package com.ynavc.Controller;
import java.sql.ResultSet;
import java.sql.SQLException;
import java.util.ArrayList;
import com.ynavc.Bean.Goods;
import com.ynavc.Dao.DbConnection;
public class Select {
public static Object[][] getGoods(String sql) {
ResultSet resultSet = DbConnection.query(sql);
ArrayList list=new ArrayList();
try {
while (resultSet.next()) {
Goods goods=new Goods();
goods.setGoodsID(resultSet.getInt(1));
goods.setGoodsName(resultSet.getString(2));
goods.setNum(resultSet.getInt(3));
goods.setPrice(resultSet.getString(4));
list.add(goods);
}
} catch (SQLException e) {
e.printStackTrace();
}
Object[][] objects=new Object[list.size()][4];
for(int i=0;i<list.size();i++) {
objects[i][0]=list.get(i).getGoodsID();
objects[i][1]=list.get(i).getGoodsName();
objects[i][2]=list.get(i).getNum();
objects[i][3]=list.get(i).getPrice();
}
return objects;
}
}
Updata.Java
package com.ynavc.Controller;
import com.ynavc.Dao.DbConnection;
public class Updata {
//添加数据
public static int addData(String sql) {
return DbConnection.updataInfo(sql);
}
}
com.ynavc.Dao
DbConnection .Java
package com.ynavc.Dao;
import java.sql.Connection;
import java.sql.DriverManager;
import java.sql.PreparedStatement;
import java.sql.ResultSet;
import java.sql.SQLException;
import javax.swing.JOptionPane;
import com.mysql.jdbc.Statement;
public class DbConnection {
//驱动类的类名
private static final String DRIVERNAME=“com.mysql.jdbc.Driver”;
//连接数据的URL路径
// private static final String URL=“jdbc:mysql://118.31.124.77:3306/mydb23660”;
private static final String URL=“jdbc:mysql://127.0.0.1:3306/dbgoods”;
//数据库登录账号
// private static final String USER=“mydb23660”;
private static final String USER=“root”;
//数据库登录密码
// private static final String PASSWORD=“Hmsyfjdglxt66”;
private static final String PASSWORD=“root”;
//加载驱动
static{
try {
Class.forName(DRIVERNAME);
} catch (ClassNotFoundException e) {
e.printStackTrace();
}
}
//获取数据库连接
public static Connection getConnection() {
try {
return DriverManager.getConnection(URL,USER,PASSWORD);
} catch (SQLException e) {
e.printStackTrace();
}
return null;
}
//查询
public static ResultSet query(String sql) {
System.out.println(sql);
//获取连接
Connection connection=getConnection();
PreparedStatement psd;
try {
psd = connection.prepareStatement(sql);
return psd.executeQuery();
} catch (SQLException e) {
JOptionPane.showMessageDialog(null,“执行语句出错\n”+e.toString());
e.printStackTrace();
}
return null;
}
//增、删、改、查
public static int updataInfo(String sql) {
System.out.println(sql);
//获取连接
Connection connection=getConnection();
try {
PreparedStatement psd=connection.prepareStatement(sql);
return psd.executeUpdate();
} catch (SQLException e) {
JOptionPane.showMessageDialog(null,“执行语句出错\n”+e.toString());
e.printStackTrace();
}
return 0;
}
//关闭连接
public static void colse(ResultSet rs,Statement stmt,Connection conn) throws Exception{
try { if (rs != null){ rs.close(); }
if (stmt != null) { stmt.cancel(); }
if (conn != null) { conn.close(); }
} catch (Exception e) {
e.printStackTrace(); throw new Exception();
}
}
}
com.ynavc.Test
Main.Java
package com.ynavc.Test;
import com.ynavc.Vive.GoodsManagement;
public class Main {
public static void main(String[] args) {
GoodsManagement t = new GoodsManagement();
t.setVisible(true);
}
}
com.ynavc.Vive
GoodsManagement.Java
package com.ynavc.Vive;
import javax.swing.JFrame;
import javax.swing.JLabel;
import javax.swing.JScrollPane;
import javax.swing.JTable;
import javax.swing.JTextField;
import javax.swing.ScrollPaneConstants;
import javax.swing.table.DefaultTableModel;
import com.ynavc.Controller.Select;
import javax.swing.JButton;
import java.awt.event.ActionListener;
import java.awt.event.ActionEvent;
public class GoodsManagement extends JFrame {
Select select = new Select();
private JTextField textField;
Object[] header= {“商品编号”,“商品名称”,“数量”,“单价”};
String sql = “SELECT goodsID,goodsname,num,price FROM goods”;
Object[][] data= select.getGoods(sql);
DefaultTableModel df = new DefaultTableModel(data, header);
int v=ScrollPaneConstants.VERTICAL_SCROLLBAR_AS_NEEDED;
int h=ScrollPaneConstants.HORIZONTAL_SCROLLBAR_AS_NEEDED;
public GoodsManagement() {
super(“商品管理系统”);
this.setBounds(0, 0, 700, 450);
this.setLocationRelativeTo(null);//让窗口在屏幕中间显示
this.setResizable(false);//让窗口大小不可改变
this.setDefaultCloseOperation(JFrame.EXIT_ON_CLOSE);//用户单击窗口的关闭按钮时程序执行的操作
getContentPane().setLayout(null);
JLabel label = new JLabel(“请输入商品名称:”);
label.setBounds(151, 39, 112, 32);
getContentPane().add(label);
textField = new JTextField();
textField.setBounds(263, 43, 127, 26);
getContentPane().add(textField);
textField.setColumns(10);
JButton button = new JButton(“查询”);
button.addActionListener(new ActionListener() {
public void actionPerformed(ActionEvent arg0) {
String sql = “SELECT goodsID,goodsname,num,price FROM goods WHERE goodsname LIKE '%”+textField.getText()+“%'”;
Object[][] data = Select.getGoods(sql);
df.setDataVector(data, header);
}
});
button.setBounds(411, 40, 90, 30);
getContentPane().add(button);
JButton button_1 = new JButton(“添加”);
button_1.setBounds(559, 140, 90, 30);
getContentPane().add(button_1);
button_1.addActionListener(new ActionListener() {
public void actionPerformed(ActionEvent arg0) {
GoodsManage t = new GoodsManage();
t.setVisible(true);
dispose();
}
});
JTable jTable = new JTable(df);
JScrollPane jsp=new JScrollPane(jTable,v,h);
jsp.setBounds(44, 103, 480, 282);
getContentPane().add(jsp);
}
public static void main(String[] args) {
GoodsManagement t = new GoodsManagement();
t.setVisible(true);
}
}
GoodsManage.Java
package com.ynavc.Vive;
import javax.swing.JFrame;
import javax.swing.JLabel;
import javax.swing.JOptionPane;
import javax.swing.JScrollPane;
import javax.swing.JTable;
import javax.swing.JTextField;
import javax.swing.ScrollPaneConstants;
import javax.swing.table.DefaultTableModel;
import com.ynavc.Bean.Goods;
import com.ynavc.Controller.Select;
import com.ynavc.Controller.Updata;
import javax.swing.JButton;
import java.awt.event.ActionListener;
import java.awt.event.WindowAdapter;
import java.awt.event.WindowEvent;
import java.awt.event.ActionEvent;
public class GoodsManage extends JFrame {
private JTextField textField;
Select select = new Select();
Updata updata = new Updata();
Object[] header= {“商品编号”,“商品名称”,“数量”,“单价”};
String sql = “SELECT goodsID,goodsname,num,price FROM goods”;
Object[][] data= select.getGoods(sql);
DefaultTableModel df = new DefaultTableModel(data, header);
int v=ScrollPaneConstants.VERTICAL_SCROLLBAR_AS_NEEDED;
int h=ScrollPaneConstants.HORIZONTAL_SCROLLBAR_AS_NEEDED;
public GoodsManage() {
super(“商品管理系统”);
this.setBounds(0, 0, 700, 450);
this.setLocationRelativeTo(null);//让窗口在屏幕中间显示
this.setResizable(false);//让窗口大小不可改变
getContentPane().setLayout(null);
JTable jTable = new JTable(df);
JScrollPane jsp=new JScrollPane(jTable,v,h);
jsp.setBounds(10, 10, 515, 320);
getContentPane().add(jsp);
JButton button_1 = new JButton(“显示所有商品”);
button_1.addActionListener(new ActionListener() {
@Override
public void actionPerformed(ActionEvent e) {
String sql = “SELECT goodsID,goodsname,num,price FROM goods”;
Object[][] data = Select.getGoods(sql);
df.setDataVector(data, header);
}
});
button_1.setBounds(535, 80, 127, 30);
getContentPane().add(button_1);
JButton button_2 = new JButton(“修改商品”);
button_2.setBounds(535, 140, 127, 30);
getContentPane().add(button_2);
button_2.addActionListener(new ActionListener() {
@Override
public void actionPerformed(ActionEvent e) {
if (jTable.getSelectedColumn()<0) {
JOptionPane.showMessageDialog(null, “请选择要修改的数据!”);
} else {
int goodsID = Integer.parseInt(jTable.getValueAt(jTable.getSelectedRow(), 0).toString());
String name = jTable.getValueAt(jTable.getSelectedRow(), 1).toString();
int num = Integer.parseInt(jTable.getValueAt(jTable.getSelectedRow(), 2).toString());
String price = jTable.getValueAt(jTable.getSelectedRow(), 3).toString();
Goods goods = new Goods(goodsID,name,num,price);
GoodsXG goodsXG = new GoodsXG(goods);
goodsXG.setVisible(true);
}
}
});
JButton button_3 = new JButton(“删除商品”);
button_3.setBounds(535, 200, 127, 30);
getContentPane().add(button_3);
button_3.addActionListener(new ActionListener() {
@Override
public void actionPerformed(ActionEvent e) {
if (jTable.getSelectedColumn()<0) {
JOptionPane.showMessageDialog(null, “请选中要删除的数据!”);
} else {
int goodsID = Integer.parseInt(jTable.getValueAt(jTable.getSelectedRow(), 0).toString());
String sql=“delete from goods where goodsid=”+goodsID;
int result = updata.addData(sql);
if (result>0) {
JOptionPane.showMessageDialog(null, “删除成功!”);
JOptionPane.showMessageDialog(null, “记得刷新一下哦!”);
} else {
JOptionPane.showMessageDialog(null, “删除失败!”);
}
}
}
});
JButton button_4 = new JButton(“添加商品”);
button_4.setBounds(535, 258, 127, 30);
getContentPane().add(button_4);
button_4.addActionListener(new ActionListener() {
public void actionPerformed(ActionEvent arg0) {
GoodsADD goodsAdd = new GoodsADD();
goodsAdd.setVisible(true);
}
});
JLabel label = new JLabel(“商品编号:”);
label.setBounds(40, 354, 112, 32);
getContentPane().add(label);
textField = new JTextField();
textField.setBounds(154, 358, 127, 26);
getContentPane().add(textField);
textField.setColumns(10);
JButton button = new JButton(“按编号查询”);
button.addActionListener(new ActionListener() {
public void actionPerformed(ActionEvent arg0) {
String sql = “SELECT goodsID,goodsname,num,price FROM goods WHERE goodsid LIKE '%”+textField.getText()+“%'”;
Object[][] data = Select.getGoods(sql);
df.setDataVector(data, header);
}
});
button.setBounds(305, 355, 112, 30);
getContentPane().add(button);
this.addWindowListener(new WindowAdapter() {
public void windowClosing(WindowEvent e) {
super.windowClosing(e);
//加入动作
GoodsManagement m = new GoodsManagement();
m.setVisible(true);
}
});
}
public static void main(String[] args) {
GoodsManage t = new GoodsManage();
t.setVisible(true);
}
}
GoodsXG.Java
package com.ynavc.Vive;
import javax.swing.JFrame;
import javax.swing.JLabel;
import javax.swing.JOptionPane;
import javax.swing.JTextField;
import com.ynavc.Bean.Goods;
import com.ynavc.Controller.Updata;
import java.awt.event.ActionEvent;
import java.awt.event.ActionListener;
import javax.swing.JButton;
public class GoodsXG extends JFrame {
private JTextField id,name,num,price;
private JButton button;
private JButton button_1;
int goodsid;
public GoodsXG(Goods goods) {
super(“商品管理系统”);
this.setBounds(0, 0, 400, 450);
this.setLocationRelativeTo(null);//让窗口在屏幕中间显示
this.setResizable(false);//让窗口大小不可改变
getContentPane().setLayout(null);
JLabel label = new JLabel(“商品编号:”);
label.setBounds(85, 89, 87, 22);
getContentPane().add(label);
id = new JTextField();
id.setBounds(147, 90, 142, 21);
getContentPane().add(id);
id.setColumns(10);
JLabel label_1 = new JLabel(“商品名称”);
label_1.setBounds(85, 139, 87, 22);
getContentPane().add(label_1);
name = new JTextField();
name.setColumns(10);
name.setBounds(147, 140, 142, 21);
getContentPane().add(name);
JLabel label_2 = new JLabel(“数量:”);
label_2.setBounds(85, 193, 87, 22);
getContentPane().add(label_2);
num = new JTextField();
num.setColumns(10);
num.setBounds(147, 194, 142, 21);
getContentPane().add(num);
JLabel label_3 = new JLabel(“单价:”);
label_3.setBounds(85, 241, 87, 22);
getContentPane().add(label_3);
price = new JTextField();
price.setColumns(10);
price.setBounds(147, 242, 142, 21);
getContentPane().add(price);
goodsid = goods.getGoodsID();
id.setText(Integer.toString(goods.getGoodsID()));
name.setText(goods.getGoodsName());
num.setText(Integer.toString(goods.getNum()));
price.setText(goods.getPrice());
button = new JButton(“确定”);
button.setBounds(78, 317, 93, 23);
getContentPane().add(button);
button.addActionListener(new ActionListener() {
public void actionPerformed(ActionEvent arg0) {
String addId = id.getText();
String addName = name.getText();
String addNum = num.getText();
String addPrice = num.getText();
if (addName.equals(“”)||addName.equals(“”)||addNum.equals(“”)||addPrice.equals(“”)) {
JOptionPane.showMessageDialog(null, “请完整输入要修改的数据”);
} else {
String sql=“UPDATE goods SET “+“Goodsid='”+addId+”‘,Goodsname=’”+addName+“‘,num=’”+addNum+“‘,price=’”+addPrice+“'where goodsid=”+goodsid;
int result = Updata.addData(sql);
if (result>0) {
JOptionPane.showMessageDialog(null, “修改成功!”);
JOptionPane.showMessageDialog(null, “记得刷新一下哦!”);
dispose();
// GoodsManage i = new GoodsManage();
// i.setVisible(true);
} else {
JOptionPane.showMessageDialog(null, “修改失败!”);
}
}
}
});
button_1 = new JButton(“取消”);
button_1.setBounds(208, 317, 93, 23);
getContentPane().add(button_1);
button_1.addActionListener(new ActionListener() {
public void actionPerformed(ActionEvent arg0) {
dispose();
}
});
}
public static void main(String[] args) {
GoodsXG g = new GoodsXG(null);
g.setVisible(true);
}
}
GoodsADD.Java
package com.ynavc.Vive;
import javax.swing.JFrame;
import javax.swing.JLabel;
import javax.swing.JOptionPane;
import javax.swing.JTextField;
import com.ynavc.Bean.Goods;
import com.ynavc.Controller.Updata;
import java.awt.event.ActionEvent;
import java.awt.event.ActionListener;
import javax.swing.JButton;
public class GoodsADD extends JFrame {
private JTextField id,name,num,price;
private JButton button;
private JButton button_1;
public GoodsADD() {
super(“商品管理系统”);
this.setBounds(0, 0, 400, 450);
this.setLocationRelativeTo(null);//让窗口在屏幕中间显示
this.setResizable(false);//让窗口大小不可改变
getContentPane().setLayout(null);
JLabel label = new JLabel(“商品编号:”);
label.setBounds(85, 89, 87, 22);
getContentPane().add(label);
id = new JTextField();
id.setBounds(147, 90, 142, 21);
getContentPane().add(id);
id.setColumns(10);
JLabel label_1 = new JLabel(“商品名称”);
label_1.setBounds(85, 139, 87, 22);
getContentPane().add(label_1);
name = new JTextField();
name.setColumns(10);
name.setBounds(147, 140, 142, 21);
getContentPane().add(name);
JLabel label_2 = new JLabel(“数量:”);
label_2.setBounds(85, 193, 87, 22);
getContentPane().add(label_2);
num = new JTextField();
num.setColumns(10);
num.setBounds(147, 194, 142, 21);
getContentPane().add(num);
JLabel label_3 = new JLabel(“单价:”);
label_3.setBounds(85, 241, 87, 22);
getContentPane().add(label_3);
price = new JTextField();
price.setColumns(10);
price.setBounds(147, 242, 142, 21);
getContentPane().add(price);
button = new JButton(“确定”);
button.setBounds(78, 317, 93, 23);
getContentPane().add(button);
button.addActionListener(new ActionListener() {
public void actionPerformed(ActionEvent arg0) {
String addId = id.getText();
String addName = name.getText();
String addNum = num.getText();
String addPrice = num.getText();
if (addName.equals(“”)||addName.equals(“”)||addNum.equals(“”)||addPrice.equals(“”)) {
JOptionPane.showMessageDialog(null, “请完整输入要添加的数据”);
} else {
String sql=“INSERT INTO goods VALUES(”+addId+“,'”+addName+“‘,’”+addNum+“‘,’”+addPrice+“')”;
int result = Updata.addData(sql);
if (result>0) {
JOptionPane.showMessageDialog(null, “添加成功!”);
JOptionPane.showMessageDialog(null, “记得刷新一下哦!”);
dispose();
// GoodsManage i = new GoodsManage();
// i.setVisible(true);
} else {
JOptionPane.showMessageDialog(null, “添加失败!”);
}
自我介绍一下,小编13年上海交大毕业,曾经在小公司待过,也去过华为、OPPO等大厂,18年进入阿里一直到现在。
深知大多数Java工程师,想要提升技能,往往是自己摸索成长或者是报班学习,但对于培训机构动则几千的学费,着实压力不小。自己不成体系的自学效果低效又漫长,而且极易碰到天花板技术停滞不前!
因此收集整理了一份《2024年Java开发全套学习资料》,初衷也很简单,就是希望能够帮助到想自学提升又不知道该从何学起的朋友,同时减轻大家的负担。
既有适合小白学习的零基础资料,也有适合3年以上经验的小伙伴深入学习提升的进阶课程,基本涵盖了95%以上Java开发知识点,真正体系化!
由于文件比较大,这里只是将部分目录截图出来,每个节点里面都包含大厂面经、学习笔记、源码讲义、实战项目、讲解视频,并且会持续更新!
如果你觉得这些内容对你有帮助,可以扫码获取!!(备注Java获取)
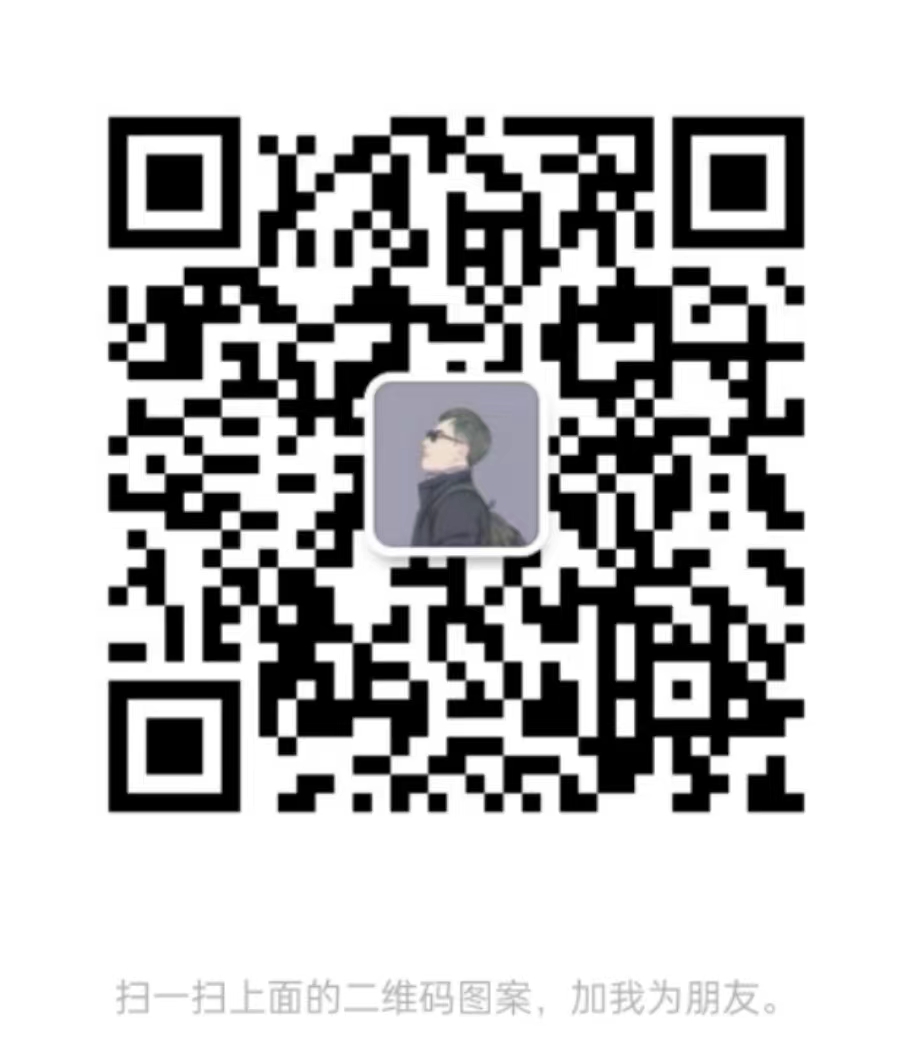
最后
现在正是金三银四的春招高潮,前阵子小编一直在搭建自己的网站,并整理了全套的**【一线互联网大厂Java核心面试题库+解析】:包括Java基础、异常、集合、并发编程、JVM、Spring全家桶、MyBatis、Redis、数据库、中间件MQ、Dubbo、Linux、Tomcat、ZooKeeper、Netty等等**
《互联网大厂面试真题解析、进阶开发核心学习笔记、全套讲解视频、实战项目源码讲义》点击传送门即可获取!
g(null, “添加成功!”);
JOptionPane.showMessageDialog(null, “记得刷新一下哦!”);
dispose();
// GoodsManage i = new GoodsManage();
// i.setVisible(true);
} else {
JOptionPane.showMessageDialog(null, “添加失败!”);
}
自我介绍一下,小编13年上海交大毕业,曾经在小公司待过,也去过华为、OPPO等大厂,18年进入阿里一直到现在。
深知大多数Java工程师,想要提升技能,往往是自己摸索成长或者是报班学习,但对于培训机构动则几千的学费,着实压力不小。自己不成体系的自学效果低效又漫长,而且极易碰到天花板技术停滞不前!
因此收集整理了一份《2024年Java开发全套学习资料》,初衷也很简单,就是希望能够帮助到想自学提升又不知道该从何学起的朋友,同时减轻大家的负担。[外链图片转存中…(img-bZrZOuBA-1713519028441)]
[外链图片转存中…(img-gXxnekSP-1713519028444)]
[外链图片转存中…(img-gKul8Hsl-1713519028445)]
既有适合小白学习的零基础资料,也有适合3年以上经验的小伙伴深入学习提升的进阶课程,基本涵盖了95%以上Java开发知识点,真正体系化!
由于文件比较大,这里只是将部分目录截图出来,每个节点里面都包含大厂面经、学习笔记、源码讲义、实战项目、讲解视频,并且会持续更新!
如果你觉得这些内容对你有帮助,可以扫码获取!!(备注Java获取)
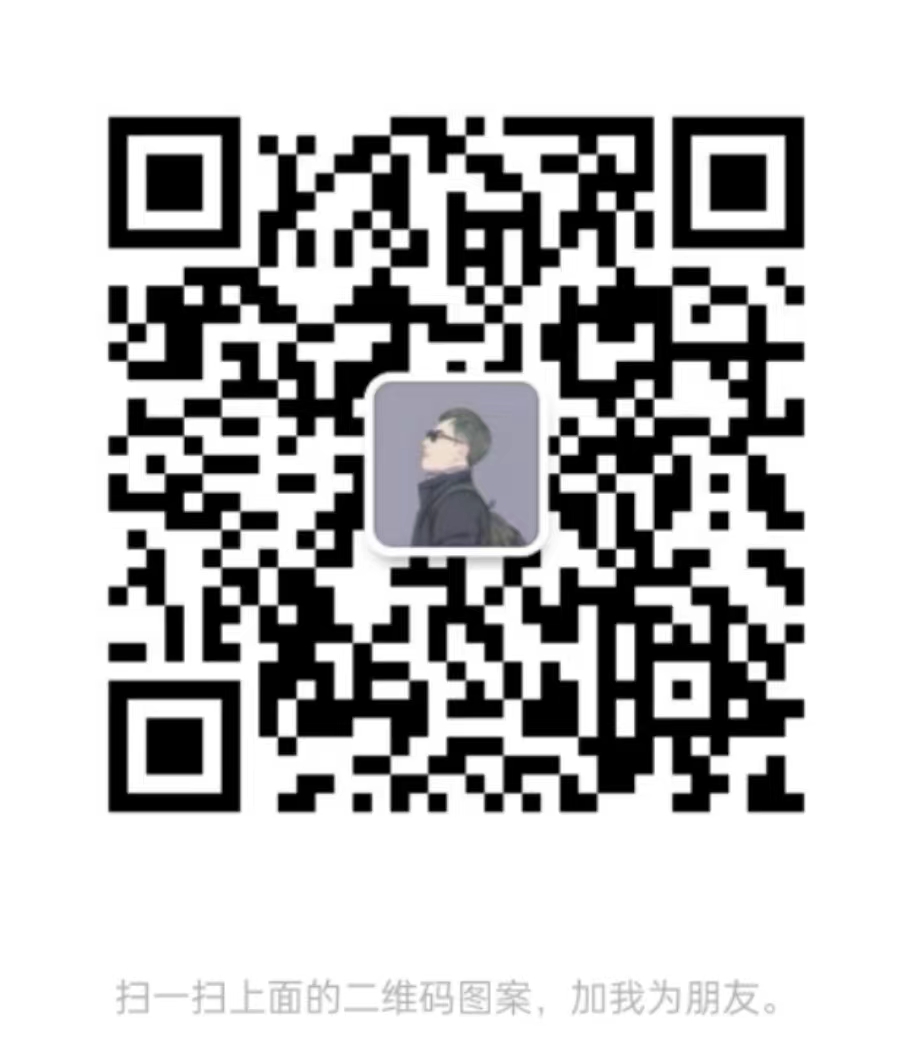
最后
现在正是金三银四的春招高潮,前阵子小编一直在搭建自己的网站,并整理了全套的**【一线互联网大厂Java核心面试题库+解析】:包括Java基础、异常、集合、并发编程、JVM、Spring全家桶、MyBatis、Redis、数据库、中间件MQ、Dubbo、Linux、Tomcat、ZooKeeper、Netty等等**
[外链图片转存中…(img-wQ8NFEUM-1713519028447)]
《互联网大厂面试真题解析、进阶开发核心学习笔记、全套讲解视频、实战项目源码讲义》点击传送门即可获取!