“专业人士笔记”系列目录:
创帆云:Python成为专业人士笔记--强烈建议收藏!每日持续更新!zhuanlan.zhihu.com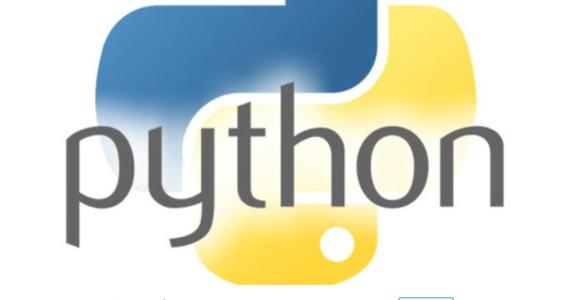
Python可以执行常见的数学运算符,包括整数和浮点除法、乘法、取幂、加法和减法,而数学math模块(包含在所有标准Python版本中)提供了扩展功能,如三角函数、根操作、对数等。
Division 除法
当两个操作数都是整数时,Python3执行后返回的结果是float数据类型,这点和Python2不同;Python不同版本对除法的处理会有不同,其是根据除号两端变量的数据类型决定如何处理的
比如, 我们先定义几个变量:
a, b, c, d, e = 3, 2, 2.0, -3, 10
在Python 3中,
‘/’ 运算符执行“ true”原始除法,即除出来是结果是多少就显示多少, 和参与的数据类型无关。但注意结果的数据类型都是小数float型(这点和pyton2不同);
‘//’运算是除完后强制取整数部分(注意,不是四舍五入),其结果的数据类型,取决于参与计算的数据类型,两个都为整数则结果为整数;其中有一个是float小数,则整个结果为小数;两个都为小数,则结果也是小数
a / b # = 1.5
e / b # = 5.0
a // b # = 1
a // c # = 1.0
import operator
# operator 模块接收两个算术参数
operator.truediv(a, b)
#输出 1.5
operator.floordiv(a, b)
= 1
#输出 1
operator.floordiv(a, c)
#输出 1.0
内置数据类型之间相除后的返回数据类型:
int and int ( Python 2 中返回 int整数结果,Python 3 中返回 float小数结果)
int and float (返回 float)
int and complex (返回 complex 复数)
float and float (返回 float)
float and complex (返回 complex)
complex and complex (返回 complex)
Addition 加法
a, b = 1, 2
用 "+" 操作符:
a + b
# = 3
# 用 "in-place" "+=" 进行变量的添加和重赋值:
a += b
# a = 3 (相当于 a = a + b,对变量操作并重赋值了)
import operator
operator.add(a, b)
# 输出 5 注意:a变量在上面一行代码变成3了
a = operator.iadd(a, b)
# a = 5 相当于 a = a + b,对变量操作并重赋值了
内置数据类型之间相加后返回的数据类型:
int and int (返回 int)
int and float (返回 float)
int and complex (返回 complex)
float and float (返回 float)
float and complex (返回 complex)
complex and complex (返回 complex)
注意:‘+’运算符也用于连接字符串、列表和元组
"first string " + "second string"
#输出: 'first string second string'
[1, 2, 3] + [4, 5, 6]
#输出:[1, 2, 3, 4, 5, 6]
取幂运算
a, b = 2, 3
(a ** b)
#= 8
pow(a, b)
#= 8
import math
math.pow(a, b)
= 8.0 (float小数,不支持complex复数)
import operator
operator.pow(a, b)
#= 8
内置的pow和数学模块math的pow之间的另一个区别是,内置的pow可以接受三个参数
a, b, c = 2, 3, 2
pow(2, 3, 2)
# 输出0,计算等效于 (2 ** 3)% 2,但根据Python文档,直接这样写更有效而不是调用pow函数
特殊函数
函数math.sqrt(x)计算x的平方根
import math
import cmath
c = 4
math.sqrt(c)
#输出 2.0 ( float小数,不支持complex复数 )
cmath.sqrt(c)
#输出 (2+0j) (提供complex复数支持)
若要计算其他根,如立方根,则将该数提到根的次数的倒数,这可以用任何指数函数或操作符来做。
import math
x = 8
math.pow(x, 1/3) # 计算结果为2.0
x**(1/3) # 计算结果为 2.0
函数math.exp(x)计算e ** x :
math.exp(0)
#输出1.0
math.exp(1)
#输出2.718281828459045 (e)
函数math.expm1(x)计算e ** x – 1。当x很小时,这比math.exp(x) – 1的精度要高得多
math.expm1(0)
#0.0
math.exp(1e-6) - 1
#1.0000004999621837e-06
math.expm1(1e-6)
#1.0000005000001665e-06
完整结果: # 1.000000500000166666708333341666…
Trigonometric Functions 三角函数
a, b = 1, 2
import math
math.sin(a)
#返回弧度“ a”的正弦 0.8414709848078965
math.cosh(b)
#返回弧度'b'的反双曲余弦值
#输出: 3.7621956910836314
math.atan(math.pi)
# 返回以弧度为单位的反正切 1.2626272556789115
math.hypot(a, b)
# 返回欧几里得范数,与math.sqrt(aa + bb)相同 2.23606797749979
请注意math.hypot(x,y)是 点(x,y) 距原点(0,0)的向量的长度(或欧几里得距离), 所以要计算两个点(x1,y1)和(x2,y2)之间的欧几里得距离,可以使用这个函数:math.hypot(x2-x1, y2-y1)
要从弧度->度和度->弧度分别使用math.degrees和math.radians进行转换
math.degrees(a)
#输出: 57.29577951308232
math.radians(57.29577951308232)
#输出: 1.0
简易操作符
在应用程序中,通常需要这样的代码 :
a = a + 1
或
a = a * 2
对于这些操作有一个有效的快捷方式
a += 1
和
a *= 2
任何数学运算符都可以使用在’=’字符之前,以进行简易运算:
-= 递减变量
+= 递增变量
*= 递乘变量
/= 递除变量
//= 递除后取整变量
%= 递取模后变量
**= 递取 幂 变量
同样,对于按位运算符(^,| 等都是适用的
Subtraction 减法
a, b = 1, 2
使用“-”操作符 :
b - a # 输出= 1
import operator
包含2个参数算术的函数
operator.sub(b, a)
#输出= 1
内置数据类型之间相减后返回的数据类型:
int and int (返回 int)
int and float ( 返回 float)
int and complex ( 返回 complex)
float and float ( 返回 float)
float and complex ( 返回 complex)
complex and complex ( 返回 complex)
Multiplication 乘法
a, b = 2, 3
a * b # 输出 6
import operator
operator.mul(a, b)
#输出 6
内置数据类型之间相乘后返回的数据类型:
int and int ( 返回 int)
int and float ( 返回 float)
int and complex ( 返回 complex)
float and float ( 返回 float)
float and complex ( 返回 complex)
complex and complex ( 返回 complex)
注意: *操作符也可用于字符串、列表和元组的重复连接 :
3 * 'ab'
#输出: 'ababab'
3 * ('a', 'b')
#元组被重复了 ('a', 'b', 'a', 'b', 'a', 'b')
Logarithms 对数
默认情况下,math.log函数计算以e为底数的对数,你可以选择指定一个底数作为第二个参数:
import math
import cmath
math.log(5)
#输出 1.6094379124341003
#基础参数可选,默认是math.e
math.log(5, math.e) # 输出 1.6094379124341003
cmath.log(5)
#输出 (1.6094379124341003+0j)
math.log(1000, 10)
#输出:3.0 (常返回 float)
cmath.log(1000, 10)
#输出:(3+0j)
Log函数的特殊变体适用于不同的情况:
# 以e - 1为底的对数(值较小时精度更高)
math.log1p(5)
#输出 1.791759469228055
#对数底2
math.log2(8)
#输出 3.0
#对数底10
math.log10(100)
#输出 2.0
cmath.log10(100)
#输出 (2+0j)
Modulus 模数
与许多其他语言一样,Python使用%运算符来取模
3 % 4
#输出3
10 % 2
#输出 0
6 % 4
#输出 2
或者使用 operator module:
import operator
operator.mod(3 , 4)
#输出 3
operator.mod(10 , 2)
#输出 0
operator.mod(6 , 4)
#输出 2
当然, 你也可以用负数 :
-9 % 7
#输出 5
9 % -7
#输出 -5
-9 % -7
#输出 -2
如果需要找到整数除法和模数的结果,可以使用divmod函数:
quotient, remainder = divmod(9, 4)
# quotient = 2, remainder = 1 因为 4 * 2 + 1 == 9
今天的分享就到这里,禁止转载,违者必究!