在代码的修炼开发过程中,我会遗忘,我也很懒,所以本文章就诞生了,方便以后cv大法的应用。
Activity 与 Fragment 之间进行数据传递是,在Activity中将要传递的数据封装在 Bundle中,然后在 Activity 中使用 Fragment 的实例通过 setArgument(Bundel bundel) 方法绑定传递,在要传递到的Fragment中 使用this.getArgment(),得到传递到的Bundle,从而获取到传递。
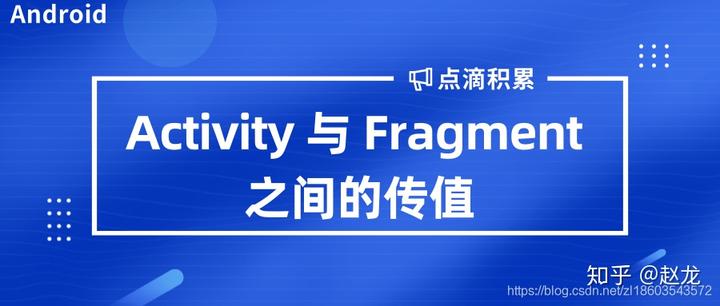
1 Activity 向 Fragment 中传递参数
1.1 写法一
如下代码清单 1-1 在 Activity 中创建 TestAFragment 然后绑定 Bundle 数据,然后 commit 显示 TestAFragment 。
//代码清单 1-1
//传递数据
private void sendTestFunction() {
//创建 Fragment 实例
TestAFragment fragment = new TestAFragment();
//构建 Bundle
Bundle bundle = new Bundle();
//设置数据
bundle.putString("title", "传递到的数据");
//绑定 Fragment
fragment.setArguments(bundle);
FragmentManager fragmentManager = getSupportFragmentManager();
FragmentTransaction fragmentTransaction = fragmentManager.beginTransaction();
fragmentTransaction.replace(R.id.framelayout, fragment);
fragmentTransaction.commit();
}
然后在 TestAFragment 中获取 Bundle 从而获取数据如下代码清单 1-2 所示:
//代码清单 1-2
public class TestAFragment extends Fragment {
public View onCreateView(@NonNull LayoutInflater inflater, @Nullable ViewGroup container, @Nullable Bundle savedInstanceState) {
/// Fragment 所使用的 View
View view = inflater.inflate(R.layout.fragment_first, container, false);
///一个文本 组件
TextView textView = (TextView) view.findViewById(R.id.tv_firster_content);
//获取Bundle 然后获取数据
Bundle bundle = this.getArguments();//得到从Activity传来的数据
String title = null;
if (bundle != null) {
title = bundle.getString("title");
}
///设置显示数据
textView.setText(title);
return view;
}
}
1.2 写法二
通过 fragment 的静态方法来创建实例并绑定数据 ,如下代码清单 1-3 在 Activity 中 :
//代码清单 1-3
//传递数据
private void sendTestBFunction() {
//创建 Fragment 实例
// 通过静态方法来创建并绑定数据
TestBFragment fragment = TestBFragment.newInstance("测试数据");
FragmentManager fragmentManager = getSupportFragmentManager();
FragmentTransaction fragmentTransaction = fragmentManager.beginTransaction();
fragmentTransaction.replace(R.id.framelayout, fragment);
fragmentTransaction.commit();
}
然后对应的 TestBFragment 中声明如下代码清单 1-4所示 :
//代码清单 1-3
public class TestBFragment extends Fragment {
/**
* @param title 参数
* @return Fragment 实例
*/
public static TestBFragment newInstance(String title) {
//创建 Fragment 实例
TestBFragment fragment = new TestBFragment();
//创建 Bundle
Bundle lBundle = new Bundle();
lBundle.putString("title", title);
fragment.setArguments(lBundle);
return fragment;
}
public View onCreateView(@NonNull LayoutInflater inflater, @Nullable ViewGroup container, @Nullable Bundle savedInstanceState) {
View view = inflater.inflate(R.layout.fragment_first,container,false);
TextView textView = (TextView)view.findViewById(R.id.tv_firster_content);
//获取Bundle 然后获取数据
Bundle bundle =this.getArguments();//得到从Activity传来的数据
String title = null;
if(bundle!=null){
title = bundle.getString("title");
}
textView.setText(title);
return view;
}
}
需要注意的是静态方法 newInstance 只是用来创建 Fragment 实例与设置绑定数据的,如果需要在 Fragment 中使用还需要通过 Bundle 执行获取操作。
2 Fragment 向 Activity 中传递参数
Fragment 是在 Activity 中加载,数据传递思路就是 Activity 中设置监听 ,然后 Fragment 发送回调消息就可以 。
首先定义 监听 如下代码清单 2-1 所示 :
//代码清单 2-1
public interface FragmentCallBack {
//定义监听方法 根据实际需求来
void test(int flag);
}
然后为 Activity 绑定监听,如下代码清单 2-2 所示:
///代码清单 2-2
public class MainActivity extends AppCompatActivity implements FragmentCallBack{
/// Fragment 消息回调的方法
@Override
public void test(int flag) {
}
... ...
}
然后在 Fragment 中 获取这个 监听并发送回调 ,如下代码清单 2-3所示:
//代码清单 1-3
public class TestBFragment extends Fragment {
//监听回调
FragmentCallBack mFragmentCallBack;
///onAttach 当 Fragment 与 Activity 绑定时调用
@Override
public void onAttach(Context context) {
super.onAttach(context);
///获取绑定的监听
if (context instanceof FragmentCallBack) {
mFragmentCallBack = (FragmentCallBack) context;
}
}
///onDetach 当 Fragment 与 Activity 解除绑定时调用
@Override
public void onDetach() {
super.onDetach();
mFragmentCallBack = null;
}
...
}
然后在业务代码中,如点击按钮传递数据给 Activity :
///消息回调到 Activity
if (mFragmentCallBack != null) {
mFragmentCallBack.test(111);
}
3 聊聊 Bundle
Bundle主要用于传递数据;它保存的数据,是以key-value(键值对)的形式存在的,是一个信息的载体,内部其实就是维护了一个Map<String,Object>。
需要注意的是 Intent 负责 Activity 之间的交互,内部是持有一个Bundle。
传递的数据可以是boolean、byte、int、long、float、double、String等基本类型或它们对应的数组,也可以是对象或对象数组,当Bundle传递的是对象或对象数组时,必须实现Serializable或Parcelable接口 。
Bundle提供了各种常用类型的putXxx()/getXxx()方法,用于读写数据,如下:
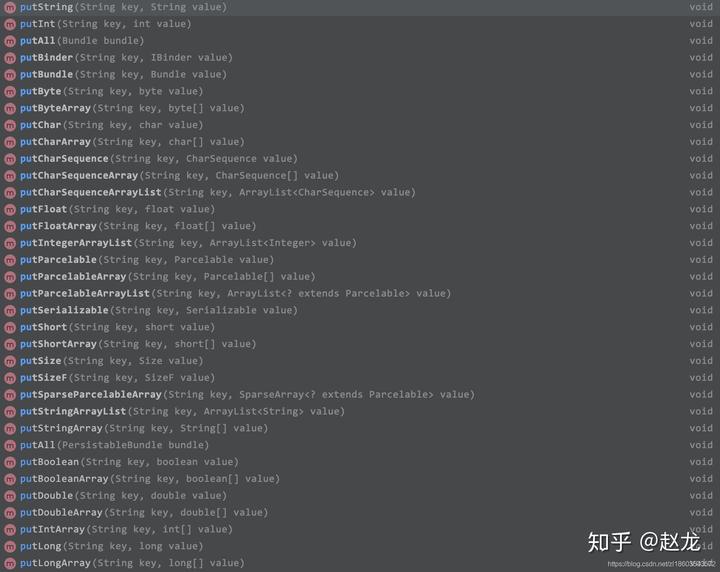
3.1 Activity
在activity间传递信息
Bundle bundle = new Bundle(); //创建bundle对象
bundle.putString("title", "张三"); //(String型)
bundle.putInt("age", 175); //int 类型,value-175
intent.putExtras(bundle); //通过intent绑定Bundle
startActivity(intent);
activity 中读取数据
//读取intent的数据给bundle对象
Bundle bundle = this.getIntent().getExtras();
//通过key得到value
String title = bundle.getString("title");
int age = bundle.getInt("age");
3.1 线程间传递
通过Handler将带有Bundle数据的message放入消息队列,其他线程就可以从队列中得到数据,代码如下:
//创建 Message对象
Message message=new Message();
//标记
message.what = 110;
//创建Bundle对象
Bundle bundle = new Bundle();
//设置数据
bundle.putString("text1","String 文本数据 ");
bundle.putInt("text2",1111);
///绑定 Message
message.setData(bundle);
//Handler将消息放入消息队列
mHandler.sendMessage(message);
读取数据 ,在 Handler 的 handleMessage(Message msg) 方法回调用
String str1=msg.getData().getString("text1");
int int1=msg.getData().getString("text2");
本公众号会首发系列专题文章,付费的视频课程会在公众号中免费刊登,在你上下班的路上或者是睡觉前的一刻,本公众号都是你浏览知识干货的一个小选择,收藏不如行动,在那一刻,公众号会提示你该学习了。
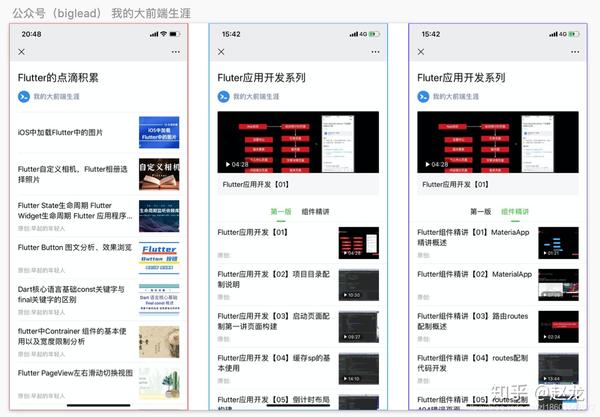