一、前言
实现功能:
这段代码实现了一个简单的电影票选座购买的功能界面。
-
在页面上展示了一个电影院的座位布局,以及右侧显示了电影信息、选座情况、票价、总计等内容。
-
用户可以通过点击座位来选择购买电影票,每个座位的状态会在点击时改变(从默认颜色变为红色),并在右侧显示已选座位的信息。
-
用户可以确认购买,确认购买后会出现购买成功的提示,并且页面会重置,清空已选座位和右侧信息。
实现逻辑:
-
首先,在 HTML 中定义了一个包含座位和右侧信息的页面结构。
-
使用 JavaScript 获取到需要操作的元素,包括座位列表、座位元素、默认座位颜色、选座数目、选座信息展示区域等。
-
使用循环创建了 64 个座位,并给每个座位添加了行列信息。同时初始化了默认座位颜色和选座数目变量。
-
定义了一个函数
addSeatClick
,用于处理座位的点击事件。当座位被点击时,根据当前座位状态(默认或已选),改变座位颜色、更新选座数目、显示已选座位信息以及计算总金额等操作。 -
遍历所有座位元素,为每个座位添加点击事件处理函数。
-
监听确认购买按钮的点击事件。点击确认购买后,弹出购买成功提示,重置所有座位颜色,清空已选座位信息和总金额,并将选座数目重置为零。
二、项目运行效果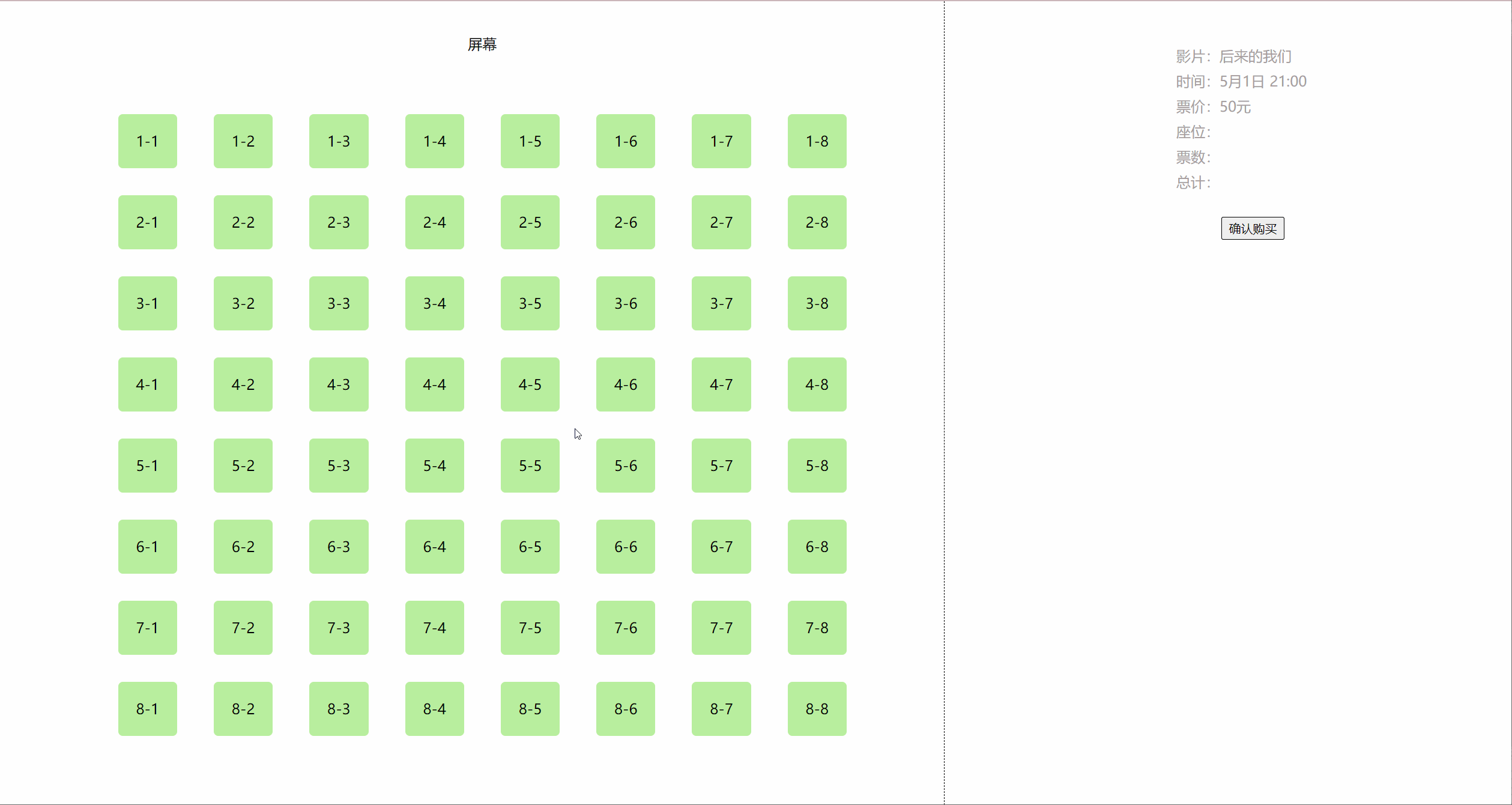
三、全部代码
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<meta name="viewport" content="width=device-width, initial-scale=1.0">
<title>购买电影票</title>
<style>
* {
margin: 0;
padding: 0;
text-decoration: none;
list-style: none;
background-repeat: no-repeat;
}
.con-box {
width: 100%;
height: 100vh;
display: flex;
justify-content: center;
gap: 10px;
margin: 0 auto;
}
.left {
width: 60%;
height: 100vh;
border-right: 1px dashed #000;
}
.left p {
width: 70%;
height: 35px;
line-height: 35px;
text-align: center;
margin: 30px auto;
background-color: #f0f0f0;
border-radius: 5px;
}
.seat {
display: flex;
width: 80%;
height: 750px;
flex-wrap: wrap;
gap: 30px;
justify-content: space-around;
align-content: center;
margin: 0 auto;
}
.seat li {
width: 8%;
height: 8%;
border-radius: 5px;
background-color: #b9ef9f;
line-height: 60px;
text-align: center;
cursor: pointer;
}
.right {
width: 35%;
height: 100vh;
color: #a79e9f;
position: relative;
}
.right-con {
width: 350px;
height: 90vh;
position: absolute;
right: 0;
top: 5%;
line-height: 28px;
}
#seatNumbers {
width: 240px;
}
.seat-number {
display: inline-block;
width: 100px;
height: 30px;
background-color: #efefef;
border-radius: 5px;
text-align: center;
margin-left: 10px;
margin-bottom: 10px;
line-height: 30px;
color: #000;
border: #d1d1d1 1px solid;
}
.right-con button {
width: 70px;
height: 25px;
margin: 25px 50px;
background-color: #efefef;
border: solid 0.5px #000;
border-radius: 2px;
cursor: pointer;
}
</style>
</head>
<body>
<div class="con-box">
<div class="left">
<p>屏幕</p>
<ul class="seat"></ul>
</div>
<div class="right">
<ul class="right-con">
<li>影片:<span>后来的我们</span></li>
<li>时间:<span>5月1日 21:00</span></li>
<li>票价:<span>50元</span></li>
<li>座位:<p id="seatNumbers"></p>
</li>
<li>票数:<span></span></li>
<li>总计:<span></span></li>
<button>确认购买</button>
</ul>
</div>
</div>
</body>
<script>
document.addEventListener('DOMContentLoaded', function () {
const seatList = document.querySelector('.seat');
for (let i = 0; i < 64; i++) {
const li = document.createElement('li');
seatList.appendChild(li);
}
const seats = document.querySelectorAll('.seat li');
const defaultColor = seats[0].style.backgroundColor;
let selectedSeats = [];
const seatNumbers = document.querySelector('#seatNumbers');
for (let i = 1; i <= 8; i++) {
for (let j = 1; j <= 8; j++) {
let seatIndex = (i - 1) * 8 + (j - 1);
seats[seatIndex].innerText = i + '-' + j;
}
}
function addSeatClick(seat) {
seat.addEventListener('click', () => {
if (seat.style.backgroundColor === defaultColor) {
seat.style.backgroundColor = 'red';
selectedSeats.push(seat.innerText);
let numberP = document.createElement('p');
numberP.classList.add('seat-number');
seatNumbers.appendChild(numberP);
let row = seat.innerText.split('-')[0];
let column = seat.innerText.split('-')[1];
numberP.innerText = `${row}排${column}座`;
document.querySelector('.right-con li:nth-child(5) span').innerText = selectedSeats.length;
let total = selectedSeats.length * 50;
document.querySelector('.right-con li:nth-child(6) span').innerText = '¥' + total;
} else {
seat.style.backgroundColor = defaultColor;
const seatIndex = selectedSeats.indexOf(seat.innerText);
if (seatIndex !== -1) {
selectedSeats.splice(seatIndex, 1);
}
seatNumbers.innerHTML = '';
selectedSeats.forEach(seat => {
let numberP = document.createElement('p');
numberP.classList.add('seat-number');
let seatInfo = document.createTextNode(`${seat.split('-')[0]}排${seat.split('-')[1]}座`);
numberP.appendChild(seatInfo);
seatNumbers.appendChild(numberP);
});
document.querySelector('.right-con li:nth-child(5) span').innerText = selectedSeats.length;
console.log('ss' + selectedSeats.length);
let total = selectedSeats.length * 50;
document.querySelector('.right-con li:nth-child(6) span').innerText = '¥' + total;
}
});
}
function but() {
const confirmButton = document.querySelector('button');
confirmButton.addEventListener('click', () => {
alert('购买成功!');
seats.forEach(seat => {
seat.style.backgroundColor = '#b9ef9f';
});
seatNumbers.innerHTML = '';
selectedSeats = [];
document.querySelector('.right-con li:nth-child(5) span').innerText = '';
document.querySelector('.right-con li:nth-child(6) span').innerText = '';
});
}
but()
seats.forEach(seat => {
addSeatClick(seat);
});
});
</script>
</html>
四、答疑解惑
这是一个非常适合前端入门练习的小案例,各位小伙伴可以自行更改样式和内容,如果大家运行时出现问题或代码有什么不懂的地方都可以随时评论留言或联系博主QQ。
还多请各位小伙伴多多点赞支持,你们的支持是我最大的动力。
博主QQ:1196094293
谢谢各位的支持~~