/**
* 定位及路线规划
* @author ZhangZhaoCheng
*/
public class BaiduLocation extends Activity implements OnClickListener {
private Toast mToast;
private BMapManager mBMapManager;
private MapView mMapView = null;
private MapController mMapController = null;
private LocationClient mLocClient;
private LocationData mLocData;
// 定位图层
private LocationOverlay myLocationOverlay = null;
private boolean isRequest = false;// 是否手动触发请求定位
private boolean isFirstLoc = true;// 是否首次定位
private PopupOverlay mPopupOverlay = null;// 弹出泡泡图层,浏览节点时使用
private View viewCache;
private BDLocation location;
private Button plan_drive;// 自驾车路线
private Button plan_bus;// 公交路线
private Button plan_walk;// 步行路线
private MKRoute mkRoute;// 保存驾车/步行线路数据变量
private TransitOverlay transitOverlay;// 保存公交线路数据变量
private RouteOverlay routeOverlay;
MKSearch mkSearch;
private int nodeIndex = -2;// 节点索引
private int searchType = -1;// 记录搜索的类型,区分驾车/步行和公交
private String gpsX;//传入的X值
private String gpsY;//传入的Y值
@Override
protected void onCreate(Bundle savedInstanceState) {
super.onCreate(savedInstanceState);
M_Application m_Application = (M_Application) getApplication();
m_Application.bMapManager = new BMapManager(getApplicationContext());
m_Application.bMapManager
.init(new M_Application.MKGeneralListenerImpl());
setContentView(R.layout.mapactivity);
gpsX = getIntent().getStringExtra("gpsx");
gpsY = getIntent().getStringExtra("gpsy");
System.out.println("gpsX:"+gpsX+"-------gpsY:"+gpsY);
// 点击按钮手动请求定位
((Button) findViewById(R.id.request))
.setOnClickListener(new OnClickListener() {
@Override
public void onClick(View v) {
requestLocation();
}
});
// 初始化按钮
plan_drive = (Button) findViewById(R.id.plan_driver);
plan_bus = (Button) findViewById(R.id.plan_bus);
plan_walk = (Button) findViewById(R.id.plan_walk);
plan_drive.setOnClickListener(this);// 开车
plan_bus.setOnClickListener(this);// 公交
plan_walk.setOnClickListener(this); // 步行
mMapView = (MapView) findViewById(R.id.bmapView); // 获取百度地图控件实例
mMapController = mMapView.getController(); // 获取地图控制器
mMapController.enableClick(true); // 设置地图是否响应点击事件
mMapController.setZoom(14); // 设置地图缩放级别
mMapView.setBuiltInZoomControls(true); // 显示内置缩放控件
viewCache = LayoutInflater.from(this)
.inflate(R.layout.pop_layout, null);
mPopupOverlay = new PopupOverlay(mMapView, new PopupClickListener() {
@Override
public void onClickedPopup(int arg0) {
mPopupOverlay.hidePop();
}
});
mLocData = new LocationData();
// 实例化定位服务,LocationClient类必须在主线程中声明
mLocClient = new LocationClient(getApplicationContext());
mLocClient.registerLocationListener(new BDLocationListenerImpl());// 注册定位监听接口
/**
* 设置定位参数
*/
LocationClientOption option = new LocationClientOption();
option.setOpenGps(true); // 打开GPRS
option.setAddrType("all");// 返回的定位结果包含地址信息
option.setCoorType("bd09ll");// 返回的定位结果是百度经纬度,默认值gcj02
// option.setScanSpan(5000); // 设置发起定位请求的间隔时间为5000ms
option.disableCache(false);// 禁止启用缓存定位
// option.setPoiNumber(5); //最多返回POI个数
// option.setPoiDistance(1000); //poi查询距离
// option.setPoiExtraInfo(true); //是否需要POI的电话和地址等详细信息
mLocClient.setLocOption(option);
mLocClient.start(); // 调用此方法开始定位
// 定位图层初始化
myLocationOverlay = new LocationOverlay(mMapView);
// 设置定位数据
myLocationOverlay.setData(mLocData);
myLocationOverlay.setMarker(getResources().getDrawable(
R.drawable.location_arrows));
mMapView.getOverlays().clear();//清楚其他图层
// 添加定位图层
mMapView.getOverlays().add(myLocationOverlay);
myLocationOverlay.enableCompass();
// 修改定位数据后刷新图层生效
mMapView.refresh();
/**
* 初始化搜索模块,注册监听
*/
mkSearch = new MKSearch();
mkSearch.init(m_Application.bMapManager, new MKSearchListener() {
@Override
public void onGetSuggestionResult(MKSuggestionResult arg0, int arg1) {
}
@Override
public void onGetShareUrlResult(MKShareUrlResult arg0, int arg1,
int arg2) {
}
@Override
public void onGetPoiResult(MKPoiResult arg0, int arg1, int arg2) {
}
@Override
public void onGetPoiDetailSearchResult(int arg0, int arg1) {
}
@Override
public void onGetTransitRouteResult(MKTransitRouteResult arg0,
int arg1) {
System.out.println("公交");
if (arg1 == MKEvent.ERROR_ROUTE_ADDR) {
return;
}
if (arg1 != 0 || arg0 == null) {
Toast.makeText(BaiduLocation.this, "抱歉,未找到结果",Toast.LENGTH_SHORT).show();
return;
}
searchType = 1;
transitOverlay = new TransitOverlay(BaiduLocation.this,
mMapView);
transitOverlay.setData(arg0.getPlan(0));// 在mapView上创建新覆盖层
mMapView.getOverlays().clear();
mMapView.getOverlays().add(transitOverlay);
mMapView.refresh();
mMapView.getController().zoomToSpan(
transitOverlay.getLatSpanE6(),
transitOverlay.getLonSpanE6());
mMapView.getController().animateTo(arg0.getStart().pt);
// 重置路线节点索引,节点浏览时使用
nodeIndex = 0;
}
@Override
public void onGetWalkingRouteResult(MKWalkingRouteResult arg0,
int arg1) {
System.out.println("步行");
if (arg1 == MKEvent.ERROR_ROUTE_ADDR) {
return;
}
if (arg1 != 0 || arg0 == null) {
Toast.makeText(BaiduLocation.this, "抱歉,未找到结果",
Toast.LENGTH_SHORT).show();
return;
}
searchType = 2;
routeOverlay = new RouteOverlay(BaiduLocation.this, mMapView);
routeOverlay.setData(arg0.getPlan(0).getRoute(0));
mMapView.getOverlays().clear();
mMapView.getOverlays().add(routeOverlay);
mMapView.refresh();
mMapView.getController().zoomToSpan(
routeOverlay.getLatSpanE6(),
routeOverlay.getLonSpanE6());
mMapView.getController().animateTo(arg0.getStart().pt);
// 将路线数据保存给全局变量
mkRoute = arg0.getPlan(0).getRoute(0);
// 重置路线节点索引,节点浏览时使用
nodeIndex = -1;
}
@Override
public void onGetDrivingRouteResult(MKDrivingRouteResult arg0,
int arg1) {
System.out.println("驾车");
if (arg1 == MKEvent.ERROR_ROUTE_ADDR) {
return;
}
if (arg1 != 0 || arg0 == null) {
Toast.makeText(BaiduLocation.this, "抱歉,未找到结果",
Toast.LENGTH_SHORT).show();
return;
}
searchType = 0;
routeOverlay = new RouteOverlay(BaiduLocation.this, mMapView);
routeOverlay.setData(arg0.getPlan(0).getRoute(0));
mMapView.getOverlays().clear();
mMapView.getOverlays().add(routeOverlay);
mMapView.refresh();
mMapView.getController().zoomToSpan(
routeOverlay.getLatSpanE6(),
routeOverlay.getLonSpanE6());
mMapView.getController().animateTo(arg0.getStart().pt);
// 将路线数据保存给全局变量
mkRoute = arg0.getPlan(0).getRoute(0);
// 重置路线节点索引,节点浏览时使用
nodeIndex = -1;
}
@Override
public void onGetBusDetailResult(MKBusLineResult arg0, int arg1) {
}
@Override
public void onGetAddrResult(MKAddrInfo arg0, int arg1) {
}
});
}
/**
* 定位接口,需要实现两个方法
* @author xiaanming
*
*/
public class BDLocationListenerImpl implements BDLocationListener {
/**
* 接收异步返回的定位结果,参数是BDLocation类型参数
*/
@Override
public void onReceiveLocation(BDLocation location) {
System.out.println("==onReceiveLocation===");
if (location == null) {
return;
}
BaiduLocation.this.location = location;
mLocData.latitude = location.getLatitude();
mLocData.longitude = location.getLongitude();
// 如果不显示定位精度圈,将accuracy赋值为0即可
mLocData.accuracy = location.getRadius();
mLocData.direction = location.getDerect();
// 将定位数据设置到定位图层里
myLocationOverlay.setData(mLocData);
mMapView.refresh();
if (isFirstLoc || isRequest) {
mMapController.animateTo(new GeoPoint((int) (location
.getLatitude() * 1e6),
(int) (location.getLongitude() * 1e6)));
showPopupOverlay(location);
isRequest = false;
}
isFirstLoc = false;
}
/**
* 接收异步返回的POI查询结果,参数是BDLocation类型参数
*/
@Override
public void onReceivePoi(BDLocation poiLocation) {
}
}
/**
* 常用事件监听,用来处理通常的网络错误,授权验证错误等
*
* @author xiaanming
*
*/
public class MKGeneralListenerImpl implements MKGeneralListener {
/**
* 一些网络状态的错误处理回调函数
*/
@Override
public void onGetNetworkState(int iError) {
if (iError == MKEvent.ERROR_NETWORK_CONNECT) {
showToast("您的网络出错啦!");
}
}
/**
* 授权错误的时候调用的回调函数
*/
@Override
public void onGetPermissionState(int iError) {
if (iError == MKEvent.ERROR_PERMISSION_DENIED) {
showToast("API KEY错误, 请检查!");
}
}
}
private class LocationOverlay extends MyLocationOverlay {
public LocationOverlay(MapView arg0) {
super(arg0);
}
@Override
protected boolean dispatchTap() {
showPopupOverlay(location);
return super.dispatchTap();
}
@Override
public void setMarker(Drawable arg0) {
super.setMarker(arg0);
}
}
private void showPopupOverlay(BDLocation location) {
TextView popText = ((TextView) viewCache
.findViewById(R.id.location_tips));
popText.setText(location.getAddrStr());
mPopupOverlay.showPopup(getBitmapFromView(popText),
new GeoPoint((int) (location.getLatitude() * 1e6),
(int) (location.getLongitude() * 1e6)), 10);
}
/**
* 手动请求定位的方法
*/
public void requestLocation() {
isRequest = true;
if (mLocClient != null && mLocClient.isStarted()) {
showToast("火速为您定位!");
mLocClient.requestLocation();
} else {
Log.d("LocSDK3", "locClient is null or not started");
}
/**
* 定位功能是异步的。 加上这个服务就行了 <service android:name="com.baidu.location.f"
* android:enabled="true" android:process=":remote"> </service>
*/
}
@Override
protected void onResume() {
// MapView的生命周期与Activity同步,当activity挂起时需调用MapView.onPause()
mMapView.onResume();
super.onResume();
}
@Override
protected void onPause() {
// MapView的生命周期与Activity同步,当activity挂起时需调用MapView.onPause()
mMapView.onPause();
super.onPause();
}
@Override
protected void onDestroy() {
// MapView的生命周期与Activity同步,当activity销毁时需调用MapView.destroy()
mMapView.destroy();
// 退出应用调用BMapManager的destroy()方法
if (mBMapManager != null) {
mBMapManager.destroy();
mBMapManager = null;
}
// 退出时销毁定位
if (mLocClient != null) {
mLocClient.stop();
}
super.onDestroy();
}
/**
* 显示Toast消息
*
* @param msg
*/
private void showToast(String msg) {
if (mToast == null) {
mToast = Toast.makeText(this, msg, Toast.LENGTH_SHORT);
} else {
mToast.setText(msg);
mToast.setDuration(Toast.LENGTH_SHORT);
}
mToast.show();
}
/**
*
* @param view
* @return
*/
public static Bitmap getBitmapFromView(View view) {
view.measure(MeasureSpec.makeMeasureSpec(0, MeasureSpec.UNSPECIFIED),
MeasureSpec.makeMeasureSpec(0, MeasureSpec.UNSPECIFIED));
view.layout(0, 0, view.getMeasuredWidth(), view.getMeasuredHeight());
view.buildDrawingCache();
Bitmap bitmap = view.getDrawingCache();
return bitmap;
}
@Override
public void onClick(View v) {
switch (v.getId()) {
case R.id.plan_driver:
SearchButtonProcess(v);
break;
case R.id.plan_bus:
SearchButtonProcess(v);
break;
case R.id.plan_walk:
SearchButtonProcess(v);
break;
}
}
/**
* 路线规划
* @param v
*/
private void SearchButtonProcess(View v) {
mkRoute = null;
transitOverlay = null;
routeOverlay = null;
//-------------------
if(location == null){
System.out.println("location is null,so can't plan route !");
return;
}
MKPlanNode startNode = new MKPlanNode();
startNode.pt = new GeoPoint((int) (location.getLatitude() * 1E6),(int) (location.getLongitude() * 1E6));
MKPlanNode endNode = new MKPlanNode();
endNode.pt = new GeoPoint((int) (Double.valueOf(gpsY) * 1E6),(int) (Double.valueOf(gpsX) * 1E6));
if (plan_drive.equals(v)) {
System.out.println("begin drive");
mkSearch.drivingSearch("北京", startNode, "北京", endNode);
} else if (plan_bus.equals(v)) {
System.out.println("begin bus");
mkSearch.transitSearch("北京", startNode, endNode);
} else if (plan_walk.equals(v)) {
System.out.println("begin walk");
mkSearch.walkingSearch("北京", startNode, "北京", endNode);
}
}
}
5.添加jar包 百度文档上下载
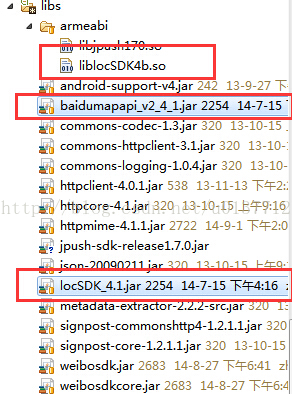