1. MVC使用
在研究源码之前,先来回顾以下springmvc 是如何配置的,这将能使我们更容易理解源码。
1.1 web.xml
1 2 mvc-dispatcher 3 org.springframework.web.servlet.DispatcherServlet 4 8 9 contextConfigLocation10 classpath:spring/spring-*.xml11 12 13 14 mvc-dispatcher15 16 /17
值的注意的是contextConfigLocation和DispatcherServlet(用此类来拦截请求)的引用和配置。
1.2 spring-web.xml
1 2 3 7 8 9 13 14 15 16 17 18 19 20 21 22 23
值的注意的是InternalResourceViewResolver,它会在ModelAndView返回的试图名前面加上prefix前缀,在后面加载suffix指定后缀。
SpringMvc主支源码分析
引用《Spring in Action》中的一张图来更好的了解执行过程:
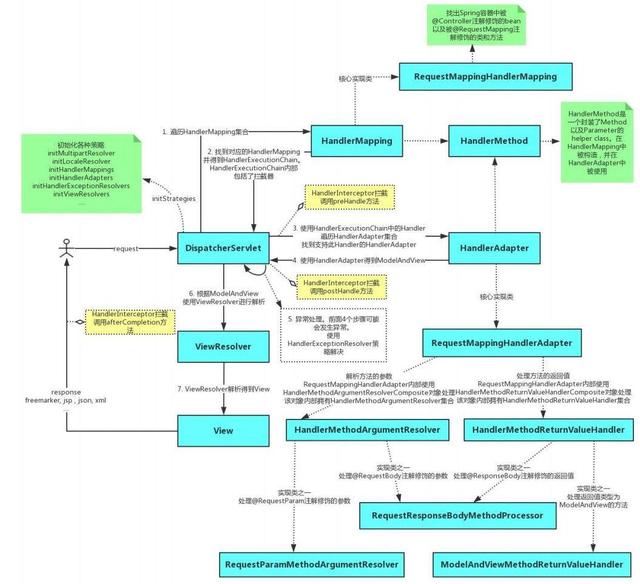
上图流程总体来说可分为三大块:
- Map的建立(并放入WebApplicationContext)
- HttpRequest请求中Url的请求拦截处理(DispatchServlet处理)
- 反射调用Controller中对应的处理方法,并返回视图
本文将围绕这三块进行分析。
1. Map的建立
在容器初始化时会建立所有 url 和 Controller 的对应关系,保存到 Map中,那是如何保存的呢。
ApplicationObjectSupport #setApplicationContext方法
如果想学习Java工程化、高性能及分布式、深入浅出。微服务、Spring,MyBatis,Netty源码分析的朋友可以加我的Java高级交流:787707172,群里有阿里大牛直播讲解技术,以及Java大型互联网技术的视频免费分享给大家。
1 // 初始化ApplicationContext2 @Override3 public void initApplicationContext() throws ApplicationContextException {4 super.initApplicationContext();5 detectHandlers();6 }
AbstractDetectingUrlHandlerMapping #detectHandlers()方法:
1 /** 2 * 建立当前ApplicationContext 中的 所有Controller 和url 的对应关系 3 * Register all handlers found in the current ApplicationContext. 4 *
The actual URL determination for a handler is up to the concrete 5 * {@link #determineUrlsForHandler(String)} implementation. A bean for 6 * which no such URLs could be determined is simply not considered a handler. 7 * @throws org.springframework.beans.BeansException if the handler couldn't be registered 8 * @see #determineUrlsForHandler(String) 9 */10 protected void detectHandlers() throws BeansException {11 if (logger.isDebugEnabled()) {12 logger.debug("Looking for URL mappings in application context: " + getApplicationContext());13 }14 // 获取容器中的beanNames15 String[] beanNames = (this.detectHandlersInAncestorContexts ?16 BeanFactoryUtils.beanNamesForTypeIncludingAncestors(getApplicationContext(), Object.class) :17 getApplicationContext().getBeanNamesForType(Object.class));18 // 遍历 beanNames 并找到对应的 url19 // Take any bean name that we can determine URLs for.20 for (String beanName : beanNames) {21 // 获取bean上的url(class上的url + method 上的 url)22 String[] urls = determineUrlsForHandler(beanName);23 if (!ObjectUtils.isEmpty(urls)) {24 // URL paths found: Let's consider it a handler.25 // 保存url 和 beanName 的对应关系26 registerHandler(urls, beanName);27 }28 else {29 if (logger.isDebugEnabled()) {30 logger.debug("Rejected bean name '" + beanName + "': no URL paths identified");31 }32 }33 }34 }
determineUrlsForHandler()方法:
该方法在不同的子类有不同的实现,我这里分析的是DefaultAnnotationHandlerMapping类的实现,该类主要负责处理@RequestMapping注解形式的声明。
1 /** 2 * 获取@RequestMaping注解中的url 3 * Checks for presence of the {@link org.springframework.web.bind.annotation.RequestMapping} 4 * annotation on the handler class and on any of its methods. 5 */ 6 @Override 7 protected String[] determineUrlsForHandler(String beanName) { 8 ApplicationContext context = getApplicationContext(); 9 Class> handlerType = context.getType(beanName);10 // 获取beanName 上的requestMapping11 RequestMapping mapping = context.findAnnotationOnBean(beanName, RequestMapping.class);12 if (mapping != null) {13 // 类上面有@RequestMapping 注解14 this.cachedMappings.put(handlerType, mapping);15 Set urls = new LinkedHashSet();16 // mapping.value()就是获取@RequestMapping注解的value值17 String[] typeLevelPatterns = mapping.value();18 if (typeLevelPatterns.length > 0) {19 // 获取Controller 方法上的@RequestMapping20 String[] methodLevelPatterns = determineUrlsForHandlerMethods(handlerType);21 for (String typeLevelPattern : typeLevelPatterns) {22 if (!typeLevelPattern.startsWith("/")) {23 typeLevelPattern = "/" + typeLevelPattern;24 }25 for (String methodLevelPattern : methodLevelPatterns) {26 // controller的映射url+方法映射的url27 String combinedPattern = getPathMatcher().combine(typeLevelPattern, methodLevelPattern);28 // 保存到set集合中29 addUrlsForPath(urls, combinedPattern);30 }31 addUrlsForPath(urls, typeLevelPattern);32 }33 // 以数组形式返回controller上的所有url34 return StringUtils.toStringArray(urls);35 }36 else {37 // controller上的@RequestMapping映射url为空串,直接找方法的映射url38 return determineUrlsForHandlerMethods(handlerType);39 }40 }41 // controller上没@RequestMapping注解42 else if (AnnotationUtils.findAnnotation(handlerType, Controller.class) != null) {43 // 获取controller中方法上的映射url44 return determineUrlsForHandlerMethods(handlerType);45 }46 else {47 return null;48 }49 }
更深的细节代码就比较简单了,有兴趣的可以继续深入。
到这里,Controller和Url的映射就装配完成,下来就分析请求的处理过程。
2. url的请求处理
我们在xml中配置了DispatcherServlet为调度器,所以我们就来看它的代码,可以
从名字上看出它是个Servlet,那么它的核心方法就是doService()
DispatcherServlet #doService():
1 /** 2 * 将DispatcherServlet特定的请求属性和委托 公开给{@link #doDispatch}以进行实际调度。 3 * Exposes the DispatcherServlet-specific request attributes and delegates to {@link #doDispatch} 4 * for the actual dispatching. 5 */ 6 @Override 7 protected void doService(HttpServletRequest request, HttpServletResponse response) throws Exception { 8 if (logger.isDebugEnabled()) { 9 String requestUri = new UrlPathHelper().getRequestUri(request);10 logger.debug("DispatcherServlet with name '" + getServletName() + "' processing " + request.getMethod() +11 " request for [" + requestUri + "]");12 }13 14 //在包含request的情况下保留请求属性的快照,15 //能够在include之后恢复原始属性。16 Map attributesSnapshot = null;17 if (WebUtils.isIncludeRequest(request)) {18 logger.debug("Taking snapshot of request attributes before include");19 attributesSnapshot = new HashMap();20 Enumeration attrNames = request.getAttributeNames();21 while (attrNames.hasMoreElements()) {22 String attrName = (String) attrNames.nextElement();23 if (this.cleanupAfterInclude || attrName.startsWith("org.springframework.web.servlet")) {24 attributesSnapshot.put(attrName, request.getAttribute(attrName));25 }26 }27 }28 29 // 使得request对象能供 handler处理和view处理 使用30 request.setAttribute(WEB_APPLICATION_CONTEXT_ATTRIBUTE, getWebApplicationContext());31 request.setAttribute(LOCALE_RESOLVER_ATTRIBUTE, this.localeResolver);32 request.setAttribute(THEME_RESOLVER_ATTRIBUTE, this.themeResolver);33 request.setAttribute(THEME_SOURCE_ATTRIBUTE, getThemeSource());34 35 try {36 doDispatch(request, response);37 }38 finally {39 // 如果不为空,则还原原始属性快照。40 if (attributesSnapshot != null) {41 restoreAttributesAfterInclude(request, attributesSnapshot);42 }43 }44 }
可以看到,它将请求拿到后,主要是给request设置了一些对象,以便于后续工作的处理(Handler处理和view处理)。比如WebApplicationContext,它里面就包含了我们在第一步完成的controller与url映射的信息。
DispatchServlet # doDispatch()
如果想学习Java工程化、高性能及分布式、深入浅出。微服务、Spring,MyBatis,Netty源码分析的朋友可以加我的Java高级交流:787707172,群里有阿里大牛直播讲解技术,以及Java大型互联网技术的视频免费分享给大家。
1 /** 2 * 控制请求转发 3 * Process the actual dispatching to the handler. 4 *
The handler will be obtained by applying the servlet's HandlerMappings in order. 5 * The HandlerAdapter will be obtained by querying the servlet's installed HandlerAdapters 6 * to find the first that supports the handler class. 7 *
All HTTP methods are handled by this method. It's up to HandlerAdapters or handlers 8 * themselves to decide which methods are acceptable. 9 * @param request current HTTP request 10 * @param response current HTTP response 11 * @throws Exception in case of any kind of processing failure 12 */ 13 protected void doDispatch(HttpServletRequest request, HttpServletResponse response) throws Exception { 14 HttpServletRequest processedRequest = request; 15 HandlerExecutionChain mappedHandler = null; 16 int interceptorIndex = -1; 17 18 try { 19 20 ModelAndView mv; 21 boolean errorView = false; 22 23 try { 24 // 1. 检查是否是上传文件 25 processedRequest = checkMultipart(request); 26 27 // Determine handler for the current request. 28 // 2. 获取handler处理器,返回的mappedHandler封装了handlers和interceptors 29 mappedHandler = getHandler(processedRequest, false); 30 if (mappedHandler == null || mappedHandler.getHandler() == null) { 31 // 返回404 32 noHandlerFound(processedRequest, response); 33 return; 34 } 35 36 // Apply preHandle methods of registered interceptors. 37 // 获取HandlerInterceptor的预处理方法 38 HandlerInterceptor[] interceptors = mappedHandler.getInterceptors(); 39 if (interceptors != null) { 40 for (int i = 0; i < interceptors.length; i++) { 41 HandlerInterceptor interceptor = interceptors[i]; 42 if (!interceptor.preHandle(processedRequest, response, mappedHandler.getHandler())) { 43 triggerAfterCompletion(mappedHandler, interceptorIndex, processedRequest, response, null); 44 return; 45 } 46 interceptorIndex = i; 47 } 48 } 49 50 // Actually invoke the handler. 51 // 3. 获取handler适配器 Adapter 52 HandlerAdapter ha = getHandlerAdapter(mappedHandler.getHandler()); 53 // 4. 实际的处理器处理并返回 ModelAndView 对象 54 mv = ha.handle(processedRequest, response, mappedHandler.getHandler()); 55 56 // Do we need view name translation? 57 if (mv != null && !mv.hasView()) { 58 mv.setViewName(getDefaultViewName(request)); 59 } 60 61 // HandlerInterceptor 后处理 62 if (interceptors != null) { 63 for (int i = interceptors.length - 1; i >= 0; i--) { 64 HandlerInterceptor interceptor = interceptors[i]; 65 // 结束视图对象处理 66 interceptor.postHandle(processedRequest, response, mappedHandler.getHandler(), mv); 67 } 68 } 69 } 70 catch (ModelAndViewDefiningException ex) { 71 logger.debug("ModelAndViewDefiningException encountered