public class MyTest {
public static void main(String[] args) {
JFrame jFrame = new JFrame("中北大学");
//jFrame.setSize(500,500);
Dimension dimension = new Dimension(500,500);
//jFrame.setLocation(500,400);
jFrame.setSize(dimension);
Point point = new Point();
jFrame.setLocation(500,400);
jFrame.setBackground(Color.cyan);
jFrame.setVisible(true);
}
}
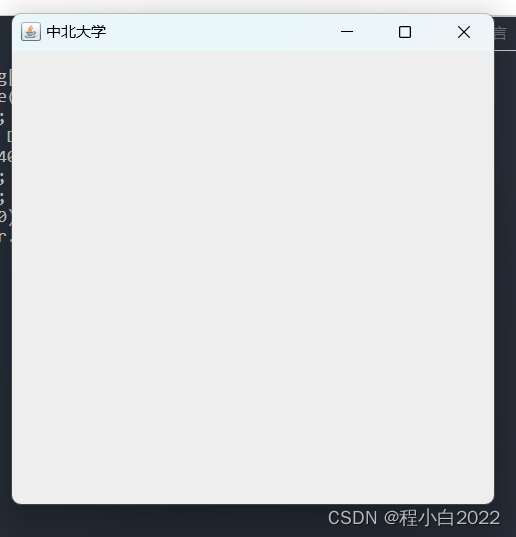
public class MyTest02 {
public static void main(String[] args) {
JFrame jFrame = new JFrame("中北大学");
Dimension dimension = new Dimension(500,500);
Point point = new Point();
jFrame.setLocation(point);
jFrame.setBackground(Color.cyan);
jFrame.setVisible(true);
}
}
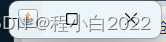
public class MyTest03 {
public static void main(String[] args) {
JFrame jFrame = new JFrame("中北大学");
JLabel jLabel = new JLabel("用户名",JLabel.CENTER);
jFrame.add(jLabel);
jLabel.setFont(new Font("Serief",Font.BOLD,60));
jLabel.setForeground(Color.pink);
jFrame.setSize(500,500);
jFrame.setLocation(500,400);
jFrame.setBackground(Color.BLACK);
jFrame.setVisible(true);
}
}
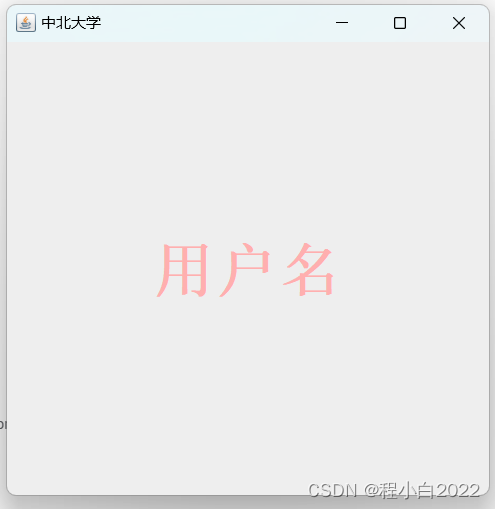
public class MyTest04 {
public static void main(String[] args) {
JFrame jFrame = new JFrame("中北大学");
jFrame.setLayout(null);
JButton jButton = new JButton("点击我");
jButton.setBounds(100,100,100,100);
jButton.setBackground(Color.pink);
jFrame.add(jButton);
jFrame.setSize(500,500);
jFrame.setLocation(500,400);
jFrame.setBackground(Color.BLACK);
jFrame.setVisible(true);
}
}
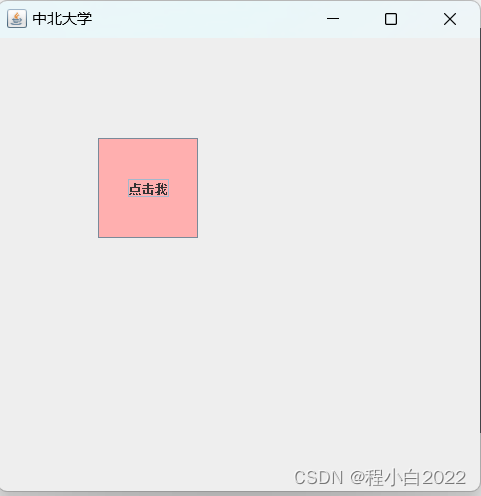
public class MyTest05 {
public static void main(String[] args) {
JFrame jFrame = new JFrame("中北大学");
jFrame.setLayout(new FlowLayout(FlowLayout.LEFT,3,3));
JButton but = null;
for (int i = 0;i < 15;i++){
but = new JButton("按钮" + i);
jFrame.add(but);
}
jFrame.setSize(500,500);
jFrame.setLocation(500,400);
jFrame.setBackground(Color.BLACK);
jFrame.setVisible(true);
}
}
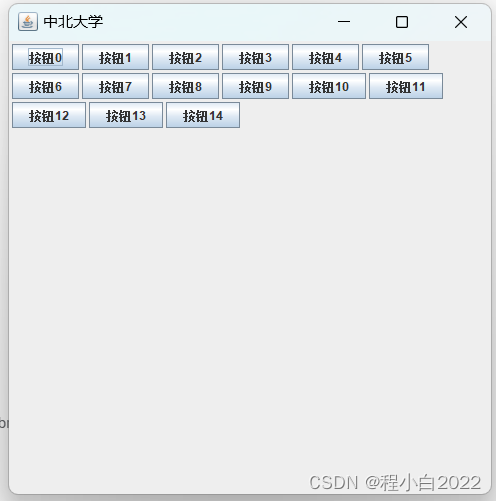
public class MyTest06 {
public static void main(String[] args) {
JFrame jFrame = new JFrame("中北大学");
jFrame.setLayout(null);
jFrame.setSize(500,500);
jFrame.setLocation(500,400);
jFrame.setBackground(Color.BLACK);
JButton jButton = new JButton("点击我");
jButton.setBounds(200,150,100,100);
jButton.setBackground(Color.pink);
jFrame.add(jButton);
jFrame.setVisible(true);
}
}
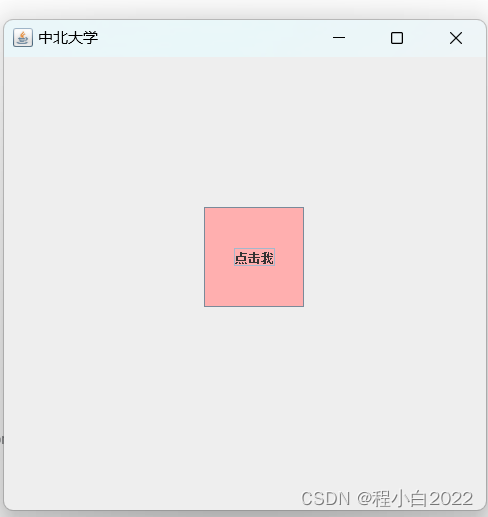
public class ActionListenerTest {
public static void main(String[] args) {
//创建窗体对象
JFrame jFrame = new JFrame("中北大学");
jFrame.setLocation(600,400);
jFrame.setSize(400,200);
//定义按钮组件
JButton jButton = new JButton("显示");
//定义文本显示组件
JLabel jLabel = new JLabel();
//定义单行文本输入框
JTextField jTextField = new JTextField(10);
//定义JPanel容器
JPanel jPanel = new JPanel();
//定义字体对象
Font font = new Font("Serief",Font.BOLD,20);
//给文本显示组件设置字体
jLabel.setFont(font);
//给文本显示组件设置默认文字
jLabel.setText("等待用户操作");
//给按钮设置监听动作事件
jButton.addActionListener(new ActionListener() {
@Override
public void actionPerformed(ActionEvent e) {
//获取文本输入框的内容
String result = jTextField.getText();
System.out.println("文本输入框的内容为" + result);
//将文本输入框输入的内容赋值给文本显示组件
jLabel.setText(result);
}
});
//将定义好的三个组件放入容器
jPanel.add(jLabel);
jPanel.add(jTextField);
jPanel.add(jButton);
jFrame.add(jPanel);
jFrame.setVisible(true);
}
}
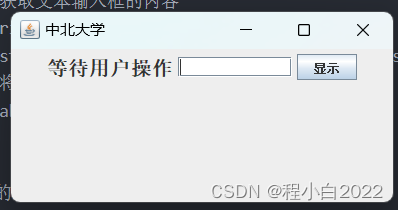
public class JCheckBoxTest {
public static void main(String[] args) {
//创建窗体对象
JFrame jFrame = new JFrame("中北大学");
jFrame.setLocation(600,400);
jFrame.setSize(400,200);
JCheckBox jb1 = new JCheckBox("打篮球");
JCheckBox jb2 = new JCheckBox("踢足球");
JCheckBox jb3 = new JCheckBox("打台球");
jb1.addItemListener(new ItemListener() {
@Override
public void itemStateChanged(ItemEvent e) {
System.out.println("jb1的状态" + e.getStateChange());
}
});
jb1.addItemListener(new ItemListener() {
@Override
public void itemStateChanged(ItemEvent e) {
System.out.println("jb1的状态" + e.getStateChange());
}
});
jb1.addItemListener(new ItemListener() {
@Override
public void itemStateChanged(ItemEvent e) {
System.out.println("jb1的状态" + e.getStateChange());
}
});
JPanel jPanel = new JPanel();
jPanel.add(jb1);
jPanel.add(jb2);
jPanel.add(jb3);
jFrame.add(jPanel);
jFrame.setVisible(true);
}
}
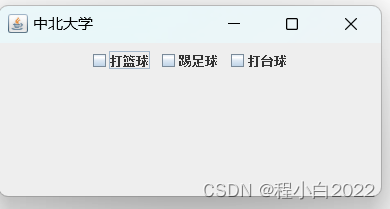
public class JMenuBarTest {
public static void main(String[] args) {
//创建窗体对象
JFrame jFrame = new JFrame("中北大学");
jFrame.setLocation(600,400);
jFrame.setSize(400,200);
JMenu jMenu1= new JMenu("文件");
JMenuItem item1 = new JMenuItem("新建");
JMenuItem item2 = new JMenuItem("打开");
jMenu1.add(item1);
jMenu1.add(item2);
JMenu jMenu2= new JMenu("帮助");
item1.addActionListener(new ActionListener() {
@Override
public void actionPerformed(ActionEvent e) {
System.out.println("菜单项:" + e.getActionCommand());
}
});
JMenu jMenu3= new JMenu("设置");
JMenuItem item3 = new JMenuItem("页面设置");
JMenuItem item4 = new JMenuItem("系统设置");
jMenu3.add(item3);
jMenu3.add(item4);
item4.addActionListener(new ActionListener() {
@Override
public void actionPerformed(ActionEvent e) {
System.out.println("设置:" + e.getActionCommand());
}
});
JMenuBar jMenuBar = new JMenuBar();
jMenuBar.add(jMenu1);
jMenuBar.add(jMenu2);
jMenuBar.add(jMenu3);
jFrame.setJMenuBar(jMenuBar);
jFrame.setVisible(true);
}
}
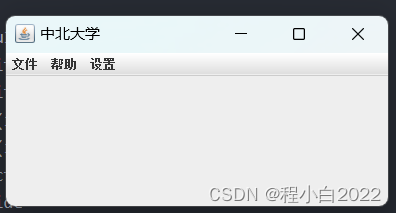
public class JPanelTest {
public static void main(String[] args) {
JFrame jFrame = new JFrame("中北大学");
JPanel jPanel = new JPanel();
jPanel.add(new JLabel("标签A"));
jPanel.add(new JLabel("标签B"));
jPanel.add(new JLabel("标签C"));
jPanel.add(new JButton("按钮 X"));
jPanel.add(new JButton("按钮 Y"));
jPanel.add(new JButton("按钮 Z"));
jFrame.add(jPanel);
jFrame.pack();
jFrame.setSize(600,400);
jFrame.setLocation(500,400);
jFrame.setVisible(true);
}
}
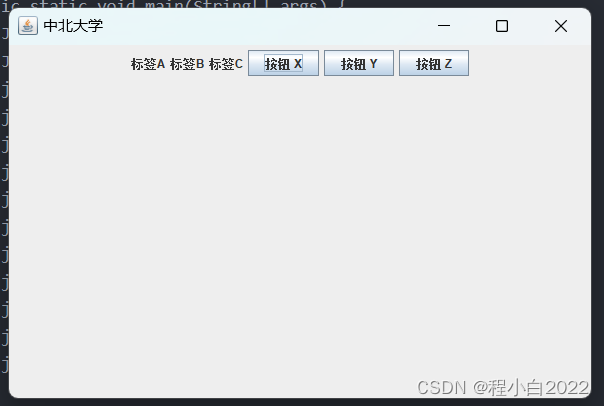
public class JRadioButtonTest {
public static void main(String[] args) {
//创建窗体对象
JFrame jFrame = new JFrame("中北大学");
jFrame.setLocation(600,400);
jFrame.setSize(400,200);
JRadioButton jb1 = new JRadioButton("男");
JRadioButton jb2 = new JRadioButton("女");
jb1.addItemListener(new ItemListener() {
@Override
public void itemStateChanged(ItemEvent e) {
System.out.println("jb1的状态" + e.getStateChange());
}
});
jb2.addItemListener(new ItemListener() {
@Override
public void itemStateChanged(ItemEvent e) {
System.out.println("jb1的状态" + e.getStateChange());
}
});
JPanel jPanel = new JPanel();
jPanel.add(jb1);
jPanel.add(jb2);
jFrame.add(jPanel);
jFrame.setVisible(true);
}
}
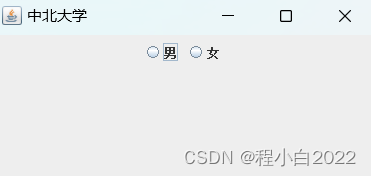
public class JTextCompoentTest {
public static void main(String[] args) {
JFrame jFrame = new JFrame("中北大学");
jFrame.setLayout(null);
JTextField jTextField = new JTextField();
//JPasswordField jPasswordField = new JPasswordField();
//JTextArea jTextArea = new JTextArea();
jTextField.setBounds(100,150,400,80);
jFrame.add(jTextField);//jPasswordField,jTextArea
jFrame.setLocation(400,500);
jFrame.setSize(600,400);
jFrame.setVisible(true);
}
}
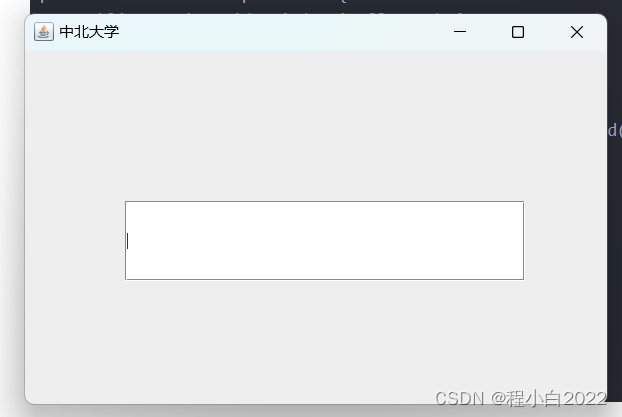
public class KeyListenerTest {
public static void main(String[] args) {
//创建窗体对象
JFrame jFrame = new JFrame("中北大学");
jFrame.setLocation(600,400);
jFrame.setSize(400,200);
JTextArea text = new JTextArea();
text.addKeyListener(new KeyListener() {
@Override
public void keyTyped(KeyEvent e) {
text.append("输入的内容是:" + e.getKeyChar() + "\n");
}
@Override
public void keyPressed(KeyEvent e) {
text.append("键盘" + KeyEvent.getKeyText(e.getKeyCode()) + "按下了/!");
}
@Override
public void keyReleased(KeyEvent e) {
text.append("键盘" + KeyEvent.getKeyText(e.getKeyCode()) + "键松开了!");
}
});
JScrollPane scr = new JScrollPane(text);
scr.setBounds(5,5,300,200);
jFrame.add(scr);
jFrame.setVisible(true);
}
}
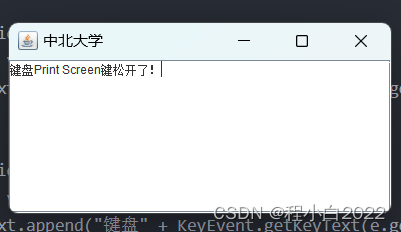
public class MouseListenerTest {
public static void main(String[] args) {
//创建窗体对象
JFrame jFrame = new JFrame("中北大学");
jFrame.setLocation(600,400);
jFrame.setSize(400,200);
JTextArea text = new JTextArea();
text.addMouseListener(new MouseListener() {
@Override
public void mouseClicked(MouseEvent e) {
text.append("鼠标单击" + e.getButton()+ "\n");
}
@Override
public void mousePressed(MouseEvent e) {
text.append("鼠标-->" + e.getButton() + "按下了" + "\n");
}
@Override
public void mouseReleased(MouseEvent e) {
text.append("鼠标-->" + e.getButton() + "松开了"+ "\n");
}
@Override
public void mouseEntered(MouseEvent e) {
text.append("鼠标进入组件"+ "\n");
}
@Override
public void mouseExited(MouseEvent e) {
text.append("鼠标离开组件"+ "\n");
}
});
JScrollPane scr = new JScrollPane(text);
scr.setBounds(5,5,300,200);
jFrame.add(scr);
jFrame.setVisible(true);
}
}
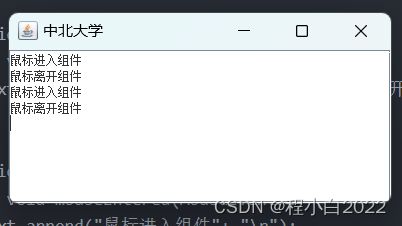
public class 作业 {
public static void main(String[] args) {
JFrame jFrame = new JFrame("登录");
jFrame.setBounds(100, 100, 300, 200);
jFrame.setDefaultCloseOperation(JFrame.EXIT_ON_CLOSE);
jFrame.getContentPane().setLayout(null);
JLabel usernameLabel = new JLabel("用户名:");
usernameLabel.setBounds(10, 20, 80, 25);
jFrame.getContentPane().add(usernameLabel);
JTextField jTextField = new JTextField();
jTextField.setBounds(100, 20, 165, 25);
jFrame.getContentPane().add(jTextField);
jTextField.setColumns(10);
JLabel passwordLabel = new JLabel("密码:");
passwordLabel.setBounds(10, 60, 80, 25);
jFrame.getContentPane().add(passwordLabel);
JPasswordField jPasswordField = new JPasswordField();
jPasswordField.setBounds(100, 60, 165, 25);
jFrame.getContentPane().add(jPasswordField);
JButton jButton = new JButton("登录");
jButton.addActionListener(new ActionListener() {
public void actionPerformed(ActionEvent e) {
String username = jTextField.getText();
char[] password = jPasswordField.getPassword();
// 在这里添加验证逻辑,比如检查用户名和密码是否匹配预设的值。
System.out.println("用户名: " + username);
System.out.println("密码: " + new String(password));
}
});
jButton.setBounds(100, 100, 80, 25);
jFrame.getContentPane().add(jButton);
jFrame.setVisible(true);
}
}
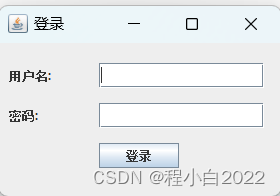