springboot中使用mybatis
使用非常简单,只需要两步:
(1)添加依赖:
<dependency>
<groupId>org.mybatis.spring.boot</groupId>
<artifactId>mybatis-spring-boot-starter</artifactId>
<version>2.1.1</version>
</dependency>
(2)写Mapper接口和xml,在接口上加@Mappe的注解即可。
【注意】xml要和class的包路径要相同。
MyBatis-Spring-Boot-Starter都做了哪些事情呢?
- 自动发现DataSource。
- 使用SqlSessionFactoryBean自动创建SqlSessionFactory,同时把DataSource传进去。
- 自动创建SqlSessionTemplate。
- 自动做Mapper扫描,并注入到Spring容器。
举个例子
这是用到的Mapper接口:
@Mapper
public interface CityMapper {
@Select("SELECT * FROM CITY WHERE state = #{state}")
City findByState(@Param("state") String state);
}
在SpringBoot工程里面可以直接注入这个Mapper:
@SpringBootApplication
public class SampleMybatisApplication implements CommandLineRunner {
private final CityMapper cityMapper;
public SampleMybatisApplication(CityMapper cityMapper) {
this.cityMapper = cityMapper;
}
public static void main(String[] args) {
SpringApplication.run(SampleMybatisApplication.class, args);
}
@Override
public void run(String... args) throws Exception {
System.out.println(this.cityMapper.findByState("CA"));
}
}
关于Mapper的扫描
(1)默认只扫描@Mapper注解的接口
(2)如果你想自定义一个注解,可以使用@MapperScan
//MapperScan的源代码
public @interface MapperScan {
String[] value() default {};
String[] basePackages() default {};
Class<?>[] basePackageClasses() default {};
Class<? extends BeanNameGenerator> nameGenerator() default BeanNameGenerator.class;
//这个就可以自定义注解类
Class<? extends Annotation> annotationClass() default Annotation.class;
Class<?> markerInterface() default Class.class;
String sqlSessionTemplateRef() default "";
String sqlSessionFactoryRef() default "";
Class<? extends MapperFactoryBean> factoryBean() default MapperFactoryBean.class;
String lazyInitialization() default "";
}
(3)如果Spring容器中有MapperFactoryBean,那么将不再做Mapper的扫描,此时你需要手动的来注册Mapper。
可以看下源码org.mybatis.spring.boot.autoconfigure.MybatisAutoConfiguration.MapperScannerRegistrarNotFoundConfiguration:
@org.springframework.context.annotation.Configuration
@Import(AutoConfiguredMapperScannerRegistrar.class)
//只有当没有MapperFactoryBean和MapperScannerConfigurer的时候才会做Mapper的扫描
@ConditionalOnMissingBean({ MapperFactoryBean.class, MapperScannerConfigurer.class })
public static class MapperScannerRegistrarNotFoundConfiguration implements InitializingBean {
}
使用SqlSession
默认会在Spring容器中注入SqlSessionTemplate,所以也可以直接使用mybatis的api:
@Component
public class CityDao {
private final SqlSession sqlSession;
public CityDao(SqlSession sqlSession) {
this.sqlSession = sqlSession;
}
public City selectCityById(long id) {
return this.sqlSession.selectOne("selectCityById", id);
}
}
Configuration的配置项
所有的属性都以mybatis开头,源码见:org.mybatis.spring.boot.autoconfigure.MybatisProperties。
比如:
# application.yml
mybatis:
type-aliases-package: com.example.domain.model
type-handlers-package: com.example.typehandler
configuration:
map-underscore-to-camel-case: true
default-fetch-size: 100
default-statement-timeout: 30
使用ConfigurationCustomizer自定义配置
@Configuration
public class MyBatisConfig {
@Bean
ConfigurationCustomizer mybatisConfigurationCustomizer() {
return new ConfigurationCustomizer() {
@Override
public void customize(Configuration configuration) {
// customize ...
}
};
}
}
发现Mybatis的其他组件
MyBatis-Spring-Boot-Starter可以自动发现:Interceptor、TypeHandler、LanguageDriver、DatabaseIdProvider。
@Configuration
public class MyBatisConfig {
@Bean
MyInterceptor myInterceptor() {
return MyInterceptor();
}
@Bean
MyTypeHandler myTypeHandler() {
return MyTypeHandler();
}
@Bean
MyLanguageDriver myLanguageDriver() {
return MyLanguageDriver();
}
@Bean
VendorDatabaseIdProvider databaseIdProvider() {
VendorDatabaseIdProvider databaseIdProvider = new VendorDatabaseIdProvider();
Properties properties = new Properties();
properties.put("SQL Server", "sqlserver");
properties.put("DB2", "db2");
properties.put("H2", "h2");
databaseIdProvider.setProperties(properties);
return databaseIdProvider;
}
}
以上只是入门级别的使用,下一篇将从源码级别详细的介绍下这个组件的具体的实现。
参考链接:http://mybatis.org/spring-boot-starter/mybatis-spring-boot-autoconfigure/
扫一扫关注微信公众号:
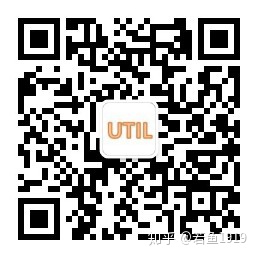