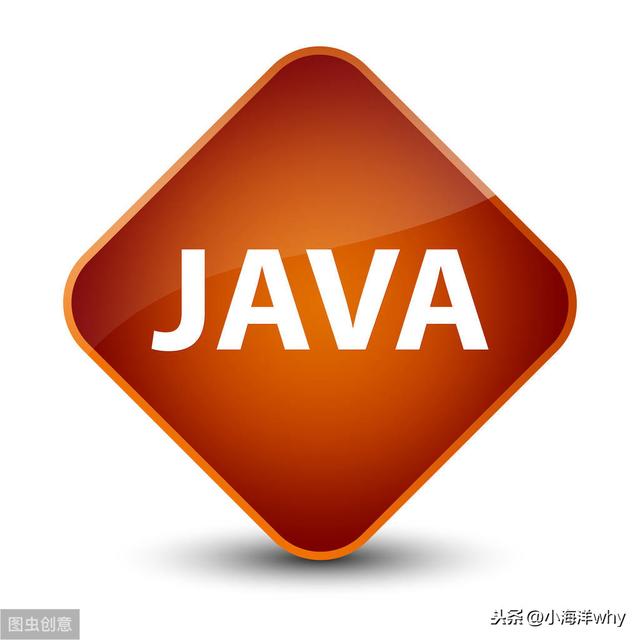
一、字符流
FileReader:读取字符文件,具体例子如下。
public static void main(String[] args) { FileReader reader = null; try { reader = new FileReader("D:/why.txt"); int len = 0; char[] buf = new char[1024]; while((len = reader.read(buf)) != -1) { System.out.println(new String(buf, 0, len)); } } catch (IOException e) { e.printStackTrace(); } finally { try { if (reader != null) { reader.close(); } } catch (IOException e) { e.printStackTrace(); } }}
BufferedReader:提供字符流读取时的缓冲区,减少IO操作,提高读取效率。具体例子如下。
public static void main(String[] args) { BufferedReader reader = null; try { reader = new BufferedReader(new FileReader("D:/why.txt")); int len = 0; char[] buf = new char[1024]; while((len = reader.read(buf)) != -1) { System.out.println(new String(buf, 0, len)); } } catch (IOException e) { e.printStackTrace(); } finally { try { if (reader != null) { reader.close(); } } catch (IOException e) { e.printStackTrace(); } }}
FileReader和BufferedReader用时对比试验,注意,选择大文件效果才明显
public static void main(String[] args) { fileReader(); bufferedReader();}private static void bufferedReader() { long starTime = System.currentTimeMillis(); BufferedReader reader = null; try { reader = new BufferedReader(new FileReader("D:/why.txt")); int len = 0; char[] buf = new char[1024]; while((len = reader.read(buf)) != -1) { System.out.println(new String(buf, 0, len)); } } catch (IOException e) { e.printStackTrace(); } finally { try { if (reader != null) { reader.close(); } } catch (IOException e) { e.printStackTrace(); } } long endTime = System.currentTimeMillis(); System.out.println("BufferedReader用时:"+(endTime - starTime)+"毫秒");}private static void fileReader() { long starTime = System.currentTimeMillis(); FileReader reader = null; try { reader = new FileReader("D:/why.txt"); int len = 0; char[] buf = new char[1024]; while((len = reader.read(buf)) != -1) { System.out.println(new String(buf, 0, len)); } } catch (IOException e) { e.printStackTrace(); } finally { try { if (reader != null) { reader.close(); } } catch (IOException e) { e.printStackTrace(); } } long endTime = System.currentTimeMillis(); System.out.println("FileReader用时:"+(endTime - starTime)+"毫秒");}
FileWriter:写文件,具体例子如下。
long starTime = System.currentTimeMillis();FileWriter writer = null;try {writer = new FileWriter("D:/why.txt");writer.write("dsss");} catch (IOException e) {e.printStackTrace();} finally {try {if (writer != null) {writer.close();}} catch (IOException e) {e.printStackTrace();}}long endTime = System.currentTimeMillis();System.out.println("+=用时:"+(endTime - starTime)+"毫秒");}
BufferedWriter:写文件,具体例子如下。
public static void main(String[] args) { long starTime = System.currentTimeMillis(); BufferedWriter writer = null; try { writer = new BufferedWriter(new FileWriter("D:/why.txt")); writer.write("dsss"); } catch (IOException e) { e.printStackTrace(); }finally { try { if (writer != null) { writer.close(); } } catch (IOException e) { e.printStackTrace(); } } long endTime = System.currentTimeMillis(); System.out.println("+=用时:"+(endTime - starTime)+"毫秒");}
二、字节流
FileInputStream:以字节流方式读文件,具体例子如下。
public static void main(String[] args) { long starTime = System.currentTimeMillis(); FileInputStream fis = null; try { fis = new FileInputStream("D:/why.txt"); int len = 0; byte[] buf = new byte[1024]; while((len = fis.read(buf)) != -1) { System.out.println(new String(buf, 0, len)); } } catch (IOException e) { e.printStackTrace(); }finally { try { if (fis != null) { fis.close(); } } catch (IOException e) { e.printStackTrace(); } } long endTime = System.currentTimeMillis(); System.out.println("+=用时:"+(endTime - starTime)+"毫秒");}
BufferedInputStream:以带缓冲区的字节流方式读,与FileInputStream的对比试验可自行参照上面例子编写。具体例子如下。
public static void main(String[] args) { long starTime = System.currentTimeMillis(); BufferedInputStream bis = null; try { bis = new BufferedInputStream(new FileInputStream("D:/why.txt")); int len = 0; byte[] buf = new byte[1024]; while((len = bis.read(buf)) != -1) { System.out.println(new String(buf, 0, len)); } } catch (IOException e) { e.printStackTrace(); }finally { try { if (bis != null) { bis.close(); } } catch (IOException e) { e.printStackTrace(); } } long endTime = System.currentTimeMillis(); System.out.println("+=用时:"+(endTime - starTime)+"毫秒");}
FileOutputStream:以字节流方式写,具体例子如下。
public static void main(String[] args) { long starTime = System.currentTimeMillis(); FileOutputStream fos = null; try { fos = new FileOutputStream("D:/why.txt"); fos.write("dsss".getBytes()); } catch (IOException e) { e.printStackTrace(); }finally { try { if (fos != null) { fos.close(); } } catch (IOException e) { e.printStackTrace(); } } long endTime = System.currentTimeMillis(); System.out.println("+=用时:"+(endTime - starTime)+"毫秒");}
BufferedOutputStream:以带缓冲区的字节流方式写,具体例子如下。
public static void main(String[] args) { long starTime = System.currentTimeMillis(); BufferedOutputStream bos = null; try { bos = new BufferedOutputStream(new FileOutputStream("D:/why.txt")); bos.write("dsss".getBytes()); } catch (IOException e) { e.printStackTrace(); }finally { try { if (bos != null) { bos.close(); } } catch (IOException e) { e.printStackTrace(); } } long endTime = System.currentTimeMillis(); System.out.println("+=用时:"+(endTime - starTime)+"毫秒");}
三、转换流
当我们读取字符数据时,使用字符流效率比字节流高,因此有时需要将字节流转换为字符流。
将字节输入流转换为字符输入流
BufferedReader reader = new BufferedReader(new InputStreamReader(new FileInputStream("D:/why.txt")));
将字节输出流转换为字符输出流
BufferedWriter writer = new BufferedWriter(new OutputStreamWriter(new FileOutputStream("D:/why.txt")));
四、File类
为了描述文件,Java提供File类,使用方法如下。
File file = new File("D:/why.txt");
File类为我们提供了很多关于文件的信息,比如文件大小、文件名等,方便我们操作。我们可将上述程序中的"D:/why.txt"替换为new File("D:/why.txt");,读者可在IDE中尝试。