12_常见对象(数组高级冒泡排序原理图解)
A:上图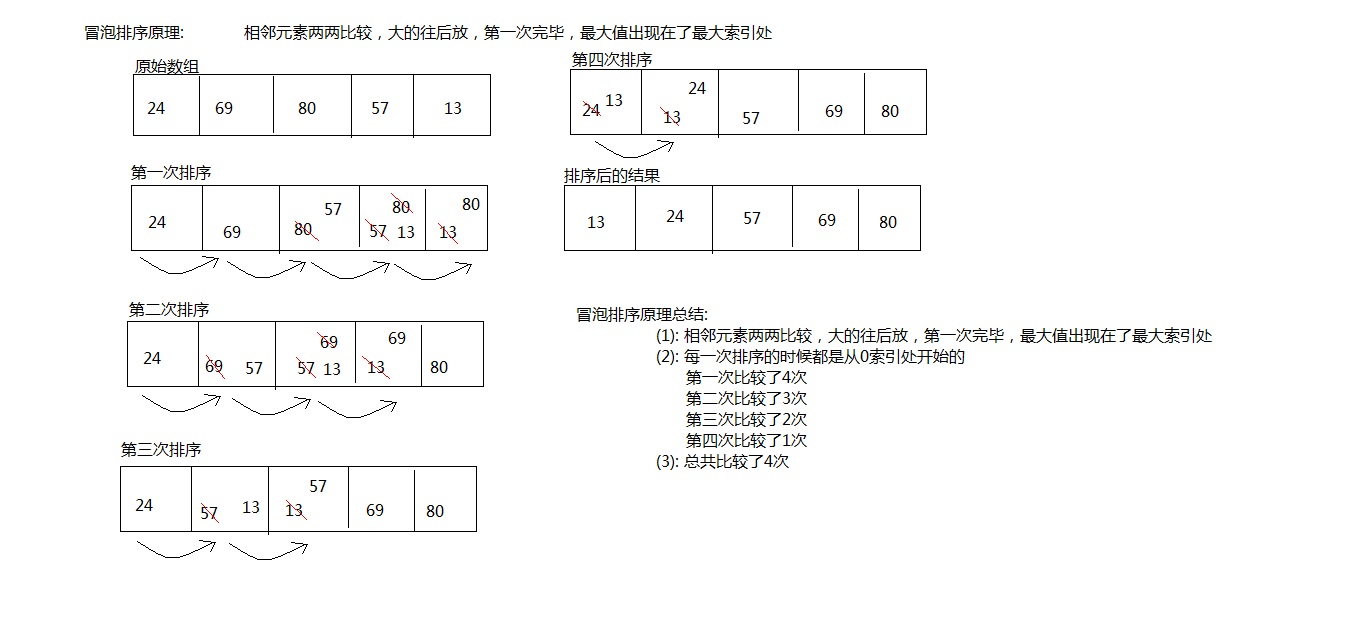
B:冒泡排序原理
相邻元素两两比较,大的往后放,第一次完毕,最大值出现在了最大索引处
public class BubbleSort {
public static void main(String[] args) {
// 定义一个数组
int[] arr = {24, 69, 80, 57, 13} ;
// 遍历
System.out.print("排序前: ");
print(arr);
// 排序
bubbleSort2(arr);
// 遍历
System.out.print("排序后: ");
print(arr);
}
/**
* 使用循环改进后的方法
*
*/
public static void bubbleSort2(int[] arr){
for(int y = 0 ; y < arr.length - 1 ; y++){
// arr.length - 1 - y:
// arr.length - 1目的: 是防止数组角标越界
// arr.length - y目的: 提供效率
for(int x = 0 ; x < arr.length - 1 - y; x++){
if(arr[x] > arr[x + 1]){
int temp = arr[x] ;
arr[x] = arr[x + 1];
arr[x + 1] = temp ;
}
}
}
}
/**
* 冒泡排序推理过程
*
*/
public static void bubbleSort(int[] arr){
// 第一次排序
// arr.length - 1 目的 : 防止数组角标越界
for(int x = 0 ; x < arr.length - 1 - 0; x++){
if(arr[x] > arr[x + 1]){
int temp = arr[x] ;
arr[x] = arr[x + 1];
arr[x + 1] = temp ;
}
}
// 第二次排序
for(int x = 0 ; x < arr.length - 1 - 1; x++){
if(arr[x] > arr[x + 1]){
int temp = arr[x] ;
arr[x] = arr[x + 1];
arr[x + 1] = temp ;
}
}
// 第三次排序
for(int x = 0 ; x < arr.length - 1 - 2; x++){
if(arr[x] > arr[x + 1]){
int temp = arr[x] ;
arr[x] = arr[x + 1];
arr[x + 1] = temp ;
}
}
// 第四次排序
for(int x = 0 ; x < arr.length - 1 - 3; x++){
if(arr[x] > arr[x + 1]){
int temp = arr[x] ;
arr[x] = arr[x + 1];
arr[x + 1] = temp ;
}
}
}
/**
* 遍历数组
*
*/
public static void print(int[] arr){
System.out.print("[ ");
for(int x = 0 ; x < arr.length ; x++){
if(x == arr.length - 1){
System.out.println(arr[x] + " ]");
}else {
System.out.print(arr[x] + ", ");
}
}
}
}
13_常见对象(数组高级选择排序原理图解)
A:上图~~!
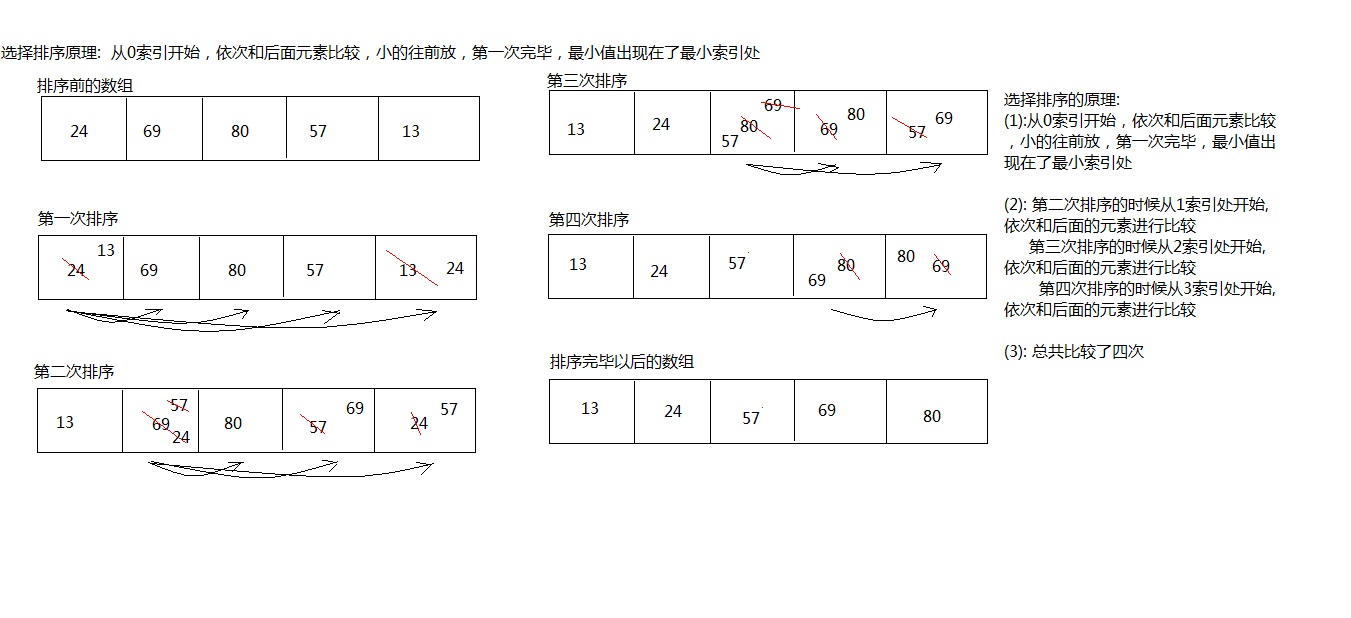
B:选择排序原理
从0索引开始,依次和后面元素比较,小的往前放,第一次完毕,最小值出现在了最小索引处
public class SelectSort {
public static void main(String[] args) {
// 定义一个数组
int[] arr = {24, 69, 80, 57, 13} ;
// 遍历
System.out.print("排序前: ");
BubbleSort.print(arr);
// 排序
selectSort2(arr);
// 遍历
System.out.print("排序前: ");
BubbleSort.print(arr);
}
/**
* 优化后的代码
*
*/
public static void selectSort2(int[] arr){
for(int y = 0 ; y < arr.length - 1 ; y++){
for(int x = 1 + y; x < arr.length ; x++){
if(arr[y] > arr[x]){
int temp = arr[y];
arr[y] = arr[x] ;
arr[x] = temp ;
}
}
}
}
/**
* 推导过程
*
*/
public static void selectSort(int[] arr){
// 第一次排序
// 定义一个int类型的变量, 记录第一次开始比较的索引
int index = 0 ;
for(int x = 1 + 0; x < arr.length ; x++){
if(arr[index] > arr[x]){
int temp = arr[index];
arr[index] = arr[x] ;
arr[x] = temp ;
}
}
// 第二次排序
index = 1 ;
for(int x = 1 + 1 ; x < arr.length ; x++){
if(arr[index] > arr[x]){
int temp = arr[index];
arr[index] = arr[x] ;
arr[x] = temp ;
}
}
// 第三次排序
index = 2 ;
for(int x = 1 + 2 ; x < arr.length ; x++){
if(arr[index] > arr[x]){
int temp = arr[index];
arr[index] = arr[x] ;
arr[x] = temp ;
}
}
// 第四次排序
index = 3 ;
for(int x = 1 + 3 ; x < arr.length ; x++){
if(arr[index] > arr[x]){
int temp = arr[index];
arr[index] = arr[x] ;
arr[x] = temp ;
}
}
}
}
14_常见对象(数组高级二分查找原理图解)
A:上图~~
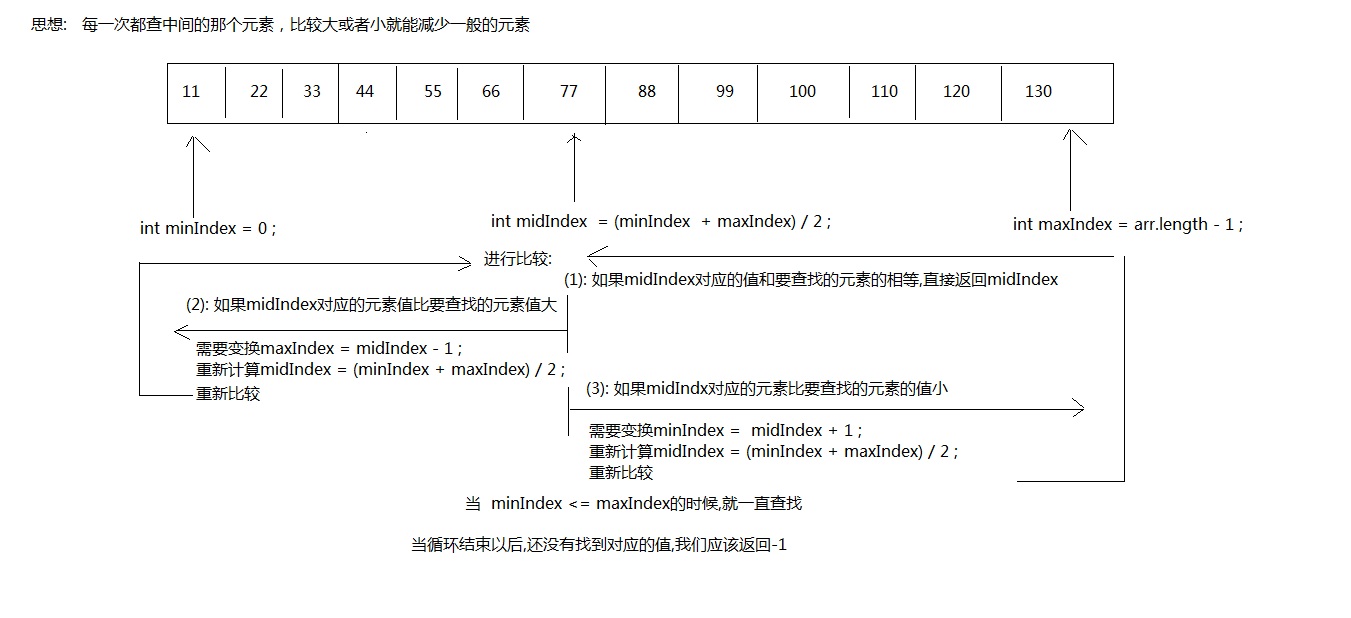
二分查找
前提:数组元素有序
B: 思想: 每一次都查中间的那个元素,比较大或者小就能减少一般的元素
public class BinarySearch {
public static void main(String[] args) {
// 定义一个数组
int[] arr = { 13 , 24 , 57 , 69 , 80} ;
// 调用方法
// 赋值调用
int index = binarySearch(arr , 24);
// 输出
System.out.println(index);
}
/**
* 二分查找
*/
public static int binarySearch(int[] arr , int value){
// 定义三个变量
int minIndex = 0 ;
int maxIndex = arr.length - 1 ;
while(minIndex <= maxIndex){
// 计算midIndex
int midIndex = (minIndex + maxIndex ) >> 1 ;
// 进行比较
if(arr[midIndex] == value){
return midIndex ;
}else if(arr[midIndex] > value){
maxIndex = midIndex - 1;
}else if(arr[midIndex] < value){
minIndex = midIndex + 1 ;
}
}
return -1;
}
}
15_常见对象(基本类型包装类的概述)
A:为什么会有基本类型包装类
为了对基本数据类型进行更多的操作,更方便的操作,java就针对每一种基本数据类型提供了对应的类类型.
B:常用操作: 常用的操作之一:用于基本数据类型与字符串之间的转换。
C:基本类型和包装类的对应
byte Byte
short Short
int Integer
long Long
float Float
double Double
char Character
boolean Boolean
/*
* Integer的构造方法:
* public Integer(int value): 把一个int类型的值转换成一个Integer对象
public Integer(String s): 把一个字符串类型的数据转换成一个Integer对象
*/
public class IntegerDemo {
public static void main(String[] args) {
// 创建对象
// public Integer(int value): 把一个int类型的值转换成一个Integer对象
Integer ii = new Integer(45);
// 输出
System.out.println(ii);
System.out.println("----------------------------");
// public Integer(String s):把一个字符串类型的数据转换成一个Integer对象
// 要求这个字符串必须是数字类型的字符串
Integer ii2 = new Integer("345");
// 输出
System.out.println(ii2);
System.out.println("----------------------------");
// java.lang.NumberFormatException
Integer ii3 = new Integer("abc");
// 输出
System.out.println(ii3);
}
}
public class IntegerDemo {
public static void main(String[] args) {
// 基本数据类型到String类型的转换
// a: 使用 + 进行拼接
int a = 45 ;
String s = a + "";
System.out.println(s);
System.out.println("------------------------");
// b: public static String valueOf(int i): 这是String类中的方法: 必须掌握
String s1 = String.valueOf(a) ;
System.out.println(s1);
System.out.println("------------------------");
// c: int -- Integer -- String
Integer ii = new Integer(a);
String s2 = ii.toString() ;
System.out.println(s2);
System.out.println("------------------------");
// public static String toString(int i): 这是Intger的方法
String s3 = Integer.toString(a) ;
System.out.println(s3);
System.out.println("------------------------");
// String到基本数据类型的转换
// a: String -- Integer -- int
String s4 = "456";
Integer ii2 = new Integer(s4);
// public int intValue()以 int 类型返回该 Integer 的值。
int result = ii2.intValue() ;
System.out.println(result);
System.out.println("------------------------");
// b: public static int parseInt(String s): 将字符串参数作为有符号的十进制整数进行解析
int result2 = Integer.parseInt("789"); // 推荐使用
System.out.println(result2);
}
}
/*
* 构造方法: public BigDecimal(String val)
* 成员方法:
* public BigDecimal add(BigDecimal augend)
public BigDecimal subtract(BigDecimal subtrahend)
public BigDecimal multiply(BigDecimal multiplicand)
public BigDecimal divide(BigDecimal divisor)
public BigDecimal divide(BigDecimal divisor,int scale,int roundingMode)
*/
public class BigDecimalDemo {
public static void main(String[] args) {
// 输出
// System.out.println(1 - 0.9);
// 创建BigDecimal对象
BigDecimal bd = new BigDecimal("12");
BigDecimal bd2 = new BigDecimal("4");
// public BigDecimal add(BigDecimal augend): 加
System.out.println(bd.add(bd2));
System.out.println("------------------");
// public BigDecimal subtract(BigDecimal subtrahend): 减
System.out.println(bd.subtract(bd2));
System.out.println("---------------------");
// public BigDecimal multiply(BigDecimal multiplicand): 乘
System.out.println(bd.multiply(bd2));
System.out.println("----------------------");
// public BigDecimal divide(BigDecimal divisor): 除
System.out.println(bd.divide(bd2));
System.out.println("------------------------");
// public BigDecimal divide(BigDecimal divisor,int scale,int roundingMode):
// scale表示的意思是保留几位小数,roundingMode按照某种方式进行取舍:
// public static final int ROUND_HALF_UP: 四舍五入
// 创建对象
BigDecimal bd3 = new BigDecimal("7.416");
BigDecimal bd4 = new BigDecimal("6");
System.out.println(bd3.divide(bd4, 2 , BigDecimal.ROUND_HALF_UP));
}
}
/**
* 构造方法:
* public BigInteger(String val): 把一个字符串转换成一个BigInteger对象
* 成员方法:
* public BigInteger add(BigInteger val) 加
public BigInteger subtract(BigInteger val) 减
public BigInteger multiply(BigInteger val) 乘
public BigInteger divide(BigInteger val) 除
public BigInteger[] divideAndRemainder(BigInteger val): 返回的是商和余数的数组
*/
public class BigIntegerDemo {
public static void main(String[] args) {
// 获取int的最大值
int maxValue = Integer.MAX_VALUE ;
// 2147483647
System.out.println(maxValue);
// 创建一个Integer对象
// java.lang.NumberFormatException
// Integer ii = new Integer("2147483648");
// System.out.println(ii);
System.out.println("-------------------------------");
// public BigInteger(String val): 把一个字符串转换成一个BigInteger对象
// 创建对象
// BigInteger bi = new BigInteger("2147483648");
// System.out.println(bi);
System.out.println("----------------------------");
// 创建BigInteger对象
BigInteger bi = new BigInteger("12");
BigInteger bi2 = new BigInteger("4");
// public BigInteger add(BigInteger val) 加
System.out.println(bi.add(bi2));
System.out.println("--------------------------");
// public BigInteger subtract(BigInteger val) 减
System.out.println(bi.subtract(bi2));
System.out.println("--------------------------");
// public BigInteger multiply(BigInteger val) 乘
System.out.println(bi.multiply(bi2));
System.out.println("-------------------------");
// public BigInteger divide(BigInteger val) 除
System.out.println(bi.divide(bi2));
System.out.println("---------------------------");
// public BigInteger[] divideAndRemainder(BigInteger val): 返回的是商和余数的数组
BigInteger[] biArr = bi.divideAndRemainder(bi2) ;
// 遍历数组
for (int i = 0; i < biArr.length; i++) {
System.out.println(biArr[i]);
}
}
}
注意:String和int类型的相互转换
A:int – String
a:和”“进行拼接
b:public static String valueOf(int i)
c:int – Integer – String
d:public static String toString(int i)
B:String – int
a:String – Integer – int
b:public static int parseInt(String s)