前言:
在前面,我们学习了C++的类与对象,认识到了C++与C语言的一些不同,今天,我们将进入C++的 关键部分——STL,学习完这部分之后,我们就可以清楚的认识到C++相比于C语言的快捷与便利
目录
一、为什么有string类
在我们学习C语言的时候,有一个点是非常难处理的,那就是字符串,在我们对字符串访问,增删查改时都是非常不便的,所以我们封装了一个string类主要来处理字符串有关的问题
二、标准库中的string类
1、什么是string类
我们可以简单的把string类理解为变长的字符数组,我们可以对它进行增删查改等一系列操作,同时有一些列封装的接口函数提供给我们可以让我们直接使用,一般我们需要的功能函数都有
string类的成员函数:
class string
{
private:
char* a;
int _capacity;
int _size;
};
2、string的常用接口函数
在使用string类时,必须包含#include头文件以及using namespace std;
这些接口函数的原理我们在后面再讲,下面我们就先讲一下这些接口函数的用法,学会了用法就可以直接使用string类来做题了,这部分内容没啥重点讲解的,下面主要是直接给出代码示例
2.1 string类对象的构造
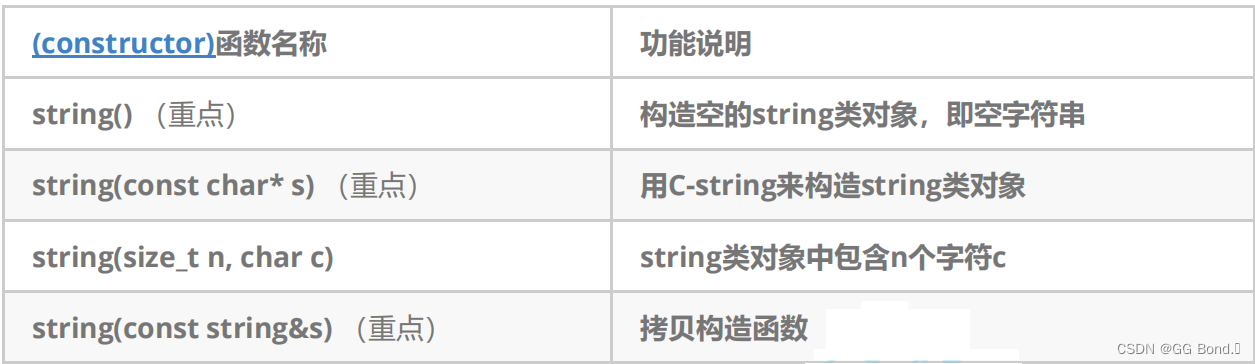
代码实例:
#include<string>
#include<iostream>
using namespace std;
int main()
{
string(); //1、构建了一个空的string对象,这个对象只在本行起作用,除非加const修饰
string s1("abc"); //2、直接构造
cout << "s1:" << s1 << endl;
char arr[] = "abc";
string s2(arr); //3、用一个字符串的首地址来构造
cout << "s2:" << s2 << endl;
string s3 = s1; //4、拷贝构造(用一个已经存在的类对象给另一个对象初始化)
cout << "s3:" << s3 << endl;
string s4(3, 'x'); //5、构造时将前N个赋值为同一个字符
cout << "s4:" << s4 << endl;
return 0;
}
运行结果:
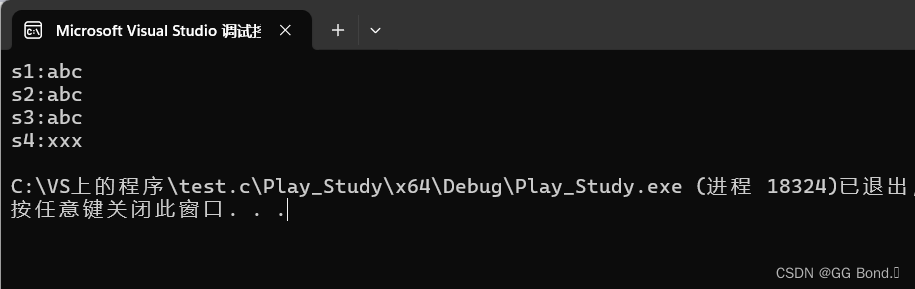
2.2 string的容量操作
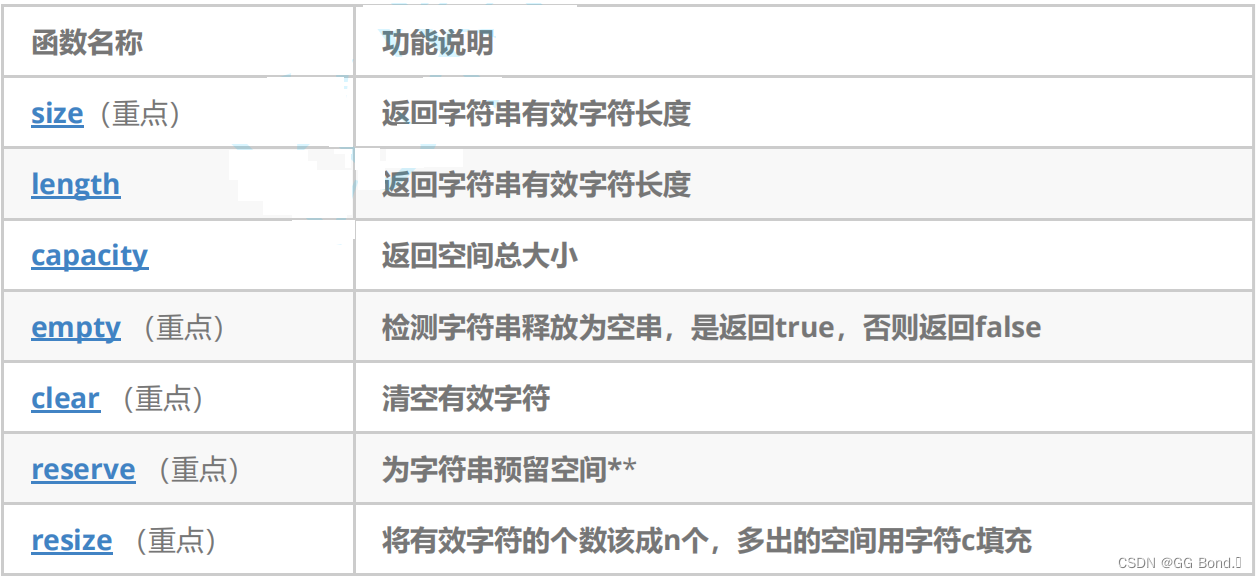
代码实例:
#include<string>
#include<iostream>
using namespace std;
int main()
{
string s1("abcdef");
cout <<"s1:"<< s1 << endl;
cout << "size:" << s1.size() << endl; //有效字符的个数
cout << "length:" << s1.length() << endl; //有效字符的个数
//上面这两个功能上差别不大,一般我们用size()用的多一点
cout << "capacity:" << s1.capacity() << endl;
//开辟的空间大小(当空间不够时会自动扩容,扩容空间为原空间的1.5倍(与环境有关))
cout << "empty:" << s1.empty() << endl; //检查字符串是否为空,0表示非空,1表示空
s1.clear(); //清空字符串
cout <<"s1:"<< s1 << endl;
s1.reserve(100); //开辟指定大小空间(一般会多一点)
cout << "capacity:" << s1.capacity() << endl;
s1.resize(5, 'a');
cout << "size:" << s1.size() << endl;
cout << "s1:" << s1 << endl;
return 0;
}
运行结果:
2.3 string类的访问与遍历
代码实例:
#include<iostream>
using namespace std;
#include<string>
int main()
{
string s1("abcdef");
//访问方法:下标访问法
cout << s1[0] << endl;
cout << s1[3] << endl;
s1[0] = 'h';
//1、下标遍历法
cout << "下标遍历法:";
for (int i = 0; i < s1.size(); i++)
{
cout << s1[i] << " ";
}
cout << endl;
//2、迭代器法(正向)
cout << "迭代器法(正向):";
string::iterator it = s1.begin();
for (; it != s1.end(); it++)
{
cout << *it << " ";
}
cout << endl;
//3、迭代器(反向)
cout << "迭代器(反向):";
string::reverse_iterator rit = s1.rbegin();
while (rit != s1.rend())
{
cout << *rit << " ";
rit++;
}
cout << endl;
//范围for法
cout << "范围for法:";
for (auto e : s1)
{
cout << e << " ";
}
cout << endl;
return 0;
}
运行结果:
2.4 string类对象的修改
代码实例:
#include<iostream>
using namespace std;
#include<string>
int main()
{
string s1("zhan");
cout << s1 << endl;
//push_back 在末尾加入字符
cout << "push_back后:";
s1.push_back('g');
cout << s1 << endl;
//append 在末端加入字符串
cout << "append后:" ;
s1.append(" san");
cout << s1 << endl;
//operator+= 在末端随意添加
cout << "+=后:";
s1 += " 18";
cout << s1 << endl;
//c_str 返回C格式字符串
cout << "c_str:";
const char* m = s1.c_str();
cout << m << endl;
//find 从pos位置开始查找字符并返回其位置
cout << "find:";
int npos1 = s1.find('a');
cout << npos1 << endl;
//rfind 从pos位置开始往前查找字符并返回其位置
cout << "rfind:";
int npos2 = s1.rfind('a');
cout << npos2 << endl;
//substr 从pos位置开始截取n个字符并返回
cout << "substr后:";
string tmp = s1.substr(npos1, npos2 - npos1);
cout << tmp << endl;
return 0;
}
运行结果:
2.5 string类常用的非成员函数
代码实例:
#include<iostream>
using namespace std;
#include<string>
int main()
{
string s1("hello ");
string s2("world");
//operator+ 涉及深层拷贝,不建议多用
cout << "operator+后:";
cout << operator+(s1, s2) << endl;
//operator>> 输入运算符重载
cout << "operator>>:";
string s3;
operator>>(cin,s3);
cout << s3 << endl;
//operator<< 输出运算符重载
cout << "operator<<:";
operator<<(cout, s1) << endl;
//getline 获取一行字符串
cout << "getline:";
string s4;
getline(cin, s4); //这个在这个程序中测不出来,需要单独测试
cout << s4 << endl;
//relational operators 比较大小
//这个函数库中有各种各样的比较函数(==、>、<......),函数类型为bool,感兴趣的可以自己探索一下
return 0;
}
运行结果:
三、总结
上面的就是我们常用的string类的类成员函数以及类外函数,由于这些函数已经封装好了,所以我们平时可以直接使用,至于如何实现这些函数,我们下章再讲
感谢各位大佬观看,创作不易,还请一键三连!!!