插入排序 O(n^2)
static void insertion_sort(int[] unsorted) { for (int i = 1; i < unsorted.Length; i++) { if (unsorted[i - 1] > unsorted[i]) { int temp = unsorted[i]; int j = i; while (j > 0 && unsorted[j - 1] > temp) { unsorted[j] = unsorted[j - 1]; j--; } unsorted[j] = temp; } } }起泡排序 O(n^2)
static void bubble_sort(int[] unsorted) { for (int i = 0; i < unsorted.Length; i++) { for (int j = i; j < unsorted.Length; j++) { if (unsorted[i] > unsorted[j]) { int temp = unsorted[i]; unsorted[i] = unsorted[j]; unsorted[j] = temp; } } } }选择排序 O(n^2)
static void selection_sort(int[] unsorted) { for (int i = 0; i < unsorted.Length; i++) { int min = unsorted[i], min_index = i; for (int j = i; j < unsorted.Length; j++) { if (unsorted[j] < min) { min = unsorted[j]; min_index = j; } } if (min_index != i) { int temp = unsorted[i]; unsorted[i] = unsorted[min_index]; unsorted[min_index] = temp; } } }希尔排序:O(log2(n))
static void shell_sort(int[] unsorted, int len) { int group, i, j, temp; for (group = len / 2; group > 0; group /= 2) { for (i = group; i < len; i++) { for (j = i - group; j >= 0; j -= group) { if (unsorted[j] > unsorted[j + group]) { temp = unsorted[j]; unsorted[j] = unsorted[j + group]; unsorted[j + group] = temp; } } } } }
快速排序:O(log2(n))
static int partition(int[] unsorted, int low, int high) { int pivot = unsorted[low]; while (low < high) { while (low < high && unsorted[high] > pivot) high--; unsorted[low] = unsorted[high]; while (low < high && unsorted[low] <= pivot) low++; unsorted[high] = unsorted[low]; } unsorted[low] = pivot; return low; } static void quick_sort(int[] unsorted, int low, int high) { int loc = 0; if (low < high) { loc = partition(unsorted, low, high); quick_sort(unsorted, low, loc - 1); quick_sort(unsorted, loc + 1, high); } }
堆排序:O(log2(n))
//array是待调整的堆数组,i是待调整的数组元素的位置,nlength是数组的长度
//本函数功能是:根据数组array构建大根堆
void
HeapAdjust(
int
array[],
int
i,
int
nLength)
{
int
nChild;
int
nTemp;
for
(;2*i+1<nLength;i=nChild)
{
//子结点的位置=2*(父结点位置)+1
nChild=2*i+1;
//得到子结点中较大的结点
if
(nChild<nLength-1&&array[nChild+1]>array[nChild])++nChild;
//如果较大的子结点大于父结点那么把较大的子结点往上移动,替换它的父结点
if
(array[i]<array[nChild])
{
nTemp=array[i];
array[i]=array[nChild];
array[nChild]=nTemp;
}
else
break
;
//否则退出循环
}
}
//堆排序算法
void
HeapSort(
int
array[],
int
length)
{
int
i;
//调整序列的前半部分元素,调整完之后第一个元素是序列的最大的元素
//length/2-1是最后一个非叶节点,此处"/"为整除
for
(i=length/2-1;i>=0;--i)
HeapAdjust(array,i,length);
//从最后一个元素开始对序列进行调整,不断的缩小调整的范围直到第一个元素
for
(i=length-1;i>0;--i)
{
//把第一个元素和当前的最后一个元素交换,
//保证当前的最后一个位置的元素都是在现在的这个序列之中最大的
array[i]=array[0]^array[i];
array[0]=array[0]^array[i];
array[i]=array[0]^array[i];
//不断缩小调整heap的范围,每一次调整完毕保证第一个元素是当前序列的最大值
HeapAdjust(array,0,i);
}
}
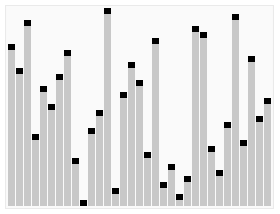
static void merge(int[] unsorted, int first, int mid, int last, int[] sorted) { int i = first, j = mid; int k = 0; while (i < mid && j < last) if (unsorted[i] < unsorted[j]) sorted[k++] = unsorted[i++]; else sorted[k++] = unsorted[j++]; while (i < mid) sorted[k++] = unsorted[i++]; while (j < last) sorted[k++] = unsorted[j++]; for (int v = 0; v < k; v++) unsorted[first + v] = sorted[v]; } static void merge_sort(int[] unsorted, int first, int last, int[] sorted) { if (first + 1 < last) { int mid = (first + last) / 2; Console.WriteLine("{0}-{1}-{2}", first, mid, last); merge_sort(unsorted, first, mid, sorted); merge_sort(unsorted, mid, last, sorted); merge(unsorted, first, mid, last, sorted); } }基数排序:
/// <summary> /// 基数排序 /// 约定:待排数字中没有0,如果某桶内数字为0则表示该桶未被使用,输出时跳过即可 /// </summary> /// <param name="unsorted">待排数组</param> /// <param name="array_x">桶数组第一维长度</param> /// <param name="array_y">桶数组第二维长度</param> static void radix_sort(int[] unsorted, int array_x = 10, int array_y = 100) { for (int i = 0; i < array_x/* 最大数字不超过999999999...(array_x个9) */; i++) { int[,] bucket = new int[array_x, array_y]; foreach (var item in unsorted) { int temp = (item / (int)Math.Pow(10, i)) % 10; for (int l = 0; l < array_y; l++) { if (bucket[temp, l] == 0) { bucket[temp, l] = item; break; } } } for (int o = 0, x = 0; x < array_x; x++) { for (int y = 0; y < array_y; y++) { if (bucket[x, y] == 0) continue; unsorted[o++] = bucket[x, y]; } } } }
引用参考博客:http://www.cnblogs.com/kkun/archive/2011/11/23/2260312.html
有个网址有动态图片:http://www.guqiankun.com/sortalgorithm(有意思)