效果图
折叠
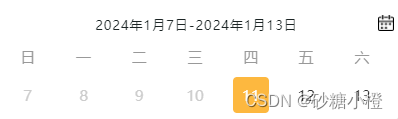
展开
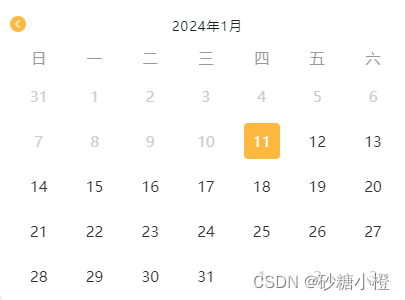
代码
1,wxml
<!-- component/calendar/calendar.wxml -->
<wxs src="./calendar.wxs" module="calculate" />
<view class="calendar">
<view class="header">
<view class="title">
<view class="title-header-wrap">
<view class="title-header-wrap-l">
<view catchtap="openChange" wx:if="{{open}}">
<image class='image' src="/images/riliback.png" mode="" />
</view>
</view>
<view class="title-header-wrap-c" wx:if="{{open}}">{{selectDay.year}}年{{selectDay.month}}月</view>
<view class="title-header-wrap-c" wx:if="{{!open}}">
<!-- <image class='t-image-l' src="/images/topleft.png" mode="" catchtap="leftclick" /> -->
{{startday.year}}年{{startday.month}}月{{startday.day}}日-{{endday.year}}年{{endday.month}}月{{endday.day}}日
<!-- <image class='t-image-r' src="/images/topright.png" mode="" catchtap="rightclick" /> -->
</view>
<view class="title-header-wrap-r">
<view catchtap="openChange" wx:if="{{!open}}">
<image class='image' src="/images/rili.png" mode="" />
</view>
</view>
</view>
<!-- <view class="header-wrap">
<view class="flex">
<view class="title">{{title}}</view>
<view class="month">
<block wx:if="{{title}}">(</block>
{{selectDay.year}}年{{selectDay.month}}月
<block wx:if="{{title}}">)</block>
</view>
</view>
<block wx:if="{{goNow}}">
<view wx:if="{{open && !(nowDay.year==selectDay.year&&nowDay.month==selectDay.month&&nowDay.day==selectDay.day)}}" class="today" bindtap="switchNowDate">
今日
</view>
</block>
</view> -->
</view>
</view>
<!-- 日历头部 -->
<view class="flex-around calendar-week">
<view class="calendar-week-item" wx:for="{{calendarHeadDate}}" wx:key="item">
{{calendarHeadDate[(index-firstDayOfWeek+7)%7]}}
</view>
</view>
<!-- 日历主体 -->
<swiper class="swiper" style="height:{{swiperHeight}}rpx" bindchange="swiperChange" circular="{{true}}" current="{{swiperCurrent}}" duration="{{swiperDuration}}">
<swiper-item wx:for="{{[dateList0, dateList1, dateList2]}}" wx:for-index="listIndex" wx:for-item="listItem" wx:key="listIndex">
<view class="flex-around flex-wrap calendar-main" style="height:{{listItem.length/7*100}}rpx">
<view wx:for="{{listItem}}" wx:key="dateList" class="day">
<view class="bg {{calculate.spot(item,selectDay,spotMap)}} {{calculate.hasNow(item,nowDay)}} {{calculate.hasNowMonth(item,selectDay)}} {{calculate.hasSelect(item,selectDay,oldCurrent,listIndex)}} {{calculate.hasDisable(item,disabledDateList)}}" catchtap="selectChange" data-day="{{item.day}}" data-year="{{item.year}}" data-month="{{item.month}}">
{{item.day}}
</view>
</view>
</view>
</swiper-item>
</swiper>
<!-- 展开收缩 -->
<!-- <view wx:if="{{showShrink}}" catchtap="openChange" class="flex list-open">
<view class="icon {{open?'fold':'unfold'}}"></view>
</view> -->
</view>
2,js
// component/calendar/calendar.js
Component({
/**
* 组件的属性列表
*/
properties: {
spotMap: {
//标点的日期
type: Object,
value: {},
},
defaultTime: {
//标记的日期,默认为今日 注意:传入格式推荐为'2022/1/2'或'2022/01/02', 其他格式在ios系统上可能出现问题
type: String,
value: '',
},
title: {
//标题
type: String,
value: '',
},
goNow: {
// 是否有快速回到今天的功能
type: Boolean,
value: true,
},
defaultOpen: {
// 是否是打开状态
type: Boolean,
value: false,
},
showShrink: {
// 是否显示收缩展开功能
type: Boolean,
value: true,
},
// 指定不可用日期
disabledDate: null,
changeTime: {
// 要改变的日期
type: String,
value: '',
},
firstDayOfWeek: {
// 周起始日
type: Number,
value: 1,
},
},
/**
* 组件的初始数据
*/
data: {
selectDay: {}, //选中的日期
nowDay: {}, //现在的日期
open: false,
swiperCurrent: 1, //选中的日期
oldCurrent: 1, //之前选中的日期
dateList0: [], //0位置的日历数组
dateList1: [], //1位置的日历数组
dateList2: [], //2位置的日历数组
swiperDuration: 500,
swiperHeight: 0,
backChange: false, //跳过change切换
disabledDateList: {}, //禁用的日期集合
calendarHeadDate: ['一', '二', '三', '四', '五', '六', '日'], //日历头部的渲染数组
startday: "", //开始日期
endday: "", //结束日期
},
/**
* 组件的方法列表
*/
methods: {
//初始化选择的日历
cominit(index) {
this.setData({
startday: this.data[`dateList${index}`][0],
endday: this.data[`dateList${index}`][this.data[`dateList${index}`].length - 1],
});
},
// 日历滑动时触发的方法
swiperChange(e) {
if (this.data.backChange) {
this.setData({
backChange: false,
});
return;
}
//计算第三个索引
let rest = 3 - e.detail.current - this.data.oldCurrent;
let dif = e.detail.current - this.data.oldCurrent;
let date;
if (dif === -2 || (dif > 0 && dif !== 2)) {
//向左划的情况,日期增加
if (this.data.open) {
date = new Date(this.data.selectDay.year, this.data.selectDay.month);
this.setMonth(date.getFullYear(), date.getMonth() + 1, undefined);
this.getIndexList({
setYear: this.data.selectDay.year,
setMonth: this.data.selectDay.month,
dateIndex: rest,
});
} else {
date = new Date(
this.data.selectDay.year,
this.data.selectDay.month - 1,
this.data.selectDay.day + 7
);
this.setMonth(
date.getFullYear(),
date.getMonth() + 1,
date.getDate()
);
this.getIndexList({
setYear: this.data.selectDay.year,
setMonth: this.data.selectDay.month - 1,
setDay: this.data.selectDay.day + 7,
dateIndex: rest,
});
}
} else {
//向右划的情况,日期减少
if (this.data.open) {
date = new Date(
this.data.selectDay.year,
this.data.selectDay.month - 2
);
this.setMonth(date.getFullYear(), date.getMonth() + 1, undefined);
this.getIndexList({
setYear: this.data.selectDay.year,
setMonth: this.data.selectDay.month - 2,
dateIndex: rest,
});
} else {
date = new Date(
this.data.selectDay.year,
this.data.selectDay.month - 1,
this.data.selectDay.day - 7
);
this.setMonth(
date.getFullYear(),
date.getMonth() + 1,
date.getDate()
);
this.getIndexList({
setYear: this.data.selectDay.year,
setMonth: this.data.selectDay.month - 1,
setDay: this.data.selectDay.day - 7,
dateIndex: rest,
});
}
}
this.setData({
oldCurrent: e.detail.current,
});
this.setSwiperHeight(e.detail.current);
},
//左点击
leftclick() {
const swiperCurrent = this.data.oldCurrent > 0 ? this.data.oldCurrent - 1 : 2;
this.swiperChange({
detail: {
current: swiperCurrent
}
})
},
//右点击
rightclick() {
const swiperCurrent = this.data.oldCurrent < 2 ? this.data.oldCurrent + 1 : 0;
this.swiperChange({
detail: {
current: swiperCurrent
}
})
},
// 根据指定位置数组的大小计算日历的高度
setSwiperHeight(index) {
this.setData({
swiperHeight: (this.data[`dateList${index}`].length / 7) * 82 + 18,
});
},
// 更新指定的索引和月份的列表
getIndexList({
setYear,
setMonth,
setDay = void 0,
dateIndex
}) {
let appointMonth;
if (setDay) appointMonth = new Date(setYear, setMonth, setDay);
else appointMonth = new Date(setYear, setMonth);
const listName = `dateList${dateIndex}`;
const dataList = this.dateInit({
setYear: appointMonth.getFullYear(),
setMonth: appointMonth.getMonth() + 1,
setDay: appointMonth.getDate(),
hasBack: true,
});
const disabledDateList = {};
if (this.data.disabledDate)
dataList.forEach((item) => {
if (
!this.data.disabledDateList[
`disabled${item.year}M${item.month}D${item.day}`
] &&
this.data.disabledDate(item)
) {
disabledDateList[
`disabled${item.year}M${item.month}D${item.day}`
] = true;
}
});
this.setData({
[listName]: dataList,
disabledDateList: Object.assign(
this.data.disabledDateList,
disabledDateList
),
});
this.cominit(this.data.oldCurrent)
},
// 根据data更新禁用日期对象
setDisabledDateList(data) {
const disabledDateList = {};
data.forEach((item) => {
if (this.data.disabledDate(item)) {
disabledDateList[
`disabled${item.year}M${item.month}D${item.day}`
] = true;
}
});
this.setData({
disabledDateList,
});
},
// 设置月份
setMonth(setYear, setMonth, setDay) {
const day = Math.min(
new Date(setYear, setMonth, 0).getDate(),
this.data.selectDay.day
);
if (
this.data.selectDay.year !== setYear ||
this.data.selectDay.month !== setMonth
) {
const data = {
selectDay: {
year: setYear,
month: setMonth,
day: setDay ? setDay : day,
},
};
if (!setDay) {
data.open = true;
}
this.setData(data, () => {
this.triggerEventSelectDay();
});
} else {
const data = {
selectDay: {
year: setYear,
month: setMonth,
day: setDay ? setDay : day,
},
};
this.setData(data, () => {
this.triggerEventSelectDay();
});
}
},
// 展开收起
openChange() {
// 展开收起事件
this.triggerEvent('openChange', {
open: !this.data.open,
});
this.setData({
open: !this.data.open,
});
// 更新数据
const selectDate = new Date(
this.data.selectDay.year,
this.data.selectDay.month - 1,
this.data.selectDay.day
);
if (this.data.oldCurrent === 0) {
this.updateList(selectDate, -1, 2);
this.updateList(selectDate, 0, 0);
this.updateList(selectDate, 1, 1);
} else if (this.data.oldCurrent === 1) {
this.updateList(selectDate, -1, 0);
this.updateList(selectDate, 0, 1);
this.updateList(selectDate, 1, 2);
} else if (this.data.oldCurrent === 2) {
this.updateList(selectDate, -1, 1);
this.updateList(selectDate, 0, 2);
this.updateList(selectDate, 1, 0);
}
this.setSwiperHeight(this.data.oldCurrent);
},
// 选中并切换今日日期
witchDate(setDate) {
const selectDate = new Date(
this.data.selectDay.year,
this.data.selectDay.month - 1,
this.data.selectDay.day
);
let dateDiff =
(selectDate.getFullYear() - setDate.getFullYear()) * 12 +
(selectDate.getMonth() - setDate.getMonth());
let diff = dateDiff === 0 ? 0 : dateDiff > 0 ? -1 : 1;
const diffSum = (x) => (3 + (x % 3)) % 3;
if (this.data.oldCurrent === 0) {
this.updateList(setDate, -1, diffSum(2 + diff));
this.updateList(setDate, 0, diffSum(0 + diff));
this.updateList(setDate, 1, diffSum(1 + diff));
} else if (this.data.oldCurrent === 1) {
this.updateList(setDate, -1, diffSum(0 + diff));
this.updateList(setDate, 0, diffSum(1 + diff));
this.updateList(setDate, 1, diffSum(2 + diff));
} else if (this.data.oldCurrent === 2) {
this.updateList(setDate, -1, diffSum(1 + diff));
this.updateList(setDate, 0, diffSum(2 + diff));
this.updateList(setDate, 1, diffSum(0 + diff));
}
this.setData({
swiperCurrent: diffSum(this.data.oldCurrent + diff),
oldCurrent: diffSum(this.data.oldCurrent + diff),
backChange: dateDiff !== 0,
});
this.setData({
selectDay: {
year: setDate.getFullYear(),
month: setDate.getMonth() + 1,
day: setDate.getDate(),
},
},
() => {
this.triggerEventSelectDay();
}
);
this.setSwiperHeight(this.data.oldCurrent);
},
// 切换到今天
switchNowDate() {
this.witchDate(new Date());
},
// 日历主体的渲染方法
dateInit({
setYear,
setMonth,
setDay = this.data.selectDay.day,
hasBack = false,
} = {
setYear: this.data.selectDay.year,
setMonth: this.data.selectDay.month,
setDay: this.data.selectDay.day,
hasBack: false,
}) {
let dateList = []; //需要遍历的日历数组数据
let now = new Date(setYear, setMonth - 1); //当前月份的1号
let startWeek = now.getDay(); //目标月1号对应的星期
let resetStartWeek = (startWeek + this.data.firstDayOfWeek - 1) % 7; //计算星期几的位置
let dayNum = new Date(setYear, setMonth, 0).getDate(); //当前月有多少天
let forNum = Math.ceil((resetStartWeek + dayNum) / 7) * 7; //当前月跨越的周数
let selectDay = setDay ? setDay : this.data.selectDay.day;
this.triggerEvent('getDateList', {
setYear: now.getFullYear(),
setMonth: now.getMonth() + 1,
});
if (this.data.open) {
//展开状态,需要渲染完整的月份
for (let i = 0; i < forNum; i++) {
const now2 = new Date(now);
now2.setDate(i - resetStartWeek + 1);
let obj = {};
obj = {
day: now2.getDate(),
month: now2.getMonth() + 1,
year: now2.getFullYear(),
};
dateList[i] = obj;
}
} else {
//非展开状态,只需要渲染当前周
for (let i = 0; i < 7; i++) {
const now2 = new Date(now);
//当前周的7天
now2.setDate(
Math.ceil((selectDay + (resetStartWeek)) / 7) * 7 -
6 -
(resetStartWeek) +
i
);
let obj = {};
obj = {
day: now2.getDate(),
month: now2.getMonth() + 1,
year: now2.getFullYear(),
};
dateList[i] = obj;
}
}
if (hasBack) {
return dateList;
}
this.setData({
dateList1: dateList,
});
},
// 一天被点击时
selectChange(e) {
const year = e.currentTarget.dataset.year;
const month = e.currentTarget.dataset.month;
const day = e.currentTarget.dataset.day;
const selectDay = {
year: year,
month: month,
day: day,
};
if (
this.data.open &&
(this.data.selectDay.year !== year ||
this.data.selectDay.month !== month)
) {
if (
year * 12 + month >
this.data.selectDay.year * 12 + this.data.selectDay.month
) {
// 下个月
if (this.data.oldCurrent == 2)
this.setData({
swiperCurrent: 0,
});
else
this.setData({
swiperCurrent: this.data.oldCurrent + 1,
});
} else {
// 点击上个月
if (this.data.oldCurrent == 0)
this.setData({
swiperCurrent: 2,
});
else
this.setData({
swiperCurrent: this.data.oldCurrent - 1,
});
}
this.setData({
['selectDay.day']: day,
},
() => {
this.triggerEventSelectDay();
}
);
} else if (this.data.selectDay.day !== day) {
this.setData({
selectDay: selectDay,
},
() => {
this.triggerEventSelectDay();
}
);
}
},
// 选择某天时触发的事件
triggerEventSelectDay() {
if (
!this.data.disabledDateList[
'disabled' +
this.data.selectDay.year +
'M' +
this.data.selectDay.month +
'D' +
this.data.selectDay.day
]
)
this.triggerEvent('selectDay', this.data.selectDay);
},
// 更新日历列表
updateList(date, offset, index) {
if (this.data.open) {
//打开状态
const setDate = new Date(
date.getFullYear(),
date.getMonth() + offset * 1
); //取得当前日期的上个月日期
this.getIndexList({
setYear: setDate.getFullYear(),
setMonth: setDate.getMonth(),
dateIndex: index,
});
} else {
const setDate = new Date(
date.getFullYear(),
date.getMonth(),
date.getDate() + offset * 7
); //取得当前日期的七天后的日期
this.getIndexList({
setYear: setDate.getFullYear(),
setMonth: setDate.getMonth(),
setDay: setDate.getDate(),
dateIndex: index,
});
}
},
},
lifetimes: {
// 加载事件
ready() {
let now = this.data.defaultTime ?
new Date(this.data.defaultTime) :
new Date();
let selectDay = {
year: now.getFullYear(),
month: now.getMonth() + 1,
day: now.getDate(),
};
this.setData({
nowDay: {
year: now.getFullYear(),
month: now.getMonth() + 1,
day: now.getDate(),
},
});
this.setMonth(selectDay.year, selectDay.month, selectDay.day);
this.updateList(now, -1, 0);
this.updateList(now, 0, 1);
this.updateList(now, 1, 2);
this.setSwiperHeight(1);
this.cominit(this.data.oldCurrent)
},
},
observers: {
// 监听切换状态
oldCurrent(value) {
const data = [this.data.dateList0, this.data.dateList1, this.data.dateList2]
this.setData({
startday: data[value][0],
endday: data[value][data[value].length - 1],
});
},
// 重新设置打开状态
defaultOpen(value) {
this.setData({
open: value,
});
},
// 切换日期
changeTime(value) {
// 检测切换日期
if (!value) return;
this.witchDate(new Date(value));
}
},
});
3,wxs
/*
* 使用逻辑判断来控制类名
*/
// 标点的逻辑计算
function spot(item, selectDay, spotMap) {
// 只有当前月才显示标点
if (item.month === selectDay.month) {
// 通过年月日拼接的key来判断是否有标点
var key = 'y' + item.year + 'm' + item.month + 'd' + item.day;
if (spotMap[key]) {
return spotMap[key];
}
}
return '';
}
// 当前日期的逻辑计算(显示今天的日期)
function hasNow(item, nowDay) {
if (
item.year === nowDay.year &&
item.month === nowDay.month &&
item.day === nowDay.day
) {
return 'now';
}
return '';
}
// 当前月的逻辑计算(其他月的日期变灰)
function hasNowMonth(item, selectDay) {
if (item.year === selectDay.year && item.month === selectDay.month) {
return '';
}
return 'other-month';
}
// 选中日期的逻辑计算(选中的日期变色)
function hasSelect(item, selectDay, oldCurrent, listIndex) {
if (
item.year === selectDay.year &&
item.month === selectDay.month &&
item.day === selectDay.day &&
oldCurrent === listIndex
) {
return 'select';
}
return '';
}
// 禁用日期的逻辑计算(禁用的日期变灰)
function hasDisable(item, disabledDateList) {
var key = 'disabled' + item.year + 'M' + item.month + 'D' + item.day;
if (disabledDateList[key]) {
return 'other-month';
}
return '';
}
module.exports = {
spot: spot,
hasNow: hasNow,
hasNowMonth: hasNowMonth,
hasSelect: hasSelect,
hasDisable: hasDisable,
}
4,wxss
/* components/calendar-sel/calendar-sel.wxss */
/* component/calendar/calendar.wxss */
.icon {
background-image: url("data:image/png;base64,iVBORw0KGgoAAAANSUhEUgAAACAAAAAgCAYAAABzenr0AAACcUlEQVRYhe2WvWtUURDFf8dNVk1ETViMX4WNna22CopELBQUkYASgzFFUPBPsLGSgAqJGDRGBEUsRA0KIthaip2I/4CEoIR8JyP33lldl7fuR1AL9xSP3Xfvmzlz7pl5jyaa+O+hq0NDqDEV8sBGYA3wDZirP4TFhxvFApAD1gPz5TGqF2Xx2pK5puwA9uuPPUA30A48Bd43Ukg2gWpQTHwe2Iax5GRuA6//BoFuwQDQaUF6xaPYBFzCYryXf4yAoAc44We/INjgJvzqGwYwWg2e1Rqzogmt9MwTjgGngBXvgLB8Axh1HyiuiV7BwVoJ1KpAqPqMu70dtFbYNWDC7SqwfoMp0r9BLHbHi2qBa2nDk0A/aAmUF9oscR1pAikJIR4g3RXqUFIoxD0nOLQqAoLjwFlgOuyVKCDGve3KMY54BOqMrBTVDSQON0rgqLfajJCF5IJ7SmdeifBIICgISgQSbUCPYH+9BI4Ag8BidLzY4VXf+V01TmIM9BhU8Gc7gNOCfVn7s0y4N1SuNNuXhbYDDwU3qyX/QUIMm7Eo6HNj7gQueKe8KyfQ4guh2gPAZW+zWdAW4Al1JC+RYjTMBKA3xYpdcZE0O974rlxIXvDeDhv6gF1Kg6UrnrcYqTv5TwwLpi0VFRTd6oTmQB/AckUPrMS5LjpR9MU6n+2rSV7EfRQH1orSwOoC2w0WlQ+XZZ9qs/57CnQFLMnk47A4FdXYx8MYMJl8ECN9hPgSyxdNGM7qC3DLibyt9Ea3rHtmFddK8DzlURvYZ1c5GrA4ueZBr5S8EPp3JjNgyc061QgvsE9gk/4x0+qKN9FEE/8QwHd9qo6ectzgFAAAAABJRU5ErkJggg==");
background-size: 100% auto;
width: 32rpx;
height: 32rpx;
}
.flex {
display: flex;
justify-content: space-between;
align-items: center;
}
.swiper {
transition: height 0.3s;
}
.header-wrap {
display: flex;
justify-content: space-between;
align-items: center;
}
.today {
width: 88rpx;
height: 42rpx;
background: #F3F4F4;
border-radius: 22rpx;
font-size: 24rpx;
line-height: 42rpx;
color: #868D8D;
text-align: center;
margin-right: 6rpx;
}
.today:active {
background: #dfdfdf;
color: #5f6464;
}
.direction-column {
flex-direction: column;
}
.flex1 {
flex: 1;
}
.flex-center {
display: flex;
justify-content: center;
align-items: center;
}
.flex-start {
display: flex;
justify-content: flex-start;
align-items: center;
}
.flex-between {
display: flex;
justify-content: space-between;
align-items: center;
}
.flex-end {
display: flex;
justify-content: flex-end;
align-items: center;
}
.flex-around {
display: flex;
justify-content: space-around;
align-items: center;
}
.flex-wrap {
flex-wrap: wrap;
}
.align-start {
align-items: flex-start;
}
.align-end {
align-items: flex-end;
}
.align-stretch {
align-items: stretch;
}
.calendar {
font-family: "PingFang SC", -apple-system, BlinkMacSystemFont, Roboto, "Helvetica Neue", Helvetica, Arial, "Hiragino Sans GB", "Source Han Sans", "Noto Sans CJK Sc", "Microsoft YaHei", "Microsoft Jhenghei", sans-serif;
}
.calendar .title {
padding: 10rpx 16rpx 10rpx 20rpx;
line-height: 60rpx;
font-size: 32rpx;
font-weight: 600;
color: #1C2525;
letter-spacing: 1px;
}
.calendar .title .year-month {
margin-right: 20rpx;
}
.calendar .title .icon {
padding: 0 16rpx;
font-size: 32rpx;
color: #999;
}
.calendar .title .open {
background-color: #f6f6f6;
color: #999;
font-size: 22rpx;
line-height: 36rpx;
border-radius: 18rpx;
padding: 0 14rpx;
}
.list-open {
position: relative;
justify-content: center;
}
.list-open .icon::after {
content: '';
position: absolute;
top: 16rpx;
right: 60rpx;
display: block;
width: 278rpx;
height: 0rpx;
border-bottom: 2rpx solid rgba(214, 219, 219, 0.68);
}
.list-open .icon::before {
content: '';
position: absolute;
top: 16rpx;
left: 60rpx;
display: block;
width: 278rpx;
height: 0rpx;
border-bottom: 2rpx solid rgba(214, 219, 219, 0.68);
}
.fold {
transform: rotate(0deg);
}
.unfold {
transform: rotate(180deg);
}
.calendar .calendar-week {
line-height: 40rpx;
padding: 0 22rpx;
font-size: 28rpx;
color: #999;
}
.calendar .calendar-week .calendar-week-item {
width: 100rpx;
text-align: center;
}
.calendar .calendar-main {
padding: 18rpx 22rpx 0rpx;
transition: height 0.3s;
align-content: flex-start;
overflow: hidden;
}
.calendar .calendar-main .day {
position: relative;
width: 90rpx;
color: #666;
text-align: center;
height: 82rpx;
}
.calendar .calendar-main .day .bg {
height: 66rpx;
line-height: 66rpx;
font-size: 28rpx;
color: #333;
}
.calendar .calendar-main .day .now {
width: 66rpx;
border-radius: 8rpx;
text-align: center;
color: #FDB83F;
background: #ffe7bd;
margin: 0 auto;
}
.calendar .calendar-main .day .select {
width: 66rpx;
border-radius: 8rpx;
text-align: center;
color: #fff;
background: #FDB83F;
margin: 0 auto;
}
.calendar .calendar-main .day .spot::after {
position: absolute;
content: "";
display: block;
width: 8rpx;
height: 8rpx;
bottom: 22rpx;
background: #FDB83F;
border-radius: 50%;
left: 0;
right: 0;
margin: auto;
}
.calendar .calendar-main .day .deep-spot::after {
position: absolute;
content: "";
display: block;
width: 8rpx;
height: 8rpx;
bottom: 22rpx;
background: #FF7416;
border-radius: 50%;
left: 0;
right: 0;
margin: auto;
}
.calendar .calendar-main .day .other-month {
color: #ccc;
background: transparent;
}
.header-wrap .month {
font-size: 28rpx;
color: #929797;
}
.title-header-wrap {
width: 100%;
display: flex;
justify-content: space-between;
align-items: center;
}
.title-header-wrap-l {
width: 30rpx;
}
.title-header-wrap-r {
width: 30rpx;
}
.image {
width: 30rpx;
height: 30rpx;
}
.t-image-l {
width: 24rpx;
height: 24rpx;
margin-right: 10rpx;
}
.t-image-r {
width: 24rpx;
height: 24rpx;
margin-left: 10rpx;
}
.title-header-wrap-c {
font-size: 24rpx;
font-family: PingFang SC;
font-weight: 400;
line-height: 0rpx;
display: flex;
align-items: center;
}