queue.append(initial_state) # 将初始状态加入队列
visited.add(tuple(initial_state)) # 将初始状态转换为元组并加入已访问集合
parent[tuple(initial_state)] = None # 初始状态没有父状态,设为None
while queue:
current_state = queue.popleft() # 取出队列中的第一个状态作为当前状态
if current_state == goal_state:
# 找到了目标状态,回溯路径
path = []
while current_state:
path.append(current_state)
current_state = parent[tuple(current_state)]
path.reverse()
return path
next_states = generate_next_states(current_state) # 生成下一步可能的状态
for next_state in next_states:
if tuple(next_state) not in visited:
queue.append(next_state)
visited.add(tuple(next_state))
parent[tuple(next_state)] = current_state # 记录下一步状态的父状态为当前状态
return None # 如果队列为空仍然没有找到目标状态,表示无解
def generate_next_states(state):
next_states = []
blank_index = state.index(0) # 找到空白方块的索引
adjacent_indices = get_adjacent_indices(blank_index) # 获取可以移动的方块的索引
for index in adjacent_indices:
new_state = list(state) # 创建当前状态的副本
new_state[blank_index] = new_state[index] # 将空白方块与相邻方块交换
new_state[index] = 0
next_states.append(new_state) # 添加生成的新状态到下一步状态列表
return next_states
def get_adjacent_indices(index):
adjacent_indices = []
if index // 3 > 0:
adjacent_indices.append(index - 3) # 上方方块
if index // 3 < 2:
adjacent_indices.append(index + 3) # 下方方块
if index % 3 > 0:
adjacent_indices.append(index - 1) # 左侧方块
if index % 3 < 2:
adjacent_indices.append(index + 1) # 右侧方块
return adjacent_indices
def print_state(state):
for i in range(0, 9, 3):
print(state[i:i + 3])
if i == 6:
print()
测试示例
initial_state = [2, 8, 3, 1, 0, 4, 7, 6, 5]
goal_state = [1, 2, 3, 8, 0, 4, 7, 6, 5]
path = breadth_first_search(initial_state, goal_state)
if path:
print(“找到了解决方案:”)
for state in path:
print_state(state)
else:
print(“无解”)
运行结果:
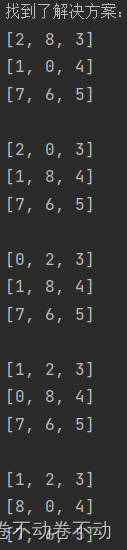
一些关键的心得体会:
1. 状态空间的组织:八数码问题可以看作是一个状态空间搜索问题,其中每个状态都是一个具有不同数字排列的八数码板。在实现BFS时,我发现合理组织和表示状态空间是非常重要的。在代码示例中,我使用了列表来表示状态,通过数字的排列和空白方块的位置来表示不同的状态。
2. 队列的作用:BFS使用队列来存储待扩展的状态,并按照先进先出的顺序进行状态扩展。队列的特性确保我们先扩展当前层的状态,然后才能扩展下一层的状态。这种按层级扩展的方式确保我们能够找到最短路径。
**自我介绍一下,小编13年上海交大毕业,曾经在小公司待过,也去过华为、OPPO等大厂,18年进入阿里一直到现在。**
**深知大多数Python工程师,想要提升技能,往往是自己摸索成长或者是报班学习,但对于培训机构动则几千的学费,着实压力不小。自己不成体系的自学效果低效又漫长,而且极易碰到天花板技术停滞不前!**
**因此收集整理了一份《2024年Python开发全套学习资料》,初衷也很简单,就是希望能够帮助到想自学提升又不知道该从何学起的朋友,同时减轻大家的负担。**
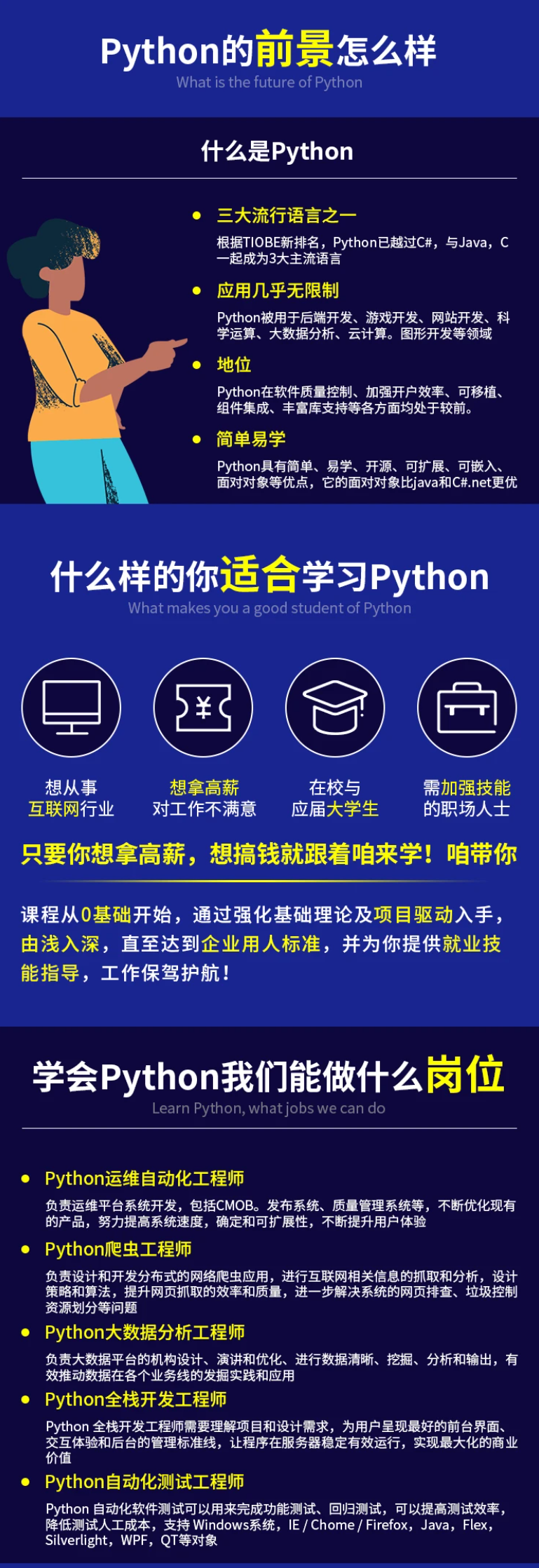
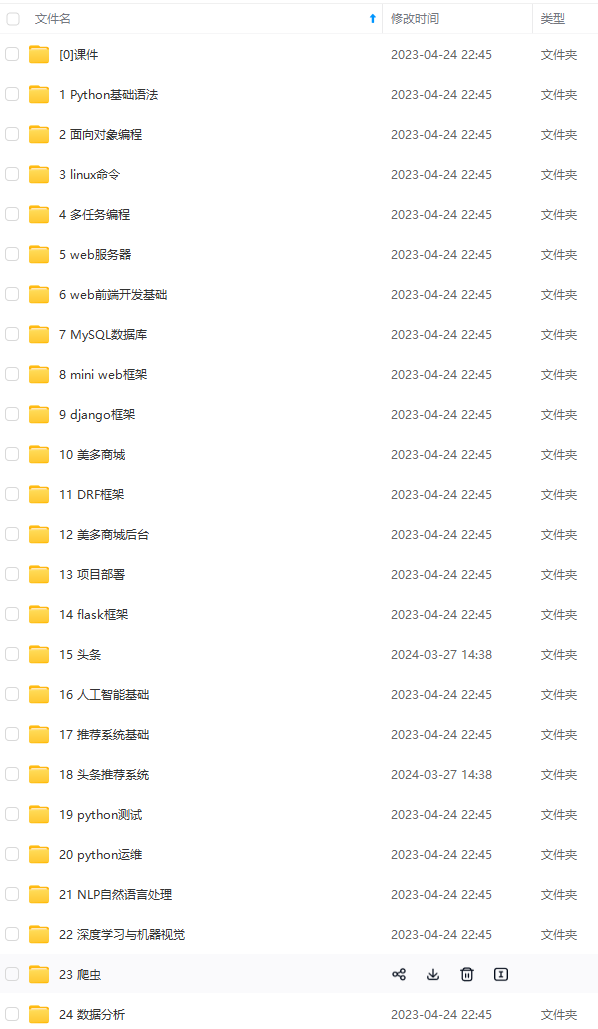
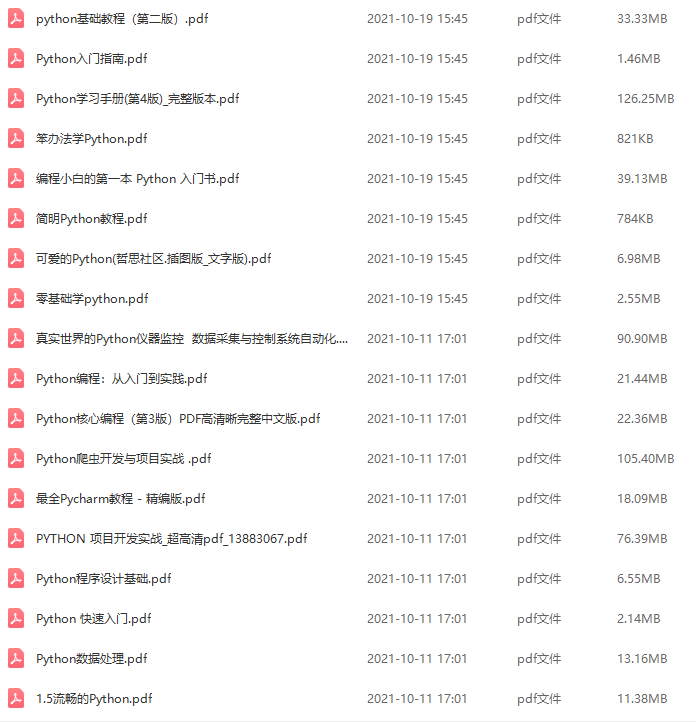
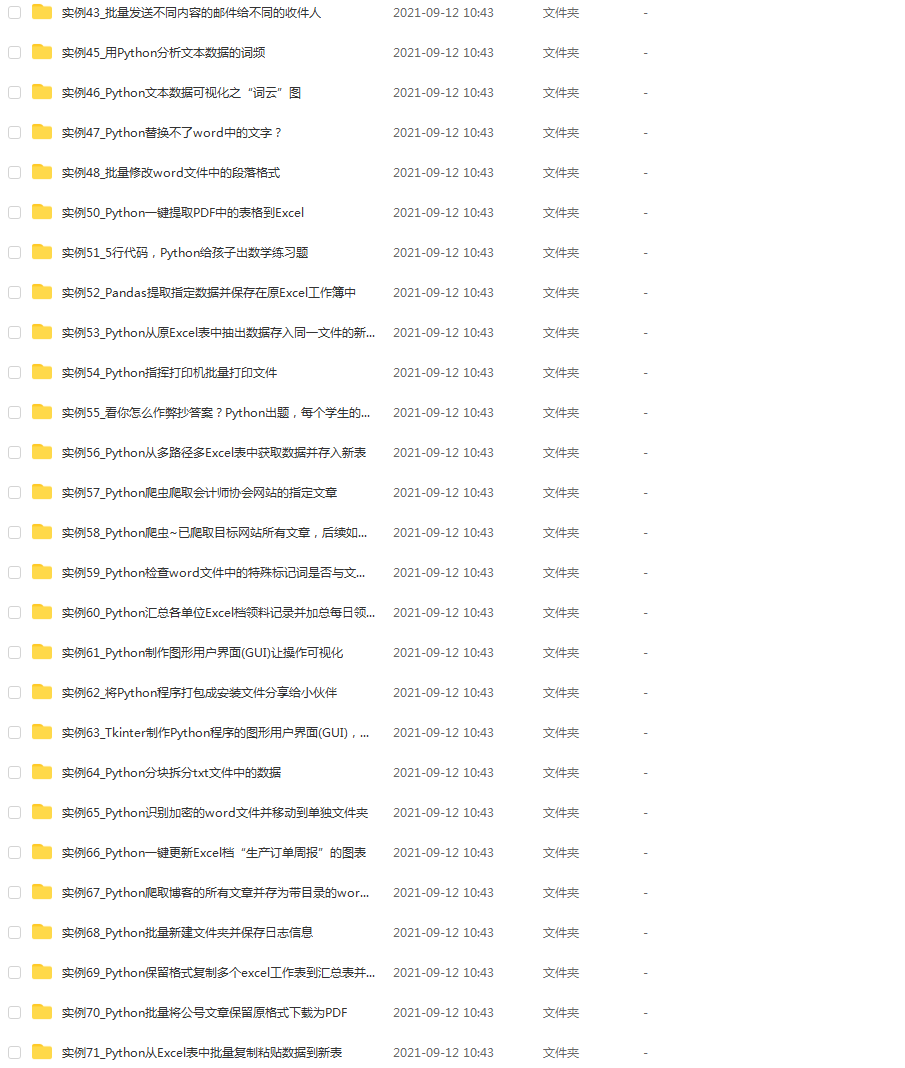
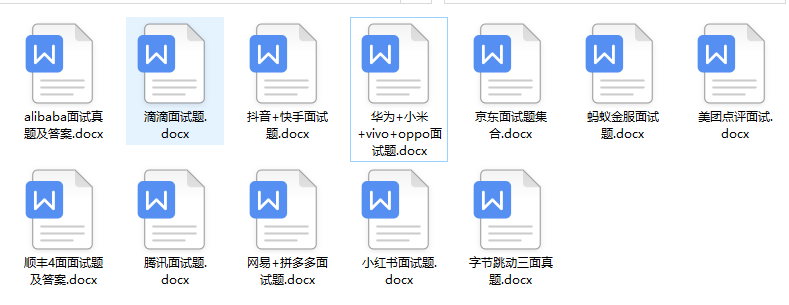
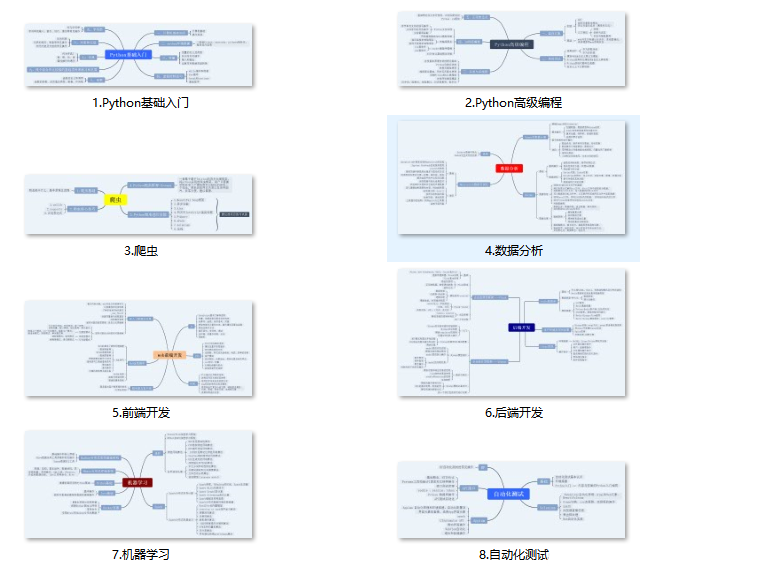
**既有适合小白学习的零基础资料,也有适合3年以上经验的小伙伴深入学习提升的进阶课程,基本涵盖了95%以上前端开发知识点,真正体系化!**
**由于文件比较大,这里只是将部分目录大纲截图出来,每个节点里面都包含大厂面经、学习笔记、源码讲义、实战项目、讲解视频,并且后续会持续更新**
**如果你觉得这些内容对你有帮助,可以扫码获取!!!(备注Python)**
**由于文件比较大,这里只是将部分目录大纲截图出来,每个节点里面都包含大厂面经、学习笔记、源码讲义、实战项目、讲解视频,并且后续会持续更新**
**如果你觉得这些内容对你有帮助,可以扫码获取!!!(备注Python)**
<img src="https://img-community.csdnimg.cn/images/fd6ebf0d450a4dbea7428752dc7ffd34.jpg" alt="img" style="zoom:50%;" />