/*GetAddress
todo 6、区块链地址的生成
根据钱包publicKey生成地址
1、获取钱包公钥publicKey
2、sha256加密publicKey => hash1
3、hash1 再进行 ripemd160算法加密 => 20字节 公钥publicKeyHash
4、拼接Version => 左半段 21字节(leftBytes)
5、右半段 进行两次sha256 => checkSum
6、取checkSum前4个字节 => checkRight
7、与左半段21字节(leftBytes) + checkRight 拼接 => 25Bytes
8、25Bytes 进行base58()编码 => address
*/ |
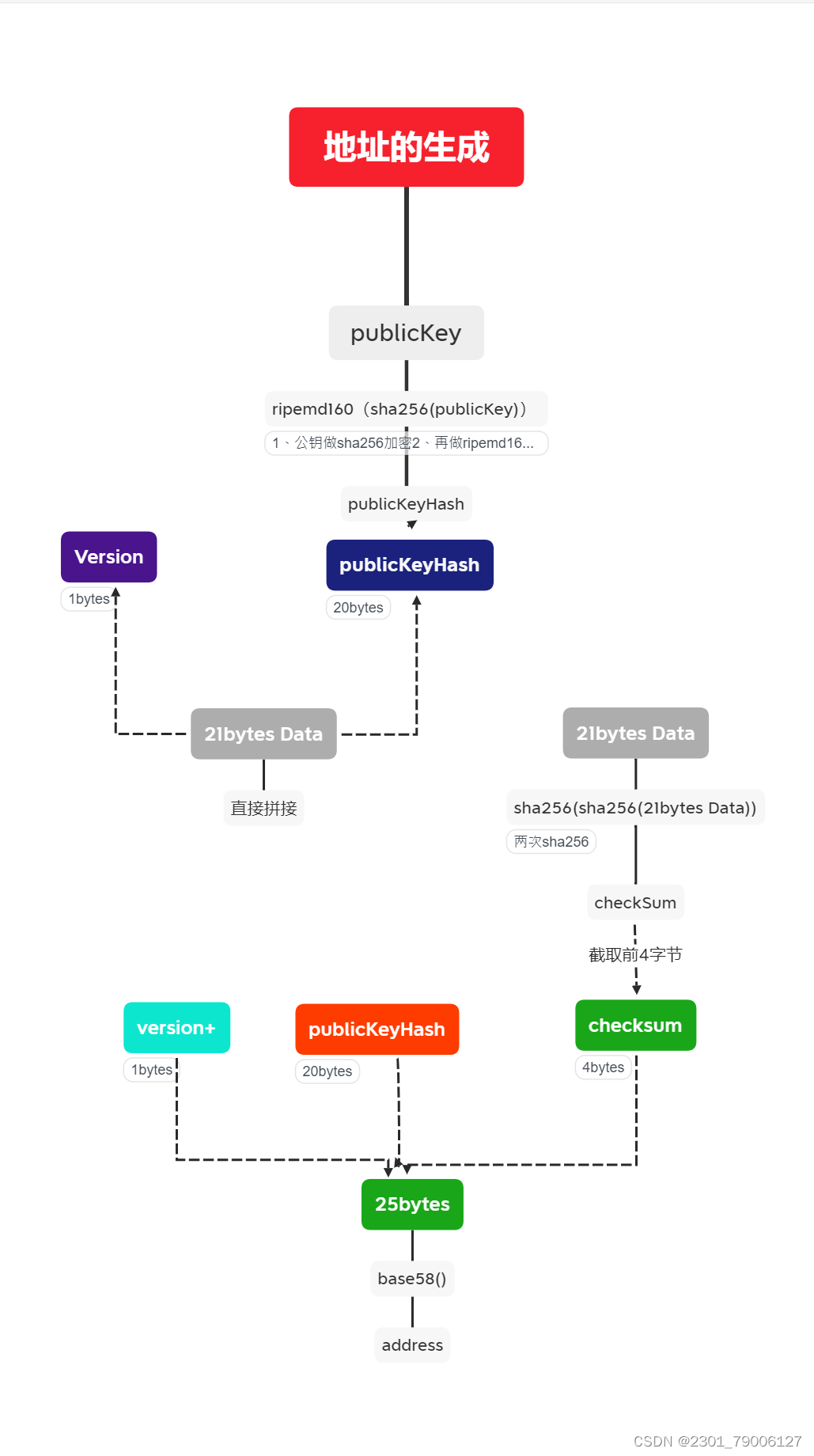
package main
import (
"crypto/ecdsa"
"crypto/elliptic"
"crypto/rand"
"crypto/sha256"
"fmt"
"github.com/iocn-io/ripemd160"
"github.com/mr-tron/base58/base58"
"log"
)
func main() {
/*
todo 5、调用区块链钱包,查看公私钥
*/
var wallet = NewWalletKeuPair()
fmt.Printf("Private:%s\n",wallet.PrivateKey)
fmt.Println("-------------")
fmt.Printf("PublickKey : %x\n",wallet.PublicKey)
/*
todo 7、获取区块链钱包地址
*/
address := wallet.GetAddress()
fmt.Printf("区块链钱包地址为:%s\n",address)
}
/*
todo 1、创建一个项目
todo 2、创建一个文件夹,创建一个address.go 文件
*/
/*WalletKeyPair
todo 3、创建区块链钱包结构体
*/
type WalletKeyPair struct {
PrivateKey *ecdsa.PrivateKey // 私钥
PublicKey []byte // 公钥
}
/*NewWalletKeuPair
todo 4、生成区块链钱包公私钥
返回区块链钱包
*/
func NewWalletKeuPair() *WalletKeyPair {
// 第一步,生成私钥
privateKey, err := ecdsa.GenerateKey(elliptic.P256(), rand.Reader)
if err != nil {
log.Panic(err)
}
publickeyRow := privateKey.PublicKey
// 第二步:拼接得到公钥
publicKey := append(publickeyRow.X.Bytes(), publickeyRow.Y.Bytes()...)
// 第三步:组装钱包地址的私钥公钥。并返回
walletKeyPair := WalletKeyPair{
privateKey,
publicKey,
}
return &walletKeyPair
}
/*GetAddress
todo 6、区块链地址的生成
根据钱包publicKey生成地址
1、获取钱包公钥publicKey
2、sha256加密publicKey => hash1
3、hash1 再进行 ripemd160算法加密 => 20字节 公钥publicKeyHash
4、拼接Version => 左半段 21字节(leftBytes)
5、右半段 进行两次sha256 => checkSum
6、取checkSum前4个字节 => checkRight
7、与左半段21字节(leftBytes) + checkRight 拼接 => 25Bytes
8、25Bytes 进行base58()编码 => address
*/
func (wallet *WalletKeyPair) GetAddress() string {
//1、获取钱包公钥publicKey
publicKey := wallet.PublicKey
//2、sha256加密publicKey => hash1
hash1 := sha256.Sum256(publicKey)
//3、hash1 再进行 ripemd160算法加密 => 20字节 公钥publicKeyHash
rip160Haher := ripemd160.New()
_, err := rip160Haher.Write(hash1[:])
if err != nil {
log.Panic(err)
}
publicKeyHash := rip160Haher.Sum(nil)
//4、拼接Version => 左半段 21字节(leftBytes)
version := 0x00
leftBytes := append([]byte{byte(version)}, publicKeyHash...)
//5、右半段 进行两次sha256 => checkSum
first := sha256.Sum256(leftBytes)
second := sha256.Sum256(first[:])
//6、取checkSum前4个字节 => checkRight
// 4个字节
checkRight := second[0:4]
// 7、与左半段21字节(leftBytes) + checkRight 拼接 => 25Bytes
t25Bytes := append(leftBytes, checkRight...)
fmt.Printf("25字节: %+v\n",t25Bytes)
//8、25Bytes 进行base58()编码 => address
// 返回地址
address := base58.Encode(t25Bytes)
return address
}