TreeMap
介绍
treeMap是一个基于红黑树实现,他的排序默认是根据key的自然排序,或者根据传入的Comparator进行排序。
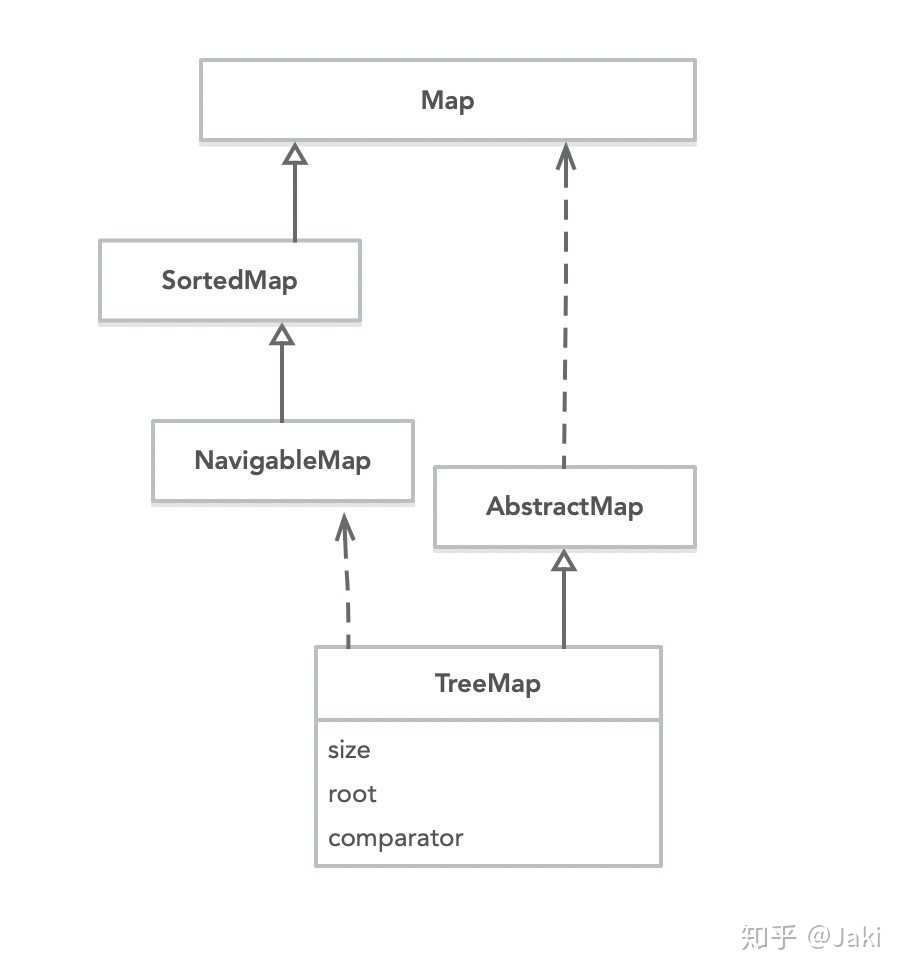
treeMap继承了AbstractMap,实现了NavigableMap接口。
构造器
treeMap有四个构造方法
//构造一个新的空的treemap,使用的是默认的根据key的自然排序
public TreeMap()
//构造一个新的空的treemap,使用的是给定的排序器进行排序
public TreeMap(Comparator<? super K> comparator)
//构造一个新的包含给定map值的map,使用的是默认的根据key的自然排序
public TreeMap(Map<? extends K, ? extends V> m)
//构造一个新的包含给定map值的map,使用给定的map的排序方式
public TreeMap(SortedMap<K, ? extends V> m)
GET
get方法遍历比较简单他的方法复杂度是log(n)
重点是getEntry方法
final Entry<K,V> getEntry(Object key) {
// Offload comparator-based version for sake of performance
if (comparator != null)
return getEntryUsingComparator(key);
//如果key是null会报空指针
if (key == null)
throw new NullPointerException();
@SuppressWarnings("unchecked")
Comparable<? super K> k = (Comparable<? super K>) key;
Entry<K,V> p = root;
//根据当前的key值进行查询,从根开始遍历
while (p != null) {
int cmp = k.compareTo(p.key);
if (cmp < 0)
p = p.left;
else if (cmp > 0)
p = p.right;
else
return p;
}
return null;
}
put
put方法相对于get比较复杂
public V put(K key, V value) {
Entry<K,V> t = root;
if (t == null) {
//一开始初始化,创建根节点
compare(key, key); // type (and possibly null) check
root = new Entry<>(key, value, null);
size = 1;
modCount++;
return null;
}
int cmp;
Entry<K,V> parent;
// split comparator and comparable paths
Comparator<? super K> cpr = comparator;
//以下代码根据比较器来找到该key的位置,如果原来有值会覆盖
if (cpr != null) {
//如果有传入的比较器
do {
parent = t;
cmp = cpr.compare(key, t.key);
if (cmp < 0)
t = t.left;
else if (cmp > 0)
t = t.right;
else
return t.setValue(value);
} while (t != null);
}
else {
if (key == null)
throw new NullPointerException();
@SuppressWarnings("unchecked")
Comparable<? super K> k = (Comparable<? super K>) key;
do {
parent = t;
cmp = k.compareTo(t.key);
if (cmp < 0)
t = t.left;
else if (cmp > 0)
t = t.right;
else
return t.setValue(value);
} while (t != null);
}
Entry<K,V> e = new Entry<>(key, value, parent);
//找到位置就插入,根据比较结果
if (cmp < 0)
parent.left = e;
else
parent.right = e;
//进行红黑树的操作
fixAfterInsertion(e);
size++;
modCount++;
return null;
}
Remove
remove和put的操作也差不多
public V remove(Object key) {
Entry<K,V> p = getEntry(key);
if (p == null)
return null;
V oldValue = p.value;
//进行红黑树删除节点
deleteEntry(p);
return oldValue;
}
代码示例
TreeMap<String,String> treeMapA = Maps.newTreeMap((o1, o2) -> 0);
treeMapA.put("b", "b");
treeMapA.put("d", "d");
treeMapA.put("a", "a");
treeMapA.put("c", "c");
System.out.println(treeMapA);
TreeMap<String,String> treeMapB = Maps.newTreeMap();
treeMapB.put("b", "b");
treeMapB.put("d", "d");
treeMapB.put("a", "a");
treeMapB.put("c", "c");
System.out.println(treeMapB);
TreeMap<String,String> treeMapC = Maps.newTreeMap(treeMapB);
treeMapB.put("e", "e");
System.out.println(treeMapC);
TreeMap<String,String> treeMapD = Maps.newTreeMap(treeMapA);
treeMapB.put("e", "e");
System.out.println(treeMapD);
打印如下结果
{b=c}
{a=a, b=b, c=c, d=d}
{a=a, b=b, c=c, d=d}
{b=c}