Vasya is interested in arranging dominoes. He is fed up with common dominoes and he uses the dominoes of different heights. He put ndominoes on the table along one axis, going from left to right. Every domino stands perpendicular to that axis so that the axis passes through the center of its base. The i-th domino has the coordinate xi and the height hi. Now Vasya wants to learn for every domino, how many dominoes will fall if he pushes it to the right. Help him do that.
Consider that a domino falls if it is touched strictly above the base. In other words, the fall of the domino with the initial coordinate x and height h leads to the fall of all dominoes on the segment [x + 1, x + h - 1].
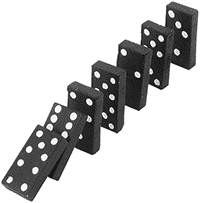
The first line contains integer n (1 ≤ n ≤ 105) which is the number of dominoes. Then follow n lines containing two integers xi and hi ( - 108 ≤ xi ≤ 108, 2 ≤ hi ≤ 108) each, which are the coordinate and height of every domino. No two dominoes stand on one point.
Print n space-separated numbers zi — the number of dominoes that will fall if Vasya pushes the i-th domino to the right (including the domino itself).
4 16 5 20 5 10 10 18 2
3 1 4 1
4 0 10 1 5 9 10 15 10
4 1 2 1
/**
题目大意:
给定一些多米诺骨牌,在x轴上从左到右排列在一起,问,每一个倒向右边的时候会压倒多少个骨牌?
注意,给定的骨牌并不是按照x从小到大的顺序排列的,而且数据比较大。
思路:
这是一个动态规划问题,开始就注意数据,因此感觉O(N^2)不靠谱,但是,动态规划问题,是要寻找
前一个状态转移到后一个状态转移方程的,一定有更有效的转移方程。
要记住,前一个状态的最优值,就是当前状态的前置。
首先,需要将无序的骨牌进行排序,这样,才能进行状态转移~~~
假设,第j个骨牌要倒向右面,而这时要考虑j之前的骨牌,考虑j之前某一个骨牌i倒下之后的最优值,
如果,j倒下后压倒i,那j的最优值需要加上i的最优值(1),
如果用O(N^2)的枚举j-1到0状态最优值的算法,还需要考虑当前的骨牌和它压倒的骨牌中重复的情况,
那这样的算法复杂度就会超过O(N^2),因此,这并不是一种高效的算法。
基于(1)处的思路,我们实际上并不需要对j-1到0的最优值进行一一枚举,而是,对于j的初始值(为1),
加上前面的第i个骨牌的最优值之后,判断第i-dp[i]个骨牌是否也会被j压倒,如此进行,直至0处。
**/
#include <map>
#include <set>
#include <list>
#include <queue>
#include <stack>
#include <cmath>
#include <ctime>
#include <vector>
#include <bitset>
#include <cstdio>
#include <string>
#include <numeric>
#include <cstring>
#include <cstdlib>
#include <iostream>
#include <algorithm>
#include <functional>
using namespace std;
typedef long long ll;
typedef unsigned long long ull;
int dx[4]= {-1,1,0,0};
int dy[4]= {0,0,-1,1}; //up down left right
bool inmap(int x,int y,int n,int m)
{
if(x<1||x>n||y<1||y>m)return false;
return true;
}
int hashmap(int x,int y,int m)
{
return (x-1)*m+y;
}
#define eps 1e-8
#define inf 0x7fffffff
#define debug puts("BUG")
#define lson l,m,rt<<1
#define rson m+1,r,rt<<1|1
#define read freopen("in.txt","r",stdin)
#define write freopen("out.txt","w",stdout)
#define lowbit(x) (-x)&x
#define mod 1000000007
#define maxn 555555
int n;
struct Node
{
int h;
int x;
int dp;
int id;
} a[maxn];
bool cmp(Node a,Node b)//排序
{
return a.x>b.x;
}
int sum[maxn];
int main()
{
scanf("%d",&n);
for(int i=0; i<n; i++)
{
scanf("%d%d",&a[i].x,&a[i].h);//将每个骨牌的信息记录下来
a[i].id=i;
}
sort(a,a+n,cmp);
for(int i=0; i<n; i++)//初始化
a[i].dp=1;
sum[a[0].id]=1;
for(int i=0; i<n; i++)
{
int j;
for(j=i-1; j>=0; j-=a[j].dp)
{
if(a[j].x>a[i].x+a[i].h-1)//判断j能否被i压倒,不能的话,就该跳出了
break;
a[i].dp+=a[j].dp;//状态转移
}
sum[a[i].id]=a[i].dp;//按照当初输入时的顺序进行记录
}
for(int i=0;i<n;i++)
printf("%d ",sum[i]);
return 0;
}