bool shouldRepaint(CustomPainter oldDelegate) => true;
}
Rect.fromCenter({ Offset center, double width, double height }) : this.fromLTRB(
center.dx - width / 2,
center.dy - height / 2,
center.dx + width / 2,
center.dy + height / 2,
);
center | 矩形中心坐标点 |
width | 矩形宽度 |
height | 矩形高度 |
class MyPainter extends CustomPainter {
@override
void paint(Canvas canvas, Size size) {
var paint = Paint()
…isAntiAlias = true
…strokeWidth=1.0
…style=PaintingStyle.fill
…color=Colors.green
…invertColors=false;
Rect rect=Rect.fromCenter(center: Offset(size.width/2,size.height/2),
width:size.width/2,height:size.height/2);
canvas.drawRect(rect, paint);
}
//在实际场景中正确利用此回调可以避免重绘开销,本示例我们简单的返回true
@override
bool shouldRepaint(CustomPainter oldDelegate) => true;
}
class MyPainter extends CustomPainter {
@override
void paint(Canvas canvas, Size size) {
var paint = Paint()
…isAntiAlias = true
…strokeWidth=1.0
…style=PaintingStyle.fill
…color=Colors.green
…invertColors=false;
Rect a=Rect.fromCircle(center: Offset(size.width/2,size.height/2),radius: size.width/2);
Rect b=Rect.fromCircle(center: Offset(size.width/2,size.height/2),radius: size.width/3);
Rect rect=Rect.lerp(a, b, 0.5);
canvas.drawRect(a, paint…color=Colors.red);
canvas.drawRect(rect, paint…color=Colors.grey);
canvas.drawRect(b, paint…color=Colors.green);
}
//在实际场景中正确利用此回调可以避免重绘开销,本示例我们简单的返回true
@override
bool shouldRepaint(CustomPainter oldDelegate) => true;
}
源码:
static Rect lerp(Rect a, Rect b, double t) {
assert(t != null);
if (a == null && b == null)
return null;
if (a == null)
return Rect.fromLTRB(b.left * t, b.top * t, b.right * t, b.bottom * t);
if (b == null) {
final double k = 1.0 - t;
return Rect.fromLTRB(a.left * k, a.top * k, a.right * k, a.bottom * k);
}
return Rect.fromLTRB(
lerpDouble(a.left, b.left, t),
lerpDouble(a.top, b.top, t),
lerpDouble(a.right, b.right, t),
lerpDouble(a.bottom, b.bottom, t),
);
}
double lerpDouble(num a, num b, double t) {
if (a == null && b == null)
return null;
a ??= 0.0;
b ??= 0.0;
return a + (b - a) * t;
}
PaintingStyle.fill(用画笔绘制边框)
class MyPainter extends CustomPainter {
@override
void paint(Canvas canvas, Size size) {
var paint = Paint()
…isAntiAlias = true
…strokeWidth=1.0
…style=PaintingStyle.stroke
…color=Colors.green
…invertColors=false;
Rect a=Rect.fromCircle(center: Offset(size.width/2,size.height/2),radius: size.width/2);
Rect b=Rect.fromCircle(center: Offset(size.width/2,size.height/2),radius: size.width/3);
Rect rect=Rect.lerp(a, b, 0.5);
canvas.drawRect(a, paint…color=Colors.red);
canvas.drawRect(rect, paint…color=Colors.grey);
canvas.drawRect(b, paint…color=Colors.green);
}
//在实际场景中正确利用此回调可以避免重绘开销,本示例我们简单的返回true
@override
bool shouldRepaint(CustomPainter oldDelegate) => true;
}
class MyPainter extends CustomPainter {
@override
void paint(Canvas canvas, Size size) {
var paint = Paint()
…isAntiAlias = true
…strokeWidth=1.0
…style=PaintingStyle.stroke
…color=Colors.green
…invertColors=false;
double cx=size.width/2,cy=size.height/2;
double radius=size.width/4;
Rect rect=Rect.fromCircle(center: Offset(cx,cy),radius: radius);
canvas.drawRect(rect, paint);
}
//在实际场景中正确利用此回调可以避免重绘开销,本示例我们简单的返回true
@override
bool shouldRepaint(CustomPainter oldDelegate) => true;
}
class MyPainter extends CustomPainter {
@override
void paint(Canvas canvas, Size size) {
var paint = Paint()
…isAntiAlias = true
…strokeWidth=1.0
…style=PaintingStyle.stroke
…color=Colors.green
…invertColors=false;
Rect rect=Rect.fromPoints(Offset(size.width/2, 0), Offset(0.0, size.height/2));
canvas.drawRect(rect, paint);
rect=Rect.fromPoints(Offset(size.width/3, 0), Offset(0.0, size.height/3));
canvas.drawRect(rect, paint…color=Colors.red);
rect=Rect.fromPoints(Offset(0, 0), Offset(size.width/4, size.height/4));
canvas.drawRect(rect, paint…color=Colors.blue);
}
//在实际场景中正确利用此回调可以避免重绘开销,本示例我们简单的返回true
@override
bool shouldRepaint(CustomPainter oldDelegate) => true;
}
俄罗斯方块的一面
class MyPainter extends CustomPainter {
@override
void paint(Canvas canvas, Size size) {
var paint = Paint()
…isAntiAlias = true
…strokeWidth = 1.0
…style = PaintingStyle.fill
…color = Colors.green
…invertColors = false;
double w = size.width / 3;
double h = size.height / 3;
for (int i = 0; i < 3; i++) {
for (int j = 0; j < 3; j++) {
Rect rect = Rect.fromPoints(
Offset(w * j, h * i), Offset(w * (j + 1), h * (i + 1)));
switch (i) {
case 0:
canvas.drawRect(rect, paint…color = (i % 2 == 0 &&j%2==0? Colors.blue : Colors.deepOrange));
break;
case 1:
canvas.drawRect(rect, paint…color = (i % 3 == 1 &&j%3==0? Colors.red : Colors.green));
break;
case 2:
canvas.drawRect(rect, paint…color = (i % 2 == 0 && j%2==0? Colors.amber : Colors.deepPurpleAccent));
break;
}
}
}
}
//在实际场景中正确利用此回调可以避免重绘开销,本示例我们简单的返回true
@override
bool shouldRepaint(CustomPainter oldDelegate) => true;
}
class MyPainter extends CustomPainter {
@override
void paint(Canvas canvas, Size size) {
var paint = Paint()
…isAntiAlias = true
…strokeWidth = 1.0
…style = PaintingStyle.fill
…color = Colors.green
…invertColors = false;
double w = size.width / 3;
double h = size.height / 3;
for (int i = 0; i < 3; i++) {
for (int j = 0; j < 3; j++) {
Rect rect = Rect.fromPoints(
Offset(w * j, h * i), Offset(w * (j + 1), h * (i + 1)));
switch (i) {
case 0:
canvas.drawRect(rect, paint…color = (i % 2 == 0 &&j%2==0? Colors.blue : Colors.deepOrange));
break;
case 1:
canvas.drawRect(rect, paint…color = (i % 3 == 1 &&j%3==1? Colors.red : Colors.green));
break;
case 2:
canvas.drawRect(rect, paint…color = (i % 2 == 0 &&j%2==0? Colors.amber : Colors.deepPurpleAccent));
break;
}
}
}
}
//在实际场景中正确利用此回调可以避免重绘开销,本示例我们简单的返回true
@override
bool shouldRepaint(CustomPainter oldDelegate) => true;
}
五彩网格
class MyPainter extends CustomPainter {
@override
void paint(Canvas canvas, Size size) {
var paint = Paint()
…isAntiAlias = true
…strokeWidth = 1.0
…style = PaintingStyle.stroke
…color = Colors.green
…invertColors = false;
double w = size.width / 9;
double h = size.height / 9;
for (int i = 0; i < 9; i++) {
for (int j = 0; j < 9; j++) {
Rect rect = Rect.fromPoints(
Offset(w * j, h * i), Offset(w * (j + 1), h * (i + 1)));
switch (i) {
case 0:
case 8:
case 6:
canvas.drawRect(rect, paint…color = (i % 2 == 0 &&j%2==0? Colors.blue : Colors.deepOrange));
break;
case 1:
case 3:
case 5:
canvas.drawRect(rect, paint…color = (i % 3 == 1 &&j%3==1? Colors.red : Colors.green));
break;
case 2:
case 7:
case 4:
canvas.drawRect(rect, paint…color = (i % 2 == 0 &&j%2==0? Colors.amber : Colors.deepPurpleAccent));
break;
}
}
}
}
自我介绍一下,小编13年上海交大毕业,曾经在小公司待过,也去过华为、OPPO等大厂,18年进入阿里一直到现在。
深知大多数初中级Android工程师,想要提升技能,往往是自己摸索成长或者是报班学习,但对于培训机构动则近万的学费,着实压力不小。自己不成体系的自学效果低效又漫长,而且极易碰到天花板技术停滞不前!
因此收集整理了一份《2024年Android移动开发全套学习资料》,初衷也很简单,就是希望能够帮助到想自学提升又不知道该从何学起的朋友,同时减轻大家的负担。
既有适合小白学习的零基础资料,也有适合3年以上经验的小伙伴深入学习提升的进阶课程,基本涵盖了95%以上Android开发知识点,真正体系化!
由于文件比较大,这里只是将部分目录截图出来,每个节点里面都包含大厂面经、学习笔记、源码讲义、实战项目、讲解视频,并且会持续更新!
如果你觉得这些内容对你有帮助,可以扫码获取!!(备注:Android)
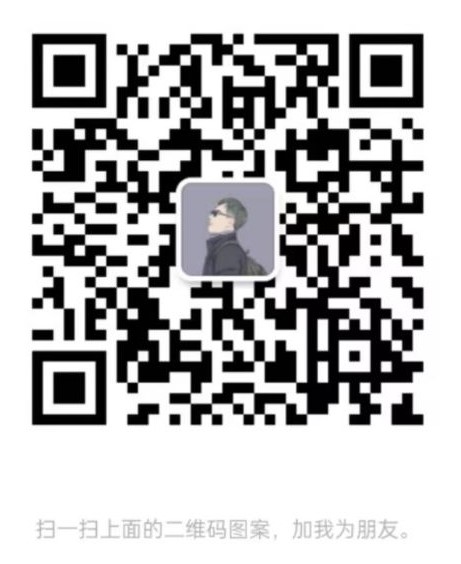
结尾
如何才能让我们在面试中对答如流呢?
答案当然是平时在工作或者学习中多提升自身实力的啦,那如何才能正确的学习,有方向的学习呢?为此我整理了一份Android学习资料路线:
这里是一份BAT大厂面试资料专题包:
好了,今天的分享就到这里,如果你对在面试中遇到的问题,或者刚毕业及工作几年迷茫不知道该如何准备面试并突破现状提升自己,对于自己的未来还不够了解不知道给如何规划。来看看同行们都是如何突破现状,怎么学习的,来吸收他们的面试以及工作经验完善自己的之后的面试计划及职业规划。
《互联网大厂面试真题解析、进阶开发核心学习笔记、全套讲解视频、实战项目源码讲义》点击传送门即可获取!
于文件比较大,这里只是将部分目录截图出来,每个节点里面都包含大厂面经、学习笔记、源码讲义、实战项目、讲解视频,并且会持续更新!**
如果你觉得这些内容对你有帮助,可以扫码获取!!(备注:Android)
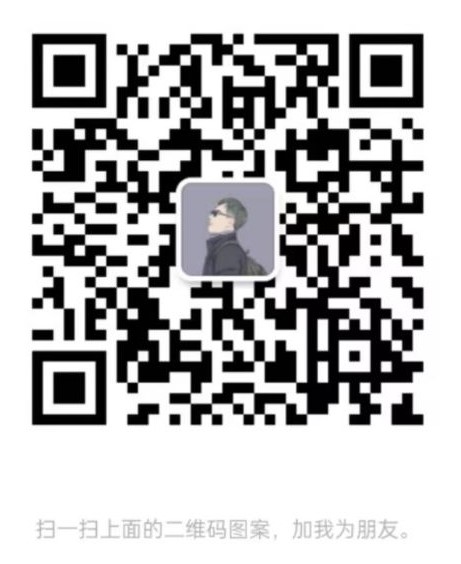
结尾
如何才能让我们在面试中对答如流呢?
答案当然是平时在工作或者学习中多提升自身实力的啦,那如何才能正确的学习,有方向的学习呢?为此我整理了一份Android学习资料路线:
[外链图片转存中…(img-aB2NE9IE-1712588694608)]
这里是一份BAT大厂面试资料专题包:
[外链图片转存中…(img-JzU4WLAN-1712588694608)]
好了,今天的分享就到这里,如果你对在面试中遇到的问题,或者刚毕业及工作几年迷茫不知道该如何准备面试并突破现状提升自己,对于自己的未来还不够了解不知道给如何规划。来看看同行们都是如何突破现状,怎么学习的,来吸收他们的面试以及工作经验完善自己的之后的面试计划及职业规划。
《互联网大厂面试真题解析、进阶开发核心学习笔记、全套讲解视频、实战项目源码讲义》点击传送门即可获取!