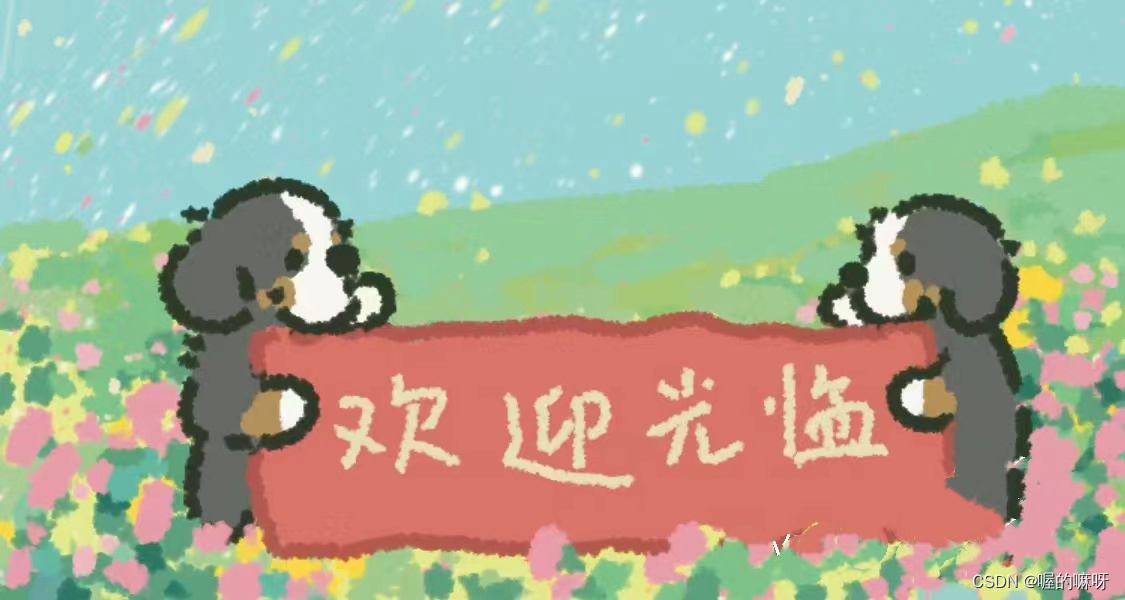
✨✨谢谢大家捧场,祝屏幕前的小伙伴们每天都有好运相伴左右,一定要天天开心哦!✨✨
🎈🎈作者主页: 喔的嘛呀🎈🎈
目录
引言
处理安全性和权限管理是Java项目中至关重要的一部分,它涉及到保护系统免受恶意攻击和非法访问。在这篇博客中,我们将介绍一些常用的安全措施和实践,帮助开发人员提高Java项目的安全性和稳定性。
一. 身份验证和授权
身份验证和授权是保护Java项目安全的重要组成部分。在Java项目中,通常使用Spring Security来实现身份验证和授权功能。下面是一个基本的示例,演示了如何在Spring Boot项目中使用Spring Security进行身份验证和授权。
首先,确保在pom.xml
中包含Spring Security的依赖:
<dependency>
<groupId>org.springframework.boot</groupId>
<artifactId>spring-boot-starter-security</artifactId>
</dependency>
然后,创建一个SecurityConfig
类来配置Spring Security:
import org.springframework.context.annotation.Bean;
import org.springframework.context.annotation.Configuration;
import org.springframework.security.config.annotation.authentication.builders.AuthenticationManagerBuilder;
import org.springframework.security.config.annotation.web.builders.HttpSecurity;
import org.springframework.security.config.annotation.web.configuration.EnableWebSecurity;
import org.springframework.security.config.annotation.web.configuration.WebSecurityConfigurerAdapter;
import org.springframework.security.crypto.bcrypt.BCryptPasswordEncoder;
import org.springframework.security.crypto.password.PasswordEncoder;
@Configuration
@EnableWebSecurity
public class SecurityConfig extends WebSecurityConfigurerAdapter {
@Override
protected void configure(HttpSecurity http) throws Exception {
http.authorizeRequests()
.antMatchers("/admin/**").hasRole("ADMIN")
.antMatchers("/user/**").hasRole("USER")
.anyRequest().authenticated()
.and()
.formLogin()
.and()
.httpBasic();
}
@Override
protected void configure(AuthenticationManagerBuilder auth) throws Exception {
auth.inMemoryAuthentication()
.withUser("admin").password(passwordEncoder().encode("admin")).roles("ADMIN")
.and()
.withUser("user").password(passwordEncoder().encode("user")).roles("USER");
}
@Bean
public PasswordEncoder passwordEncoder() {
return new BCryptPasswordEncoder();
}
}
在上面的示例中,SecurityConfig
类配置了两个用户:一个管理员(admin)和一个普通用户(user),并定义了访问权限规则。管理员可以访问/admin/**
路径,而普通用户可以访问/user/**
路径。所有其他路径都需要身份验证。
在Spring Security中,密码需要进行加密存储。我们使用BCryptPasswordEncoder
来加密密码,并在configure
方法中将加密后的密码存储到内存中。
最后,在application.properties
文件中关闭默认的安全配置,以便我们可以自定义安全配置:
spring.security.enabled=false
这样,我们就完成了基本的Spring Security配置。在实际项目中,你可以根据需要进行更复杂的配置,如使用数据库存储用户信息、配置HTTPS等。
二. 输入验证和过滤
在Java项目中进行输入验证和过滤是确保系统安全的重要步骤。本文将介绍如何使用OWASP ESAPI(Enterprise Security API)来实现输入验证和过滤,以防止常见的安全漏洞,如SQL注入和跨站脚本攻击(XSS)。
2.1. 添加OWASP ESAPI依赖
首先,在项目的pom.xml
文件中添加OWASP ESAP
# ESAPI Configuration
ESAPI.Encoder.AllowMultipleEncoding=false
ESAPI.Encoder.DefaultCodecList=HTMLEntityCodec,PercentCodec,JavaScriptCodec
ESAPI.Logger=org.owasp.esapi.reference.Log4JLogFactory
ESAPI.Logger.LogApplicationName=myApplication
ESAPI.Logger.ApplicationName=myApplication
I依赖:
<dependency>
<groupId>org.owasp.esapi</groupId>
<artifactId>esapi</artifactId>
<version>2.2.0.0</version>
</dependency>
2.2. 配置ESAPI
在项目中创建一个ESAPI.properties
文件,用于配置ESAPI的基本设置:
# ESAPI Configuration
ESAPI.Encoder.AllowMultipleEncoding=false
ESAPI.Encoder.DefaultCodecList=HTMLEntityCodec,PercentCodec,JavaScriptCodec
ESAPI.Logger=org.owasp.esapi.reference.Log4JLogFactory
ESAPI.Logger.LogApplicationName=myApplication
ESAPI.Logger.ApplicationName=myApplication
2.3. 使用ESAPI进行输入验证和过滤
在Java代码中使用ESAPI提供的工具类进行输入验证和过滤。例如,对用户输入进行HTML编码和过滤:
import org.owasp.esapi.ESAPI;
import org.owasp.esapi.errors.ValidationException;
import org.owasp.esapi.filters.SafeRequest;
public class InputValidationFilter {
public static String sanitizeInput(String userInput) {
try {
SafeRequest safeRequest = new SafeRequest();
safeRequest.put("userInput", userInput);
return safeRequest.getString("userInput");
} catch (ValidationException e) {
// 处理验证异常
e.printStackTrace();
return null;
}
}
public static void main(String[] args) {
String userInput = "<script>alert('XSS')</script>";
String sanitizedInput = sanitizeInput(userInput);
System.out.println("Sanitized Input: " + sanitizedInput);
}
}
在上面的示例中,我们使用SafeRequest
类的getString
方法来过滤和验证用户输入,并防止XSS攻击。通过使用OWASP ESAPI,可以简化输入验证和过滤的过程,并提高系统的安全性。
请注意,除了使用ESAPI进行输入验证和过滤外,还应该注意其他安全最佳实践,如使用预编译语句防止SQL注入、限制用户输入长度等。
三. 数据加密
对于敏感数据的加密处理,我们可以使用Java中的javax.crypto
包提供的AES(高级加密标准)算法进行加密存储。下面是一个简单的示例,演示了如何使用AES算法对用户密码进行加密存储:
import javax.crypto.Cipher;
import javax.crypto.SecretKey;
import javax.crypto.SecretKeyFactory;
import javax.crypto.spec.IvParameterSpec;
import javax.crypto.spec.PBEKeySpec;
import javax.crypto.spec.SecretKeySpec;
import java.security.spec.KeySpec;
import java.util.Base64;
public class AESUtil {
private static final String SECRET_KEY = "your_secret_key";
private static final String SALT = "your_salt";
private static final String INIT_VECTOR = "your_init_vector";
public static String encrypt(String value) {
try {
IvParameterSpec iv = new IvParameterSpec(INIT_VECTOR.getBytes("UTF-8"));
SecretKeySpec skeySpec = new SecretKeySpec(SECRET_KEY.getBytes("UTF-8"), "AES");
Cipher cipher = Cipher.getInstance("AES/CBC/PKCS5PADDING");
cipher.init(Cipher.ENCRYPT_MODE, skeySpec, iv);
byte[] encrypted = cipher.doFinal(value.getBytes());
return Base64.getEncoder().encodeToString(encrypted);
} catch (Exception ex) {
ex.printStackTrace();
}
return null;
}
public static String decrypt(String encrypted) {
try {
IvParameterSpec iv = new IvParameterSpec(INIT_VECTOR.getBytes("UTF-8"));
SecretKeySpec skeySpec = new SecretKeySpec(SECRET_KEY.getBytes("UTF-8"), "AES");
Cipher cipher = Cipher.getInstance("AES/CBC/PKCS5PADDING");
cipher.init(Cipher.DECRYPT_MODE, skeySpec, iv);
byte[] original = cipher.doFinal(Base64.getDecoder().decode(encrypted));
return new String(original);
} catch (Exception ex) {
ex.printStackTrace();
}
return null;
}
public static void main(String[] args) {
String password = "my_secret_password";
String encryptedPassword = AESUtil.encrypt(password);
System.out.println("Encrypted password: " + encryptedPassword);
String decryptedPassword = AESUtil.decrypt(encryptedPassword);
System.out.println("Decrypted password: " + decryptedPassword);
}
}
在上面的示例中,encrypt
方法用于对密码进行加密,decrypt
方法用于解密加密后的密码。请注意,为了安全起见,SECRET_KEY、SALT和INIT_VECTOR应该根据实际情况进行随机生成,并且不应该硬编码在代码中。
四.防止会话劫持
防止会话劫持是保护Web应用程序安全的重要措施。除了使用HTTPS来加密数据传输和定期更换会话ID外,还可以采取其他措施来增强安全性。下面是一个简单的示例,演示了如何在Java Web应用中使用Spring Security来防止会话劫持:
首先,确保在pom.xml
中包含Spring Security的依赖:
<dependency>
<groupId>org.springframework.boot</groupId>
<artifactId>spring-boot-starter-security</artifactId>
</dependency>
然后,创建一个SecurityConfig
类来配置Spring Security:
import org.springframework.context.annotation.Bean;
import org.springframework.context.annotation.Configuration;
import org.springframework.security.config.annotation.web.builders.HttpSecurity;
import org.springframework.security.config.annotation.web.configuration.EnableWebSecurity;
import org.springframework.security.config.annotation.web.configuration.WebSecurityConfigurerAdapter;
@Configuration
@EnableWebSecurity
public class SecurityConfig extends WebSecurityConfigurerAdapter {
@Override
protected void configure(HttpSecurity http) throws Exception {
http
.sessionManagement()
.sessionFixation().newSession() // 每次认证时创建新的会话
.sessionAuthenticationErrorUrl("/login?error=session") // 会话认证错误跳转页面
.maximumSessions(1) // 最大会话数为1
.maxSessionsPreventsLogin(false); // 不阻止新的会话登录
}
}
在上面的示例中,SecurityConfig
类配置了会话管理策略,包括每次认证时创建新的会话、设置会话认证错误时的跳转页面、限制最大会话数为1以及不阻止新的会话登录。
接下来,在Spring Boot应用的application.properties
文件中启用HTTPS:
server.port=8443
server.ssl.key-store=classpath:keystore.jks
server.ssl.key-store-password=your_password
server.ssl.key-password=your_password
确保将keystore.jks
文件替换为你自己的SSL证书文件,并设置正确的密码。
通过这些步骤,你可以在Java Web应用中使用Spring Security来防止会话劫持。同时,建议定期审查和更新应用程序的安全措施,以应对新的安全威胁。
五. 安全日志和监控
安全日志和监控是确保Java项目安全的重要组成部分。下面是一个简单的示例,演示了如何在Java项目中使用Log4j记录安全日志,并使用Zabbix监控系统安全状况:
首先,确保在pom.xml
中包含Log4j和Zabbix Java客户端的依赖:
<dependency>
<groupId>org.apache.logging.log4j</groupId>
<artifactId>log4j-api</artifactId>
<version>2.14.1</version>
</dependency>
<dependency>
<groupId>org.apache.logging.log4j</groupId>
<artifactId>log4j-core</artifactId>
<version>2.14.1</version>
</dependency>
<dependency>
<groupId>io.github.mikaelmello</groupId>
<artifactId>zabbix-sender</artifactId>
<version>2.0.0</version>
</dependency>
然后,配置Log4j2的日志文件和Zabbix监控:
在src/main/resources
目录下创建log4j2.xml
文件,用于配置Log4j2:
<?xml version="1.0" encoding="UTF-8"?>
<Configuration>
<Appenders>
<Console name="Console" target="SYSTEM_OUT">
<PatternLayout pattern="%d{HH:mm:ss.SSS} [%t] %-5level %logger{36} - %msg%n"/>
</Console>
<File name="SecurityFile" fileName="security.log" append="true">
<PatternLayout pattern="%d{HH:mm:ss.SSS} [%t] %-5level %logger{36} - %msg%n"/>
</File>
</Appenders>
<Loggers>
<Root level="info">
<AppenderRef ref="Console"/>
<AppenderRef ref="SecurityFile"/>
</Root>
</Loggers>
</Configuration>
在上面的示例中,配置了一个控制台和一个文件Appender,分别用于输出日志到控制台和文件中。安全日志将记录在security.log
文件中。
接下来,创建一个类来处理安全事件,并使用Log4j记录日志:
import org.apache.logging.log4j.LogManager;
import org.apache.logging.log4j.Logger;
public class SecurityLogger {
private static final Logger logger = LogManager.getLogger(SecurityLogger.class);
public static void logSecurityEvent(String event) {
logger.info(event);
// 向Zabbix发送监控信息
ZabbixSender sender = new ZabbixSender("zabbix_server_hostname", 10051);
sender.send(new DataObject("JavaApp.security.event", event));
sender.close();
}
}
在上面的示例中,SecurityLogger
类使用Log4j记录安全事件,并通过ZabbixSender发送监控信息到Zabbix服务器。你需要将zabbix_server_hostname
替换为你的Zabbix服务器主机名。
通过以上步骤,你可以在Java项目中使用Log4j记录安全日志,并使用Zabbix监控系统安全状况。这些措施有助于及时发现和处理安全事件,提高系统的安全性。
总结
在Java项目中处理安全性和权限管理是至关重要的,开发人员应该时刻关注系统的安全性,并采取适当的措施保护系统不受攻击。通过使用合适的身份验证和授权机制、严格的输入验证和过滤、数据加密、防止会话劫持以及安全日志和监控等措施,可以大大提高Java项目的安全性和稳定性。