项目已上传码云:小朋友/JavaWeb 错误登记簿(文件上传)
知乎视频www.zhihu.com篇章目录:
- 项目预览与介绍
- 文件上传实现
项目预览与介绍:
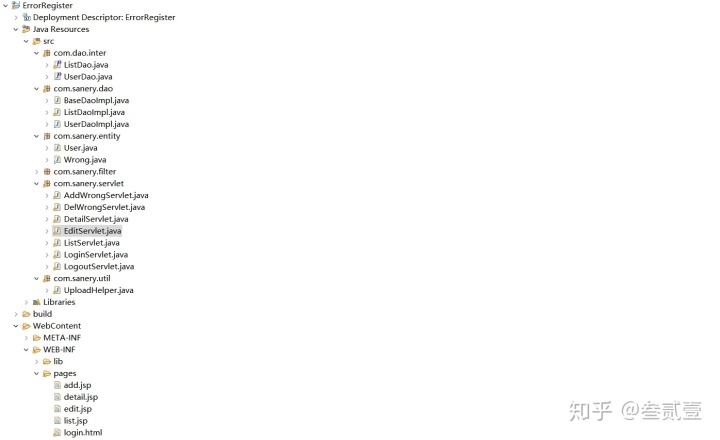
com.dao.inter
这个包放的是接口interface
com.sanery.dao
这个包实现com.dao.inter
里面的接口com.sanery.entity
这个包放的是封装对应数据库两张表的类,users
和wrongs
两张表com.sanery.filter
过滤器,两个过滤器,第一是过滤编码、统一编码为UTF-8
,第二是验证登录、控制访问权限com.sanery.servlet
控制器com.sanery.util
封装的文件上传的类UploadServlet.java
文件上传实现:
- 首先,
from
表单必须设置上传文件,enctype="multipart/form-data"
<!--举例-->
<form action="" method="post" enctype="multipart/form-data">
<input name="photo" type="file"><!--文件类型-->
<input type="submit" value="提交">
</form>
2. 其次,这种格式上传的文件并不能使用 request.getParameter()
, 因为enctype="multipart/form-data"
指定传输数据为二进制类型;必须要使用其他插件( commons-io
下载地址 和 commons-fileupload
下载地址 ) 才能接收此参数
使用fileUpload固定步骤:
1. 创建工厂类:DiskFileItemFactory factory=new DiskFileItemFactory();
2. 创建解析器:ServletFileUpload upload=new ServletFileUpload(factory);
3. 使用解析器解析request对象:List<FileItem> list=upload.parseRequest(request);
一个FileItem对象对应一个表单项。FileItem类有如下方法:
String getFieldName()
:获取表单项的name的属性值。String getName()
:获取文件字段的文件名。如果是普通字段,则返回nullString getString()
:获取字段的内容。如果是普通字段,则是它的value值;如果是文件字段,则是文件内容。String getContentType()
:获取上传的文件类型,例如text/plain、image。如果是普通字段,则返回null。long getSize()
:获取字段内容的大小,单位是字节。boolean isFormField()
:判断是否是普通表单字段,若是,返回true,否则返回false。InputStream getInputStream()
:获得文件内容的输入流。如果是普通字段,则返回value值的输入流。
但是,下面封装的 UploadHelper.java
已经封装好了
try {
String prjdir = request.getServletContext().getRealPath("/"); // D:APPJavaapache-tomcat-8.5.11webappsErrorRegister
String dir = new File(prjdir).getParent() + "imagesdir"; // D:APPJavaapache-tomcat-8.5.11webappsimagesdir
Map<String, String> map = UploadHelper.upload2(request, dir, 10485760, ".jpg.png.gif.bmp");
list.add(map.get("title"));
list.add(map.get("img"));
list.add(map.get("answer"));
list.add(map.get("tip"));
list.add(map.get("dealtype"));
} catch (Exception e) {
e.printStackTrace();
}
调用 upload2
方法后,会返回 Map 集合,根据对应 JSP 中 <form>
标签 对应的name值为key值获取对应的value值即可。
3. 最后,封装的 UploadHelper.java
import java.io.File;
import java.util.Date;
import java.util.HashMap;
import java.util.List;
import java.util.Map;
import javax.servlet.http.HttpServletRequest;
import org.apache.commons.fileupload.FileItem;
import org.apache.commons.fileupload.disk.DiskFileItemFactory;
import org.apache.commons.fileupload.servlet.ServletFileUpload;
public class UploadHelper {
public static Map<String, Object> upload(HttpServletRequest request, long max, String allowtype) throws Exception {
Map<String, Object> map = new HashMap<String, Object>();
request.setCharacterEncoding("utf-8");
if (ServletFileUpload.isMultipartContent(request)) {
DiskFileItemFactory fty = new DiskFileItemFactory();
// fty.setRepository(new File("D:uploadtmp"));
// fty.setSizeThreshold(4*1024);
ServletFileUpload upload = new ServletFileUpload(fty);// 上传核心组件
if (max > 0)
upload.setSizeMax(max);
List<FileItem> items = upload.parseRequest(request);// 分解上传上来的东西
for (FileItem item : items) {
if (item.isFormField())
map.put(item.getFieldName(), item.getString("utf-8"));
else {
String fname = item.getName();
if (fname == null || fname.isEmpty())
continue;
String ext = fname.substring(fname.lastIndexOf('.') + 1);// 那他的后缀名
if (allowtype != null && allowtype.indexOf(ext) == -1)
throw new RuntimeException("不符合上传格式");
byte[] bytes = item.get();
item.delete();
map.put(item.getFieldName(), bytes);// 返回二进制数组
map.put("filename", fname);
}
}
}
return map;
}
public static Map<String, String> upload(HttpServletRequest request, String dirpath// 文件保存到哪里去
, int max// 不能超过多大
, String allowtype// 允许什么类型
) throws Exception {
Map<String, String> map = new HashMap<String, String>();
String dir = request.getSession().getServletContext().getRealPath(dirpath); // /imgs-->物理路径
request.setCharacterEncoding("utf-8");
if (ServletFileUpload.isMultipartContent(request)) {
DiskFileItemFactory fty = new DiskFileItemFactory();
// fty.setRepository(new File("D:uploadtmp"));
// fty.setSizeThreshold(4*1024);
ServletFileUpload upload = new ServletFileUpload(fty);// 上传核心组件
upload.setSizeMax(max);// 设置最大大小
List<FileItem> items = upload.parseRequest(request);// 分解上传上来的东西
for (FileItem item : items) {
if (item.isFormField())
map.put(item.getFieldName(), item.getString("utf-8"));
else {
String fname = item.getName();
if (fname == null || fname.isEmpty())
continue;
String ext = fname.substring(fname.lastIndexOf('.') + 1);// 那他的后缀名
if (allowtype.indexOf(ext) == -1)
throw new RuntimeException("不符合图片格式");
String filename = new Date().getTime() + "." + ext;
item.write(new File(dir + "/" + filename));
byte[] imgByte = item.get();
item.delete();// 清空临时文件夹(过渡用的)中的数据
map.put(item.getFieldName(), filename);// 把处理好的文件名放字典,后面要用到
}
}
}
return map;
}
public static Map<String, String> upload2(HttpServletRequest request, String dir// 文件保存的绝对路径
, int max// 不能超过多大
, String allowtype// 允许什么类型
) throws Exception {
Map<String, String> map = new HashMap<String, String>();
request.setCharacterEncoding("utf-8");
if (ServletFileUpload.isMultipartContent(request))// 校验一下是不是对应的格式
{
DiskFileItemFactory fty = new DiskFileItemFactory();
// fty.setRepository(new File("D:uploadtmp"));
// fty.setSizeThreshold(4*1024);
ServletFileUpload upload = new ServletFileUpload(fty);// 上传核心组件
upload.setSizeMax(max);// 设置最大大小
List<FileItem> items = upload.parseRequest(request);// 分解上传上来的东西
for (FileItem item : items) {
if (item.isFormField())// 是不是普通的文本,等效于request.getParameter("")
map.put(item.getFieldName(), item.getString("utf-8"));
else {
String fname = item.getName();
if (fname == null || fname.isEmpty())
continue;
String ext = fname.substring(fname.lastIndexOf('.') + 1);// 拿他的后缀名.jpg,.png
if (allowtype != null && allowtype.indexOf(ext) == -1)
throw new RuntimeException("不符合图片格式");
String filename = new Date().getTime() + "." + ext;
item.write(new File(dir + "/" + filename));
// byte[] imgByte=item.get();
item.delete();// 清空临时文件夹(过渡用的)中的数据
map.put(item.getFieldName(), filename);// 把处理好的文件名放字典,后面要用到
}
}
}
return map;
}
}
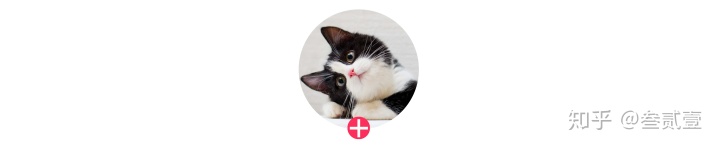
CSDN:https://blog.csdn.net/weixin_43148062
简书:https://www.jianshu.com/u/45339cbb7573