目录
2. 用 compare 和 compareToIgnoreCase 方法进行字符串比较
3. int indexOf(int ch, int fromIndex)
4. int indexOf(String str) 和 int indexOf(String str, int fromIndex)
5. int lastIndexOf(int ch) 和 int lastIndexOf(int ch, int fromIndex)
前言
在C语言中我们想要使用字符串就要用到数组或指针,但是这种将数据和操作数据方法分离的方式不符合面向对象的思想,且对字符串应用又非常广泛,所以JAVA中就有了String类。因为关于引用数据类型大家前面也都用过了,对 String 也已经非常熟悉,这一节就 String 类深入认识一下。
一、常用方法
1.1 字符串构造
String 提供的构造方法常用以下三类:
1. 直接赋值
String str1 = "hello";
System.out.println(str1);
2. new一个 String 对象
String str2 = new String("world");
System.out.println(str2);
3. 使用字符串数组
char[] array = {'a','b','c'};
String str3 = new String();
System.out.println(str3);
注意: String 是引用数据类型,内部并不存储字符串本身。
public static void main2(String[] args) {
String str1 = new String("hello");
String str2 = new String("world");
String str3 = str1;
System.out.println(str1);
System.out.println(str2);
System.out.println(str3);
}
这串代码 str1 和 str2 引用的不是同一个对象,但 str1 和 str3 引用的是同一个对象。
public static void main2(String[] args) {
String str4 = "";
String str5 = null;
System.out.println(str4.length());
System.out.println(str4.isEmpty());//判断字符串是否为空
//System.out.println(str5.length());
System.out.println(str5.isEmpty());
}
这段代码代表 str4 指向一个空字符串,但 str5 不指向任何字符串,所以 str4 是有长度的,长度为 0,判断字符串是否为空方法输出 true 。str5 因为不指向任何对象,所以他既没长度,也无法判断是否为空,两个方法都会报错。
1.2 String 对象的比较
字符串的比较是我们经常要用到的操作,比如字符串排序等等。比较的几种方式:
1.== 比较是否引用同一个对象
注意:对于基本数据类型, == 比较的是两边的值;对于引用数据类型,== 比较的是引用的是否为同一个地址。
public static void main(String[] args) {
int a = 1;
int b = 2;
int c = 1;
System.out.println(a == b);
System.out.println(a == c);
}
运行结果:
值一样,输出 true , 值不一样,输出 false 。
public static void main(String[] args) {
String s1 = new String("hello");
String s2 = new String("hello");
System.out.println(s1 == s2);
}
运行结果:
我们发现,虽然 s1 和 s2 给的字符串一样,但输出的却是 false 。因为 s2 自己 new 了一个新的对象,s1 和 s2 指向的地址是不一样的,所以会输出 false 。怎样让他们仅仅比较字符串是否一样呢,可以用到 equals 方法:
public static void main(String[] args) {
String s1 = new String("hello");
String s2 = new String("hello");
//System.out.println(s1 == s2);
System.out.println(s1.equals(s2));//true
}
2. 用 compare 和 compareToIgnoreCase 方法进行字符串比较
也可以用到 compare 和 compareToIgnoreCase 方法,如果 s1 大于 s2 ,返回值是正数;s1 等于 s2 ,返回 0 ;s1 小于 s2 ,返回值是负数。这两个的区别是:是否区分字符串大小写,compare 区分大小写, compareToIgnoreCase 不区分大小写。
public static void main(String[] args) {
String s1 = new String("hello");
String s2 = new String("Hello");
/**
* 比较字符串大小
* 如果 s1 > s2 ,返回正数
* 如果 s1 == s2 ,返回0
* 如果 s1 < s2 ,返回负数
*/
System.out.println(s1.compareTo(s2));
//忽略大小写比较大小
System.out.println(s1.compareToIgnoreCase(s2));
}
运行结果:
在 compare 方法中,s1大于 s2,返回的数取决于比较字符的ACSII码值, compareToIgnoreCase 方法中,s1 等于 s2。
1.3字符串的查找
方法 | 功能 |
char charArt(int index) |
返回
index
位置上字符,如果
index
为负数或者越界,抛出IndexOutOfBoundsException异常
|
int indexOf(int ch) | 返回ch第一次出现的位置,没有返回-1 |
int indexOf(int ch, int
fromIndex)
| 从fromIndex位置开始找ch第一次出现的位置,没有返回-1 |
int indexOf(String str) | 返回str第一次出现的位置,没有返回-1 |
int indexOf(String str, int fromIndex)
| 从fromIndex位置开始找str第一次出现的位置,没有返回-1 |
int lastIndexOf(int ch) | 从后往前找,返回ch第一次出现的位置,没有返回-1 |
int lastIndexOf(int ch, int
fromIndex)
|
从
fromIndex
位置开始找,从后往前找
ch
第一次出现的位置,没有返回-1
|
int lastIndexOf(String str) | 从后往前找,返回str第一次出现的位置,没有返回-1 |
int lastIndexOf(String str, int fromIndex)
|
从
fromIndex
位置开始找,从后往前找
str
第一次出现的位置,没有返回-1
|
1. char charArt()
public static void main(String[] args) {
String str1 = "hello";
for (int i = 0;i < str1.length();i++) {
char ch = str1.charAt(i);
System.out.println(ch);
}
}
但注意字符下标不要为负数或者越界,要不然会报错。
2. int indexOf(int ch)
char charArt 方法根据下标查找字符 , int indexOf( ) 则是根据字符输出下标。
注意:是从头到尾查找字符,然后输出那个字符第一次出现的下标。
public static void main(String[] args) {
//根据字符输出下标
String str2 = "world";
int index = str2.indexOf('w');
System.out.println(index); //0
}
3. int indexOf(int ch, int fromIndex)
从指定位置查找字符,然后输出那个字符第一次出现的下标。
public static void main(String[] args) {
//从指定位置,根据字符输出下标
String str2 = "hello";
int index = str2.indexOf('l',3);
System.out.println(index); //3
}
4. int indexOf(String str) 和 int indexOf(String str, int fromIndex)
和上述两个方法同理,但是是根据字符串查找下标和指定位置查找字符串下标。
public static void main(String[] args) {
String str3 = "aaaabcabc";
int index1 = str3.indexOf("abc");
int index2 = str3.indexOf("abc",4);
System.out.println(index1); //3
System.out.println(index2); //6
}
5. int lastIndexOf(int ch) 和 int lastIndexOf(int ch, int fromIndex)
和上边两个方法大同小异,只不过是倒着,从后往前找。根据字符串反向查找下标和从指定位置反向查找字符串下标。
public static void main(String[] args) {
String str4 = "aaaabcabc";
int index3 = str4.lastIndexOf("abc");
int index4 = str4.lastIndexOf("abc",4);
System.out.println(index3); //6
System.out.println(index4); //3
}
1.4 转化
1. 字符串和数字的转化
public static void main(String[] args) {
//字符串转化成数字
String s1 = String.valueOf(19.9);
String s2 = String.valueOf(199);
System.out.println(s1); // 19.9
System.out.println(s2); // 199
//数字转化成字符串
int data1 = Integer.parseInt("199");
double data2 = Double.parseDouble("19.9");
System.out.println(data1); //199
System.out.println(data2); //19.9
}
2. 大小写之间的互相转换
public static void main(String[] args) {
//小写转换为大写
String s1 = "hello";
String ret = s1.toUpperCase();
System.out.println(ret);//HELLO
//大写转换为小写
String s2 = "HEllo";
String ret2 = s2.toLowerCase();
System.out.println(ret2);//hello
}
要注意的是:大小写转换并不是在原来的基础上转换,而是创建了一个新的对象,通过新的对象来接收。
3. 字符串与数组之间的转换
public static void main(String[] args) {
//字符串转换为数组
String s1 = "hello";
char[] array = s1.toCharArray();
System.out.println(Arrays.toString(array));//[h, e, l, l, o]
//数组转换为字符串
char[] array2 = {'a','b','c'};
String s2 = new String(array2);
System.out.println(s2);//abc
}
要注意的是:字符串转化为数组时要用 char[ ] 来接收,输出时可以用 for循环遍历输出,也可以调用toString 方法输出 ,不能直接用数组名输出!
4. 格式化
public static void main(String[] args) {
String s3 = String.format("%d-%d-%d",2023,8,13);
System.out.println(s3);//2023-8-13
}
1.5 字符串的替换
public static void main(String[] args) {
String s1 = "abcsssabcdef";
String ret = s1.replace("abc","bb");
System.out.println(ret);
ret = s1.replace('a','b');
System.out.println(ret);
ret = s1.replaceFirst("abc","wa");
System.out.println(ret);
ret = s1.replaceAll("abc","za");
System.out.println(ret);
}
有三个方法:replace , replaceFirst , replaceAll 。
replace 可以实现 char 类型字符的替换,但因为实现接口的关系,String 类型字符串替换也可以实现。replaceFirst 可以实现String 类型的字符串第一次出现时的替换。replace 可以实现 String 类型的全部字符串实现,但不能实现 char 类型。
1.6 字符串的拆分
public static void main(String[] args) {
String str = "hello abc world";
//空格分隔,但不分组
String[] s1 = str.split(" ");
for (int i = 0; i < s1.length; i++) {
System.out.println(s1[i]);
}
//空格分割 ,分组
s1 = str.split(" ",2);
for (int i = 0; i < s1.length; i++) {
System.out.println(s1[i]);
}
}
注意:分割字符串时,要创建一个新的字符串数组类型的变量来接收。在 split 方法中,定义了分割后如果输入一个整数类型的参数,则会部分分割。
但也会存在一些特殊情况:比如拆分ip地址
public static void main(String[] args) {
String s1 = "192.168.1.1";
String[] str1 = s1.split("\\.");
for (int i = 0;i < str1.length;i++) {
System.out.println(str1[i]);
}
}
我们本想用 . 进行分割,但只输入一个点,他会分割失败,需要前边加上两个转义字符\\。
注意:
1. 字符" | ", " * " , " + "都得加上转义字符,前面加上 "\\" .
2. 而如果是 "\" ,那么就得写成 "\\\\" .
3. 如果一个字符串中有多个分隔符,可以用"|"作为连字符。
public static void main(String[] args) {
String s1 = "name=zhangsan&age=20";
String[] str1 = s1.split("=|&");
for (int i = 0;i < str1.length;i++) {
System.out.println(str1[i]);
}
}
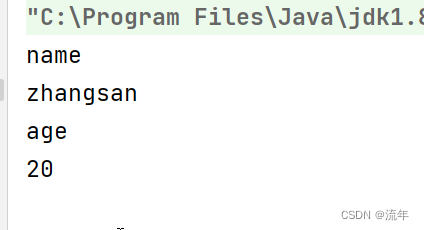
同一个例子也可以采用多次分割的方法:
public static void main(String[] args) {
String s1 = "name=zhangsan&age=20";
String[] str1 = s1.split("&");
for (int i = 0; i < str1.length; i++) {
String x = str1[i];
String[] str2 = x.split("=");
for (int j = 0; j < str2.length; j++) {
System.out.println(str2[j]);
}
}
}
1.7 字符串的截取
public static void main(String[] args) {
String str1 = "abcdef";
String ret = str1.substring(0,3);//左闭右开 [ )
System.out.println(ret); //abc
ret = str1.substring(2);
System.out.println(ret);//cdef
}
注意:当在 substring 方法中给到0 和 3 两个参数,代表截取下标从 0 到 2 的元素,不包括下标是3的元素,因为 0 - 3 是个左闭右开的区间。如果只有一个参数,代表从下标为2的元素开始截取。
1.8 其他字符串操作
public static void main(String[] args) {
String str1 = " abc def ";
System.out.println(str1);
System.out.println(str1.trim());
}
trim 方法可以消除字符串左右两边的空格,但无法消除中间空格。
二、总结
这一节讲了特别多的方法,在这里对出现在这节的方法做一个统一的整理:
方法 | 功能 |
lenght() | 字符串的长度方法 |
isEmpty() | 判断字符串是否为空方法 |
equals() | 字符串的比较方法,返回值为Boolean类型 |
compare() | 字符串的比较方法,不能忽略大小写 |
compareTolgnoreCase() | 字符串的比较方法,忽略大小写 |
toUpperCase() | 字符串小写替换成大写方法 |
toLowerCase() | 字符串大写替换成小写方法 |
toCharArray() | 字符串转化成数组方法 |
format() | 字符串格式化方法 |
replace() | 字符串的替换方法,可char 可 String |
replaceFirst() | 替换第一次出现的字符串,必须 String |
replaceAll() | 替换全部的字符串,必须 String |
split() | 字符串的分割方法 |
substring() | 字符串的截取方法 |
trim() | 去掉字符串左右两边的空格,中间的无法去掉 |
关于字符串的查找方法看上表。