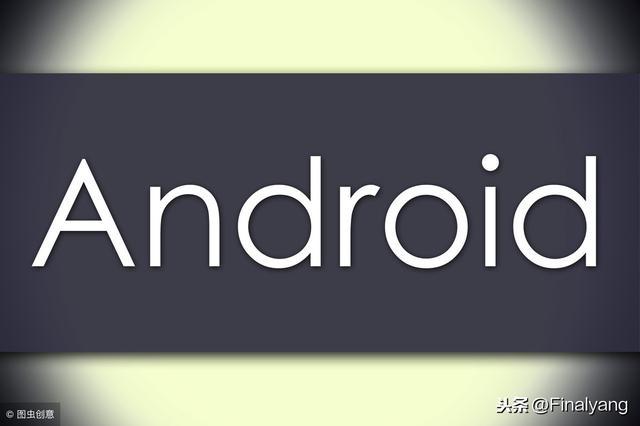
使用HttpUrlConnection GET方式提交数据给服务器
[1]搭建服务器
String name = request.getParameter("username"); name = URLEncoder.encode(name, "iso-8859-1"); name = URLDecoder.decode(name, "utf-8"); String pwd = request.getParameter("pwd"); System.out.println(request.getMethod()+" name = " + name + " pwd = " + pwd); if (name.equals("aaa") && pwd.equals("123")) { response.getOutputStream().write("成功".getBytes()); } else { response.getOutputStream().write("fail".getBytes()); }
[2]客户端发送数据
Get请求代码
// [1]拼接URL地址 String path = "http://192.168.1.101:8080/login/login?username=" + name + "&pwd=" + pwd + ""; // [2]准备一个URL对象 URL url = new URL(path); // [3]打开一个连接通道 HttpURLConnection connection = (HttpURLConnection) url.openConnection(); // [4]设置连接超时时间 connection.setConnectTimeout(5000); // [5]设置请求方式 connection.setRequestMethod("GET"); // [6]发送请求 获取响应码 int responseCode = connection.getResponseCode(); // [7]判断响应码 如果==200说明请求成功了 if (responseCode == HttpURLConnection.HTTP_OK) { // [8]获取服务端返回的数据流 InputStream inputStream = connection.getInputStream(); // [9]将inputStream转成String String content = parserStream(inputStream); // [10]将服务端返回的内容显示到Toast上面 showToast(content); }
parserStream代码
protected String parserStream(InputStream inputStream) throws IOException { ByteArrayOutputStream bops = new ByteArrayOutputStream(); int len = 0; byte[] buffer = new byte[1024]; while ((len = inputStream.read(buffer)) != -1) { bops.write(buffer, 0, len); } return new String(bops.toByteArray()); }
showToast代码
protected void showToast(final String content) { runOnUiThread(new Runnable() { @Override public void run() { Toast.makeText(MainActivity.this, content, 0).show(); } });}
使用HttpUrlConnection post方式提交数据给服务器
[1]开启输出流setDoOutput(true) 默认是falsesetDoInput(true) 默认是true[2]在默认时候,Http是直接将流发送给服务器的,如果在执行大文件上传时,如果没有控制流,很容易OOM。[2.1]如果你知道流的大小 那么使用这个方法传入流的大小 这种方式是一次性传输完成 容易oomsetFixedLengthStreamingMode(输出流的固定长度);[2.2]如果你不知道流的大小,那么你可以指定底层使用byte缓冲区的方式发送,默认是1024setChunkedStreamingMode();参数之间是不能带空格的,否则会报错
Get请求代码
// [1]拼接URL地址 String path = "http://192.168.1.101:8080/login/login"; // [2]准备一个URL对象 URL url = new URL(path); // [3]打开一个连接通道 HttpURLConnection connection = (HttpURLConnection) url.openConnection(); // [4]设置连接超时时间 connection.setConnectTimeout(5000);
下列代码与Get请求不同 --------
// [5]设置请求方式 connection.setRequestMethod("POST"); // [6]设置传输模式 这里采用的是分块传输 connection.setChunkedStreamingMode(0); // [7]获取输出流 OutputStream ops = connection.getOutputStream(); // [8]拼接请求体 String data = "username=" + name + "&pwd=" + pwd + ""; // [9]利用输出流把请求体的内容发送到服务端 ops.write(data.getBytes());
上列代码与Get请求不同 --------
// [10]发送请求 获取响应码 int responseCode = connection.getResponseCode(); // [11]判断响应码 如果==200说明请求成功了 if (responseCode == HttpURLConnection.HTTP_OK) { // [12]获取服务端返回的数据流 InputStream inputStream = connection.getInputStream(); // [13]将inputStream转成String String content = parserStream(inputStream); // [14]将服务端返回的内容显示到Toast上面 showToast(content); }
乱码问题解决
乱码的原因:1.服务端响应有中文: [1]android的默认编码是utf-8 [2]非android端String getbytes的编码如果没有指定。那就采用平台默认编码,这个平台默认编码是文件的编码方式2.客户端请求有中文 [1]android端需要对参数进行url编码 get和post都需要对参数进行url编码 "username=" + URLEncoder.encode(name, "utf-8") + "&pwd=" + URLEncoder.encode(pwd, "utf-8") + "" [2]服务端需要对接收的参数进行重新编码 String name = request.getParameter("username"); name = URLEncoder.encode(name, "iso-8859-1"); name = URLDecoder.decode(name, "utf-8");
使用HtppClient Get方式提交数据给服务器
[1]HttpClient是一个开源项目,谷歌把这个开源项目封装到了Android里面[2]使用这个项目引入第三方jar包[3]Httpclient是一个接口,不可以直接new,要找他的子类
Get请求
// [1]拼接URL地址String path = "http://192.168.1.104:8080/login/login?username=" + URLEncoder.encode(name, "utf-8") + "&pwd=" + URLEncoder.encode(pwd, "utf-8") + "";// [2]创建一个HttpClient对象DefaultHttpClient client = new DefaultHttpClient();// [3]创建一个HttpGet请求对象HttpGet get = new HttpGet(path);// [4]把HttpGet对象给HttpClient执行 会返回一个HttpResponseHttpResponse response = client.execute(get);// [5]通过HttpResponse获取返回状态码int statusCode = response.getStatusLine().getStatusCode();// [6]判断返回状态码if (statusCode == HttpStatus.SC_OK) { // [7]通过HttpResponse获取输入流 InputStream inputStream = response.getEntity().getContent(); // [8]解析输入流的内容转成String String content = parserStream(inputStream); // [9]将内容展示到Toast上面去 showToast(content);}
使用HtppClient Post方式提交数据给服务器
HttpClient Post请求
// [1]拼接URL地址String path = "http://192.168.1.104:8080/login/login";// [2]创建一个HttpClient对象DefaultHttpClient client = new DefaultHttpClient();// [3]创建一个HttpPost请求对象HttpPost post = new HttpPost(path);
下列代码与Get请求不同
// [4]创建一个List对象封装 请求体里面的参数List parameters = new ArrayList();BasicNameValuePair nameValuePair = new BasicNameValuePair("username