#include<iostream>
using namespace std;
typedef char ElemType;
//定义循环单链表数据结构
typedef struct LNode
{
ElemType data;
struct LNode *next;
}LinkNode;
//初始化循环单链表
void InitList(LinkNode *&h)
{
h=(LinkNode *)malloc(sizeof(LinkNode));
h->next=h;
}
//尾插法
void CreateListR(LinkNode *&h,ElemType a[],int n)
{
LinkNode *s,*r;
r=h;
for(int i=0;i<n;i++)
{
s=(LinkNode *)malloc(sizeof(LinkNode));
s->data=a[i];
r->next=s;
r=s;
}
r->next=h;
}
//输出循环单链表
void DispList(LinkNode *h)
{
LinkNode *p=h->next;
while(p!=h)
{
cout<<p->data<<" ";
p=p->next;
}
printf("\n");
}
//输出循环单链表长度
int ListLength(LinkNode *h)
{
int n=0;
LinkNode *p=h;
while(p->next!=h)
{
n++;
p=p->next;
}
return n;
}
//判断循环单链表是否空
bool ListEmpty(LinkNode *h)
{
return(h->next==h);
}
//输出某个元素
bool GetElem(LinkNode *h,int i,ElemType &e)
{
int j=0;
LinkNode *p=h;
if(i<=0) return false;
while(j<i&&p->next!=h)
{
j++;
p=p->next;
}
if(p->next==h)
return false;
else
{
e=p->data;
return true;
}
}
//输出某个元素的位置
int LocateElem(LinkNode *h,ElemType e)
{
int i=1;
LinkNode *p=h->next;
while(p!=h&&p->data!=e)
{
p=p->next;
i++;
}
if(p==h)
return (0);
else
return(i);
}
//在某位置插入元素
bool ListInsert(LinkNode *&h,int i,ElemType e)
{
int j=0;
LinkNode *p=h,*s;
if(i<=0) return false;
while(j<i-1&&p->next!=h)
{
j++;
p=p->next;
}
if(p->next==h)
return false;
else
{
s=(LinkNode *)malloc(sizeof(LinkNode));
s->data=e;
s->next=p->next;
p->next=s;
return true;
}
}
//删除某个元素
bool ListDelete(LinkNode *&h,int i,ElemType &e)
{
int j=0;
LinkNode *p=h,*q;
if(i<=0) return false;
while(j<i-1&&p->next!=h)
{
j++;
p=p->next;
}
if(p->next==h)
return false;
else
{
q=p->next;
if(q==h)
return false;
e=q->data;
p->next=q->next;
free(q);
return true;
}
}
//销毁线性表
void DestroyList(LinkNode *&h)
{
LinkNode *pre=h,*p=h->next;
while(p!=h)
{
free(pre);
pre=p;
p=pre->next;
}
free(pre);
}
//主函数
int main()
{
LinkNode *h1;
cout<<"1.初始化循环单链表:"<<endl;InitList(h1);
cout<<"\n2.尾插法插入元素:"<<endl;
ElemType a[5]={'a','b','c','d','e'};
CreateListR(h1,a,5);
cout<<"\n3.循环单链表为:";
DispList(h1);
cout<<"\n4.循环单链表的长度:"<<ListLength(h1)<<endl;
cout<<"\n5.该循环链表";
if(ListEmpty(h1))
cout<<"为空"<<endl;
else
cout<<"不为空"<<endl;
cout<<"\n6.取元素"<<endl;
ElemType temp;cout<<"请输入取的位置:";int k;cin>>k;
if(GetElem(h1,k,temp))
cout<<"取值成功,该循环链表的第"<<k<<"个元素的值为:"<<temp<<endl;
else
cout<<"取值失败,你输入的位置"<<k<<"越界"<<endl;
cout<<"\n7.查找元素:"<<endl<<"请输入查找元素的值:";cin>>temp;
if(LocateElem(h1,temp))
cout<<"输出元素"<<temp<<"的位置为:"<<LocateElem(h1,temp);
else
cout<<"元素"<<temp<<"不存在";
cout<<"\n8.在循环链表指定位置插入元素:"<<endl;
cout<<"请输入插入的位置:";cin>>k;
cout<<"请输入插入元素的值:";cin>>temp;
if(ListInsert(h1,k,temp))
cout<<"插入成功"<<endl;
else
cout<<"插入失败"<<endl;
cout<<"\n9.输出循环链表"<<endl;
DispList(h1);
cout<<"\n10.删除循环链表指定位置的元素"<<endl;
cout<<"请输入删除的位置:";cin>>k;
if(ListDelete(h1,k,temp))
cout<<"删除成功,删除的元素为:"<<temp<<endl;
else
cout<<"删除失败"<<endl;
cout<<"\n11.输出循环链表"<<endl;
DispList(h1);
cout<<"\n12.释放循环链表"<<endl;
DestroyList(h1);
}
c++实现循环单链表的各种运算
于 2024-04-08 21:41:30 首次发布
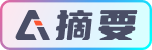