#include <iostream>
#include <boost/date_time/gregorian/gregorian.hpp>
#include <boost/static_assert.hpp>
using namespace std;
using namespace boost::gregorian;
int _tmain(int argc, _TCHAR* argv[])
{
date d1;
date d2(2012,12,12);
date d3(2012, Jan, 1);
date d4(d2);
assert(d1==date(not_a_date_time));
assert(d2==d4);
d1=from_string("2012-12-10");
d2=(from_string("2012/12/10"));
d3=from_undelimited_string("20121210");
cout<<d1<<endl<<d2<<endl<<d3<<endl<<d4<<endl;
cout<<day_clock::local_day()<<endl;
cout<<day_clock::universal_day()<<endl;
return 0;
}
#include <boost/date_time/gregorian/gregorian.hpp>
#include <boost/static_assert.hpp>
using namespace std;
using namespace boost::gregorian;
int _tmain(int argc, _TCHAR* argv[])
{
date d1;
date d2(2012,12,12);
date d3(2012, Jan, 1);
date d4(d2);
assert(d1==date(not_a_date_time));
assert(d2==d4);
d1=from_string("2012-12-10");
d2=(from_string("2012/12/10"));
d3=from_undelimited_string("20121210");
cout<<d1<<endl<<d2<<endl<<d3<<endl<<d4<<endl;
cout<<day_clock::local_day()<<endl;
cout<<day_clock::universal_day()<<endl;
return 0;
}
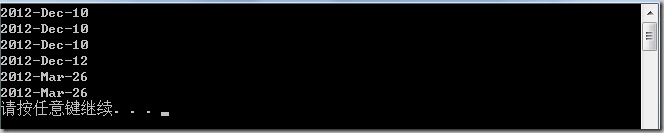
#include <iostream> #include <boost/date_time/gregorian/gregorian.hpp> #include <boost/static_assert.hpp> using namespace std; using namespace boost::gregorian; int _tmain(int argc, _TCHAR* argv[]) { date d(2012,3,26); assert(d.year()==2012); assert(d.month()==3); assert(d.day()==26); //一次性的获得年月日数据 date::ymd_type ymd=d.year_month_day(); assert(ymd.year==2012); assert(ymd.month==3); assert(ymd.day==26); cout<<"今天是"<<d.day_of_week()<<endl; //输出date的星期数,0表示星期日 cout<<"今天是今年的第"<<d.day_of_year()<<"天"<<endl; cout<<"本周是今年的第"<<d.week_number()<<"周"<<endl; cout<<"本月的最后一天是:"<<d.end_of_month()<<endl; //几种输出格式的比较 cout<<to_simple_string(d)<<endl; cout<<to_iso_string(d)<<endl; cout<<to_iso_extended_string(d)<<endl; cout<<d<<endl; return 0; }
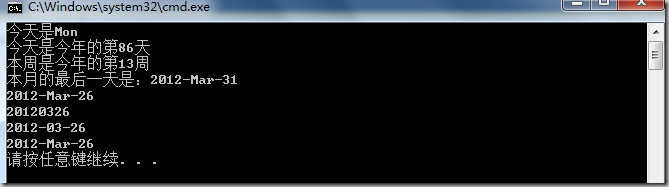
日期长度:
#include <iostream> #include <boost/date_time/gregorian/gregorian.hpp> #include <boost/static_assert.hpp> using namespace std; using namespace boost::gregorian; int _tmain(int argc, _TCHAR* argv[]) { days d1(100); days d2(-100); assert(d1+d2==days(0)); assert((d1+d2).days()==0); weeks w(3); //三个星期 assert(w.days()==21); months m(5); //5个月 years y(2); //2年 months m2=y+m; //2年零5个月 assert(m2.number_of_months()==29); assert(y.number_of_years()*2==4); return 0; }
一些日期的运算:
#include <iostream> #include <boost/date_time/gregorian/gregorian.hpp> #include <boost/static_assert.hpp> using namespace std; using namespace boost::gregorian; //一些日期的运算 int _tmain(int argc, _TCHAR* argv[]) { date d1(2012,3,26); date d2(1991,2,10); cout<<"我已经出生了"<<d1-d2<<"天了"<<endl; d1+=days(10); cout<<to_iso_extended_string(d1)<<endl; d1+=months(2); cout<<to_iso_extended_string(d1)<<endl; d1+=weeks(1); cout<<to_iso_extended_string(d1)<<endl; d1-=years(2); cout<<to_iso_extended_string(d1)<<endl; return 0; }
日期区间:
#include <iostream> #include <boost/date_time/gregorian/gregorian.hpp> #include <boost/static_assert.hpp> using namespace std; using namespace boost::gregorian; //日期区间 int _tmain(int argc, _TCHAR* argv[]) { date_period dp(date(2012,1,1),days(10)); assert(!dp.is_null()); assert(dp.begin().day()==1); assert(dp.last().day()==10); assert(dp.end().day()==11); assert(dp.length().days()==10); cout<<dp<<endl; return 0; }

日期区间的运算
#include <iostream> #include <boost/date_time/gregorian/gregorian.hpp> #include <boost/static_assert.hpp> using namespace std; using namespace boost::gregorian; //日期区间的运算 int _tmain(int argc, _TCHAR* argv[]) { date_period dp(date(2012,1,1),days(10)); dp.shift(days(3)); assert(dp.begin().day()==4); assert(dp.length().days()==10); dp.expand(days(3)); assert(dp.begin().day()==1); assert(dp.length().days()==16); assert(dp.is_after(date(2000,12,12))); assert(dp.is_before(date(2013,12,12))); assert(dp.contains(date(2012,1,2))); return 0; }
在Boost::Date_Time的使用过程中还发现了一个问题,就是在用到time_from_string()时会报链接错误,如下:
错误 1 error LNK2019: 无法解析的外部符号 "public: static class boost::shared_ptr<class stlpd_std::map<class stlpd_std::basic_string<char,class stlpd_std::char_traits<char>,class stlpd_std::allocator<char> >,unsigned short,struct stlpd_std::less<class stlpd_std::basic_string<char,class stlpd_std::char_traits<char>,class stlpd_std::allocator<char> > >,class stlpd_std::allocator<struct stlpd_std::pair<class stlpd_std::basic_string<char,class stlpd_std::char_traits<char>,class stlpd_std::allocator<char> > const ,unsigned short> > > > __cdecl boost::gregorian::greg_month::get_month_map_ptr(void)" (?get_month_map_ptr@greg_month@gregorian@boost@@SA?AV?$shared_ptr@V?$map@V?$basic_string@DV?$char_traits@D@stlpd_std@@V?$allocator@D@2@@stlpd_std@@GU?$less@V?$basic_string@DV?$char_traits@D@stlpd_std@@V?$allocator@D@2@@stlpd_std@@@2@V?$allocator@U?$pair@$$CBV?$basic_string@DV?$char_traits@D@stlpd_std@@V?$allocator@D@2@@stlpd_std@@G@stlpd_std@@@2@@stlpd_std@@@3@XZ),该符号在函数 "unsigned short __cdecl boost::date_time::month_str_to_ushort<class boost::gregorian::greg_month>(class stlpd_std::basic_string<char,class stlpd_std::char_traits<char>,class stlpd_std::allocator<char> > const &)" (??$month_str_to_ushort@Vgreg_month@gregorian@boost@@@date_time@boost@@YAGABV?$basic_string@DV?$char_traits@D@stlpd_std@@V?$allocator@D@2@@stlpd_std@@@Z) 中被引用
在网上找解决办法时,都是说不能只包含头文件,还要链接相应的库,实际上不是没有链接库产生的问题。
不使用date_time库的时候需要添加
#define BOOST_DATE_TIME_SOURCE
#include <libs/date_time/src/gregorian/greg_names.hpp>
#include <libs/date_time/src/gregorian/date_generators.cpp>
#include <libs/date_time/src/gregorian/greg_month.cpp>
#include <libs/date_time/src/gregorian/greg_weekday.cpp>
#include <libs/date_time/src/gregorian/gregorian_types.cpp>
#include <libs/date_time/src/gregorian/date_generators.cpp>
#include <libs/date_time/src/gregorian/greg_month.cpp>
#include <libs/date_time/src/gregorian/greg_weekday.cpp>
#include <libs/date_time/src/gregorian/gregorian_types.cpp>