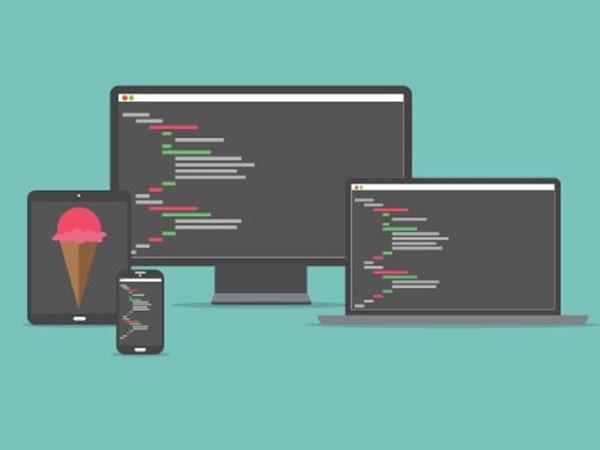
在本文中,您将了解如何正确构造和格式化python程序。
Python语句,通常解释器逐行(即顺序)读取和执行语句。不过,有些语句可以改变这种行为,比如条件语句。
大多数情况下,python语句都是以这样一种格式编写的:一条语句只写在一行中。解释器将“换行符”视为一条指令的终止符。但是,您也可以在下面找到每行写多个语句的方法。
示例:
# Example 1 print('Welcome to Geeks for Geeks')
输出:
Welcome to Geeks for Geeks
# Example 2 x = [1, 2, 3, 4] # x[1:3] means that start from the index # 1 and go upto the index 2 print(x[1:3]) """ In the above mentioned format, the first index is included, but the last index is not included."""
输出:
[2, 3]
每行多条语句
我们也可以每行多条语句,但这不是一个好习惯,因为它会降低代码的可读性。尽量避免在一行中写多个语句。但是,仍然可以通过在“;”的帮助下终止一条语句来编写多行代码。在这种情况下,“;”用作一个语句的终止符。
例如,请考虑以下代码。
# Example a = 10; b = 20; c = b + a print(a); print(b); print(c)
输出:
102030
多行中编写单个语句,避免左右滚动
某些语句可能会变得很长,并可能迫使您频繁向左和向右滚动屏幕。 您可以以无需滚动的方式调整代码。 Python允许您在多行中编写单个语句,也称为行连续。 行的延续也增强了可读性。
# Bad Practice as width of this code is# too much.#codex = 10y = 20z = 30no_of_teachers = xno_of_male_students = yno_of_female_students = zif (no_of_teachers == 10 && no_of_female_students == 30 && no_of_male_students == 20 && (x + y) == 30): print('The course is valid')
行连续的类型
通常,行连续有两种类型。
1)隐式线连续
这是编写跨越多行语句的最直接的技术。
在遇到所有匹配的括号,方括号和花括号之前,任何包含开括号“(”,方括号“ [”或花括号“ {”的语句都被认为是不完整的。语句可以跨行隐式继续而不引起错误。
例如:
# Example 1 # The following code is valid a = [ [1, 2, 3], [3, 4, 5], [5, 6, 7] ] print(a)
输出:
[[1, 2, 3], [3, 4, 5], [5, 6, 7]]
# Example 2 # The following code is also valid person_1 = 18person_2 = 20person_3 = 12 if ( person_1 >= 18 and person_2 >= 18 and person_3 < 18 ): print('2 Persons should have ID Cards')
输出:
2 Persons should have ID Cards
2)显式行继续
显式行联接通常在不适用隐式行联接时使用。在这种方法中,您必须使用一个字符来帮助解释器理解特定的语句跨越多行。
反斜杠()用于指示一条语句跨越多行。需要注意的一点是,”必须是该行的最后一个字符,甚至不允许使用空格。
请参见以下示例
# Example x = 1 + 2 + 5 + 6 + 10 print(x)
输出:
24
Python注释
在代码中编写注释非常重要,它们有助于提高代码的可读性,并提供有关代码的更多信息。它可以帮助您根据语句或代码块编写详细信息。解释器将忽略注释,并且不将其计入命令中。在本部分中,我们将学习如何用Python编写注释。
用于写注释的符号包括哈希(#)或三重双引号(“””)。哈希用于编写不跨越多行的单行注释。三重引号用于编写多行注释。 三个三引号开始注释,并再次三个引号结束注释。
请考虑以下示例:
# Example 1 ####### This example will print Hello World print('Hello World') # This is a comment
# Example 2 """ This example will demonstrate multiple comments """ """ The following a variable contains the string 'How old are you?' """a = 'How old are you?' """ The following statement prints what's inside the variable a """print(a)
请注意,字符串中的哈希(#)不会使其成为注释。考虑以下示例进行演示。
# Example """ The following statement prints the string stored in the variable """ a = 'This is # not a comment #'print(a) # Prints the string stored in a
空格
最常见的空格字符如下:
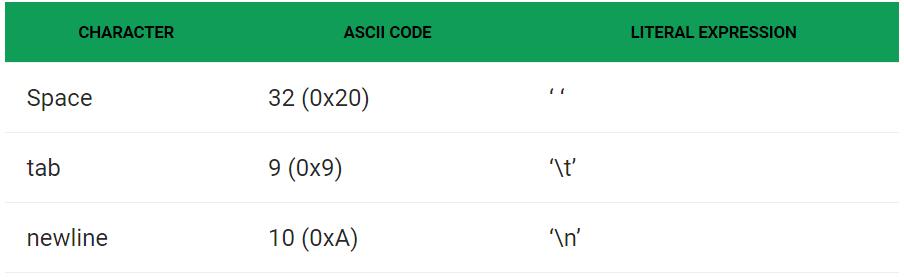
*您可以通过单击此处(http://www.asciitable.com/)来查看ASCII表。
Python解释器通常会忽略空格,并且大多数情况下不需要空格。
示例:
# Example 1 # This is correct but whitespace can improve readability a = 1-2 # Better way is a = 1 - 2 print(a) filter_noneeditplay_arrowbrightness_4# Example 2 # This is correct # Whitespace here can improve readability. x = 10flag =(x == 10)and(x<12) print(flag) """ Readable form could be as follows x = 10 flag = (x == 10) and (x < 12) print(flag) """ # Try the more readable code yourself
在将关键字与变量或其他关键字分开时,必须使用空格。考虑以下示例。
# Example x = [1, 2, 3] y = 2 """ Following is incorrect, and will generate syntax error a = yin x """ # Corrected version is written as a = y in x print(a)
空格作为缩进
Python的语法非常简单,但是在编写代码时仍然要多加注意。缩进用于编写python代码。
语句前的空格具有重要作用,并在缩进中使用。语句前的空白可能具有不同的含义。让我们尝试一个例子。
# Example print('foo') # Correct print('foo') # This will generate an error # The error would be somewhat 'unexpected indent'
前导空格用于确定语句的分组,例如循环或控制结构等。
示例:
# Example x = 10 while(x != 0): if(x > 5): # Line 1 print('x > 5') # Line 2 else: # Line 3 print('x < 5') # Line 4 x -= 2 # Line 5 """ Lines 1, 3, 5 are on same level Line 2 will only be executed if if condition becomes true. Line 4 will only be executed if if condition becomes false. """
输出:
x > 5x > 5x > 5x < 5x < 5