前言
本文介绍Spring Boot中读取配置属性的几种方式,项目示例中用到的application.yml和application.properties定义如下:
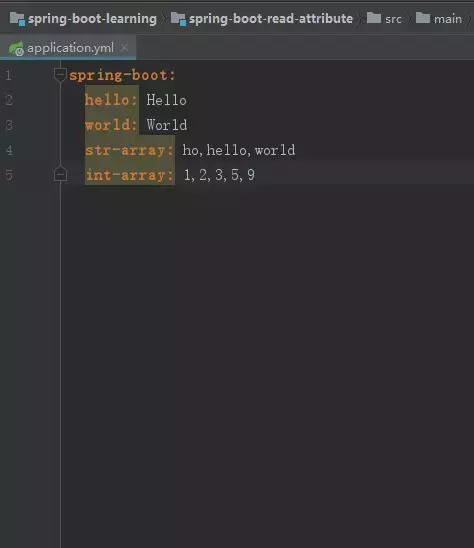
application.yml
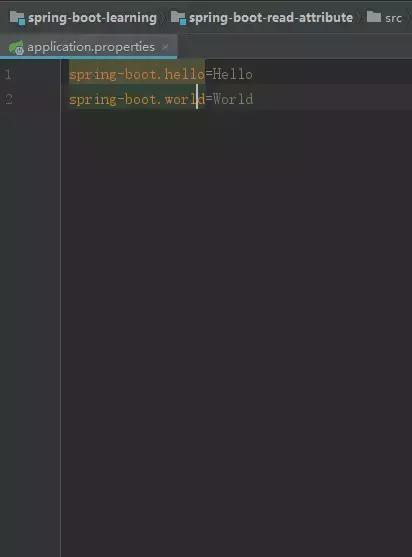
application.properties
@Value
@Value是比较常见的注入方式,功能强大但一般可读性较差。
@Value("str") private String str; // 注入普通字符串 @Value("${hello}") private String hello; // 注入配置属性 @Value("#{systemProperties['os.name']}") private String systemPropertiesName; // 注入操作系统属性 @Value("#{ T(java.lang.Math).random() * 100.0 }") private double randomNumber; //注入表达式结果 @Value("#{userBean.name}") private String name; // 注入Bean属性
下面通过@Value注解获取定义在配置文件的属性值:
@SpringBootApplication public class AttributeApplication { private static final String SPRING_BOOT_HELLO = "spring-boot.hello"; @Value("${" + SPRING_BOOT_HELLO + "}") private String hello; /** * 1. 通过@Value注解获取值 */ public void getAttrByValueAnnotation() { System.out.println("1. 通过@Value注解获取值: " + hello); } public static void main(String[] args) { ConfigurableApplicationContext applicationContext = SpringApplication.run(AttributeApplication.class, args); AttributeApplication bean = applicationContext.getBean(AttributeApplication.class); bean.getAttrByValueAnnotation(); } }
扩展说明:
@SpringBootApplication public class AttributeApplication { private static final String SPRING_BOOT_STR_ARRAY = "spring-boot.str-array"; private static final String SPRING_BOOT_INT_ARRAY = "spring-boot.int-array"; /** * Attention : it is error if use Integer[] */ @Value("${" + SPRING_BOOT_INT_ARRAY + "}") private int[] array; /** * 通过@Value注解获取数组 */ public void getArrayAttr() { System.out.println("5. 通过@Value注解获取数组: " + Arrays.toString(array)); } @Value("#{'${" + SPRING_BOOT_STR_ARRAY + "}'.split(',')}") private List list; /** * 通过@Value注解获取List */ public void getListAttr() { System.out.println("6. 通过@Value注解获取List: " + list.toString()); } public static void main(String[] args) { ConfigurableApplicationContext applicationContext = SpringApplication.run(AttributeApplication.class, args); AttributeApplication bean = applicationContext.getBean(AttributeApplication.class); bean.getArrayAttr(); bean.getListAttr(); } }
Environment
通过注入获取Environment对象,然后再获取定义在配置文件的属性值:
@SpringBootApplication public class AttributeApplication { private static final String SPRING_BOOT_HELLO = "spring-boot.hello"; @Resource private Environment environment; /** * 2. 通过注入Environment获取值 */ public void getAttrByEnvironment() { String property = environment.getProperty(SPRING_BOOT_HELLO); System.out.println("2-1. 通过注入Environment获取值: " + property); } public static void main(String[] args) { ConfigurableApplicationContext applicationContext = SpringApplication.run(AttributeApplication.class, args); AttributeApplication bean = applicationContext.getBean(AttributeApplication.class); bean.getAttrByEnvironment(); } }
还可以在启动类中通过ApplicationContext获取Environment对象后再获取值:
@SpringBootApplication public class AttributeApplication { private static final String UNDEFINED = "undefined"; private static final String SPRING_BOOT_HELLO = "spring-boot.hello"; public static void main(String[] args) { ConfigurableApplicationContext applicationContext = SpringApplication.run(AttributeApplication.class, args); System.out.println("2-2. 通过ApplicationContext获取Environment后再获取值: " + applicationContext .getEnvironment().getProperty(SPRING_BOOT_HELLO, UNDEFINED)); } }
@ConfigurationProperties
@ConfigurationProperties作用在类上,用于注入Bean属性,然后再通过当前Bean获取注入值:
@SpringBootApplication public class AttributeApplication { private static final String APPLICATION_YML = "application.yml"; private static final String SPRING_BOOT_PREFIX = "spring-boot"; @Data @Component @PropertySource("classpath:" + APPLICATION_YML) @ConfigurationProperties(prefix = SPRING_BOOT_PREFIX) class Attribute { private String hello; private String world; } @Resource private Attribute attribute; /** * 3. 通过@ConfigurationProperties注入对象属性获取 */ public void getAttrByConfigurationPropertiesAnnotation() { System.out.println("3. 通过@ConfigurationProperties注入对象属性获取: " + attribute); } public static void main(String[] args) { ConfigurableApplicationContext applicationContext = SpringApplication.run(AttributeApplication.class, args); AttributeApplication bean = applicationContext.getBean(AttributeApplication.class); bean.getAttrByConfigurationPropertiesAnnotation(); } }
PropertiesLoaderUtils
@SpringBootApplication public class AttributeApplication { private static final String UNDEFINED = "undefined"; private static final String APPLICATION_PROPERTIES = "application.properties"; private static final String SPRING_BOOT_HELLO = "spring-boot.hello"; /** * 4. 通过PropertiesLoaderUtils获取(注意,此工具类仅可处理.properties或.xml配置文件) */ public void getAttrByPropertiesLoaderUtils() { try { ClassPathResource resource = new ClassPathResource(APPLICATION_PROPERTIES); Properties properties = PropertiesLoaderUtils.loadProperties(resource); String property = properties.getProperty(SPRING_BOOT_HELLO, UNDEFINED); System.out.println("4. 通过PropertiesLoaderUtils获取: " + property); } catch (IOException e) { e.printStackTrace(); } } public static void main(String[] args) { ConfigurableApplicationContext applicationContext = SpringApplication.run(AttributeApplication.class, args); AttributeApplication bean = applicationContext.getBean(AttributeApplication.class); bean.getAttrByPropertiesLoaderUtils(); } }
源码:https://github.com/happyjared/spring-boot-learning/tree/master/spring-boot-read-attribute