contextConfigLocation
classpath:app.xml
1
springmvc
*.do
app.xml
<?xml version="1.0" encoding="UTF-8"?><beans xmlns=“http://www.springframework.org/schema/beans”
xmlns:xsi=“http://www.w3.org/2001/XMLSchema-instance”
xmlns:context=“http://www.springframework.org/schema/context”
xmlns:jdbc=“http://www.springframework.org/schema/jdbc”
xmlns:jee=“http://www.springframework.org/schema/jee”
xmlns:tx=“http://www.springframework.org/schema/tx”
xmlns:aop=“http://www.springframework.org/schema/aop”
xmlns:mvc=“http://www.springframework.org/schema/mvc”
xmlns:util=“http://www.springframework.org/schema/util”
xmlns:jpa=“http://www.springframework.org/schema/data/jpa”
xsi:schemaLocation="
http://www.springframework.org/schema/beans http://www.springframework.org/schema/beans/spring-beans-3.2.xsd
http://www.springframework.org/schema/context http://www.springframework.org/schema/context/spring-context-3.2.xsd
http://www.springframework.org/schema/jdbc http://www.springframework.org/schema/jdbc/spring-jdbc-3.2.xsd
http://www.springframework.org/schema/jee http://www.springframework.org/schema/jee/spring-jee-3.2.xsd
http://www.springframework.org/schema/tx http://www.springframework.org/schema/tx/spring-tx-3.2.xsd
http://www.springframework.org/schema/data/jpa http://www.springframework.org/schema/data/jpa/spring-jpa-1.3.xsd
http://www.springframework.org/schema/aop http://www.springframework.org/schema/aop/spring-aop-3.2.xsd
http://www.springframework.org/schema/mvc http://www.springframework.org/schema/mvc/spring-mvc-3.2.xsd
http://www.springframework.org/schema/util http://www.springframework.org/schema/util/spring-util-3.2.xsd">
<context:component-scan base-package=“controller”/>
mvc:annotation-driven/
HelloController.java
package controller;
import java.io.UnsupportedEncodingException;
import java.util.HashMap;
import java.util.Map;
import javax.servlet.http.HttpServletRequest;
import javax.servlet.http.HttpSession;
import org.springframework.stereotype.Controller;
import org.springframework.ui.ModelMap;
import org.springframework.web.bind.annotation.RequestMapping;
import org.springframework.web.bind.annotation.RequestParam;
import org.springframework.web.servlet.ModelAndView;
import org.springframework.web.servlet.view.RedirectView;
/**
-
用注解的方式来开发Controller
-
1.不用实现Controller接口。
-
2.可以添加多个处理方法。
-
3.处理方法要求:
-
a.方法名不做要求。
-
b.返回值可以是ModelAndView,可以是
-
String,也可以是void。
-
4.使用到的注解:
-
@Controller:要加在类前面。
-
@RequestMapping:可以加到类前面,也可以
-
添加到处理方法前面。用来设置请求路径与
-
处理方法的对应关系。
-
@author Cher_du
*/
@Controller
public class HelloController {
@RequestMapping(“/hello.do”)
public ModelAndView toHello(){
System.out.println(“HelloController的”+
“toHello方法…”);
return new ModelAndView(“hello”);
}
/*
-
处理方法的返回值可以是ModelAndView,
-
也可以是String。区别:
-
如果返回的既有数据,也有视图名,可以
-
使用ModelAndView。如果返回值只有视图名,
-
可以直接返回String。
*/
@RequestMapping(“/toLogin.do”)
public String toLogin(){
System.out.println(“HelloController的”+
“toLogin方法…”);
return “login”;
}
@RequestMapping(“/login.do”)
public String checkLogin(HttpServletRequest request) throws UnsupportedEncodingException{
System.out.println(“HelloController的”+
“checkLogin方法”);
request.setCharacterEncoding(“utf-8”);
String username = request.getParameter(“username”);
String pwd = request.getParameter(“pwd”);
System.out.println(“username:”+username+" pwd:"+pwd);
//默认情况下,前端控制器会转发
//到某个jsp页面。
return “success”;
}
@RequestMapping(“/login2.do”)
/*
*获得请求参数值的第二种方式:
*处理方法的入参与请求参数名一致。
*注:
-
如果不一致,可以使用
-
@RequestParam(请求参数名)
*/
public String checkLogin2(String username,@RequestParam(“pwd”)String password){
System.out.println(“HelloController的”+
“checkLogin2方法…”);
System.out.println(“username:”+username+" pwd:"+password);
return “success”;
}
@RequestMapping(“/login3.do”)
/*
-
读取请求参数的第三种方式:
-
step1.先写一个java类,该类要添加
-
与请求参数名一致的属性,并且为这些
-
属性添加相应的get/set方法。
-
step2.将这个类作为处理方法的入参。
*/
public String checkLogin3(User user){
System.out.println(“HelloController的”+
“checkLogin3方法…”);
System.out.println(“username:”+user.getUsername()+" pwd:"+user.getPwd());
return “success”;
}
//====================================================================//
/*
- 使用ModelAndView向页面传值
*/
@RequestMapping(“/login4.do”)
public ModelAndView checkLogin4(User user){
System.out.println(“HelloController的”+
“checkLogin4方法…”);
System.out.println(“username:”+user.getUsername()+" pwd:"+user.getPwd());
//要将处理结果封装成一个Map对象。
Map<String,Object> data = new HashMap<String,Object>();
//相当于执行了request.setAttribute
data.put(“user”, user);
ModelAndView mav = new ModelAndView(“success”,data);
return mav;
}
/*
*使用request对象向页面传值。
*/
@RequestMapping(“/login5.do”)
public String checkLogin5(User user,
HttpServletRequest request) throws UnsupportedEncodingException{
System.out.println(“HelloController的”+
“checkLogin5方法…”);
request.setCharacterEncoding(“utf-8”);
request.setAttribute(“user”, user);
return “success”;
}
@RequestMapping(“/login6.do”)
/*
- 使用ModelMap对象来向页面传值。
*/
public String checkLogin6(User user,ModelMap data){
System.out.println(“HelloController的”+
“checkLogin6方法…”);
//相当于request.setAttribute
data.addAttribute(“user”,user);
return “success”;
}
@RequestMapping(“/login7.do”)
/*
- 使用session向页面传值
*/
public String checkLogin7(User user,
HttpSession session){
System.out.println(“HelloController的”+
“checkLogin7方法…”);
session.setAttribute(“user”, user);
return “success”;
}
//==================================================//
@RequestMapping(“/login8.do”)
/*
-
演示如何重定向。
-
如果处理方法的返回值是String,
-
只需要将"redirect:"作为返回值的前缀。
*/
public String checkLogin8(User user){
System.out.println(“HelloController的”+
“checkLogin8方法…”);
return “redirect:toSuccess.do”;
}
@RequestMapping(“/login9.do”)
/*
-
演示重定向。
-
返回值是ModelAndView。
*/
public ModelAndView checkLogin9(){
System.out.println(“HelloController的”+
“checkLogin9方法…”);
RedirectView rv = new RedirectView(“toSuccess.do”);
return new ModelAndView(rv);
}
@RequestMapping(“/toSuccess.do”)
public String toSuccess(){
System.out.println(“…toSuccess…”);
return “success”;
}
}
User.java
自我介绍一下,小编13年上海交大毕业,曾经在小公司待过,也去过华为、OPPO等大厂,18年进入阿里一直到现在。
深知大多数Java工程师,想要提升技能,往往是自己摸索成长或者是报班学习,但对于培训机构动则几千的学费,着实压力不小。自己不成体系的自学效果低效又漫长,而且极易碰到天花板技术停滞不前!
因此收集整理了一份《2024年Java开发全套学习资料》,初衷也很简单,就是希望能够帮助到想自学提升又不知道该从何学起的朋友,同时减轻大家的负担。
既有适合小白学习的零基础资料,也有适合3年以上经验的小伙伴深入学习提升的进阶课程,基本涵盖了95%以上Java开发知识点,真正体系化!
由于文件比较大,这里只是将部分目录截图出来,每个节点里面都包含大厂面经、学习笔记、源码讲义、实战项目、讲解视频,并且会持续更新!
如果你觉得这些内容对你有帮助,可以扫码获取!!(备注Java获取)
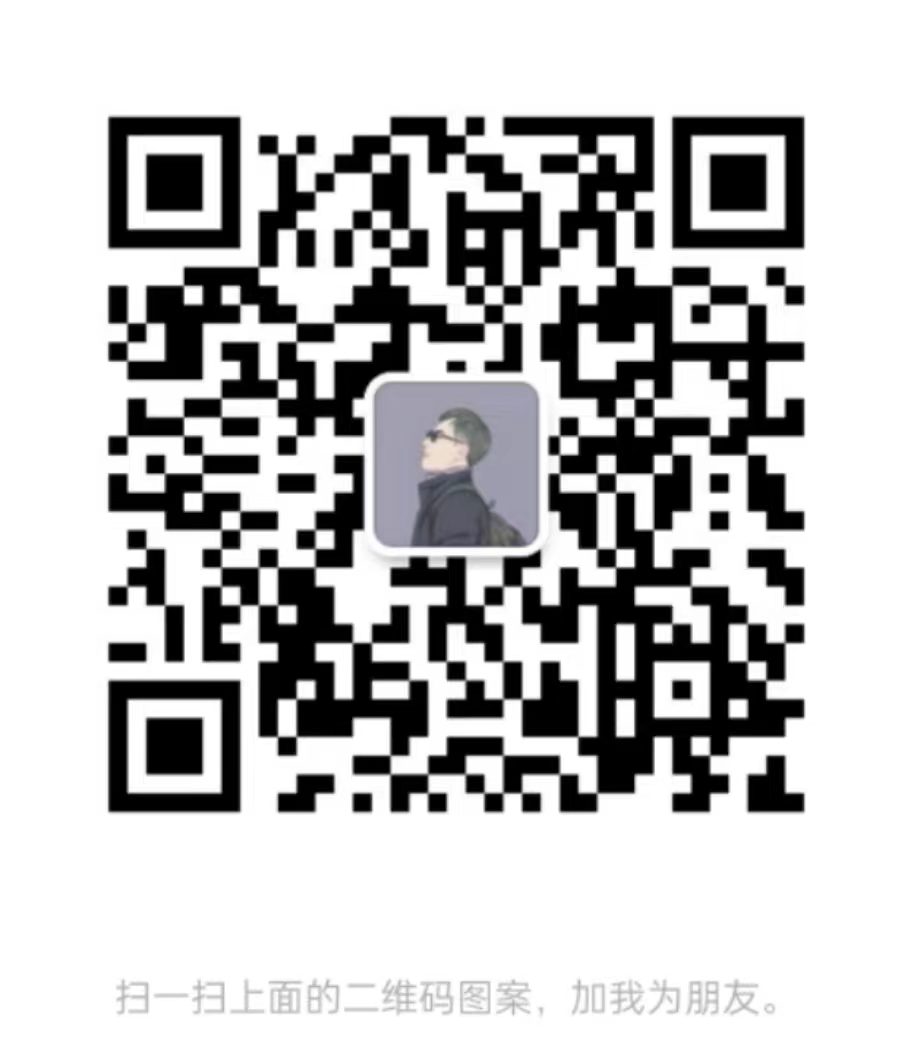
总结
这个月马上就又要过去了,还在找工作的小伙伴要做好准备了,小编整理了大厂java程序员面试涉及到的绝大部分面试题及答案,希望能帮助到大家
《互联网大厂面试真题解析、进阶开发核心学习笔记、全套讲解视频、实战项目源码讲义》点击传送门即可获取!
**
由于文件比较大,这里只是将部分目录截图出来,每个节点里面都包含大厂面经、学习笔记、源码讲义、实战项目、讲解视频,并且会持续更新!
如果你觉得这些内容对你有帮助,可以扫码获取!!(备注Java获取)
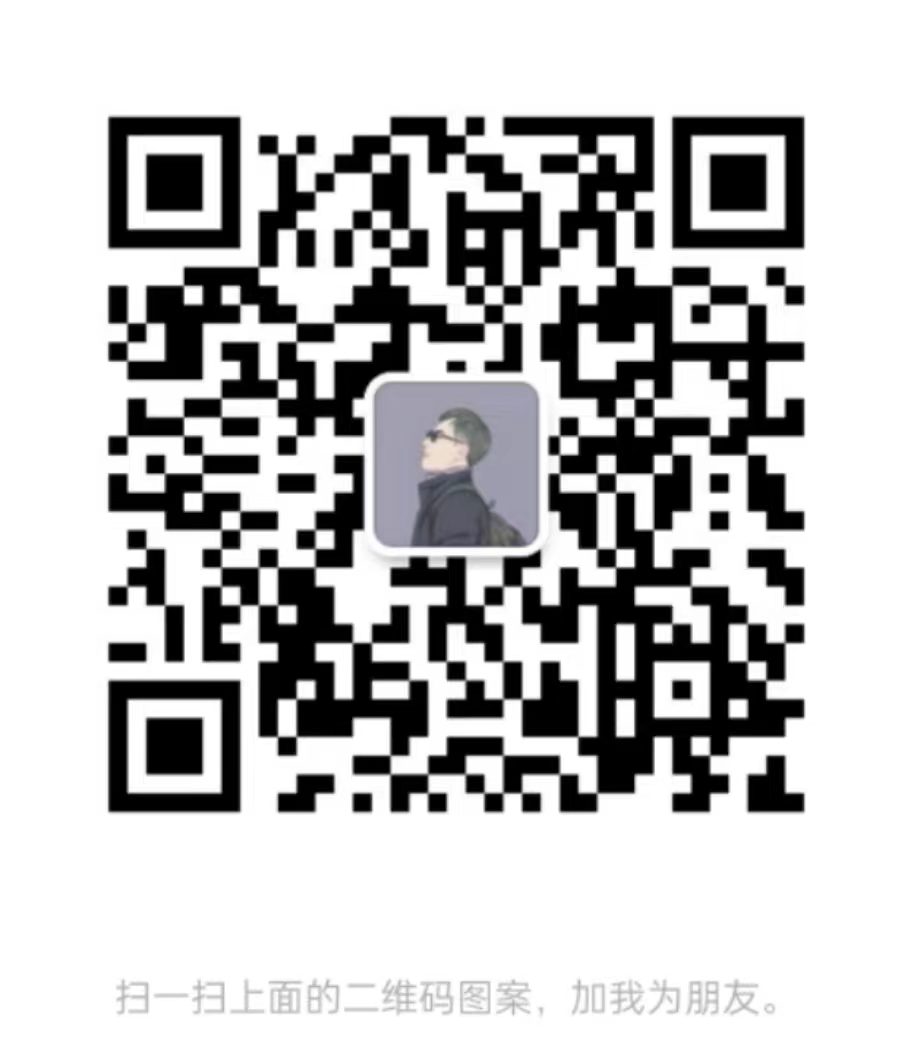
总结
这个月马上就又要过去了,还在找工作的小伙伴要做好准备了,小编整理了大厂java程序员面试涉及到的绝大部分面试题及答案,希望能帮助到大家
[外链图片转存中…(img-lAfKSJ14-1712879075800)]
[外链图片转存中…(img-cmbjuacY-1712879075800)]
《互联网大厂面试真题解析、进阶开发核心学习笔记、全套讲解视频、实战项目源码讲义》点击传送门即可获取!