学习整理了一下
(一).功能
用HttpHandler实现图片验证码
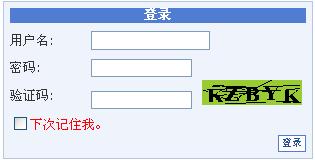
(二).代码如下
1. 处理程序文件 ValidateImageHandler.ashx代码如下
1
<%
@ WebHandler Language
=
"
C#
"
Class
=
"
ValidateImageHandler
"
%>
2
3 using System;
4 using System.Web;
5 using System.Web.SessionState;
6 using System.Drawing;
7 using System.Drawing.Imaging;
8 using System.Text;
9
10 /// <summary>
11 /// ValidateImageHandler 生成网站验证码功能
12 /// </summary>
13 public class ValidateImageHandler : IHttpHandler, IRequiresSessionState
14 {
15 int intLength = 5 ; // 长度
16 string strIdentify = " Identify " ; // 随机字串存储键值,以便存储到Session中
17 public ValidateImageHandler()
18 {
19 }
20
21 /// <summary>
22 /// 生成验证图片核心代码
23 /// </summary>
24 /// <param name="hc"></param>
25 public void ProcessRequest(HttpContext hc)
26 {
27 // 设置输出流图片格式
28 hc.Response.ContentType = " image/gif " ;
29
30 Bitmap b = new Bitmap( 200 , 60 );
31 Graphics g = Graphics.FromImage(b);
32 g.FillRectangle( new SolidBrush(Color.YellowGreen), 0 , 0 , 200 , 60 );
33 Font font = new Font(FontFamily.GenericSerif, 48 , FontStyle.Bold, GraphicsUnit.Pixel);
34 Random r = new Random();
35
36 // 合法随机显示字符列表
37 string strLetters = " abcdefghijklmnopqrstuvwxyzABCDEFGHIJKLMNOPQRSTUVWXYZ1234567890 " ;
38 StringBuilder s = new StringBuilder();
39
40 // 将随机生成的字符串绘制到图片上
41 for ( int i = 0 ; i < intLength; i ++ )
42 {
43 s.Append(strLetters.Substring(r.Next( 0 , strLetters.Length - 1 ), 1 ));
44 g.DrawString(s[s.Length - 1 ].ToString(), font, new SolidBrush(Color.Blue), i * 38 , r.Next( 0 , 15 ));
45 }
46
47 // 生成干扰线条
48 Pen pen = new Pen( new SolidBrush(Color.Blue), 2 );
49 for ( int i = 0 ; i < 10 ; i ++ )
50 {
51 g.DrawLine(pen, new Point(r.Next( 0 , 199 ), r.Next( 0 , 59 )), new Point(r.Next( 0 , 199 ), r.Next( 0 , 59 )));
52 }
53 b.Save(hc.Response.OutputStream, ImageFormat.Gif);
54 hc.Session[strIdentify] = s.ToString(); // 先保存在Session中,验证与用户输入是否一致
55 hc.Response.End();
56
57 }
58
59 /// <summary>
60 /// 表示此类实例是否可以被多个请求共用(重用可以提高性能)
61 /// </summary>
62 public bool IsReusable
63 {
64 get
65 {
66 return true ;
67 }
68 }
69 }
70
2
3 using System;
4 using System.Web;
5 using System.Web.SessionState;
6 using System.Drawing;
7 using System.Drawing.Imaging;
8 using System.Text;
9
10 /// <summary>
11 /// ValidateImageHandler 生成网站验证码功能
12 /// </summary>
13 public class ValidateImageHandler : IHttpHandler, IRequiresSessionState
14 {
15 int intLength = 5 ; // 长度
16 string strIdentify = " Identify " ; // 随机字串存储键值,以便存储到Session中
17 public ValidateImageHandler()
18 {
19 }
20
21 /// <summary>
22 /// 生成验证图片核心代码
23 /// </summary>
24 /// <param name="hc"></param>
25 public void ProcessRequest(HttpContext hc)
26 {
27 // 设置输出流图片格式
28 hc.Response.ContentType = " image/gif " ;
29
30 Bitmap b = new Bitmap( 200 , 60 );
31 Graphics g = Graphics.FromImage(b);
32 g.FillRectangle( new SolidBrush(Color.YellowGreen), 0 , 0 , 200 , 60 );
33 Font font = new Font(FontFamily.GenericSerif, 48 , FontStyle.Bold, GraphicsUnit.Pixel);
34 Random r = new Random();
35
36 // 合法随机显示字符列表
37 string strLetters = " abcdefghijklmnopqrstuvwxyzABCDEFGHIJKLMNOPQRSTUVWXYZ1234567890 " ;
38 StringBuilder s = new StringBuilder();
39
40 // 将随机生成的字符串绘制到图片上
41 for ( int i = 0 ; i < intLength; i ++ )
42 {
43 s.Append(strLetters.Substring(r.Next( 0 , strLetters.Length - 1 ), 1 ));
44 g.DrawString(s[s.Length - 1 ].ToString(), font, new SolidBrush(Color.Blue), i * 38 , r.Next( 0 , 15 ));
45 }
46
47 // 生成干扰线条
48 Pen pen = new Pen( new SolidBrush(Color.Blue), 2 );
49 for ( int i = 0 ; i < 10 ; i ++ )
50 {
51 g.DrawLine(pen, new Point(r.Next( 0 , 199 ), r.Next( 0 , 59 )), new Point(r.Next( 0 , 199 ), r.Next( 0 , 59 )));
52 }
53 b.Save(hc.Response.OutputStream, ImageFormat.Gif);
54 hc.Session[strIdentify] = s.ToString(); // 先保存在Session中,验证与用户输入是否一致
55 hc.Response.End();
56
57 }
58
59 /// <summary>
60 /// 表示此类实例是否可以被多个请求共用(重用可以提高性能)
61 /// </summary>
62 public bool IsReusable
63 {
64 get
65 {
66 return true ;
67 }
68 }
69 }
70
2. 前台页面代码
1
<
asp:Login ID
=
"
Login1
"
runat
=
"
server
"
BackColor
=
"
#EFF3FB
"
BorderColor
=
"
#B5C7DE
"
BorderPadding
=
"
4
"
BorderStyle
=
"
Solid
"
BorderWidth
=
"
1px
"
Font
-
Names
=
"
Verdana
"
Font
-
Size
=
"
0.8em
"
ForeColor
=
"
#333333
"
OnAuthenticate
=
"
Login1_Authenticate
"
>
2 < TitleTextStyle BackColor = " #507CD1 " Font - Bold = " True " Font - Size = " 0.9em " ForeColor = " White " />
3 < InstructionTextStyle Font - Italic = " True " ForeColor = " Black " />
4 < TextBoxStyle Font - Size = " 0.8em " />
5 < LoginButtonStyle BackColor = " White " BorderColor = " #507CD1 " BorderStyle = " Solid " BorderWidth = " 1px "
6 Font - Names = " Verdana " Font - Size = " 0.8em " ForeColor = " #284E98 " />
7 < LayoutTemplate >
8 < table border = " 0 " cellpadding = " 4 " cellspacing = " 0 " style = " border-collapse: collapse " >
9 < tr >
10 < td style = " width: 292px " >
11 < table border = " 0 " cellpadding = " 0 " >
12 < tr >
13 < td align = " center " colspan = " 2 " style = " font-weight: bold; font-size: 0.9em; color: white;
14 background - color: #507cd1 " >
15 登录 </ td >
16 </ tr >
17 < tr >
18 < td align = " left " style = " width: 84px; height: 31px; " >
19 < asp:Label ID = " UserNameLabel " runat = " server " AssociatedControlID = " UserName " > 用户名: </ asp:Label ></ td >
20 < td style = " height: 31px; width: 215px; " >
21 < asp:TextBox ID = " UserName " runat = " server " Font - Size = " 0.8em " Width = " 113px " ></ asp:TextBox >
22 < asp:RequiredFieldValidator ID = " UserNameRequired " runat = " server " ControlToValidate = " UserName "
23 ErrorMessage = " 必须填写“用户名”。 " ToolTip = " 必须填写“用户名”。 " ValidationGroup = " Login1 " >*</ asp:RequiredFieldValidator >
24 </ td >
25 </ tr >
26 < tr >
27 < td align = " left " style = " width: 84px " >
28 < asp:Label ID = " PasswordLabel " runat = " server " AssociatedControlID = " Password " > 密码: </ asp:Label ></ td >
29 < td style = " width: 215px " >
30 < asp:TextBox ID = " Password " runat = " server " Font - Size = " 0.8em " TextMode = " Password " ></ asp:TextBox >
31 < asp:RequiredFieldValidator ID = " PasswordRequired " runat = " server " ControlToValidate = " Password "
32 ErrorMessage = " 必须填写“密码”。 " ToolTip = " 必须填写“密码”。 " ValidationGroup = " Login1 " >*</ asp:RequiredFieldValidator >
33 </ td >
34 </ tr >
35 < tr >
36 < td style = " width: 84px; height: 4px; " align = " left " >
37 验证码: </ td >
38 < td valign = " middle " style = " height: 31px; width: 215px; " align = " left " >
39 < asp:TextBox ID = " TextBox1 " runat = " server " Font - Size = " 0.8em " TextMode = " Password " ></ asp:TextBox >& nbsp;
40
2 < TitleTextStyle BackColor = " #507CD1 " Font - Bold = " True " Font - Size = " 0.9em " ForeColor = " White " />
3 < InstructionTextStyle Font - Italic = " True " ForeColor = " Black " />
4 < TextBoxStyle Font - Size = " 0.8em " />
5 < LoginButtonStyle BackColor = " White " BorderColor = " #507CD1 " BorderStyle = " Solid " BorderWidth = " 1px "
6 Font - Names = " Verdana " Font - Size = " 0.8em " ForeColor = " #284E98 " />
7 < LayoutTemplate >
8 < table border = " 0 " cellpadding = " 4 " cellspacing = " 0 " style = " border-collapse: collapse " >
9 < tr >
10 < td style = " width: 292px " >
11 < table border = " 0 " cellpadding = " 0 " >
12 < tr >
13 < td align = " center " colspan = " 2 " style = " font-weight: bold; font-size: 0.9em; color: white;
14 background - color: #507cd1 " >
15 登录 </ td >
16 </ tr >
17 < tr >
18 < td align = " left " style = " width: 84px; height: 31px; " >
19 < asp:Label ID = " UserNameLabel " runat = " server " AssociatedControlID = " UserName " > 用户名: </ asp:Label ></ td >
20 < td style = " height: 31px; width: 215px; " >
21 < asp:TextBox ID = " UserName " runat = " server " Font - Size = " 0.8em " Width = " 113px " ></ asp:TextBox >
22 < asp:RequiredFieldValidator ID = " UserNameRequired " runat = " server " ControlToValidate = " UserName "
23 ErrorMessage = " 必须填写“用户名”。 " ToolTip = " 必须填写“用户名”。 " ValidationGroup = " Login1 " >*</ asp:RequiredFieldValidator >
24 </ td >
25 </ tr >
26 < tr >
27 < td align = " left " style = " width: 84px " >
28 < asp:Label ID = " PasswordLabel " runat = " server " AssociatedControlID = " Password " > 密码: </ asp:Label ></ td >
29 < td style = " width: 215px " >
30 < asp:TextBox ID = " Password " runat = " server " Font - Size = " 0.8em " TextMode = " Password " ></ asp:TextBox >
31 < asp:RequiredFieldValidator ID = " PasswordRequired " runat = " server " ControlToValidate = " Password "
32 ErrorMessage = " 必须填写“密码”。 " ToolTip = " 必须填写“密码”。 " ValidationGroup = " Login1 " >*</ asp:RequiredFieldValidator >
33 </ td >
34 </ tr >
35 < tr >
36 < td style = " width: 84px; height: 4px; " align = " left " >
37 验证码: </ td >
38 < td valign = " middle " style = " height: 31px; width: 215px; " align = " left " >
39 < asp:TextBox ID = " TextBox1 " runat = " server " Font - Size = " 0.8em " TextMode = " Password " ></ asp:TextBox >& nbsp;
40
<img width="100px" height="25px" src="ValidateImageHandler.ashx"/>
</
td
>
41 </ tr >
42 < tr >
43 < td align = " left " colspan = " 2 " style = " color: red " >
44 < asp:CheckBox ID = " RememberMe " runat = " server " Text = " 下次记住我。 " />& nbsp; </ td >
45 </ tr >
46 < tr >
47 < td align = " right " colspan = " 2 " >
48 < asp:Button ID = " LoginButton " runat = " server " BackColor = " White " BorderColor = " #507CD1 "
49 BorderStyle = " Solid " BorderWidth = " 1px " CommandName = " Login " Font - Names = " Verdana "
50 Font - Size = " 0.8em " ForeColor = " #284E98 " Text = " 登录 " ValidationGroup = " Login1 " />
51 </ td >
52 </ tr >
53 </ table >
54 </ td >
55 </ tr >
56 </ table >
57 </ LayoutTemplate >
58
59 </ asp:Login >
60
41 </ tr >
42 < tr >
43 < td align = " left " colspan = " 2 " style = " color: red " >
44 < asp:CheckBox ID = " RememberMe " runat = " server " Text = " 下次记住我。 " />& nbsp; </ td >
45 </ tr >
46 < tr >
47 < td align = " right " colspan = " 2 " >
48 < asp:Button ID = " LoginButton " runat = " server " BackColor = " White " BorderColor = " #507CD1 "
49 BorderStyle = " Solid " BorderWidth = " 1px " CommandName = " Login " Font - Names = " Verdana "
50 Font - Size = " 0.8em " ForeColor = " #284E98 " Text = " 登录 " ValidationGroup = " Login1 " />
51 </ td >
52 </ tr >
53 </ table >
54 </ td >
55 </ tr >
56 </ table >
57 </ LayoutTemplate >
58
59 </ asp:Login >
60
3.这里因为使用的是默认 *.asah处理文件类型,在machine.config文件中已经有此类型的默认注册,
因为这里不需要注册
4
注意:
1.再注册一下也不会出错,会覆盖machine.config文件配置
2.如果在同一个配置文件中注册多次,默认后者也会覆盖前者.
3.如果其它格式(系统默认没有注册)的,务必要在Web.config文件中注册一下.
(三).示例代码下载