前言
这是统计github上的开源java项目,整理出最常用的11个开源工具类及其方法。
大部分方法可以望文知意,请务必浏览一遍,知道有哪些好用的工具类,不必自己造轮子了。
绝对的好东西,直接收藏吧!
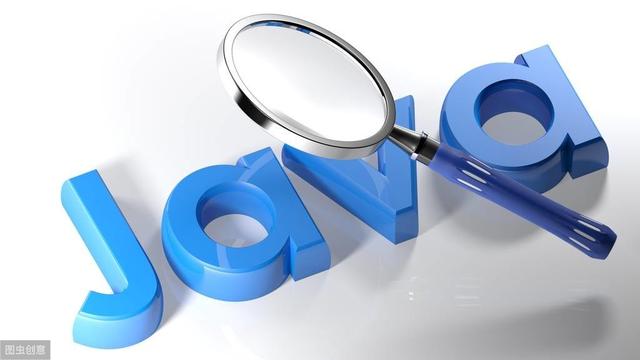
1.json转换工具
1. package com.taotao.utils; 3. import java.util.List; 5. import com.fasterxml.jackson.core.JsonProcessingException; 6. import com.fasterxml.jackson.databind.JavaType; 7. import com.fasterxml.jackson.databind.JsonNode; 8. import com.fasterxml.jackson.databind.ObjectMapper; 10. /** 11. * json转换工具类 12. */ 13. public class JsonUtils { 15. // 定义jackson对象 16. private static final ObjectMapper MAPPER = new ObjectMapper(); 18. /** 19. * 将对象转换成json字符串。 20. *
Title: pojoToJson
21. *
Description:
22. * @param data 23. * @return 24. */ 25. public static String objectToJson(Object data) { 26. try { 27. String string = MAPPER.writeValueAsString(data); 28. return string; 29. } catch (JsonProcessingException e) { 30. e.printStackTrace(); 31. } 32. return null; 33. } 35. /** 36. * 将json结果集转化为对象 37. * 38. * @param jsonData json数据 39. * @param clazz 对象中的object类型 40. * @return 41. */ 42. public static T jsonToPojo(String jsonData, Class beanType) { 43. try { 44. T t = MAPPER.readValue(jsonData, beanType); 45. return t; 46. } catch (Exception e) { 47. e.printStackTrace(); 48. } 49. return null; 50. } 52. /** 53. * 将json数据转换成pojo对象list 54. *
Title: jsonToList
55. *
Description:
56. * @param jsonData 57. * @param beanType 58. * @return 59. */ 60. public static List jsonToList(String jsonData, Class beanType) { 61. JavaType javaType = MAPPER.getTypeFactory().constructParametricType(List.class, beanType); 62. try { 63. List list = MAPPER.readValue(jsonData, javaType); 64. return list; 65. } catch (Exception e) { 66. e.printStackTrace(); 67. } 69. return null; 70. } 72. }
2.cookie的读写
1. package com.taotao.common.utils; 3. import java.io.UnsupportedEncodingException; 4. import java.net.URLDecoder; 5. import java.net.URLEncoder; 7. import javax.servlet.http.Cookie; 8. import javax.servlet.http.HttpServletRequest; 9. import javax.servlet.http.HttpServletResponse; 12. /** 13. * 14. * Cookie 工具类 15. * 16. */ 17. public final class CookieUtils { 19. /** 20. * 得到Cookie的值, 不编码 21. * 22. * @param request 23. * @param cookieName 24. * @return 25. */ 26. public static String getCookieValue(HttpServletRequest request, String cookieName) { 27. return getCookieValue(request, cookieName, false); 28. } 30. /** 31. * 得到Cookie的值, 32. * 33. * @param request 34. * @param cookieName 35. * @return 36. */ 37. public static String getCookieValue(HttpServletRequest request, String cookieName, boolean isDecoder) { 38. Cookie[] cookieList = request.getCookies(); 39. if (cookieList == null || cookieName == null) { 40. return null; 41. } 42. String retValue = null; 43. try { 44. for (int i = 0; i < cookieList.length; i++) { 45. if (cookieList[i].getName().equals(cookieName)) { 46. if (isDecoder) { 47. retValue = URLDecoder.decode(cookieList[i].getValue(), "UTF-8"); 48. } else { 49. retValue = cookieList[i].getValue(); 50. } 51. break; 52. } 53. } 54. } catch (UnsupportedEncodingException e) { 55. e.printStackTrace(); 56. } 57. return retValue; 58. } 60. /** 61. * 得到Cookie的值, 62. * 63. * @param request 64. * @param cookieName 65. * @return 66. */ 67. public static String getCookieValue(HttpServletRequest request, String cookieName, String encodeString) { 68. Cookie[] cookieList = request.getCookies(); 69. if (cookieList == null || cookieName == null) { 70. return null; 71. } 72. String retValue = null; 73. try { 74. for (int i = 0; i < cookieList.length; i++) { 75. if (cookieList[i].getName().equals(cookieName)) { 76. retValue = URLDecoder.decode(cookieList[i].getValue(), encodeString); 77. break; 78. } 79. } 80. } catch (UnsupportedEncodingException e) { 81. e.printStackTrace(); 82. } 83. return retValue; 84. } 86. /** 87. * 设置Cookie的值 不设置生效时间默认浏览器关闭即失效,也不编码 88. */ 89. public static void setCookie(HttpServletRequest request, HttpServletResponse response, String cookieName, 90. String cookieValue) { 91. setCookie(request, response, cookieName, cookieValue, -1); 92. } 94. /** 95. * 设置Cookie的值 在指定时间内生效,但不编码 96. */ 97. public static void setCookie(HttpServletRequest request, HttpServletResponse response, String cookieName, 98. String cookieValue, int cookieMaxage) { 99. setCookie(request, response, cookieName, cookieValue, cookieMaxage, false); 100. } 102. /** 103. * 设置Cookie的值 不设置生效时间,但编码 104. */ 105. public static void setCookie(HttpServletRequest request, HttpServletResponse response, String cookieName, 106. String cookieValue, boolean isEncode) { 107. setCookie(request, response, cookieName, cookieValue, -1, isEncode); 108. } 110. /** 111. * 设置Cookie的值 在指定时间内生效, 编码参数 112. */ 113. public static void setCookie(HttpServletRequest request, HttpServletResponse response, String cookieName, 114. String cookieValue, int cookieMaxage, boolean isEncode) { 115. doSetCookie(request, response, cookieName, cookieValue, cookieMaxage, isEncode); 116. } 118. /** 119. * 设置Cookie的值 在指定时间内生效, 编码参数(指定编码) 120. */ 121. public static void setCookie(HttpServletRequest request, HttpServletResponse response, String cookieName, 122. String cookieValue, int cookieMaxage, String encodeString) { 123. doSetCookie(request, response, cookieName, cookieValue, cookieMaxage, encodeString); 124. } 126. /** 127. * 删除Cookie带cookie域名 128. */ 129. public static void deleteCookie(HttpServletRequest request, HttpServletResponse response, 130. String cookieName) { 131. doSetCookie(request, response, cookieName,