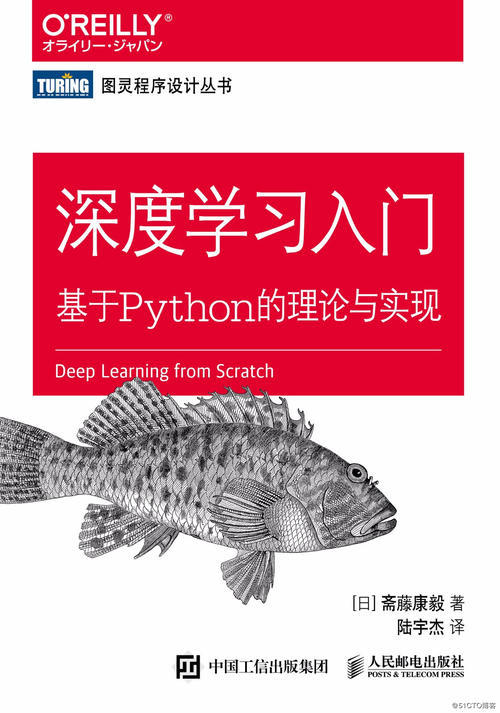
与门(AND)
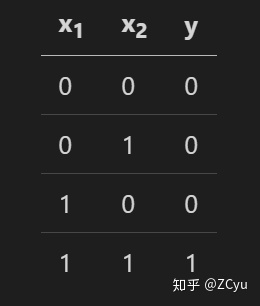
权重需满足的条件:
0<=theta
w2<=theta
w1<=theta
w1+w2>theta
# theta:阈值
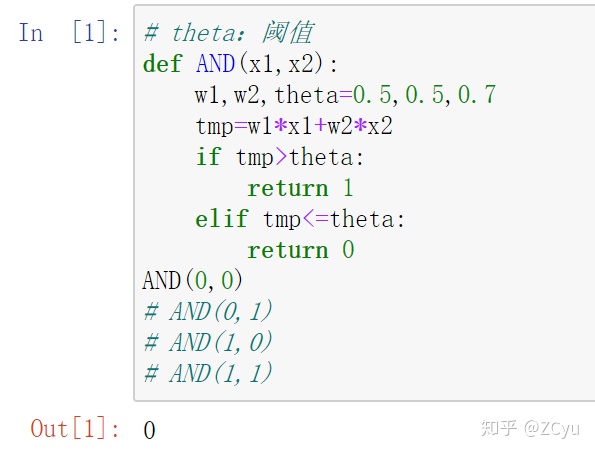
# 导入权重和偏置
# 设b=-theta
#w1*x1+w2*x2+b>0
import numpy as np
def AND(x1,x2):
x=np.array([x1,x2])
w=np.array([0.5,0.5])
b=-0.7
tmp=np.sum(w*x)+b
if tmp>0:
return 1
elif tmp<=0:
return 0
AND(0,1)
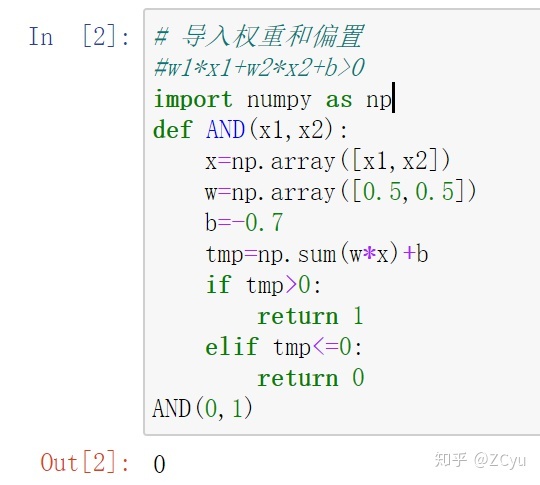
与非门(NAND)
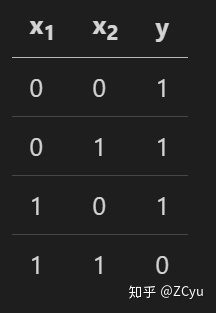
权重需满足的条件:
0>theta
w2>theta
w1>theta
w1+w2<=theta
# 与非门
import numpy as np
def NAND(x1,x2):
x=np.array([x1,x2])
w=np.array([-0.5,-0.5])
b=0.7
tmp=np.sum(w*x)+b
if tmp>0:
return 1
elif tmp<=0:
return 0
NAND(1,1)
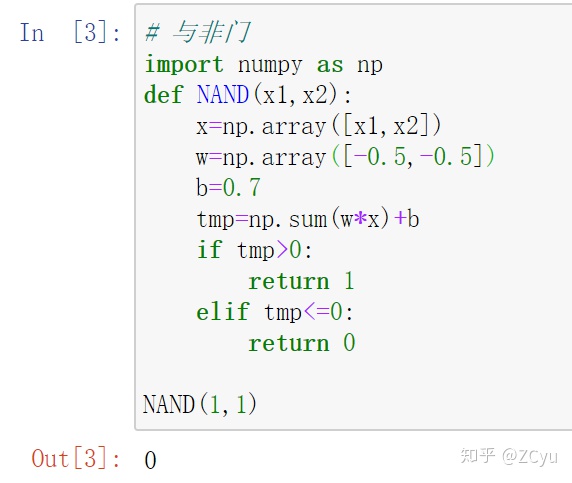
或门(OR)
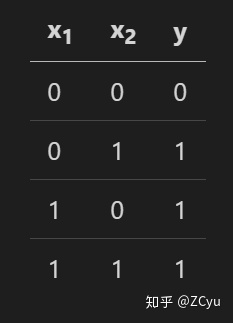
权重需满足的条件:
0<=theta
w2>theta
w1>theta
w1+w2>theta
# 或门
import numpy as np
def OR(x1,x2):
x=np.array([x1,x2])
w=np.array([0.5,0.5])
b=-0.2
tmp=np.sum(w*x)+b
if tmp>0:
return 1
elif tmp<=0:
return 0
OR(1,0)
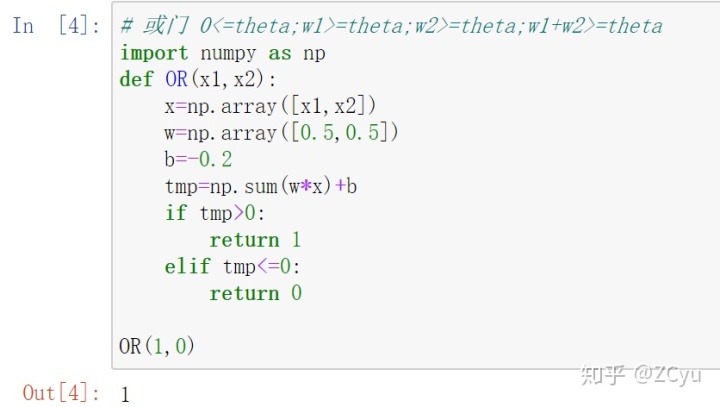
异或门(XOR)
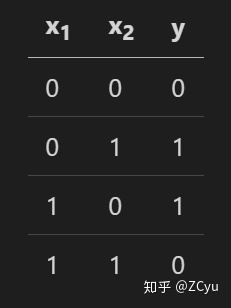
权重需满足的条件:
0<=theta
w2>theta
w1>theta
w1+w2<=theta
显然用前面介绍的感知机是无法实现这个异或门的,我们可以使用多层感知机实现异或门。
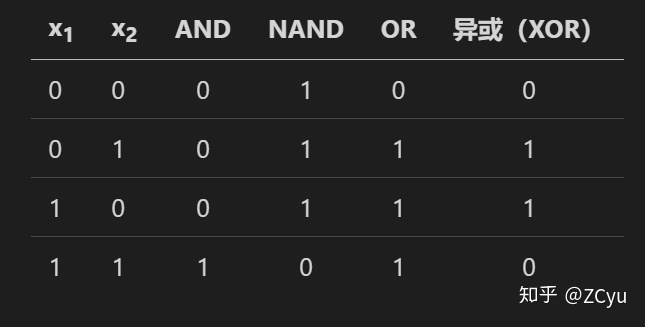
# 感知机的局限-异或门
# 单层感知机无法实现异或门 0<=theta;w1>theta;w2>theta;w1+w2<=theta 显然不成立
# 但是我们可以使用 多层感知机 来解决异或门的问题
import numpy as np
def XOR(x1,x2):
s1=NAND(x1,x2)
s2=OR(x1,x2)
y=AND(s1,s2)
return s1,s2,y
r1,r2,r3=XOR(0,1)
print("result=",r3)
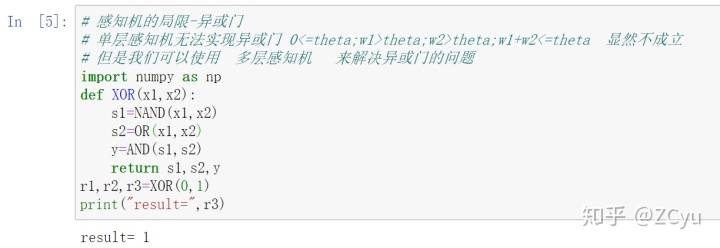
ZCyu's python 学习笔记 5