ASP.NET
应用
-DataGrid
使用最佳实践
列表控件是如何工作的
•
DataSource
属性:
最简单地讲,就是一组相同特征的对象或者一个相同对象的集合
•
Items
集合:每一个列表绑定控件都有一个
Items
集合,集合中的每一个
Item
是
DataSource
所指定的一个对象
数据绑定
–列表绑定控件基于
ASP.NET
框架,需要你明确地进行数据绑定。这就意味着:只有当
DataBind
方法被调用时,才真正需要轮询其
DataSource
所代表的数据。
–当
DataBind
方法被调用时,列表绑定控件将轮询
DataSource
,创建
Items
集合,并从
DataSource
取回数据,以初始化
Items
集合
DataGrid
基本使用
•
DataGrid
控件可用于创建各种样式的表格。它还支持对项目的选择和操作,是最复杂、功能最强大!
•
DataGrid
的
AutoGenerateColumns
属性缺省是
True
。当
AutoGenerateColumns
为
True
时,
DataGrid
将检查其数据源和其对象映射,并为每一个共有属性或者字段创建一个列。
•
每一个自动产生的列称为一个
BoundColumn
(绑定列)。绑定列根据其数据表对应列的数据类型,自动将其转化为一个字符串,显示在表格的一个单元中。
•
DataGrid
列的类型包括:
–
绑定列
–
模板列
–
编辑和删除列
•
事件
–
编辑
–
取消
–
更新
–
删除
–
分页
•
日期的显示
d
将日显示为不带前导零的数字(如
1
)。
dd
将日显示为带前导零的数字(如
01
)。
ddd
将日显示为缩写形式(例如
Sun
)。
dddd
将日显示为全名(例如
Sunday
)。
M
将月份显示为不带前导零的数字(如一月表示为
1
)
MM
将月份显示为带前导零的数字(例如
01/12/01
)。
MMM
将月份显示为缩写形式(例如
Jan
)。
MMMM
将月份显示为完整月份名(例如
January
)。
h
使用
12
小时制将小时显示为不带前导零的数字(例如
1:15:15 PM
)。
hh
使用
12
小时制将小时显示为带前导零的数字(例如
01:15:15 PM
)。
H
使用
24
小时制将小时显示为不带前导零的数字(例如
1:15:15
)。
HH
使用
24
小时制将小时显示为带前导零的数字(例如
01:15:15
)。
m
将分钟显示为不带前导零的数字(例如
12:1:15
)。
mm
将分钟显示为带前导零的数字(例如
12:01:15
)。
s
将秒显示为不带前导零的数字(例如
12:15:5
)。
ss
将秒显示为带前导零的数字(例如
12:15:05
)。
y
将年份
(0-9)
显示为不带前导零的数字。
yy
以带前导零的两位数字格式显示年份(如果适用)。
yyy
以三位数字格式显示年份。
yyyy
以四位数字格式显示年份。
•
DataGrid
控件可用于创建各种样式的表格。它还支持对项目的选择和操作,是最复杂、功能最强大!
•
DataGrid
的
AutoGenerateColumns
属性缺省是
True
。当
AutoGenerateColumns
为
True
时,
DataGrid
将检查其数据源和其对象映射,并为每一个共有属性或者字段创建一个列。
•
每一个自动产生的列称为一个
BoundColumn
(绑定列)。绑定列根据其数据表对应列的数据类型,自动将其转化为一个字符串,显示在表格的一个单元中。
绑定数据代码
(
在
Page_Load
处添加
):
private
void Page_Load(object sender, System.EventArgs e)
{
//
在此处放置用户代码以初始化页面
if(!IsPostBack)
BindData();
}
private
void BindData()
{
string
strCon = System.Configurati
on.ConfigurationSettings.AppSettings["DSN"];
SqlConnection con = new SqlConnection(strCon);
SqlDataAdapter da = new SqlDataAdapter("Select * from tbStudentinfo",con);
DataSet ds = new DataSet();
da.Fill(ds,"studentinfo");
dgShow.DataSource = ds.Tables["studentinfo"].DefaultView;
dgShow.DataBind();
}
绑定列使用
如下图:(需设置
”
页眉文本
”
和
”
数据字段
”
)
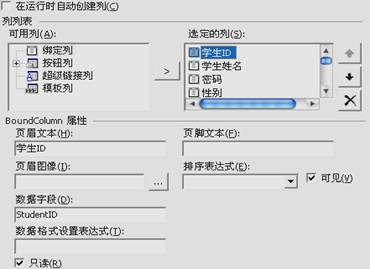
当数据为只读列是,选上只读选项.
按钮列响应事件
,
需重新绑定数据,代码如下:
private
void dgShow_EditCommand(object source, System.Web.UI.WebControls.DataGridCommandEventArgs e)
{
dgShow.EditItemIndex = e.Item.ItemIndex;
BindData();
}
private
void dgShow_CancelCommand(object source, System.Web.UI.WebControls.DataGridCommandEventArgs e)
{
dgShow.EditItemIndex = -1;
BindData();
}
响应按钮删除事件
代码如下:
private
void dgShow_DeleteCommand(object source, System.Web.UI.WebControls.DataGridCommandEventArgs e)
{
if
(dgShow.Items.Count==1) //
这几行代码防止只剩下一行数据
{
if
(dgShow.CurrentPageIndex!=0)
dgShow.CurrentPageIndex = dgShow.CurrentPageIndex-1;
}
string
strSql = "delete from tbStudentinfo where studentid="+e.Item.Cells[0].Text+"";
ExecuteSql(strSql);
BindData();
}
//
说明:执行制定SQL语句/
///
private
void ExecuteSql(string strSql)
{
try
{
string
strconn = System.Configuration.ConfigurationSettings.AppSettings["DSN"];//
从Web.config中读取
SqlConnection conn =new SqlConnection(strconn);
SqlCommand com = new SqlCommand(strSql,conn);
conn.Open();
com.ExecuteNonQuery();
conn.Close();
}
catch
(Exception e)
{
Response.Write("<script language = 'javascript'>alert('"+e.Message+"');</script>") ;
}
}
响应更新按钮事件
代码如下:
private
void dgShow_UpdateCommand(object source, System.Web.UI.WebControls.DataGridCommandEventArgs e)
{
string strStudentID = e.Item.Cells[0].Text;//
处于非编辑状态
string
strName = ((TextBox)(e.Item.Cells[1].Controls[0])).Text;//
处于编辑状态
string strPass =((TextBox)(e.Item.Cells[2].Controls[0])).Text;
string
strSex = ((CheckBox)(e.Item.Cells[3].FindControl("cbSex"))).Checked?"1":"0";
string
strBirthday =((TextBox)(e.Item.Cells[4].Controls[0])).Text;
string
strEmail =((TextBox)(e.Item.Cells[5].Controls[0])).Text;
string
strSql = "update tbStudentinfo set StudentName='"+strName+"',StudentPass='"+strPass+"'";
strSql+=",Sex="+strSex+",Birthday='"+strBirthday+"',Email='"+strEmail+"' where studentid="+strStudentID+"";
ExecuteSql(strSql);
dgShow.EditItemIndex = -1;
BindData();
}
分页
具体操作如图:
响应分页
PageIndexChanged
事件代码如下:
private
void dgShow_PageIndexChanged(object source, System.Web.UI.WebControls.DataGridPageChangedEventArgs e)
{
dgShow.CurrentPageIndex = e.NewPageIndex;
BindData();
}
在DataGorad
添加RadioButton
操作如下:
1.
添加模板列
2.
编辑模板
3.
在
ItemTemplate
处添加
RadioButton
4.
RadioButton
属性
GroupName
需一样
,
即设置为一组
RadioButton
绑定数据
库数据操作如下:
1.
设置RadioButton数据源(DataBindings)
2.
选择Checked属性,自定义绑定表达式输入表达式.
例如:
DataBinder.Eval(Container, "DataItem.Sex")
!(bool)DataBinder.Eval(Container, "DataItem.Sex")
日期格式设置
:
在列的数据格式设置表达式处填入静态式.
例如:
{0:yyyy-M-d}
{0:yyyy-MM-dd}
日期格式参考上日期格式表.
超级链接列:
添加
自定义删除
:
1.
添加模板列.
2.
在ItemTemplate处添加删除按钮.
3.
更改按钮名字btnDelete,添加CommandName属性 UserDelete.
响应自定义
删除事件:
1.
添加DataGrid ItemCreated事件.
private
void dgShow_ItemCreated(object sender, System.Web.UI.WebControls.DataGridItemEventArgs e)
{
switch
(e.Item.ItemType)
{
case
ListItemType.Item:
case
ListItemType.EditItem:
case
ListItemType.AlternatingItem:
Button myDeleteButton = (Button)e.Item.FindControl("btnDelete");
myDeleteButton.Text = "
删除此行";
myDeleteButton.Attributes.Add("onclick", "return confirm('
您真的要删除第 " + e.Item.ItemIndex.ToString() + " 行吗?');");
break;
}
}
2.
添加DataGrid ItemCommand事件.
private
void dgShow_ItemCommand(object source, System.Web.UI.WebControls.DataGridCommandEventArgs e)
{
if(e.CommandName=="UserDelete")
dgShow_DeleteCommand(source,e);
}
多行操作
.
1.
添加模板列,如右图:
2.
设置CheckBox的AutoPostBack属性为True
3.
修改HTML页面,在
”
全选
”
代码处修改为:
<asp:CheckBox id="cbAll" runat="server"
OnCheckedChanged="CheckAll"
Text="
全选"
AutoPostBack="True"></asp:CheckBox>
4.
添加响应
”
全选
”
按钮函数
public
void CheckAll(object sender, System.EventArgs e)
{
CheckBox cbAll = (CheckBox)sender;
if(cbAll.Text=="
全选")
{
foreach(DataGridItem dgi in dgShow.Items)
{
CheckBox cb = (CheckBox)dgi.FindControl("cbSelect");
cb.Checked = cbAll.Checked;
}
}
}
5.
添加多行操作按钮:(例如删除)
a.
添加删除按钮属性.
private
void Page_Load(object sender, System.EventArgs e)
{
//
在此处放置用户代码以初始化页面
btnDelete.Attributes.Add("onclick", "return confirm('
您真的要删除所选项吗?');");
if
(!IsPostBack)
BindData();
}
b.
响应删除按钮事件:
private
void btnDelete_Click(object sender, System.EventArgs e)
{
foreach(DataGridItem dgi in dgShow.Items)
{
CheckBox cb = (CheckBox)dgi.FindControl("cbSelect");
if
(cb.Checked)
{
//
以下执行删除操作
int nID = int.Parse(dgi.Cells[0].Text);
string strSql = "delete from tbStudentinfo where studentid="+nID;
ExecuteSql(strSql);
}
}
dgShow.CurrentPageIndex = 0;
BindData();
}
在DataGrid
添加DropDownList控件
1.
添加模板列.
两个DropDownList ID 分别为ddlSexI和ddlSexE.
添加Items
Text:
男 Value:1
Text:
女 Value:0
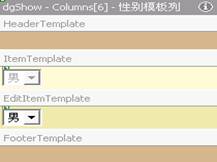
2.
绑定数据库数据:
private
void BindData()
{
string strCon = System.Configuration.ConfigurationSettings.AppSettings["DSN"];
SqlConnection con = new SqlConnection(strCon);
SqlDataAdapter da = new SqlDataAdapter("Select * from tbStudentinfo",con);
DataSet ds = new DataSet();
da.Fill(ds,"studentinfo");
dgShow.DataSource = ds.Tables["studentinfo"].DefaultView;
dgShow.DataBind();
foreach(DataGridItem dgi in dgShow.Items)
{
//
以下绑定非编辑状态下拉列表
DropDownList ddI = (DropDownList)dgi.FindControl("ddlSexI");
if(ddI!=null)
{
ool bSex = (bool)ds.Tables["studentinfo"].Rows[dgi.ItemIndex]["Sex"];
if(bSex)
ddI.SelectedIndex = 0;
else
ddI.SelectedIndex = 1;
}
//
以下绑定编辑状态下拉列表
DropDownList ddE = (DropDownList)dgi.FindControl("ddlSexE");
if(ddE!=null)
{
bool bSex = (bool)ds.Tables["studentinfo"].Rows[dgi.ItemIndex]["Sex"];
if(bSex)
ddE.SelectedIndex = 0;
else
ddE.SelectedIndex = 1;
}
}
}
3.
更新数据:
private
void dgShow_UpdateCommand(object source, System.Web.UI.WebControls.DataGridCommandEventArgs e)
{
string strStudentID = e.Item.Cells[0].Text;//
处于非编辑状态
string strName = ((TextBox)(e.Item.Cells[1].Controls[0])).Text;//
处于编辑状态
string strPass =((TextBox)(e.Item.Cells[2].Controls[0])).Text;
string strSex = ((DropDownList)(e.Item.FindControl("ddlSexE"))).SelectedItem.Value;
string strBirthday =((TextBox)(e.Item.Cells[4].Controls[0])).Text;
string strEmail =((TextBox)(e.Item.Cells[5].Controls[0])).Text;
string
strSql = "update tbStudentinfo set StudentName='"+strName+"',StudentPass='"+strPass+"'";
strSql +=",Sex="+strSex+",Birthday='"+strBirthday+"',Email='"+strEmail+"' where studentid="+strStudentID+"";
ExecuteSql(strSql);
dgShow.EditItemIndex = -1;
BindData();
}
统计数据
:
1.
设置
DataGrid
的ShowFooter属性为True
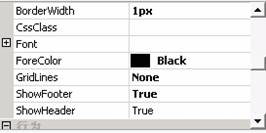
2.
添加代码显示统计
:
private
void BindData()
{
string strCon = System.Configuration.ConfigurationSettings.AppSettings["DSN"];
SqlConnection con = new SqlConnection(strCon);
SqlDataAdapter da = new SqlDataAdapter("Select * from tbStudentinfo",con);
DataSet ds = new DataSet();
da.Fill(ds,"studentinfo");
dgShow.DataSource = ds.Tables["studentinfo"].DefaultView;
dgShow.DataBind();
//
以下作分数和的统计
int count=0;
for (int i = 0; i < ds.Tables[0].Rows.Count; i++)
{
count += int.Parse(ds.Tables[0].Rows[i]["Score"].ToString());
}
int nAv = count/ds.Tables[0].Rows.Count;
foreach(DataGridItem dgi in dgShow.Controls[0].Controls)
{
if (dgi.ItemType == ListItemType.Footer)
dgi.Cells[6].Text = "
平均:"+nAv.ToString();
}
}
如何调整DataGrid编程状态下的TextBox宽度
1.
在DataGrid添加ItemDataBound事件.
2.
响应ItemDataBound事件代码:
private
void dgShow_ItemDataBound(object sender, System.Web.UI.WebControls.DataGridItemEventArgs e)
{
if (e.Item.ItemType == ListItemType.EditItem)
{
for (int i=0;i<e.Item.Cells.Count;i++)
{
if(e.Item.Cells[i].Controls.Count>0)
{
try
{
TextBox t =(TextBox)e.Item.Cells[i].Controls[0];
t.Width=130;
}
catch(Exception ee)
{
}
}
}
}
}
隐藏DataGrid里面某一列数据
1.
在数据绑定处添加代码如下:
private
void BindData()
{
string strCon = System.Configuration.ConfigurationSettings.AppSettings["DSN"];
SqlConnection con = new SqlConnection(strCon);
SqlDataAdapter da = new SqlDataAdapter("Select * from tbStudentinfo",con);
DataSet ds = new DataSet();
da.Fill(ds,"studentinfo");
dgShow.DataSource = ds.Tables["studentinfo"].DefaultView;
dgShow.DataBind();
dgShow.Columns[0].Visible = false;
}
如何装DataGrid导出成一个Excel文件
1.
添加导出按钮.
2.
响应按钮点击事件,添加代码如下:
private
void btnMIME_Click(object sender, System.EventArgs e)
{
Response.ContentType = "application/vnd.ms-excel";
Response.Charset = "";
this.EnableViewState = false;
System.IO.StringWriter sw = new System.IO.StringWriter();
System.Web.UI.HtmlTextWriter hw = new System.Web.UI.HtmlTextWriter(sw);
int nCur = dgShow.CurrentPageIndex;
int nSize = dgShow.PageSize;
dgShow.AllowPaging = false;
BindData();
dgShow.Columns[7].Visible =false;
dgShow.RenderControl(hw);
dgShow.Columns[7].Visible =true;
//
以下恢复分页
dgShow.AllowPaging = true;
dgShow.CurrentPageIndex = nCur;
dgShow.PageSize = nSize;
BindData();
Response.Write(sw.ToString());
Response.End();
}