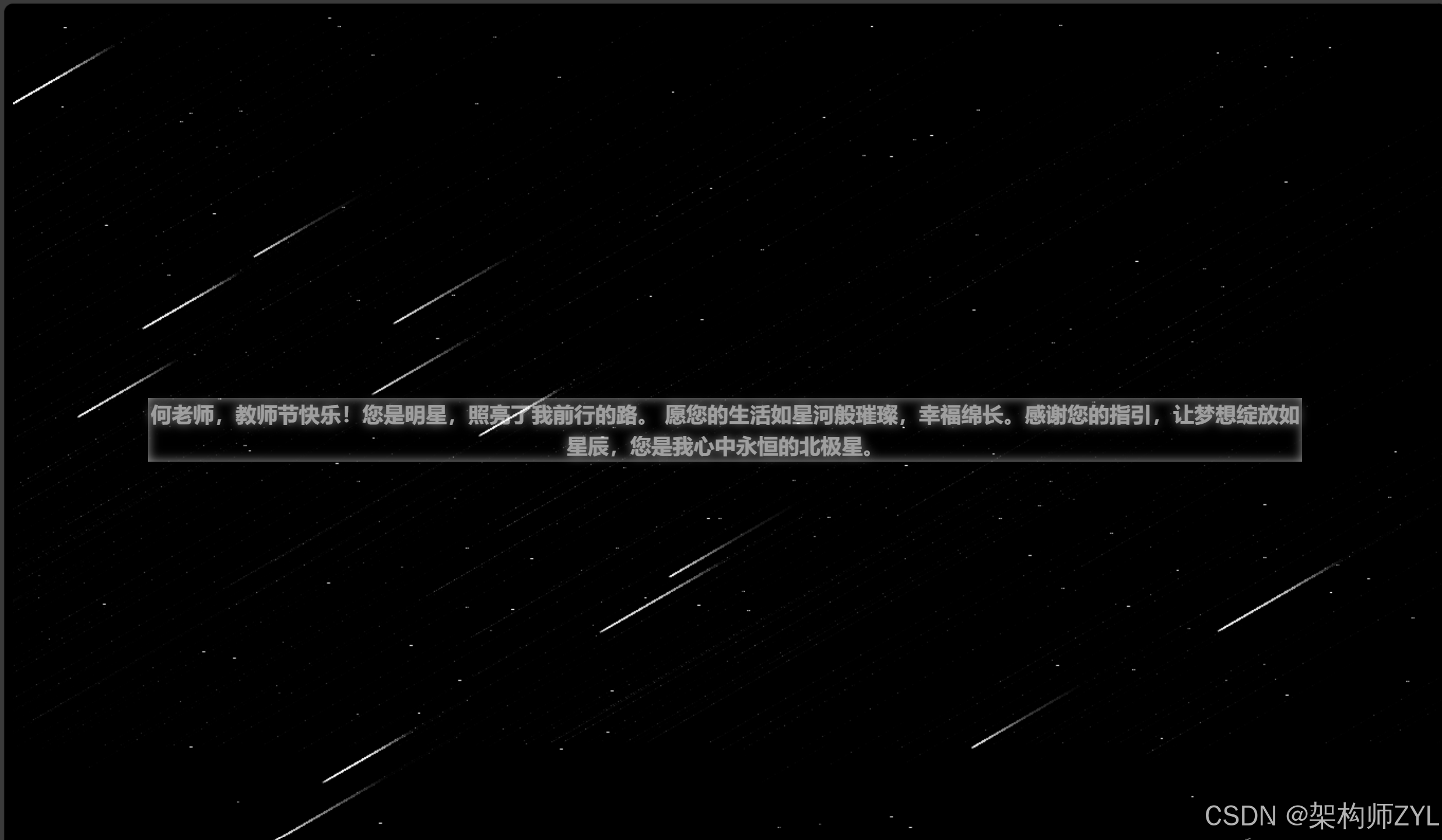
效果图
-
🚀HTML结构揭秘🚀:
-
📝<head>
中包含了元信息和样式表。
-
🖼️<body>
拥有一个<canvas>
元素与一个.text
类的<div>
,为星空与教师节信息搭建舞台。
-
🎨CSS魔法调色盘🎨:
-
🌑背景深邃的黑色,奠定星空基调。
-
✨.text
类让祝福语居中闪烁,加上阴影特效,确保在星光中夺目。
-
🤖JavaScript功能拆解🤖:
-
💡初始化:画布尺寸设定,获取绘图上下文。
-
🌠Star类:星点随机闪烁,颜色变换,绘制夜空的每一颗星。
-
💨MeteorRain类:流星雨的绚丽登场,从初始化到动态绘制,每一颗流星都有其独特轨迹。
-
🎭动画效果背后的逻辑🎭:
-
🌑星空:遍历星体数组,draw
方法重现浩瀚星河。
-
✨流星雨:动态更新位置与透明度,每一次移动都是一次视觉盛宴。
-
💡细节巧思💡:
-
🌟星体颜色随机变换,流星长度、速度、角度各异,造就独一无二的夜空景象。
-
🕰️定时器setTimeout
精心调校,星空与流星雨动画流畅不卡顿。
-
🌟用户体验升级🌟:
-
🌌动画平滑,祝福语清晰可读,星空下的信息传递温暖而有力。
-
🎧虽未在代码中体现,加入背景音乐或月亮相伴,定能让视觉盛宴更添几分感动。
代码如下
<!DOCTYPE html>
<html>
<head>
<meta charset="utf-8">
<title>教师节快乐</title>
<style type="text/css">
body {
background-color: black;
z-index: 1;
}
body, html {
width: 100%;
height: 100%;
overflow: hidden;
}
.text {
color: white;
font-size: 20px;
text-align: center;
position: absolute;
top: 50%;
left: 50%;
transform: translate(-50%, -50%);
z-index: 333;
font-family: "微软雅黑";
font-weight: bold;
line-height: 1.5;
width: 80%;
animation: blink 1s infinite;
animation-timing-function: linear;
animation-direction: alternate;
animation-iteration-count: infinite;
animation-delay: 0.5s;
animation-fill-mode: forwards;
animation-play-state: running;
box-shadow: inset 0 0 10px white;
text-shadow: 0 0 10px white;
font-size: 20px;
}
@keyframes blink {
0% {
opacity: 1;
}
50% {
opacity: 0;
}
100% {
opacity: 1;
}
}
</style>
</head>
<body>
<canvas id="stars"></canvas>
<div class="text">
何老师,教师节快乐!您是明星,照亮了我前行的路。
愿您的生活如星河般璀璨,幸福绵长。感谢您的指引,让梦想绽放如星辰,您是我心中永恒的北极星。
</div>
<script>
var context;
var arr = [];
var starCount = 800;
var rains = [];
var rainCount = 20;
function init() {
var stars = document.getElementById("stars");
windowWidth = window.innerWidth;
stars.width = windowWidth;
stars.height = window.innerHeight;
context = stars.getContext("2d");
}
var Star = function () {
this.x = windowWidth * Math.random();
this.y = 5000 * Math.random();
this.text = ".";
this.color = "white";
this.getColor = function () {
var _r = Math.random();
if (_r < 0.5) {
this.color = "#333";
} else {
this.color = "white";
}
}
this.init = function () {
this.getColor();
}
this.draw = function () {
context.fillStyle = this.color;
context.fillText(this.text, this.x, this.y);
}
}
function drawMoon() {
var moon = new Image();
moon.src = "./images/moon.jpg";
moon.onload = function() {
context.drawImage(moon, -5, -10);
}
}
var MeteorRain = function () {
this.x = -1;
this.y = -1;
this.length = -1;
this.angle = 30;
this.width = -1;
this.height = -1;
this.speed = 1;
this.offset_x = -1;
this.offset_y = -1;
this.alpha = 1;
this.color1 = "";
this.color2 = "";
this.init = function () {
this.getPos();
this.alpha = 1;
this.getRandomColor();
var x = Math.random() * 80 + 150;
this.length = Math.ceil(x);
this.angle = 30;
x = Math.random() + 0.5;
this.speed = Math.ceil(x);
var cos = Math.cos(this.angle * Math.PI / 180);
var sin = Math.sin(this.angle * Math.PI / 180);
this.width = this.length * cos;
this.height = this.length * sin;
this.offset_x = this.speed * cos;
this.offset_y = this.speed * sin;
}
this.getRandomColor = function () {
var a = Math.ceil(255 - 240 * Math.random());
this.color1 = "rgba(" + a + "," + a + "," + a + ",1)";
this.color2 = "black";
}
this.countPos = function () {
this.x = this.x - this.offset_x;
this.y = this.y + this.offset_y;
}
this.getPos = function () {
this.x = Math.random() * window.innerWidth;
this.y = Math.random() * window.innerHeight;
}
this.draw = function () {
context.save();
context.beginPath();
context.lineWidth = 1;
context.globalAlpha = this.alpha;
var line = context.createLinearGradient(this.x, this.y, this.x + this.width, this.y - this.height);
line.addColorStop(0, "white");
line.addColorStop(0.3, this.color1);
line.addColorStop(0.6, this.color2);
context.strokeStyle = line;
context.moveTo(this.x, this.y);
context.lineTo(this.x + this.width, this.y - this.height);
context.closePath();
context.stroke();
context.restore();
}
this.move = function () {
context.clearRect(this.x + this.width - this.offset_x, this.y - this.height, this.offset_x + 5, this.offset_y + 5);
this.countPos();
this.alpha -= 0.002;
this.draw();
}
}
window.onload = function () {
init();
for (var i = 0; i < starCount; i++) {
var star = new Star();
star.init();
star.draw();
arr.push(star);
}
for (var i = 0; i < rainCount; i++) {
var rain = new MeteorRain();
rain.init();
rain.draw();
rains.push(rain);
}
drawMoon();
playStars();
playRains();
}
function playStars() {
for (var n = 0; n < starCount; n++) {
arr[n].getColor();
arr[n].draw();
}
setTimeout(playStars, 100);
}
function playRains() {
for (var n = 0; n < rainCount; n++) {
var rain = rains[n];
rain.move();
if (rain.y > window.innerHeight) {
context.clearRect(rain.x, rain.y - rain.height, rain.width, rain.height);
rains[n] = new MeteorRain();
rains[n].init();
}
}
setTimeout(playRains, 2);
}
</script>
</body>
</html>