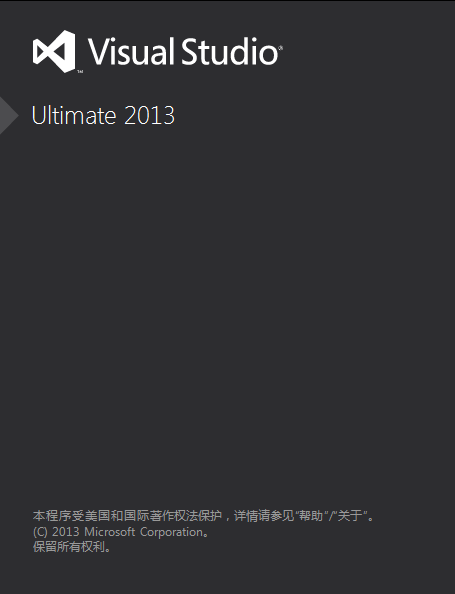
题目
单向循环链表的操作
解决代码及点评
#include <stdio.h>
#include <stdlib.h>
#include <time.h>
#include <malloc.h>
#include<assert.h>
typedef struct LoopLink
{
int nValue;
struct LoopLink *pNext;
}LoopLink, *PLoopLink;
PLoopLink Create()
{
PLoopLink pHead = (PLoopLink)malloc(sizeof(LoopLink));
if (NULL == pHead)
{
printf("分配内存失败!\n");
}
pHead->nValue = -9999;
pHead->pNext = pHead;
return pHead;
}
void Insert(PLoopLink pHead, int nValue)
{
if (NULL == pHead)
{
printf("链表未创建成功!\n");
return ;
}
PLoopLink pCur = pHead;
PLoopLink pTmp = (PLoopLink)malloc(sizeof(LoopLink));
pTmp->nValue = nValue;
pTmp->pNext = NULL;
while (pCur->pNext != pHead)
{
if (pCur->pNext->nValue > nValue)
{
break;
}
pCur = pCur->pNext;
}
pTmp->pNext = pCur->pNext;
pCur->pNext = pTmp;
}
void Delete(PLoopLink pHead, int nValue)
{
if (pHead == NULL)
{
printf("链表未创建成功!\n");
return;
}
PLoopLink pCur = pHead;
while (pCur->pNext!= pHead)
{
if (pCur->pNext->nValue == nValue)
{
PLoopLink pTmp = pCur->pNext;
pCur->pNext = pTmp->pNext;
free(pTmp);
pTmp = NULL;
}
else
{
pCur = pCur->pNext;
}
}
}
PLoopLink Find(PLoopLink pHead, int nValue)
{
if (pHead == NULL)
{
printf("链表未创建成功!\n");
return NULL;
}
PLoopLink pCur = pHead;
while (pCur->pNext!= pHead)
{
if (pCur->pNext->nValue == nValue)
{
return pCur->pNext;
}
else
{
pCur = pCur->pNext;
}
}
return NULL;
}
bool IsEmpty(PLoopLink pHead)
{
if (NULL == pHead)
{
printf("链表未创建成功!\n");
}
return pHead->pNext == pHead;
}
void Print(PLoopLink pHead)
{
if (pHead == NULL)
{
printf("链表未创建成功!\n");
return;
}
if (pHead->pNext == pHead)
{
printf("链表为空!\n");
return;
}
PLoopLink pCur = pHead->pNext;
while (pCur != pHead)
{
printf("%d ", pCur->nValue);
pCur = pCur->pNext;
}
printf("\n");
}
int main()
{
PLoopLink pHead = Create();
// printf("%d\n", IsEmpty(pHead));
Insert(pHead, 1);
Insert(pHead, 5);
Insert(pHead, 8);
Insert(pHead, 4);
Insert(pHead, 0);
if (NULL != Find(pHead, 2))
{
printf("链表中有该数据!\n");
}
else
{
printf("链表中没有该数据!\n");
}
Delete(pHead, 8);
Print(pHead);
system("pause");
return 0;
}
代码下载及其运行
代码下载地址:http://download.csdn.net/detail/yincheng01/6858815
解压密码:c.itcast.cn
下载代码并解压后,用VC2013打开interview.sln,并设置对应的启动项目后,点击运行即可,具体步骤如下:
1)设置启动项目:右键点击解决方案,在弹出菜单中选择“设置启动项目”
2)在下拉框中选择相应项目,项目名和博客编号一致
3)点击“本地Windows调试器”运行
程序运行结果