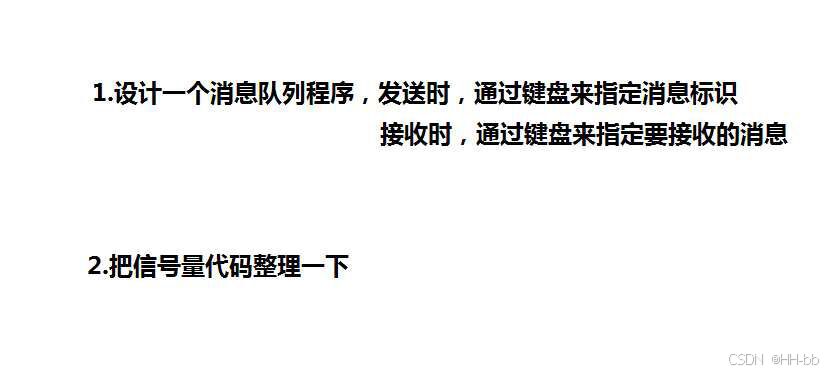
//写
#include <stdio.h>
#include <sys/types.h>
#include <sys/ipc.h>
#include <sys/msg.h>
#include <string.h>
#define SIZE 1024
//用消息队列的IPC发送消息
typedef struct
{
long mytype;
char mtext[SIZE];
}BUF;
int main(int argc, const char *argv[])
{
//1.创建key值
key_t key = ftok("./",0);
if(key == -1)
{
printf("create key fail");
return -1;
}
//2.创建消息队列
int msgid = msgget(key, IPC_CREAT | 0777);
if(msgid == -1)
{
printf("create msgid fail");
return -1;
}
BUF buf;
long tag;
printf("Please enter an TAG\n");
scanf("%ld",&tag);
buf.mytype = tag;
getchar();
//3.通过消息队列发送
while(1)
{
memset(buf.mtext,0,SIZE);
//消息从键盘获取
printf("Please enter a message\n");
fgets(buf.mtext,SIZE,stdin);//以回车结尾
int snd = msgsnd(msgid, &buf,sizeof(buf),0);
if(snd == -1)
{
printf("snde fail");
}
if(strcmp(buf.mtext, "bye\n") == 0)
{
break;
}
}
return 0;
}
//读
#include <stdio.h>
#include <sys/types.h>
#include <sys/ipc.h>
#include <sys/msg.h>
#include <string.h>
#define SIZE 1024
//用消息队列rcv消息
typedef struct
{
long mytype;
char mtext[SIZE];
}BUF;
int main(int argc, const char *argv[])
{
//1.创建key值
key_t key = ftok("./",0);
if(key == -1)
{
printf("create key fail");
return -1;
}
//2.创建消息队列
int msgid = msgget(key, IPC_CREAT|0777);
if(msgid == -1)
{
printf("create msgid fail");
return -1;
}
BUF buf;
long tag;
printf("Please enter an TAG\n");
scanf("%ld",&tag);
//3.通过消息队列接收
while(1)
{
memset(buf.mtext,0,SIZE);
ssize_t rcv = msgrcv(msgid,&buf,sizeof(buf),tag,0);
if(rcv == -1)
{
printf("rcv fail");
}
if(strcmp(buf.mtext, "bye\n") == 0)
{
break;
}
printf("MSG:%s\n",buf.mtext);
}
return 0;
}