-
return heap;
-
}
-
public HeapImpl() {
-
this.heap = new ArrayList<>();
-
}
-
/**
-
* 插入对应上浮
-
*
-
* @param start
-
*/
-
protected void adjustUp(int start) {
-
int currentIndex = start;
-
int parentIndex = (currentIndex - 1) / 2;
-
T tmp = heap.get(currentIndex);
-
while (currentIndex > 0) {
-
int cmp = heap.get(parentIndex).compareTo(tmp);
-
if (cmp >= 0) {
-
break;
-
} else {
-
heap.set(currentIndex, heap.get(parentIndex));
-
currentIndex = parentIndex;
-
parentIndex = (parentIndex - 1) / 2;
-
}
-
}
-
heap.set(currentIndex, tmp);
-
}
-
public void insert(T data) {
-
int size = heap.size();
-
heap.add(data); // 将"数组"插在表尾
-
adjustUp(size); // 向上调整堆
-
}
-
public void remove(int index) {
-
int size = heap.size();
-
heap.set(index, heap.get(size - 1));
-
heap.remove(size - 1);
-
adjustDown(index);
-
}
-
/**
-
* 删除对应下沉
-
*
-
* @param index
-
*/
-
private void adjustDown(int index) {
-
int currentIndex = index;
-
int leftChildIndex = index * 2 + 1;
-
int rightChildIndex = index * 2 + 2;
-
T tmp = heap.get(currentIndex);
-
int size = heap.size();
-
while (leftChildIndex < size) {
-
T left = null;
-
T right = null;
-
if (leftChildIndex < size) {
-
left = heap.get(leftChildIndex);
-
}
-
if (rightChildIndex < size) {
-
right = heap.get(rightChildIndex);
-
}
-
int maxIndex = right == null ? leftChildIndex : (left.compareTo(right) >= 0 ? leftChildIndex : rightChildIndex);
-
T max = heap.get(maxIndex);
-
if(tmp.compareTo(max)>= 0){
-
break;
-
} else{
-
heap.set(currentIndex, max);
-
heap.set(maxIndex, tmp);
-
leftChildIndex = maxIndex * 2 + 1;
-
rightChildIndex = maxIndex + 1;
-
}
-
}
-
}
-
public void makeHeap(int first, int last) {
-
for ( int i = first; i < last; i++) {
-
insert(orginList.get(i));
-
}
-
}
-
public void popHeap(int first, int last) {
-
remove(first);
-
}
-
public void pushHeap(int first, int last) {
-
adjustUp(last - 1);
-
}
-
public void display() {
-
System.out.println(heap);
-
}
-
public static void main(String[] args) {
-
IHeap heap = new HeapImpl<>();
-
heap.initOriginList(Arrays.asList( 10, 20, 30, 5, 15));
-
System.out.println( “初始构建堆:”);
-
heap.makeHeap( 0, 5);
自我介绍一下,小编13年上海交大毕业,曾经在小公司待过,也去过华为、OPPO等大厂,18年进入阿里一直到现在。
深知大多数Java工程师,想要提升技能,往往是自己摸索成长或者是报班学习,但对于培训机构动则几千的学费,着实压力不小。自己不成体系的自学效果低效又漫长,而且极易碰到天花板技术停滞不前!
因此收集整理了一份《2024年Java开发全套学习资料》,初衷也很简单,就是希望能够帮助到想自学提升又不知道该从何学起的朋友,同时减轻大家的负担。
既有适合小白学习的零基础资料,也有适合3年以上经验的小伙伴深入学习提升的进阶课程,基本涵盖了95%以上Java开发知识点,真正体系化!
由于文件比较大,这里只是将部分目录截图出来,每个节点里面都包含大厂面经、学习笔记、源码讲义、实战项目、讲解视频,并且会持续更新!
如果你觉得这些内容对你有帮助,可以扫码获取!!(备注Java获取)
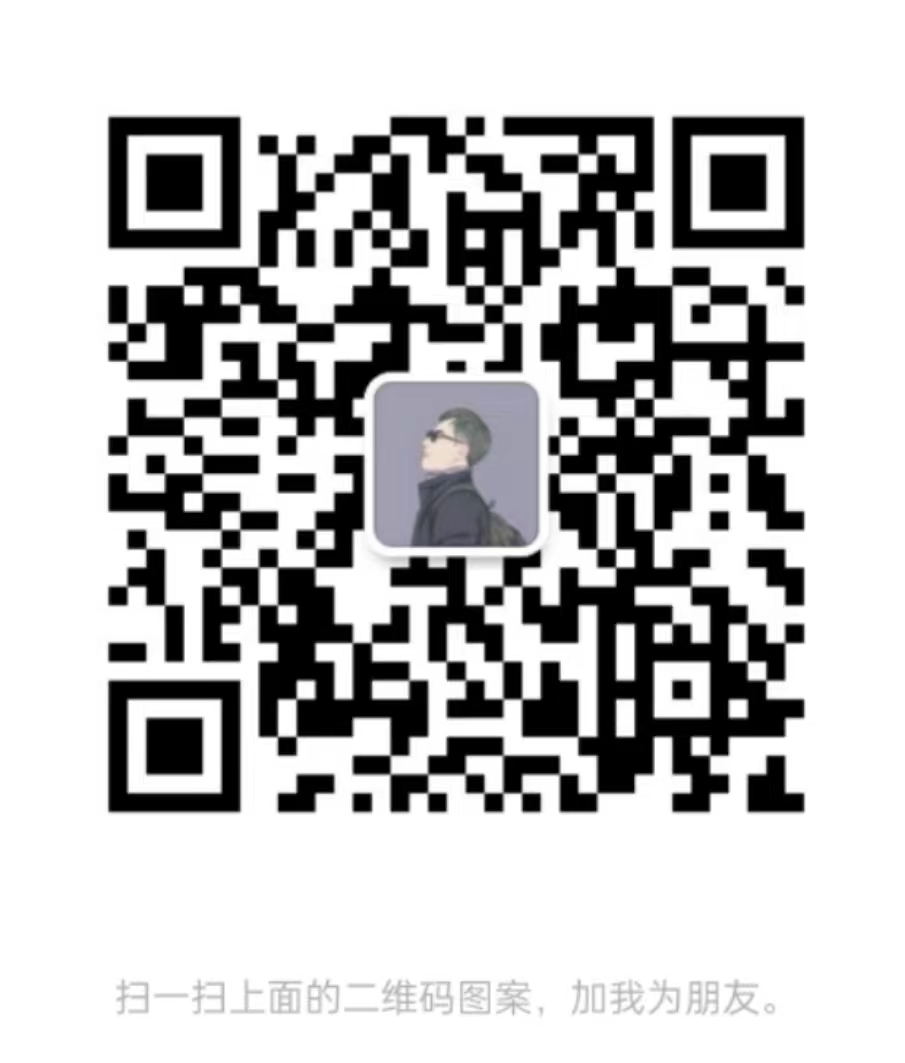
如何快速更新自己的技术积累?
- 在现有的项目里,深挖技术,比如用到netty可以把相关底层代码和要点都看起来。
- 如果不知道目前的努力方向,就看自己的领导或公司里技术强的人在学什么。
- 知道努力方向后不知道该怎么学,就到处去找相关资料然后练习。
- 学习以后不知道有没有学成,则可以通过面试去检验。
我个人觉得面试也像是一场全新的征程,失败和胜利都是平常之事。所以,劝各位不要因为面试失败而灰心、丧失斗志。也不要因为面试通过而沾沾自喜,等待你的将是更美好的未来,继续加油!
以上面试专题的答小编案整理成面试文档了,文档里有答案详解,以及其他一些大厂面试题目
《互联网大厂面试真题解析、进阶开发核心学习笔记、全套讲解视频、实战项目源码讲义》点击传送门即可获取!
知道该怎么学,就到处去找相关资料然后练习。
- 学习以后不知道有没有学成,则可以通过面试去检验。
我个人觉得面试也像是一场全新的征程,失败和胜利都是平常之事。所以,劝各位不要因为面试失败而灰心、丧失斗志。也不要因为面试通过而沾沾自喜,等待你的将是更美好的未来,继续加油!
以上面试专题的答小编案整理成面试文档了,文档里有答案详解,以及其他一些大厂面试题目
[外链图片转存中…(img-RESgpY12-1713471507315)]
[外链图片转存中…(img-wjGiZpN1-1713471507316)]
《互联网大厂面试真题解析、进阶开发核心学习笔记、全套讲解视频、实战项目源码讲义》点击传送门即可获取!