先拿出我学习java的小秘籍——java学习小册子,每段学习的过程中总离不开学习文档,只有实践+理论相结合才能事半功倍,所以我谈谈我的一些感受吧
java学习小册子note.youdao.com1.json转换工具
1. package com.taotao.utils;
3. import java.util.List;
5. import com.fasterxml.jackson.core.JsonProcessingException;
6. import com.fasterxml.jackson.databind.JavaType;
7. import com.fasterxml.jackson.databind.JsonNode;
8. import com.fasterxml.jackson.databind.ObjectMapper;
10. /**
11. * json转换工具类
12. */
13. public class JsonUtils {
15. // 定义jackson对象
16. private static final ObjectMapper MAPPER = new ObjectMapper();
18. /**
19. * 将对象转换成json字符串。
20. * <p>Title: pojoToJson</p>
21. * <p>Description: </p>
22. * @param data
23. * @return
24. */
25. public static String objectToJson(Object data) {
26. try {
27. String string = MAPPER.writeValueAsString(data);
28. return string;
29. } catch (JsonProcessingException e) {
30. e.printStackTrace();
31. }
32. return null;
33. }
35. /**
36. * 将json结果集转化为对象
37. *
38. * @param jsonData json数据
39. * @param clazz 对象中的object类型
40. * @return
41. */
42. public static <T> T jsonToPojo(String jsonData, Class<T> beanType) {
43. try {
44. T t = MAPPER.readValue(jsonData, beanType);
45. return t;
46. } catch (Exception e) {
47. e.printStackTrace();
48. }
49. return null;
50. }
52. /**
53. * 将json数据转换成pojo对象list
54. * <p>Title: jsonToList</p>
55. * <p>Description: </p>
56. * @param jsonData
57. * @param beanType
58. * @return
59. */
60. public static <T>List<T> jsonToList(String jsonData, Class<T> beanType) {
61. JavaType javaType = MAPPER.getTypeFactory().constructParametricType(List.class, beanType);
62. try {
63. List<T> list = MAPPER.readValue(jsonData, javaType);
64. return list;
65. } catch (Exception e) {
66. e.printStackTrace();
67. }
69. return null;
70. }
72. }
2.cookie的读写
1. package com.taotao.common.utils;
3. import java.io.UnsupportedEncodingException;
4. import java.net.URLDecoder;
5. import java.net.URLEncoder;
7. import javax.servlet.http.Cookie;
8. import javax.servlet.http.HttpServletRequest;
9. import javax.servlet.http.HttpServletResponse;
12. /**
13. *
14. * Cookie 工具类
15. *
16. */
17. public final class CookieUtils {
19. /**
20. * 得到Cookie的值, 不编码
21. *
22. * @param request
23. * @param cookieName
24. * @return
25. */
26. public static String getCookieValue(HttpServletRequest request, String cookieName) {
27. return getCookieValue(request, cookieName, false);
28. }
30. /**
31. * 得到Cookie的值,
32. *
33. * @param request
34. * @param cookieName
35. * @return
36. */
37. public static String getCookieValue(HttpServletRequest request, String cookieName, boolean isDecoder) {
38. Cookie[] cookieList = request.getCookies();
39. if (cookieList == null || cookieName == null) {
40. return null;
41. }
42. String retValue = null;
43. try {
44. for (int i = 0; i < cookieList.length; i++) {
45. if (cookieList[i].getName().equals(cookieName)) {
46. if (isDecoder) {
47. retValue = URLDecoder.decode(cookieList[i].getValue(), "UTF-8");
48. } else {
49. retValue = cookieList[i].getValue();
50. }
51. break;
52. }
53. }
54. } catch (UnsupportedEncodingException e) {
55. e.printStackTrace();
56. }
57. return retValue;
58. }
60. /**
61. * 得到Cookie的值,
62. *
63. * @param request
64. * @param cookieName
65. * @return
66. */
67. public static String getCookieValue(HttpServletRequest request, String cookieName, String encodeString) {
68. Cookie[] cookieList = request.getCookies();
69. if (cookieList == null || cookieName == null) {
70. return null;
71. }
72. String retValue = null;
73. try {
74. for (int i = 0; i < cookieList.length; i++) {
75. if (cookieList[i].getName().equals(cookieName)) {
76. retValue = URLDecoder.decode(cookieList[i].getValue(), encodeString);
77. break;
78. }
79. }
80. } catch (UnsupportedEncodingException e) {
81. e.printStackTrace();
82. }
83. return retValue;
84. }
86. /**
87. * 设置Cookie的值 不设置生效时间默认浏览器关闭即失效,也不编码
88. */
89. public static void setCookie(HttpServletRequest request, HttpServletResponse response, String cookieName,
90. String cookieValue) {
91. setCookie(request, response, cookieName, cookieValue, -1);
92. }
94. /**
95. * 设置Cookie的值 在指定时间内生效,但不编码
96. */
97. public static void setCookie(HttpServletRequest request, HttpServletResponse response, String cookieName,
98. String cookieValue, int cookieMaxage) {
99. setCookie(request, response, cookieName, cookieValue, cookieMaxage, false);
100. }
102. /**
103. * 设置Cookie的值 不设置生效时间,但编码
104. */
105. public static void setCookie(HttpServletRequest request, HttpServletResponse response, String cookieName,
106. String cookieValue, boolean isEncode) {
107. setCookie(request, response, cookieName, cookieValue, -1, isEncode);
108. }
110. /**
111. * 设置Cookie的值 在指定时间内生效, 编码参数
112. */
113. public static void setCookie(HttpServletRequest request, HttpServletResponse response, String cookieName,
114. String cookieValue, int cookieMaxage, boolean isEncode) {
115. doSetCookie(request, response, cookieName, cookieValue, cookieMaxage, isEncode);
116. }
118. /**
119. * 设置Cookie的值 在指定时间内生效, 编码参数(指定编码)
120. */
121. public static void setCookie(HttpServletRequest request, HttpServletResponse response, String cookieName,
122. String cookieValue, int cookieMaxage, String encodeString) {
123. doSetCookie(request, response, cookieName, cookieValue, cookieMaxage, encodeString);
124. }
126. /**
127. * 删除Cookie带cookie域名
128. */
129. public static void deleteCookie(HttpServletRequest request, HttpServletResponse response,
130. String cookieName) {
131. doSetCookie(request, response, cookieName, "", -1, false);
132. }
134. /**
135. * 设置Cookie的值,并使其在指定时间内生效
136. *
137. * @param cookieMaxage cookie生效的最大秒数
138. */
139. private static final void doSetCookie(HttpServletRequest request, HttpServletResponse response,
140. String cookieName, String cookieValue, int cookieMaxage, boolean isEncode) {
141. try {
142. if (cookieValue == null) {
143. cookieValue = "";
144. } else if (isEncode) {
145. cookieValue = URLEncoder.encode(cookieValue, "utf-8");
146. }
147. Cookie cookie = new Cookie(cookieName, cookieValue);
148. if (cookieMaxage > 0)
149. cookie.setMaxAge(cookieMaxage);
150. if (null != request) {// 设置域名的cookie
151. String domainName = getDomainName(request);
152. System.out.println(domainName);
153. if (!"localhost".equals(domainName)) {
154. cookie.setDomain(domainName);
155. }
156. }
157. cookie.setPath("/");
158. response.addCookie(cookie);
159. } catch (Exception e) {
160. e.printStackTrace();
161. }
162. }
164. /**
165. * 设置Cookie的值,并使其在指定时间内生效
166. *
167. * @param cookieMaxage cookie生效的最大秒数
168. */
169. private static final void doSetCookie(HttpServletRequest request, HttpServletResponse response,
170. String cookieName, String cookieValue, int cookieMaxage, String encodeString) {
171. try {
172. if (cookieValue == null) {
173. cookieValue = "";
174. } else {
175. cookieValue = URLEncoder.encode(cookieValue, encodeString);
176. }
177. Cookie cookie = new Cookie(cookieName, cookieValue);
178. if (cookieMaxage > 0)
179. cookie.setMaxAge(cookieMaxage);
180. if (null != request) {// 设置域名的cookie
181. String domainName = getDomainName(request);
182. System.out.println(domainName);
183. if (!"localhost".equals(domainName)) {
184. cookie.setDomain(domainName);
185. }
186. }
187. cookie.setPath("/");
188. response.addCookie(cookie);
189. } catch (Exception e) {
190. e.printStackTrace();
191. }
192. }
194. /**
195. * 得到cookie的域名
196. */
197. private static final String getDomainName(HttpServletRequest request) {
198. String domainName = null;
200. String serverName = request.getRequestURL().toString();
201. if (serverName == null || serverName.equals("")) {
202. domainName = "";
203. } else {
204. serverName = serverName.toLowerCase();
205. serverName = serverName.substring(7);
206. final int end = serverName.indexOf("/");
207. serverName = serverName.substring(0, end);
208. final String[] domains = serverName.split(".");
209. int len = domains.length;
210. if (len > 3) {
211. // www.xxx.com.cn
212. domainName = "." + domains[len - 3] + "." + domains[len - 2] + "." + domains[len - 1];
213. } else if (len <= 3 && len > 1) {
214. // xxx.com or xxx.cn
215. domainName = "." + domains[len - 2] + "." + domains[len - 1];
216. } else {
217. domainName = serverName;
218. }
219. }
221. if (domainName != null && domainName.indexOf(":") > 0) {
222. String[] ary = domainName.split(":");
223. domainName = ary[0];
224. }
225. return domainName;
226. }
228. }
分享我的学习方法,学习提升只因为我有它
java学习分享note.youdao.com3.HttpClientUtil
1. package com.taotao.utils;
3. import java.io.IOException;
4. import java.net.URI;
5. import java.util.ArrayList;
6. import java.util.List;
7. import java.util.Map;
9. import org.apache.http.NameValuePair;
10. import org.apache.http.client.entity.UrlEncodedFormEntity;
11. import org.apache.http.client.methods.CloseableHttpResponse;
12. import org.apache.http.client.methods.HttpGet;
13. import org.apache.http.client.methods.HttpPost;
14. import org.apache.http.client.utils.URIBuilder;
15. import org.apache.http.entity.ContentType;
16. import org.apache.http.entity.StringEntity;
17. import org.apache.http.impl.client.CloseableHttpClient;
18. import org.apache.http.impl.client.HttpClients;
19. import org.apache.http.message.BasicNameValuePair;
20. import org.apache.http.util.EntityUtils;
22. public class HttpClientUtil {
24. public static String doGet(String url, Map<String, String> param) {
26. // 创建Httpclient对象
27. CloseableHttpClient httpclient = HttpClients.createDefault();
29. String resultString = "";
30. CloseableHttpResponse response = null;
31. try {
32. // 创建uri
33. URIBuilder builder = new URIBuilder(url);
34. if (param != null) {
35. for (String key : param.keySet()) {
36. builder.addParameter(key, param.get(key));
37. }
38. }
39. URI uri = builder.build();
41. // 创建http GET请求
42. HttpGet httpGet = new HttpGet(uri);
44. // 执行请求
45. response = httpclient.execute(httpGet);
46. // 判断返回状态是否为200
47. if (response.getStatusLine().getStatusCode() == 200) {
48. resultString = EntityUtils.toString(response.getEntity(), "UTF-8");
49. }
50. } catch (Exception e) {
51. e.printStackTrace();
52. } finally {
53. try {
54. if (response != null) {
55. response.close();
56. }
57. httpclient.close();
58. } catch (IOException e) {
59. e.printStackTrace();
60. }
61. }
62. return resultString;
63. }
65. public static String doGet(String url) {
66. return doGet(url, null);
67. }
69. public static String doPost(String url, Map<String, String> param) {
70. // 创建Httpclient对象
71. CloseableHttpClient httpClient = HttpClients.createDefault();
72. CloseableHttpResponse response = null;
73. String resultString = "";
74. try {
75. // 创建Http Post请求
76. HttpPost httpPost = new HttpPost(url);
77. // 创建参数列表
78. if (param != null) {
79. List<NameValuePair> paramList = new ArrayList<>();
80. for (String key : param.keySet()) {
81. paramList.add(new BasicNameValuePair(key, param.get(key)));
82. }
83. // 模拟表单
84. UrlEncodedFormEntity entity = new UrlEncodedFormEntity(paramList);
85. httpPost.setEntity(entity);
86. }
87. // 执行http请求
88. response = httpClient.execute(httpPost);
89. resultString = EntityUtils.toString(response.getEntity(), "utf-8");
90. } catch (Exception e) {
91. e.printStackTrace();
92. } finally {
93. try {
94. response.close();
95. } catch (IOException e) {
96. // TODO Auto-generated catch block
97. e.printStackTrace();
98. }
99. }
101. return resultString;
102. }
104. public static String doPost(String url) {
105. return doPost(url, null);
106. }
108. public static String doPostJson(String url, String json) {
109. // 创建Httpclient对象
110. CloseableHttpClient httpClient = HttpClients.createDefault();
111. CloseableHttpResponse response = null;
112. String resultString = "";
113. try {
114. // 创建Http Post请求
115. HttpPost httpPost = new HttpPost(url);
116. // 创建请求内容
117. StringEntity entity = new StringEntity(json, ContentType.APPLICATION_JSON);
118. httpPost.setEntity(entity);
119. // 执行http请求
120. response = httpClient.execute(httpPost);
121. resultString = EntityUtils.toString(response.getEntity(), "utf-8");
122. } catch (Exception e) {
123. e.printStackTrace();
124. } finally {
125. try {
126. response.close();
127. } catch (IOException e) {
128. // TODO Auto-generated catch block
129. e.printStackTrace();
130. }
131. }
133. return resultString;
134. }
135. }
4.FastDFSClient工具类
1. package cn.itcast.fastdfs.cliennt;
3. import org.csource.common.NameValuePair;
4. import org.csource.fastdfs.ClientGlobal;
5. import org.csource.fastdfs.StorageClient1;
6. import org.csource.fastdfs.StorageServer;
7. import org.csource.fastdfs.TrackerClient;
8. import org.csource.fastdfs.TrackerServer;
10. public class FastDFSClient {
12. private TrackerClient trackerClient = null;
13. private TrackerServer trackerServer = null;
14. private StorageServer storageServer = null;
15. private StorageClient1 storageClient = null;
17. public FastDFSClient(String conf) throws Exception {
18. if (conf.contains("classpath:")) {
19. conf = conf.replace("classpath:", this.getClass().getResource("/").getPath());
20. }
21. ClientGlobal.init(conf);
22. trackerClient = new TrackerClient();
23. trackerServer = trackerClient.getConnection();
24. storageServer = null;
25. storageClient = new StorageClient1(trackerServer, storageServer);
26. }
28. /**
29. * 上传文件方法
30. * <p>Title: uploadFile</p>
31. * <p>Description: </p>
32. * @param fileName 文件全路径
33. * @param extName 文件扩展名,不包含(.)
34. * @param metas 文件扩展信息
35. * @return
36. * @throws Exception
37. */
38. public String uploadFile(String fileName, String extName, NameValuePair[] metas) throws Exception {
39. String result = storageClient.upload_file1(fileName, extName, metas);
40. return result;
41. }
43. public String uploadFile(String fileName) throws Exception {
44. return uploadFile(fileName, null, null);
45. }
47. public String uploadFile(String fileName, String extName) throws Exception {
48. return uploadFile(fileName, extName, null);
49. }
51. /**
52. * 上传文件方法
53. * <p>Title: uploadFile</p>
54. * <p>Description: </p>
55. * @param fileContent 文件的内容,字节数组
56. * @param extName 文件扩展名
57. * @param metas 文件扩展信息
58. * @return
59. * @throws Exception
60. */
61. public String uploadFile(byte[] fileContent, String extName, NameValuePair[] metas) throws Exception {
63. String result = storageClient.upload_file1(fileContent, extName, metas);
64. return result;
65. }
67. public String uploadFile(byte[] fileContent) throws Exception {
68. return uploadFile(fileContent, null, null);
69. }
71. public String uploadFile(byte[] fileContent, String extName) throws Exception {
72. return uploadFile(fileContent, extName, null);
73. }
74. }
1. <span style="font-size:14px;font-weight:normal;">public class FastDFSTest {
3. @Test
4. public void testFileUpload() throws Exception {
5. // 1、加载配置文件,配置文件中的内容就是tracker服务的地址。
6. ClientGlobal.init("D:/workspaces-itcast/term197/taotao-manager-web/src/main/resources/resource/client.conf");
7. // 2、创建一个TrackerClient对象。直接new一个。
8. TrackerClient trackerClient = new TrackerClient();
9. // 3、使用TrackerClient对象创建连接,获得一个TrackerServer对象。
10. TrackerServer trackerServer = trackerClient.getConnection();
11. // 4、创建一个StorageServer的引用,值为null
12. StorageServer storageServer = null;
13. // 5、创建一个StorageClient对象,需要两个参数TrackerServer对象、StorageServer的引用
14. StorageClient storageClient = new StorageClient(trackerServer, storageServer);
15. // 6、使用StorageClient对象上传图片。
16. //扩展名不带“.”
17. String[] strings = storageClient.upload_file("D:/Documents/Pictures/images/200811281555127886.jpg", "jpg", null);
18. // 7、返回数组。包含组名和图片的路径。
19. for (String string : strings) {
20. System.out.println(string);
21. }
22. }
23. }</span>
# 
5.获取异常的堆栈信息
1. package com.taotao.utils;
3. import java.io.PrintWriter;
4. import java.io.StringWriter;
6. public class ExceptionUtil {
8. /**
9. * 获取异常的堆栈信息
10. *
11. * @param t
12. * @return
13. */
14. public static String getStackTrace(Throwable t) {
15. StringWriter sw = new StringWriter();
16. PrintWriter pw = new PrintWriter(sw);
18. try {
19. t.printStackTrace(pw);
20. return sw.toString();
21. } finally {
22. pw.close();
23. }
24. }
25. }
#6.easyUIDataGrid对象返回值
1. package com.taotao.result;
3. import java.util.List;
5. /**
6. * easyUIDataGrid对象返回值
7. * <p>Title: EasyUIResult</p>
8. * <p>Description: </p>
9. * <p>Company: www.itcast.com</p>
10. * @author 入云龙
11. * @date 2015年7月21日下午4:12:52
12. * @version 1.0
13. */
14. public class EasyUIResult {
16. private Integer total;
18. private List<?> rows;
20. public EasyUIResult(Integer total, List<?> rows) {
21. this.total = total;
22. this.rows = rows;
23. }
25. public EasyUIResult(long total, List<?> rows) {
26. this.total = (int) total;
27. this.rows = rows;
28. }
30. public Integer getTotal() {
31. return total;
32. }
33. public void setTotal(Integer total) {
34. this.total = total;
35. }
36. public List<?> getRows() {
37. return rows;
38. }
39. public void setRows(List<?> rows) {
40. this.rows = rows;
41. }
44. }
7.ftp上传下载工具类
1. package com.taotao.utils;
3. import java.io.File;
4. import java.io.FileInputStream;
5. import java.io.FileNotFoundException;
6. import java.io.FileOutputStream;
7. import java.io.IOException;
8. import java.io.InputStream;
9. import java.io.OutputStream;
11. import org.apache.commons.net.ftp.FTP;
12. import org.apache.commons.net.ftp.FTPClient;
13. import org.apache.commons.net.ftp.FTPFile;
14. import org.apache.commons.net.ftp.FTPReply;
16. /**
17. * ftp上传下载工具类
18. * <p>Title: FtpUtil</p>
19. * <p>Description: </p>
20. * <p>Company: www.itcast.com</p>
21. * @author 入云龙
22. * @date 2015年7月29日下午8:11:51
23. * @version 1.0
24. */
25. public class FtpUtil {
27. /**
28. * Description: 向FTP服务器上传文件
29. * @param host FTP服务器hostname
30. * @param port FTP服务器端口
31. * @param username FTP登录账号
32. * @param password FTP登录密码
33. * @param basePath FTP服务器基础目录
34. * @param filePath FTP服务器文件存放路径。例如分日期存放:/2015/01/01。文件的路径为basePath+filePath
35. * @param filename 上传到FTP服务器上的文件名
36. * @param input 输入流
37. * @return 成功返回true,否则返回false
38. */
39. public static boolean uploadFile(String host, int port, String username, String password, String basePath,
40. String filePath, String filename, InputStream input) {
41. boolean result = false;
42. FTPClient ftp = new FTPClient();
43. try {
44. int reply;
45. ftp.connect(host, port);// 连接FTP服务器
46. // 如果采用默认端口,可以使用ftp.connect(host)的方式直接连接FTP服务器
47. ftp.login(username, password);// 登录
48. reply = ftp.getReplyCode();
49. if (!FTPReply.isPositiveCompletion(reply)) {
50. ftp.disconnect();
51. return result;
52. }
53. //切换到上传目录
54. if (!ftp.changeWorkingDirectory(basePath+filePath)) {
55. //如果目录不存在创建目录
56. String[] dirs = filePath.split("/");
57. String tempPath = basePath;
58. for (String dir : dirs) {
59. if (null == dir || "".equals(dir)) continue;
60. tempPath += "/" + dir;
61. if (!ftp.changeWorkingDirectory(tempPath)) {
62. if (!ftp.makeDirectory(tempPath)) {
63. return result;
64. } else {
65. ftp.changeWorkingDirectory(tempPath);
66. }
67. }
68. }
69. }
70. //设置上传文件的类型为二进制类型
71. ftp.setFileType(FTP.BINARY_FILE_TYPE);
72. //上传文件
73. if (!ftp.storeFile(filename, input)) {
74. return result;
75. }
76. input.close();
77. ftp.logout();
78. result = true;
79. } catch (IOException e) {
80. e.printStackTrace();
81. } finally {
82. if (ftp.isConnected()) {
83. try {
84. ftp.disconnect();
85. } catch (IOException ioe) {
86. }
87. }
88. }
89. return result;
90. }
92. /**
93. * Description: 从FTP服务器下载文件
94. * @param host FTP服务器hostname
95. * @param port FTP服务器端口
96. * @param username FTP登录账号
97. * @param password FTP登录密码
98. * @param remotePath FTP服务器上的相对路径
99. * @param fileName 要下载的文件名
100. * @param localPath 下载后保存到本地的路径
101. * @return
102. */
103. public static boolean downloadFile(String host, int port, String username, String password, String remotePath,
104. String fileName, String localPath) {
105. boolean result = false;
106. FTPClient ftp = new FTPClient();
107. try {
108. int reply;
109. ftp.connect(host, port);
110. // 如果采用默认端口,可以使用ftp.connect(host)的方式直接连接FTP服务器
111. ftp.login(username, password);// 登录
112. reply = ftp.getReplyCode();
113. if (!FTPReply.isPositiveCompletion(reply)) {
114. ftp.disconnect();
115. return result;
116. }
117. ftp.changeWorkingDirectory(remotePath);// 转移到FTP服务器目录
118. FTPFile[] fs = ftp.listFiles();
119. for (FTPFile ff : fs) {
120. if (ff.getName().equals(fileName)) {
121. File localFile = new File(localPath + "/" + ff.getName());
123. OutputStream is = new FileOutputStream(localFile);
124. ftp.retrieveFile(ff.getName(), is);
125. is.close();
126. }
127. }
129. ftp.logout();
130. result = true;
131. } catch (IOException e) {
132. e.printStackTrace();
133. } finally {
134. if (ftp.isConnected()) {
135. try {
136. ftp.disconnect();
137. } catch (IOException ioe) {
138. }
139. }
140. }
141. return result;
142. }
144. public static void main(String[] args) {
145. try {
146. FileInputStream in=new FileInputStream(new File("D:tempimagegaigeming.jpg"));
147. boolean flag = uploadFile("192.168.25.133", 21, "ftpuser", "ftpuser", "/home/ftpuser/www/images","/2015/01/21", "gaigeming.jpg", in);
148. System.out.println(flag);
149. } catch (FileNotFoundException e) {
150. e.printStackTrace();
151. }
152. }
153. }
8.各种id生成策略
1. package com.taotao.utils;
3. import java.util.Random;
5. /**
6. * 各种id生成策略
7. * <p>Title: IDUtils</p>
8. * <p>Description: </p>
9. * @date 2015年7月22日下午2:32:10
10. * @version 1.0
11. */
12. public class IDUtils {
14. /**
15. * 图片名生成
16. */
17. public static String genImageName() {
18. //取当前时间的长整形值包含毫秒
19. long millis = System.currentTimeMillis();
20. //long millis = System.nanoTime();
21. //加上三位随机数
22. Random random = new Random();
23. int end3 = random.nextInt(999);
24. //如果不足三位前面补0
25. String str = millis + String.format("%03d", end3);
27. return str;
28. }
30. /**
31. * 商品id生成
32. */
33. public static long genItemId() {
34. //取当前时间的长整形值包含毫秒
35. long millis = System.currentTimeMillis();
36. //long millis = System.nanoTime();
37. //加上两位随机数
38. Random random = new Random();
39. int end2 = random.nextInt(99);
40. //如果不足两位前面补0
41. String str = millis + String.format("%02d", end2);
42. long id = new Long(str);
43. return id;
44. }
46. public static void main(String[] args) {
47. for(int i=0;i< 100;i++)
48. System.out.println(genItemId());
49. }
50. }
##9.上传图片返回值
1. package com.result;
2. /**
3. * 上传图片返回值
4. * <p>Title: PictureResult</p>
5. * <p>Description: </p>
6. * <p>Company: www.itcast.com</p>
7. * @author 入云龙
8. * @date 2015年7月22日下午2:09:02
9. * @version 1.0
10. */
11. public class PictureResult {
13. /**
14. * 上传图片返回值,成功:0 失败:1
15. */
16. private Integer error;
17. /**
18. * 回显图片使用的url
19. */
20. private String url;
21. /**
22. * 错误时的错误消息
23. */
24. private String message;
25. public PictureResult(Integer state, String url) {
26. this.url = url;
27. this.error = state;
28. }
29. public PictureResult(Integer state, String url, String errorMessage) {
30. this.url = url;
31. this.error = state;
32. this.message = errorMessage;
33. }
34. public Integer getError() {
35. return error;
36. }
37. public void setError(Integer error) {
38. this.error = error;
39. }
40. public String getUrl() {
41. return url;
42. }
43. public void setUrl(String url) {
44. this.url = url;
45. }
46. public String getMessage() {
47. return message;
48. }
49. public void setMessage(String message) {
50. this.message = message;
51. }
53. }
10.自定义响应结构
1. package com.result;
3. import java.util.List;
5. import com.fasterxml.jackson.databind.JsonNode;
6. import com.fasterxml.jackson.databind.ObjectMapper;
8. /**
9. * 自定义响应结构
10. */
11. public class TaotaoResult {
13. // 定义jackson对象
14. private static final ObjectMapper MAPPER = new ObjectMapper();
16. // 响应业务状态
17. private Integer status;
19. // 响应消息
20. private String msg;
22. // 响应中的数据
23. private Object data;
25. public static TaotaoResult build(Integer status, String msg, Object data) {
26. return new TaotaoResult(status, msg, data);
27. }
29. public static TaotaoResult ok(Object data) {
30. return new TaotaoResult(data);
31. }
33. public static TaotaoResult ok() {
34. return new TaotaoResult(null);
35. }
37. public TaotaoResult() {
39. }
41. public static TaotaoResult build(Integer status, String msg) {
42. return new TaotaoResult(status, msg, null);
43. }
45. public TaotaoResult(Integer status, String msg, Object data) {
46. this.status = status;
47. this.msg = msg;
48. this.data = data;
49. }
51. public TaotaoResult(Object data) {
52. this.status = 200;
53. this.msg = "OK";
54. this.data = data;
55. }
57. // public Boolean isOK() {
58. // return this.status == 200;
59. // }
61. public Integer getStatus() {
62. return status;
63. }
65. public void setStatus(Integer status) {
66. this.status = status;
67. }
69. public String getMsg() {
70. return msg;
71. }
73. public void setMsg(String msg) {
74. this.msg = msg;
75. }
77. public Object getData() {
78. return data;
79. }
81. public void setData(Object data) {
82. this.data = data;
83. }
85. /**
86. * 将json结果集转化为TaotaoResult对象
87. *
88. * @param jsonData json数据
89. * @param clazz TaotaoResult中的object类型
90. * @return
91. */
92. public static TaotaoResult formatToPojo(String jsonData, Class<?> clazz) {
93. try {
94. if (clazz == null) {
95. return MAPPER.readValue(jsonData, TaotaoResult.class);
96. }
97. JsonNode jsonNode = MAPPER.readTree(jsonData);
98. JsonNode data = jsonNode.get("data");
99. Object obj = null;
100. if (clazz != null) {
101. if (data.isObject()) {
102. obj = MAPPER.readValue(data.traverse(), clazz);
103. } else if (data.isTextual()) {
104. obj = MAPPER.readValue(data.asText(), clazz);
105. }
106. }
107. return build(jsonNode.get("status").intValue(), jsonNode.get("msg").asText(), obj);
108. } catch (Exception e) {
109. return null;
110. }
111. }
113. /**
114. * 没有object对象的转化
115. *
116. * @param json
117. * @return
118. */
119. public static TaotaoResult format(String json) {
120. try {
121. return MAPPER.readValue(json, TaotaoResult.class);
122. } catch (Exception e) {
123. e.printStackTrace();
124. }
125. return null;
126. }
128. /**
129. * Object是集合转化
130. *
131. * @param jsonData json数据
132. * @param clazz 集合中的类型
133. * @return
134. */
135. public static TaotaoResult formatToList(String jsonData, Class<?> clazz) {
136. try {
137. JsonNode jsonNode = MAPPER.readTree(jsonData);
138. JsonNode data = jsonNode.get("data");
139. Object obj = null;
140. if (data.isArray() && data.size() > 0) {
141. obj = MAPPER.readValue(data.traverse(),
142. MAPPER.getTypeFactory().constructCollectionType(List.class, clazz));
143. }
144. return build(jsonNode.get("status").intValue(), jsonNode.get("msg").asText(), obj);
145. } catch (Exception e) {
146. return null;
147. }
148. }
150. }
##11.jedis操作
1. package com.taotao.jedis;
3. public interface JedisClient {
5. String set(String key, String value);
6. String get(String key);
7. Boolean exists(String key);
8. Long expire(String key, int seconds);
9. Long ttl(String key);
10. Long incr(String key);
11. Long hset(String key, String field, String value);
12. String hget(String key, String field);
13. Long hdel(String key, String... field);
14. }
1. package com.taotao.jedis;
3. import org.springframework.beans.factory.annotation.Autowired;
5. import redis.clients.jedis.JedisCluster;
7. public class JedisClientCluster implements JedisClient {
9. @Autowired
10. private JedisCluster jedisCluster;
12. @Override
13. public String set(String key, String value) {
14. return jedisCluster.set(key, value);
15. }
17. @Override
18. public String get(String key) {
19. return jedisCluster.get(key);
20. }
22. @Override
23. public Boolean exists(String key) {
24. return jedisCluster.exists(key);
25. }
27. @Override
28. public Long expire(String key, int seconds) {
29. return jedisCluster.expire(key, seconds);
30. }
32. @Override
33. public Long ttl(String key) {
34. return jedisCluster.ttl(key);
35. }
37. @Override
38. public Long incr(String key) {
39. return jedisCluster.incr(key);
40. }
42. @Override
43. public Long hset(String key, String field, String value) {
44. return jedisCluster.hset(key, field, value);
45. }
47. @Override
48. public String hget(String key, String field) {
49. return jedisCluster.hget(key, field);
50. }
52. @Override
53. public Long hdel(String key, String... field) {
54. return jedisCluster.hdel(key, field);
55. }
57. }
1. package com.taotao.jedis;
4. import org.springframework.beans.factory.annotation.Autowired;
7. import redis.clients.jedis.Jedis;
8. import redis.clients.jedis.JedisPool;
11. public class JedisClientPool implements JedisClient {
13. @Autowired
14. private JedisPool jedisPool;
17. @Override
18. public String set(String key, String value) {
19. Jedis jedis = jedisPool.getResource();
20. String result = jedis.set(key, value);
21. jedis.close();
22. return result;
23. }
26. @Override
27. public String get(String key) {
28. Jedis jedis = jedisPool.getResource();
29. String result = jedis.get(key);
30. jedis.close();
31. return result;
32. }
35. @Override
36. public Boolean exists(String key) {
37. Jedis jedis = jedisPool.getResource();
38. Boolean result = jedis.exists(key);
39. jedis.close();
40. return result;
41. }
44. @Override
45. public Long expire(String key, int seconds) {
46. Jedis jedis = jedisPool.getResource();
47. Long result = jedis.expire(key, seconds);
48. jedis.close();
49. return result;
50. }
53. @Override
54. public Long ttl(String key) {
55. Jedis jedis = jedisPool.getResource();
56. Long result = jedis.ttl(key);
57. jedis.close();
58. return result;
59. }
62. @Override
63. public Long incr(String key) {
64. Jedis jedis = jedisPool.getResource();
65. Long result = jedis.incr(key);
66. jedis.close();
67. return result;
68. }
71. @Override
72. public Long hset(String key, String field, String value) {
73. Jedis jedis = jedisPool.getResource();
74. Long result = jedis.hset(key, field, value);
75. jedis.close();
76. return result;
77. }
80. @Override
81. public String hget(String key, String field) {
82. Jedis jedis = jedisPool.getResource();
83. String result = jedis.hget(key, field);
84. jedis.close();
85. return result;
86. }
89. @Override
90. public Long hdel(String key, String... field) {
91. Jedis jedis = jedisPool.getResource();
92. Long result = jedis.hdel(key, field);
93. jedis.close();
94. return result;
95. }
98. }
阿里P8级别大牛精心整理SPRING核心文档知识点。PDF图文最全文档
Spring 原理
它是一个全面的、企业应用开发一站式的解决方案,贯穿表现层、业务层、持久层。但是 Spring 仍然可以和其他的框架无缝整合。
Spring 特点
1. 轻量级
2. 控制反转
3. 面向切面
4. 容器
5. 框架集合
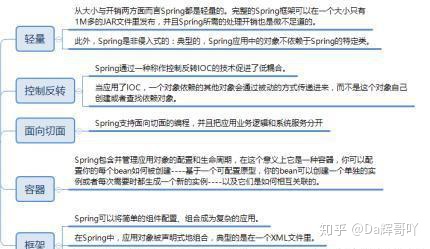
Spring 核心组件
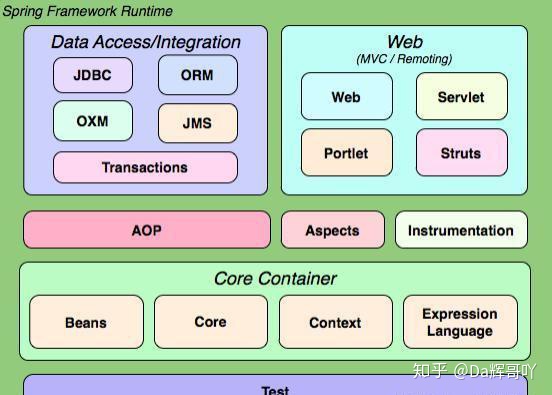
Spring 常用模块
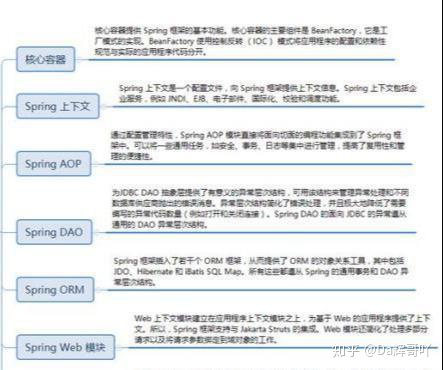
Spring 常用注解
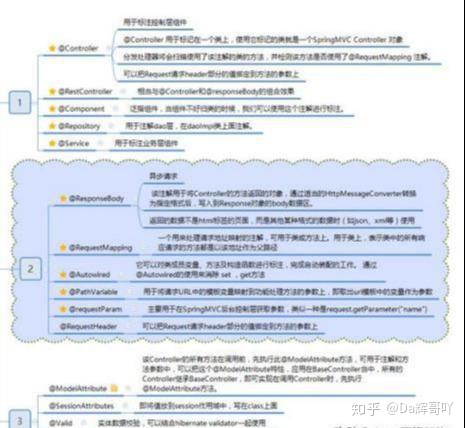
Spring IOC 原理
概念
Spring 通过一个配置文件描述 Bean 及 Bean 之间的依赖关系,利用 Java 语言的反射功能实例化 Bean 并建立 Bean 之间的依赖关系。 Spring 的 IoC 容器在完成这些底层工作的基础上,还提供 了 Bean 实例缓存、生命周期管理、 Bean 实例代理、事件发布、资源装载等高级服务。
Spring 容器高层视图
Spring 启动时读取应用程序提供的 Bean 配置信息,并在 Spring 容器中生成一份相应的 Bean 配 置注册表,然后根据这张注册表实例化 Bean,装配好 Bean 之间的依赖关系,为上层应用提供准 备就绪的运行环境。其中 Bean 缓存池为 HashMap 实现
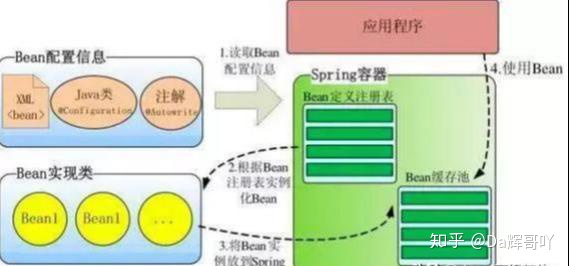
IOC 容器实现
BeanFactory-框架基础设施
BeanFactory 是 Spring 框架的基础设施,面向 Spring 本身;ApplicationContext 面向使用 Spring 框架的开发者,几乎所有的应用场合我们都直接使用 ApplicationContext 而非底层 的 BeanFactory。
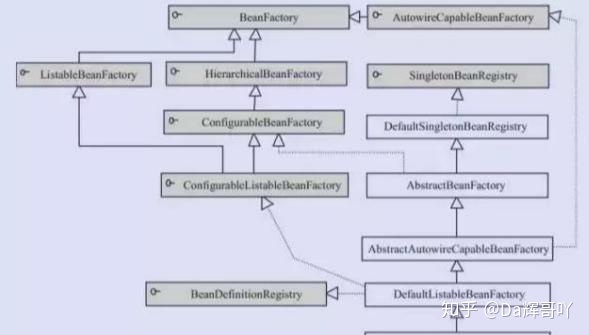
ApplicationContext 面向开发应用
ApplicationContext 由 BeanFactory 派 生 而 来 , 提 供 了 更 多 面 向 实 际 应 用 的 功 能 。 ApplicationContext 继承了 HierarchicalBeanFactory 和 ListableBeanFactory 接口,在此基础 上,还通过多个其他的接口扩展了 BeanFactory 的功能:
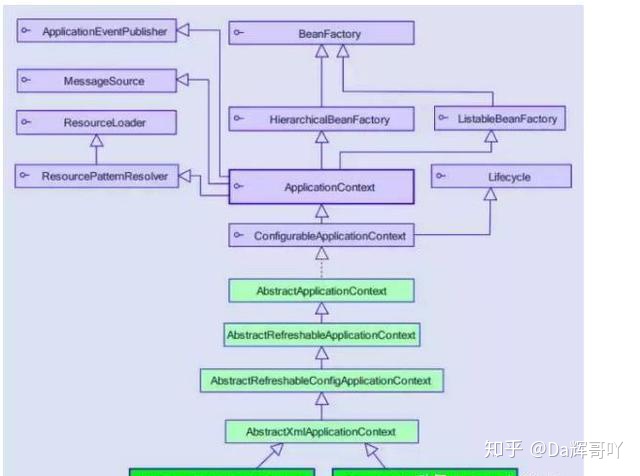
WebApplication 体系架构
WebApplicationContext 是专门为 Web 应用准备的,它允许从相对于 Web 根目录的 路径中装载配置文件完成初始化工作。从 WebApplicationContext 中可以获得 ServletContext 的引用,整个 Web 应用上下文对象将作为属性放置到 ServletContext 中,以便 Web 应用环境可以访问 Spring 应用上下文。
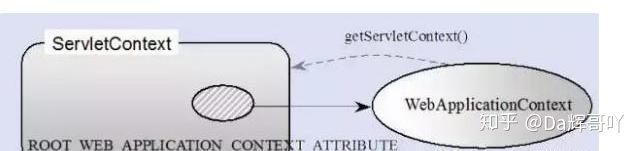
实例化
Spring Bean 生命周期
实例化一个 Bean,也就是我们常说的 new。
IOC 依赖注入
按照 Spring 上下文对实例化的 Bean 进行配置,也就是 IOC 注入。
setBeanName 实现
如果这个 Bean 已经实现了 BeanNameAware 接口,会调用它实现的 setBeanName(String) 方法,此处传递的就是 Spring 配置文件中 Bean 的 id 值
BeanFactoryAware 实现
如果这个 Bean 已经实现了 BeanFactoryAware 接口,会调用它实现的 setBeanFactory, setBeanFactory(BeanFactory)传递的是 Spring 工厂自身(可以用这个方式来获取其它 Bean, 只需在 Spring 配置文件中配置一个普通的 Bean 就可以)
ApplicationContextAware 实现
如果这个 Bean 已经实现了 ApplicationContextAware 接口,会调用 setApplicationContext(ApplicationContext)方法,传入 Spring 上下文(同样这个方式也 可以实现步骤 4 的内容,但比 4 更好,因为 ApplicationContext 是 BeanFactory 的子接 口,有更多的实现方法)
postProcessBeforeInitialization 接口实现-初始化预处理
. 如果这个 Bean 关联了 BeanPostProcessor 接口,将会调用 postProcessBeforeInitialization(Object obj, String s)方法,BeanPostProcessor 经常被用 作是 Bean 内容的更改,并且由于这个是在 Bean 初始化结束时调用那个的方法,也可以被应 用于内存或缓存技术。
init-method
如果 Bean 在 Spring 配置文件中配置了 init-method 属性会自动调用其配置的初始化方法。
postProcessAfterInitialization
如果这个 Bean 关联了 BeanPostProcessor 接口,将会调用 postProcessAfterInitialization(Object obj, String s)方法。 注:以上工作完成以后就可以应用这个 Bean 了,那这个 Bean 是一个 Singleton 的,所以一 般情况下我们调用同一个 id 的 Bean 会是在内容地址相同的实例,当然在 Spring 配置文件中 也可以配置非 Singleton。
Destroy 过期自动清理阶段
当 Bean 不再需要时,会经过清理阶段,如果 Bean 实现了 DisposableBean 这个接口,会调 用那个其实现的 destroy()方法;
destroy-method 自配置清理
最后,如果这个 Bean 的 Spring 配置中配置了 destroy-method 属性,会自动调用其配置的 销毁方法。
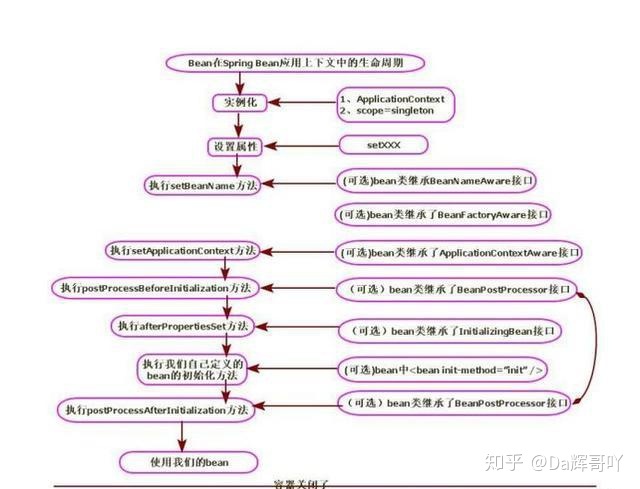
bean 标签有两个重要的属性(init-method 和 destroy-method)。用它们你可以自己定制 初始化和注销方法。它们也有相应的注解(@PostConstruct 和@PreDestroy)。