this.id = id;
this.goodsTypeName = goodsTypeName;
this.goodsTypeDesc = goodsTypeDesc;
}
@Override
public String toString() {
return goodsTypeName;
}
// Set和Get方法
public int getId() {
return id;
}
public void setId(int id) {
this.id = id;
}
public String getGoodsTypeName() {
return goodsTypeName;
}
public void setGoodsTypeName(String goodsTypeName) {
this.goodsTypeName = goodsTypeName;
}
public String getGoodsTypeDesc() {
return goodsTypeDesc;
}
public void setGoodsTypeDesc(String goodsTypeDesc) {
this.goodsTypeDesc = goodsTypeDesc;
}
}
package com.sjsq.model;
/**
-
管理员t_user表的User实体类
-
@author shuijianshiqing
*/
public class User {
private int id;// id字段
private String username;// username字段
private String password;// password字段
// 继承父类的构造方法
public User() {
super();
}
/**
-
重载父类的构造方法带2个参数
-
@param userName
-
@param password
*/
public User(String username, String password) {
super();
this.username = username;
this.password = password;
}
/**
-
Set及Get方法
-
@return
*/
public int getId() {
return id;
}
public void setId(int id) {
this.id = id;
}
public String getUsername() {
return username;
}
public void setUsername(String username) {
this.username = username;
}
public String getPassword() {
return password;
}
public void setPassword(String password) {
this.password = password;
}
}
package com.sjsq.util;
import java.sql.Connection;
import java.sql.DriverManager;
import java.sql.ResultSet;
import java.sql.SQLException;
/**
-
数据库的连接
-
@author shuijianshiqing
*/
public class DbUtil {
// 数据库驱动名
private static String DriverName = “com.mysql.cj.jdbc.Driver”;
// 数据库协议
private static String dbUril = “jdbc:mysql://localhost:3306/db_warehouse?serverTimezone=UTC”;
// 数据库用户名
private static String dbName = “root”;
// 数据库密码
private static String dbPassword = “admin”;
/**
-
数据库驱动的加载与数据库连接
-
@return
-
@throws Exception
*/
public static Connection getCon() throws Exception {
Class.forName(DriverName);
Connection conn = DriverManager.getConnection(dbUril, dbName, dbPassword);
return conn;
}
/**
-
关闭数据库连接
-
@param conn
-
@throws SQLException
*/
public static void close(Connection conn) throws SQLException {
if (conn != null) {
conn.close();
}
}
/**
-
关闭数据库连接
-
@param conn
-
@param rs
-
@throws SQLException
*/
public static void close(Connection conn, ResultSet rs) throws SQLException {
if (rs != null) {
rs.close();
if (conn != null) {
conn.close();
}
}
}
/**
-
测试main()方法
-
@param args
*/
public static void main(String[] args) {
try {
getCon();
System.out.println(“数据库连接成功!”);
} catch (Exception e) {
// TODO Auto-generated catch block
e.printStackTrace();
System.out.println(“数据库连接失败!”);
}
}
}
package com.sjsq.util;
/**
-
判断用户的输入是否为空方法类
-
@author shuijianshiqing
*/
public class StringUtil {
/**
-
判断字符串是否为空
-
@param str
-
@return
*/
public static boolean isEmpty(String str) {
if (str == null || “”.equals(str.trim())) {
return true;
} else {
return false;
}
}
/**
-
判断字符串是否不为空
-
@param str
-
@return
*/
public static boolean isNotEmpty(String str) {
if (str != null && !“”.equals(str.trim())) {
return true;
} else {
return false;
}
}
}
package com.sjsq.dao;
import java.sql.Connection;
import java.sql.PreparedStatement;
import java.sql.ResultSet;
import com.sjsq.model.Goods;
import com.sjsq.util.DbUtil;
import com.sjsq.util.StringUtil;
/**
-
货物控制操作包
-
@author Peter
-
@author shuijianshiqing
*/
public class GoodsDao {
/**
-
货物删除事件
-
@param conn
-
@param goods
-
@return
-
@throws Exception
*/
public static int deleteGoods(Connection conn, Goods goods) throws Exception {
String sql = “delete from t_goods where id = ?”;
PreparedStatement pstmt = conn.prepareStatement(sql);
pstmt.setInt(1, goods.getId());
return pstmt.executeUpdate();
}
/**
-
货物更新事件
-
@param conn
-
@param goods
-
@return
-
@throws Exception
*/
public static int updateGoods(Connection conn, Goods goods) throws Exception {
String sql = “update t_goods set goodsName=?, goodsSupplier=?, sex=?,”
- " price=?, goodsDesc=? , goodsTypeId = ? where id = ?";
PreparedStatement pstmt = conn.prepareStatement(sql);
pstmt.setString(1, goods.getGoodsName());
pstmt.setString(2, goods.getGoodsSupplier());
pstmt.setString(3, goods.getSex());
pstmt.setDouble(4, goods.getPrice());
pstmt.setString(5, goods.getGoodsDesc());
pstmt.setInt(6, goods.getGoodsTypeId());
pstmt.setInt(7, goods.getId());
return pstmt.executeUpdate();
}
/**
-
货物查询事件
-
@param conn
-
@param goods
-
@return
-
@throws Exception
*/
public static ResultSet listGoods(Connection conn, Goods goods) throws Exception {
StringBuffer sb = new StringBuffer(“select * from t_goods t_g, t_goodsType t_gt where t_g.goodsTypeId=t_gt.id”);
if (StringUtil.isNotEmpty(goods.getGoodsName())) {
sb.append(" and t_g.goodsName like ‘%" + goods.getGoodsName() + "%’");
}
if (StringUtil.isNotEmpty(goods.getGoodsSupplier())) {
sb.append(" and t_g.goodsSupplier like ‘%" + goods.getGoodsSupplier() + "%’");
}
if (goods.getGoodsTypeId() != null && goods.getGoodsTypeId() != -1) {
sb.append(" and t_g.goodsTypeId like ‘%" + goods.getGoodsTypeId() + "%’");
}
PreparedStatement pstmt = conn.prepareStatement(sb.toString());
return pstmt.executeQuery();
}
/**
-
添加货物
-
@param conn
-
@param goods
-
@return
-
@throws Exception
*/
public static int addGoods(Connection conn, Goods goods) throws Exception {
String sql = “insert into t_goods values(null, ?, ?, ?, ?, ?, ?,?)”;
PreparedStatement pstmt = conn.prepareStatement(sql);
pstmt.setString(1, goods.getGoodsName());
pstmt.setString(2, goods.getGoodsSupplier());
pstmt.setString(3, goods.getSex());
pstmt.setDouble(4, goods.getPrice());
pstmt.setString(5, goods.getGoodsDesc());
pstmt.setInt(6, goods.getGoodsTypeId());
pstmt.setString(7, goods.getGoodsTypeName());
return pstmt.executeUpdate();
}
public static void main(String[] args) {
Connection conn;
try {
conn = DbUtil.getCon();
GoodsDao goodsDao = new GoodsDao();
Goods goods = new Goods(1,“q”, “q”, “nv”, 2, “q”, 1,“AA”);
System.out.println(goods);
int result = goodsDao.addGoods(conn, goods);
System.out.println(result);
} catch (Exception e) {
e.printStackTrace();
}
}
}
package com.sjsq.dao;
import java.sql.Connection;
import java.sql.PreparedStatement;
import java.sql.ResultSet;
import com.sjsq.model.User;
/**
-
UserDao实体类是对user管理员的操做控制
-
@author shuijianshiqing
*/
public class UserDao {
/**
-
管理员登录操作
-
@param conn
-
@param user
-
@return
-
@throws Exception
*/
public User login(Connection conn, User user) throws Exception {
User resultUser = null;
String sql = “select * from t_user where username=? and password=?”;
PreparedStatement pstmt = conn.prepareStatement(sql);
pstmt.setString(1, user.getUsername());
pstmt.setString(2, user.getPassword());
ResultSet rs = pstmt.executeQuery();
if (rs.next()) {
resultUser = new User();
resultUser.setId(rs.getInt(“id”));
resultUser.setUsername(rs.getString(“username”));
resultUser.setPassword(rs.getString(“password”));
}
return resultUser;
}
}
package com.sjsq.view;
import java.awt.EventQueue;
import java.awt.Font;
import java.awt.Toolkit;
import java.awt.event.ActionEvent;
import java.awt.event.ActionListener;
import java.sql.Connection;
import java.sql.SQLException;
import javax.swing.GroupLayout;
import javax.swing.GroupLayout.Alignment;
import javax.swing.ImageIcon;
import javax.swing.JButton;
import javax.swing.JFrame;
import javax.swing.JLabel;
import javax.swing.JOptionPane;
import javax.swing.JPanel;
import javax.swing.JPasswordField;
import javax.swing.JTextField;
import javax.swing.UIManager;
import javax.swing.border.EmptyBorder;
import com.sjsq.dao.UserDao;
import com.sjsq.model.User;
import com.sjsq.util.DbUtil;
import com.sjsq.util.StringUtil;
/**
-
登录页面视图层
-
@author shuijianshiqing
*/
public class LoginFrm extends JFrame {
private static DbUtil dbUtil = new DbUtil();
private static UserDao userDao = new UserDao();
// 主板
private JPanel contentPane;
// 账号
private JTextField userNameTxt;
// 密码
private JPasswordField passwordTxt;
/**
- 创建窗体
*/
public LoginFrm() {
// 该表系统默认字体
Font font = new Font(“Dialog”, Font.PLAIN, 12);
java.util.Enumeration keys = UIManager.getDefaults().keys();
while (keys.hasMoreElements()) {
Object key = keys.nextElement();
Object value = UIManager.get(key);
if (value instanceof javax.swing.plaf.FontUIResource) {
UIManager.put(key, font);
}
}
setIconImage(Toolkit.getDefaultToolkit().getImage(LoginFrm.class.getResource(“/images/goods_logo.png”)));
setTitle(“仓库管理系统”);
setDefaultCloseOperation(JFrame.EXIT_ON_CLOSE);
setBounds(100, 100, 546, 369);
contentPane = new JPanel();
contentPane.setBorder(new EmptyBorder(5, 5, 5, 5));
setContentPane(contentPane);
JLabel label = new JLabel(“仓库管理系统管理员登录界面”);
label.setFont(new Font(“宋体”, Font.BOLD, 20));
label.setIcon(new ImageIcon(LoginFrm.class.getResource(“/images/goods_logo.png”)));
JLabel label_1 = new JLabel(“账号”);
label_1.setIcon(new ImageIcon(LoginFrm.class.getResource(“/images/user.png”)));
JLabel label_2 = new JLabel(“密码”);
label_2.setIcon(new ImageIcon(LoginFrm.class.getResource(“/images/password.png”)));
userNameTxt = new JTextField();
userNameTxt.setColumns(10);
passwordTxt = new JPasswordField();
JButton btnNewButton = new JButton(“登录”);
btnNewButton.addActionListener(new ActionListener() {
public void actionPerformed(ActionEvent arg0) {
loginActionPerformed(arg0);
}
});
btnNewButton.setIcon(new ImageIcon(LoginFrm.class.getResource(“/images/login.png”)));
JButton button = new JButton(“重置”);
button.addActionListener(new ActionListener() {
public void actionPerformed(ActionEvent arg0) {
resetValueActionPerformed(arg0);
}
});
button.setIcon(new ImageIcon(LoginFrm.class.getResource(“/images/reset.png”)));
GroupLayout gl_contentPane = new GroupLayout(contentPane);
gl_contentPane.setHorizontalGroup(gl_contentPane.createParallelGroup(Alignment.LEADING)
.addGroup(gl_contentPane.createSequentialGroup().addContainerGap(99, Short.MAX_VALUE)
.addGroup(gl_contentPane.createParallelGroup(Alignment.LEADING).addComponent(label)
.addGroup(gl_contentPane.createSequentialGroup().addGap(38)
.addGroup(gl_contentPane.createParallelGroup(Alignment.TRAILING)
.addComponent(label_2).addComponent(label_1))
.addGap(18)
.addGroup(gl_contentPane.createParallelGroup(Alignment.LEADING, false)
.addComponent(passwordTxt)
.addComponent(userNameTxt, GroupLayout.DEFAULT_SIZE, 167,
Short.MAX_VALUE)
.addComponent(button, Alignment.TRAILING))))
.addGap(96))
.addGroup(gl_contentPane.createSequentialGroup().addGap(176).addComponent(btnNewButton)
.addContainerGap(261, Short.MAX_VALUE)));
gl_contentPane.setVerticalGroup(gl_contentPane.createParallelGroup(Alignment.LEADING)
.addGroup(gl_contentPane.createSequentialGroup().addGap(29).addComponent(label).addGap(42)
.addGroup(gl_contentPane.createParallelGroup(Alignment.BASELINE)
.addComponent(userNameTxt, GroupLayout.PREFERRED_SIZE, GroupLayout.DEFAULT_SIZE,
GroupLayout.PREFERRED_SIZE)
.addComponent(label_1))
.addGap(18)
.addGroup(gl_contentPane.createParallelGroup(Alignment.BASELINE).addComponent(label_2)
.addComponent(passwordTxt, GroupLayout.PREFERRED_SIZE, GroupLayout.DEFAULT_SIZE,
GroupLayout.PREFERRED_SIZE))
.addGap(48)
.addGroup(gl_contentPane.createParallelGroup(Alignment.BASELINE)
.addComponent(btnNewButton, GroupLayout.PREFERRED_SIZE, 28, GroupLayout.PREFERRED_SIZE)
.addComponent(button, GroupLayout.PREFERRED_SIZE, 27, GroupLayout.PREFERRED_SIZE))
.addContainerGap(37, Short.MAX_VALUE)));
contentPane.setLayout(gl_contentPane);
// 居中显示
this.setLocationRelativeTo(null);
}
/**
-
管理员登录
-
@param arg0
*/
private void loginActionPerformed(ActionEvent arg0) {
String userName = this.userNameTxt.getText();
String password = new String(this.passwordTxt.getPassword());
if (StringUtil.isEmpty(userName)) {
JOptionPane.showMessageDialog(null, “用户名不能为空!”);
return;
}
if (StringUtil.isEmpty(password)) {
JOptionPane.showMessageDialog(null, “密码不能为空!”);
return;
}
User user = new User(userName, password);
Connection conn = null;
try {
conn = dbUtil.getCon();
User currentUser = userDao.login(conn, user);
if (currentUser != null) {
// JOptionPane.showMessageDialog(null, “登录成功!”);
dispose();
new MainFrm().setVisible(true);
} else {
JOptionPane.showMessageDialog(null, “登录失败!”);
}
} catch (Exception e) {
e.printStackTrace();
JOptionPane.showMessageDialog(null, “登录失败!”);
} finally {
try {
dbUtil.close(conn);
} catch (SQLException e) {
e.printStackTrace();
}
}
}
/**
-
重置事件
-
@param arg0
*/
private void resetValueActionPerformed(ActionEvent arg0) {
// TODO Auto-generated method stub
this.resetValue();
}
/**
- 重置表单事件
*/
private void resetValue() {
this.userNameTxt.setText(“”);
this.passwordTxt.setText(“”);
}
/**
- 运行程序
*/
public static void main(String[] args) {
EventQueue.invokeLater(new Runnable() {
public void run() {
try {
LoginFrm frame = new LoginFrm();
frame.setVisible(true);
} catch (Exception e) {
e.printStackTrace();
}
}
});
}
}
package com.sjsq.view;
import java.awt.BorderLayout;
import java.awt.EventQueue;
import javax.swing.JFrame;
import javax.swing.JPanel;
import javax.swing.border.EmptyBorder;
import java.awt.Toolkit;
import javax.swing.JDesktopPane;
import javax.swing.JMenuBar;
import javax.swing.JMenu;
import javax.swing.JMenuItem;
import javax.swing.JOptionPane;
import javax.swing.ImageIcon;
import java.awt.event.ActionListener;
import java.awt.event.ActionEvent;
/**
-
主页面视图层
-
@author shuijianshiqing
*/
public class MainFrm extends JFrame {
private JPanel contentPane;
private JDesktopPane table;
/**
- 创建窗体
*/
public MainFrm() {
setTitle(“仓库系统主界面”);
setIconImage(Toolkit.getDefaultToolkit().getImage(MainFrm.class.getResource(“/images/goods_logo.png”)));
setDefaultCloseOperation(JFrame.EXIT_ON_CLOSE);
setBounds(100, 200, 900, 800);
JMenuBar menuBar = new JMenuBar();
setJMenuBar(menuBar);
JMenu menu = new JMenu(“仓库系统管理”);
menu.setIcon(new ImageIcon(MainFrm.class.getResource(“/images/manager.png”)));
menuBar.add(menu);
JMenu menu_2 = new JMenu(“仓库系统管理”);
menu_2.setIcon(new ImageIcon(MainFrm.class.getResource(“/images/goodmanager.png”)));
menu.add(menu_2);
JMenuItem menuItem_2 = new JMenuItem(“货物类型添加”);
menuItem_2.addActionListener(new ActionListener() {
public void actionPerformed(ActionEvent e) {
GoodsTypeAddInterFrm goodsTypeAddInterFrm = new GoodsTypeAddInterFrm();
goodsTypeAddInterFrm.setVisible(true);
table.add(goodsTypeAddInterFrm);
}
});
menuItem_2.setIcon(new ImageIcon(MainFrm.class.getResource(“/images/add.png”)));
menu_2.add(menuItem_2);
JMenuItem menuItem_3 = new JMenuItem(“货物类型修改”);
menuItem_3.addActionListener(new ActionListener() {
public void actionPerformed(ActionEvent e) {
GoodsTypeManagerInterFrm goodsTypeManagerInterFrm = new GoodsTypeManagerInterFrm();
goodsTypeManagerInterFrm.setVisible(true);
table.add(goodsTypeManagerInterFrm);
}
});
menuItem_3.setIcon(new ImageIcon(MainFrm.class.getResource(“/images/modify.png”)));
menu_2.add(menuItem_3);
JMenu menu_3 = new JMenu(“货物物品管理”);
menu_3.setIcon(new ImageIcon(MainFrm.class.getResource(“/images/goods.png”)));
menu.add(menu_3);
JMenuItem menuItem_4 = new JMenuItem(“货物物品添加”);
menuItem_4.addActionListener(new ActionListener() {
public void actionPerformed(ActionEvent arg0) {
GoodsAddInterFrm goodsAddInterFrm = new GoodsAddInterFrm();
goodsAddInterFrm.setVisible(true);
table.add(goodsAddInterFrm);
}
});
menuItem_4.setIcon(new ImageIcon(MainFrm.class.getResource(“/images/add.png”)));
menu_3.add(menuItem_4);
JMenuItem menuItem_5 = new JMenuItem(“货物物品修改”);
menuItem_5.addActionListener(new ActionListener() {
public void actionPerformed(ActionEvent arg0) {
GoodsManagerInterFrm goodsManagerInterFrm = new GoodsManagerInterFrm();
goodsManagerInterFrm.setVisible(true);
table.add(goodsManagerInterFrm);
}
});
menuItem_5.setIcon(new ImageIcon(MainFrm.class.getResource(“/images/modify.png”)));
menu_3.add(menuItem_5);
JMenuItem menuItem_1 = new JMenuItem(“安全退出”);
menuItem_1.addActionListener(new ActionListener() {
public void actionPerformed(ActionEvent e) {
exitSystemActionPerformed(e);
}
});
menuItem_1.setIcon(new ImageIcon(MainFrm.class.getResource(“/images/exit.png”)));
menu.add(menuItem_1);
JMenu menu_1 = new JMenu(“联系我们”);
menu_1.setIcon(new ImageIcon(MainFrm.class.getResource(“/images/contact.png”)));
menuBar.add(menu_1);
JMenuItem menuItem = new JMenuItem(“联系方式”);
menuItem.addActionListener(new ActionListener() {
public void actionPerformed(ActionEvent arg0) {
MyContactInterFrm myContactInterFrm = new MyContactInterFrm();
myContactInterFrm.setVisible(true);
table.add(myContactInterFrm);
}
});
menuItem.setIcon(new ImageIcon(MainFrm.class.getResource(“/images/phnoe.png”)));
menu_1.add(menuItem);
contentPane = new JPanel();
contentPane.setBorder(new EmptyBorder(5, 5, 5, 5));
contentPane.setLayout(new BorderLayout(0, 0));
setContentPane(contentPane);
table = new JDesktopPane();
contentPane.add(table, BorderLayout.CENTER);
// 居中显示
this.setLocationRelativeTo(null);
// 最大化处理
// this.setExtendedState(JFrame.MAXIMIZED_BOTH);
}
/**
-
安全退出系统
-
@param e
*/
private void exitSystemActionPerformed(ActionEvent e) {
int n = JOptionPane.showConfirmDialog(null, “你确定要离开系统么”);
if (n == 0) {
dispose();
return;
}
}
/**
- 运行程序
*/
public static void main(String[] args) {
EventQueue.invokeLater(new Runnable() {
public void run() {
try {
MainFrm frame = new MainFrm();
frame.setVisible(true);
} catch (Exception e) {
e.printStackTrace();
}
}
});
}
}
package com.sjsq.view;
import java.awt.EventQueue;
import java.awt.event.ActionEvent;
import java.awt.event.ActionListener;
import java.sql.Connection;
import java.sql.ResultSet;
import java.sql.SQLException;
import javax.swing.ButtonGroup;
import javax.swing.GroupLayout;
import javax.swing.GroupLayout.Alignment;
import javax.swing.JButton;
import javax.swing.JComboBox;
import javax.swing.JInternalFrame;
import javax.swing.JLabel;
import javax.swing.JOptionPane;
import javax.swing.JRadioButton;
import javax.swing.JTextArea;
import javax.swing.JTextField;
import javax.swing.LayoutStyle.ComponentPlacement;
import com.sjsq.dao.GoodsDao;
import com.sjsq.dao.GoodsTypeDao;
import com.sjsq.model.Goods;
import com.sjsq.model.GoodsType;
import com.sjsq.util.DbUtil;
import com.sjsq.util.StringUtil;
import javax.swing.ImageIcon;
/**
-
货物添加视图层
-
@author shuijianshiqing
*/
public class GoodsAddInterFrm extends JInternalFrame {
private JTextField goodsNameTxt;
private JTextField goodsSupplierTxt;
private JTextField priceTxt;
private JTextArea goodsDescTxt;
private JComboBox goodsTypeNameJcb;
private JRadioButton manJrb;
private JRadioButton femaleJrb;
private final ButtonGroup buttonGroup = new ButtonGroup();
private static DbUtil dbUtil = new DbUtil();
private static GoodsDao goodsDao = new GoodsDao();
/**
- 创建窗体
*/
public GoodsAddInterFrm() {
setClosable(true);
setIconifiable(true);
setTitle(“货物添加”);
setBounds(100, 100, 596, 399);
JLabel lblNewLabel = new JLabel(“货物名称:”);
goodsNameTxt = new JTextField();
goodsNameTxt.setColumns(10);
JLabel lblNewLabel_1 = new JLabel(“货物供应商:”);
goodsSupplierTxt = new JTextField();
goodsSupplierTxt.setColumns(10);
JLabel label = new JLabel(“供应商性别:”);
manJrb = new JRadioButton(“男”);
buttonGroup.add(manJrb);
manJrb.setSelected(true);
femaleJrb = new JRadioButton(“女”);
buttonGroup.add(femaleJrb);
JLabel lblNewLabel_2 = new JLabel(“货物价格:”);
priceTxt = new JTextField();
priceTxt.setColumns(10);
JLabel label_1 = new JLabel(“货物类别:”);
goodsTypeNameJcb = new JComboBox();
JLabel label_2 = new JLabel(“货物描述:”);
goodsDescTxt = new JTextArea();
JButton button = new JButton(“添加”);
button.setIcon(new ImageIcon(GoodsAddInterFrm.class.getResource(“/images/add.png”)));
button.addActionListener(new ActionListener() {
public void actionPerformed(ActionEvent arg0) {
addGoodsActionPerformed(arg0);
}
});
JButton button_1 = new JButton(“重置”);
button_1.setIcon(new ImageIcon(GoodsAddInterFrm.class.getResource(“/images/reset.png”)));
button_1.addActionListener(new ActionListener() {
public void actionPerformed(ActionEvent e) {
resetValueActionPerformed(e);
}
});
GroupLayout groupLayout = new GroupLayout(getContentPane());
groupLayout.setHorizontalGroup(groupLayout.createParallelGroup(Alignment.LEADING).addGroup(groupLayout
.createSequentialGroup()
.addGroup(groupLayout.createParallelGroup(Alignment.LEADING)
.addGroup(groupLayout.createSequentialGroup().addGap(45)
.addGroup(groupLayout.createParallelGroup(Alignment.LEADING)
.addGroup(groupLayout
.createSequentialGroup().addComponent(label_2).addGap(
.addComponent(goodsDescTxt, GroupLayout.PREFERRED_SIZE, 346,
GroupLayout.PREFERRED_SIZE))
.addGroup(groupLayout.createSequentialGroup().addGroup(groupLayout
.createParallelGroup(Alignment.LEADING, false)
.addGroup(groupLayout.createSequentialGroup().addComponent(label_1)
.addPreferredGap(ComponentPlacement.RELATED).addComponent(
goodsTypeNameJcb, 0, GroupLayout.DEFAULT_SIZE,
Short.MAX_VALUE))
.addGroup(groupLayout.createSequentialGroup().addComponent(lblNewLabel)
.addPreferredGap(ComponentPlacement.RELATED)
.addComponent(goodsNameTxt, GroupLayout.PREFERRED_SIZE, 99,
GroupLayout.PREFERRED_SIZE))
.addGroup(groupLayout.createSequentialGroup().addComponent(label)
.addPreferredGap(ComponentPlacement.UNRELATED)
.addComponent(manJrb)
.addPreferredGap(ComponentPlacement.UNRELATED)
.addComponent(femaleJrb)))
.addGap(65)
.addGroup(groupLayout.createParallelGroup(Alignment.LEADING, false)
.addGroup(groupLayout
.createSequentialGroup().addComponent(lblNewLabel_1)
.addPreferredGap(ComponentPlacement.RELATED)
.addComponent(goodsSupplierTxt,
GroupLayout.PREFERRED_SIZE, 102,
GroupLayout.PREFERRED_SIZE))
.addGroup(groupLayout.createSequentialGroup()
.addComponent(lblNewLabel_2).addGap(18)
.addComponent(priceTxt))))))
.addGroup(groupLayout.createSequentialGroup().addGap(149).addComponent(button).addGap(119)
.addComponent(button_1)))
.addContainerGap(126, Short.MAX_VALUE)));
groupLayout.setVerticalGroup(groupLayout.createParallelGroup(Alignment.LEADING)
.addGroup(groupLayout.createSequentialGroup().addGap(38)
.addGroup(groupLayout.createParallelGroup(Alignment.BASELINE).addComponent(lblNewLabel)
.addComponent(goodsNameTxt, GroupLayout.PREFERRED_SIZE, GroupLayout.DEFAULT_SIZE,
GroupLayout.PREFERRED_SIZE)
.addComponent(lblNewLabel_1).addComponent(goodsSupplierTxt, GroupLayout.PREFERRED_SIZE,
GroupLayout.DEFAULT_SIZE, GroupLayout.PREFERRED_SIZE))
.addGap(28)
.addGroup(groupLayout.createParallelGroup(Alignment.BASELINE).addComponent(label)
.addComponent(manJrb).addComponent(femaleJrb).addComponent(lblNewLabel_2)
.addComponent(priceTxt, GroupLayout.PREFERRED_SIZE, GroupLayout.DEFAULT_SIZE,
GroupLayout.PREFERRED_SIZE))
.addGap(28)
.addGroup(groupLayout.createParallelGroup(Alignment.BASELINE).addComponent(label_1)
.addComponent(goodsTypeNameJcb, GroupLayout.PREFERRED_SIZE, GroupLayout.DEFAULT_SIZE,
GroupLayout.PREFERRED_SIZE))
.addGroup(groupLayout.createParallelGroup(Alignment.LEADING)
.addGroup(groupLayout.createSequentialGroup().addGap(35).addComponent(label_2))
.addGroup(groupLayout.createSequentialGroup().addGap(36).addComponent(goodsDescTxt,
GroupLayout.PREFERRED_SIZE, 83, GroupLayout.PREFERRED_SIZE)))
.addPreferredGap(ComponentPlacement.RELATED, 25, Short.MAX_VALUE).addGroup(groupLayout
.createParallelGroup(Alignment.BASELINE).addComponent(button_1).addComponent(button))
.addGap(22)));
getContentPane().setLayout(groupLayout);
// 填充下拉列表
this.fillGoodsType();
}
/**
-
货物添加事件
-
@param arg0
*/
private void addGoodsActionPerformed(ActionEvent arg0) {
String goodsName = this.goodsNameTxt.getText();
String goodsSupplier = this.goodsSupplierTxt.getText();
String price = this.priceTxt.getText();
String goodsDesc = this.goodsDescTxt.getText();
if (StringUtil.isEmpty(goodsName)) {
JOptionPane.showMessageDialog(null, “货物名称不能为空!”);
return;
}
if (StringUtil.isEmpty(goodsSupplier)) {
JOptionPane.showMessageDialog(null, “货物供货商不能为空!”);
return;
}
if (StringUtil.isEmpty(goodsDesc)) {
JOptionPane.showMessageDialog(null, “货物描述不能为空!”);
return;
}
if (StringUtil.isEmpty(price)) {
JOptionPane.showMessageDialog(null, “货物价格不能为空!”);
return;
}
String sex = “”;
if (manJrb.isSelected()) {
sex = “男”;
} else if (femaleJrb.isSelected()) {
sex = “女”;
}
GoodsType goodsTypeName = (GoodsType) this.goodsTypeNameJcb.getSelectedItem();
int goodsTypeId = goodsTypeName.getId();
String goodsTypeNames = goodsTypeName.getGoodsTypeName();
Goods goods = new Goods(goodsName, goodsSupplier, sex, Double.parseDouble(price), goodsDesc, goodsTypeId,goodsTypeNames);
Connection conn = null;
try {
conn = DbUtil.getCon();
int result = goodsDao.addGoods(conn, goods);
if (result == 1) {
JOptionPane.showMessageDialog(null, “货物添加成功!”);
this.resetValue();
} else {
JOptionPane.showMessageDialog(null, “货物添加失败!”);
}
} catch (Exception e) {
e.printStackTrace();
} finally {
try {
dbUtil.close(conn);
} catch (SQLException e) {
e.printStackTrace();
}
}
}
/**
-
货物表单重置事件
-
@param e
*/
private void resetValueActionPerformed(ActionEvent e) {
this.resetValue();
}
/**
- 表单重置事件
*/
private void resetValue() {
this.goodsNameTxt.setText(“”);
this.goodsSupplierTxt.setText(“”);
this.priceTxt.setText(“”);
this.goodsDescTxt.setText(“”);
this.manJrb.setSelected(true);
if (goodsTypeNameJcb.getItemCount() > 0) {
goodsTypeNameJcb.setSelectedIndex(0);
}
}
/**
- 填充下拉列表货物类型
*/
private void fillGoodsType() {
Connection conn = null;
GoodsType goodsType = null;
ResultSet rs = null;
try {
conn = DbUtil.getCon();
rs = GoodsTypeDao.listGoodsType(conn, new GoodsType());
while (rs.next()) {
goodsType = new GoodsType();
goodsType.setId(rs.getInt(“id”));
goodsType.setGoodsTypeName(rs.getString(“goodsTypeName”));
this.goodsTypeNameJcb.addItem(goodsType);
}
} catch (Exception e) {
e.printStackTrace();
} finally {
try {
DbUtil.close(conn, rs);
} catch (SQLException e) {
e.printStackTrace();
}
}
}
/**
- 运行程序
*/
public static void main(String[] args) {
EventQueue.invokeLater(new Runnable() {
public void run() {
try {
GoodsAddInterFrm frame = new GoodsAddInterFrm();
frame.setVisible(true);
} catch (Exception e) {
e.printStackTrace();
}
}
});
}
}
package com.sjsq.view;
import java.awt.EventQueue;
import java.awt.event.ActionEvent;
import java.awt.event.ActionListener;
import java.awt.event.MouseAdapter;
import java.awt.event.MouseEvent;
import java.sql.Connection;
import java.sql.ResultSet;
import java.sql.SQLException;
import java.util.Vector;
import javax.swing.ButtonGroup;
import javax.swing.GroupLayout;
import javax.swing.GroupLayout.Alignment;
import javax.swing.ImageIcon;
import javax.swing.JButton;
import javax.swing.JComboBox;
import javax.swing.JInternalFrame;
import javax.swing.JLabel;
import javax.swing.JOptionPane;
import javax.swing.JPanel;
import javax.swing.JRadioButton;
import javax.swing.JScrollPane;
import javax.swing.JTable;
import javax.swing.JTextArea;
import javax.swing.JTextField;
import javax.swing.LayoutStyle.ComponentPlacement;
import javax.swing.border.TitledBorder;
import javax.swing.table.DefaultTableModel;
import com.sjsq.dao.GoodsDao;
import com.sjsq.dao.GoodsTypeDao;
import com.sjsq.model.Goods;
import com.sjsq.model.GoodsType;
import com.sjsq.util.DbUtil;
import com.sjsq.util.StringUtil;
/**
-
货物管理视图层
-
@author shuijianshiqing
*/
public class GoodsManagerInterFrm extends JInternalFrame {
private JTable goodsTable;
private JTextField s_goodsNameTxt;
private JTextField s_goodsSupplierTxt;
private JTextField goodsIdTxt;
private JTextField goodsNameTxt;
private JTextField priceTxt;
private JTextField goodsSupplierTxt;
private JTextArea goodsDescTxt;
private JComboBox goodsTypeNameJcb;
private JRadioButton manJrb;
private JRadioButton femaleJrb;
private JComboBox s_goodsTypeNameJcbTxt;
private final ButtonGroup buttonGroup = new ButtonGroup();
private static DbUtil dbUtil = new DbUtil();
private static GoodsDao goodsDao = new GoodsDao();
private static GoodsTypeDao goodsTypeDao = new GoodsTypeDao();
/**
- 创建窗体
*/
public GoodsManagerInterFrm() {
setTitle(“货物物品管理”);
setIconifiable(true);
setClosable(true);
setBounds(100, 100, 732, 532);
JScrollPane scrollPane = new JScrollPane();
JPanel panel = new JPanel();
panel.setBorder(
new TitledBorder(null, “货物物品添加”, TitledBorder.LEADING, TitledBorder.TOP, null, null));
// 搜索部分
JLabel label = new JLabel(“货物名称:”);
s_goodsNameTxt = new JTextField();
s_goodsNameTxt.setColumns(10);
JLabel label_1 = new JLabel(“供货商:”);
s_goodsSupplierTxt = new JTextField();
s_goodsSupplierTxt.setColumns(10);
JLabel label_2 = new JLabel(“货物类别:”);
s_goodsTypeNameJcbTxt = new JComboBox();
JButton button = new JButton(“搜索:”);
button.addActionListener(new ActionListener() {
public void actionPerformed(ActionEvent arg0) {
searchGoodsActionPerformed(arg0);
}
});
button.setIcon(new ImageIcon(GoodsManagerInterFrm.class.getResource(“/images/Search.png”)));
GroupLayout gl_panel = new GroupLayout(panel);
gl_panel.setHorizontalGroup(gl_panel.createParallelGroup(Alignment.LEADING).addGroup(gl_panel
.createSequentialGroup().addContainerGap().addComponent(label)
.addPreferredGap(ComponentPlacement.RELATED)
.addComponent(s_goodsNameTxt, GroupLayout.PREFERRED_SIZE, 87, GroupLayout.PREFERRED_SIZE).addGap(30)
.addComponent(label_1).addPreferredGap(ComponentPlacement.RELATED)
.addComponent(s_goodsSupplierTxt, GroupLayout.PREFERRED_SIZE, 86, GroupLayout.PREFERRED_SIZE).addGap(18)
.addComponent(label_2).addPreferredGap(ComponentPlacement.RELATED)
.addComponent(s_goodsTypeNameJcbTxt, GroupLayout.PREFERRED_SIZE, 119, GroupLayout.PREFERRED_SIZE)
.addPreferredGap(ComponentPlacement.RELATED, 22, Short.MAX_VALUE).addComponent(button)
.addContainerGap()));
gl_panel.setVerticalGroup(
gl_panel.createParallelGroup(Alignment.LEADING)
.addGroup(gl_panel.createSequentialGroup().addContainerGap()
.addGroup(gl_panel.createParallelGroup(Alignment.BASELINE).addComponent(label)
.addComponent(s_goodsNameTxt, GroupLayout.PREFERRED_SIZE,
GroupLayout.DEFAULT_SIZE, GroupLayout.PREFERRED_SIZE)
.addComponent(label_1)
.addComponent(s_goodsSupplierTxt, GroupLayout.PREFERRED_SIZE,
GroupLayout.DEFAULT_SIZE, GroupLayout.PREFERRED_SIZE)
.addComponent(label_2)
.addComponent(s_goodsTypeNameJcbTxt, GroupLayout.PREFERRED_SIZE,
GroupLayout.DEFAULT_SIZE, GroupLayout.PREFERRED_SIZE)
.addComponent(button))
.addContainerGap(17, Short.MAX_VALUE)));
panel.setLayout(gl_panel);
// 修改部分
JPanel panel_1 = new JPanel();
panel_1.setBorder(
new TitledBorder(null, “货物物品修改”, TitledBorder.LEADING, TitledBorder.TOP, null, null));
GroupLayout groupLayout = new GroupLayout(getContentPane());
groupLayout.setHorizontalGroup(groupLayout.createParallelGroup(Alignment.TRAILING)
.addGroup(groupLayout.createSequentialGroup().addGap(31)
.addGroup(groupLayout.createParallelGroup(Alignment.LEADING)
.addComponent(panel_1, Alignment.TRAILING, GroupLayout.DEFAULT_SIZE,
GroupLayout.DEFAULT_SIZE, Short.MAX_VALUE)
.addGroup(Alignment.TRAILING,
groupLayout.createParallelGroup(Alignment.LEADING, false)
.addComponent(panel, GroupLayout.DEFAULT_SIZE, GroupLayout.DEFAULT_SIZE,
Short.MAX_VALUE)
.addComponent(scrollPane, GroupLayout.DEFAULT_SIZE, 631,
Short.MAX_VALUE)))
.addGap(40)));
groupLayout.setVerticalGroup(groupLayout.createParallelGroup(Alignment.LEADING)
.addGroup(groupLayout.createSequentialGroup().addGap(23)
.addComponent(panel, GroupLayout.PREFERRED_SIZE, 66, GroupLayout.PREFERRED_SIZE).addGap(18)
.addComponent(scrollPane, GroupLayout.PREFERRED_SIZE, 119, GroupLayout.PREFERRED_SIZE)
.addGap(18).addComponent(panel_1, GroupLayout.DEFAULT_SIZE, 249, Short.MAX_VALUE)
.addContainerGap()));
JLabel lblNewLabel = new JLabel(“货物名称:”);
goodsIdTxt = new JTextField();
goodsIdTxt.setEnabled(false);
goodsIdTxt.setColumns(10);
JLabel label_3 = new JLabel(“货物名称:”);
goodsNameTxt = new JTextField();
goodsNameTxt.setColumns(10);
JLabel label_4 = new JLabel(“供货商性别:”);
manJrb = new JRadioButton(“男”);
buttonGroup.add(manJrb);
manJrb.setSelected(true);
femaleJrb = new JRadioButton(“女”);
buttonGroup.add(femaleJrb);
JLabel lblNewLabel_1 = new JLabel(“\u8D27\u7269\u4EF7\u683C\uFF1A”);
priceTxt = new JTextField();
priceTxt.setColumns(10);
JLabel label_5 = new JLabel(“货物价格:”);
goodsSupplierTxt = new JTextField();
goodsSupplierTxt.setColumns(10);
JLabel label_6 = new JLabel(“供货商:”);
goodsTypeNameJcb = new JComboBox();
JLabel label_7 = new JLabel(“货物类别:”);
goodsDescTxt = new JTextArea();
JButton button_1 = new JButton(“修改”);
button_1.addActionListener(new ActionListener() {
public void actionPerformed(ActionEvent arg0) {
modifyGoodsActionPerformed(arg0);
}
});
button_1.setIcon(new ImageIcon(GoodsManagerInterFrm.class.getResource(“/images/modify.png”)));
JButton button_2 = new JButton(“重置”);
button_2.addActionListener(new ActionListener() {
public void actionPerformed(ActionEvent arg0) {
resetValueActionPerformed(arg0);
}
});
button_2.setIcon(new ImageIcon(GoodsManagerInterFrm.class.getResource(“/images/reset.png”)));
JButton button_3 = new JButton(“删除”);
button_3.addActionListener(new ActionListener() {
public void actionPerformed(ActionEvent arg0) {
deleteGoodsActionPerformed(arg0);
}
});
button_3.setIcon(new ImageIcon(GoodsManagerInterFrm.class.getResource(“/images/delete.png”)));
GroupLayout gl_panel_1 = new GroupLayout(panel_1);
gl_panel_1.setHorizontalGroup(gl_panel_1.createParallelGroup(Alignment.LEADING).addGroup(gl_panel_1
.createSequentialGroup().addContainerGap()
.addGroup(gl_panel_1.createParallelGroup(Alignment.LEADING).addGroup(gl_panel_1.createSequentialGroup()
.addGroup(gl_panel_1.createParallelGroup(Alignment.LEADING).addGroup(gl_panel_1
.createSequentialGroup().addComponent(lblNewLabel)
.addPreferredGap(ComponentPlacement.RELATED)
.addComponent(goodsIdTxt, GroupLayout.PREFERRED_SIZE, 53, GroupLayout.PREFERRED_SIZE)
.addGap(26).addComponent(label_3).addPreferredGap(ComponentPlacement.RELATED)
.addComponent(goodsNameTxt, GroupLayout.PREFERRED_SIZE, 111,
GroupLayout.PREFERRED_SIZE))
.addGroup(gl_panel_1.createSequentialGroup().addComponent(lblNewLabel_1)
.addPreferredGap(ComponentPlacement.RELATED)
.addComponent(priceTxt, GroupLayout.PREFERRED_SIZE, 84,
GroupLayout.PREFERRED_SIZE)
.addGap(26).addComponent(label_5).addPreferredGap(ComponentPlacement.RELATED)
.addComponent(goodsSupplierTxt, GroupLayout.PREFERRED_SIZE, 112,
GroupLayout.PREFERRED_SIZE)))
.addGap(50)
.addGroup(gl_panel_1.createParallelGroup(Alignment.LEADING)
.addGroup(gl_panel_1.createSequentialGroup().addComponent(label_4)
.addPreferredGap(ComponentPlacement.UNRELATED).addComponent(manJrb).addGap(18)
.addComponent(femaleJrb))
.addGroup(gl_panel_1.createSequentialGroup().addComponent(label_6).addGap(18)
.addComponent(goodsTypeNameJcb, GroupLayout.PREFERRED_SIZE, 127,
GroupLayout.PREFERRED_SIZE))))
.addComponent(label_7).addComponent(goodsDescTxt, Alignment.TRAILING,
GroupLayout.PREFERRED_SIZE, 518, GroupLayout.PREFERRED_SIZE))
.addContainerGap(30, Short.MAX_VALUE))
.addGroup(gl_panel_1.createSequentialGroup().addGap(93).addComponent(button_1)
.addPreferredGap(ComponentPlacement.RELATED, 128, Short.MAX_VALUE).addComponent(button_2)
.addGap(112).addComponent(button_3).addGap(69)));
gl_panel_1.setVerticalGroup(gl_panel_1.createParallelGroup(Alignment.LEADING)
.addGroup(gl_panel_1
.createSequentialGroup().addGap(
.addGroup(gl_panel_1.createParallelGroup(Alignment.BASELINE).addComponent(lblNewLabel)
.addComponent(goodsIdTxt, GroupLayout.PREFERRED_SIZE, GroupLayout.DEFAULT_SIZE,
GroupLayout.PREFERRED_SIZE)
.addComponent(label_3)
.addComponent(goodsNameTxt, GroupLayout.PREFERRED_SIZE, GroupLayout.DEFAULT_SIZE,
GroupLayout.PREFERRED_SIZE)
.addComponent(femaleJrb).addComponent(manJrb).addComponent(label_4))
.addGap(18)
.addGroup(gl_panel_1.createParallelGroup(Alignment.BASELINE).addComponent(lblNewLabel_1)
.addComponent(priceTxt, GroupLayout.PREFERRED_SIZE, GroupLayout.DEFAULT_SIZE,
GroupLayout.PREFERRED_SIZE)
.addComponent(label_5)
.addComponent(goodsSupplierTxt, GroupLayout.PREFERRED_SIZE, GroupLayout.DEFAULT_SIZE,
GroupLayout.PREFERRED_SIZE)
.addComponent(label_6).addComponent(goodsTypeNameJcb, GroupLayout.PREFERRED_SIZE,
GroupLayout.DEFAULT_SIZE, GroupLayout.PREFERRED_SIZE))
.addGap(18)
.addGroup(gl_panel_1.createParallelGroup(Alignment.LEADING).addComponent(label_7)
.addComponent(goodsDescTxt, GroupLayout.PREFERRED_SIZE, 62, GroupLayout.PREFERRED_SIZE))
.addPreferredGap(ComponentPlacement.RELATED, 29, Short.MAX_VALUE)
.addGroup(gl_panel_1.createParallelGroup(Alignment.BASELINE).addComponent(button_1)
.addComponent(button_3).addComponent(button_2))
.addContainerGap()));
panel_1.setLayout(gl_panel_1);
goodsTable = new JTable();
goodsTable.addMouseListener(new MouseAdapter() {
@Override
public void mousePressed(MouseEvent arg0) {
MouseClickGoodsTableActionPerformed(arg0);
}
});
goodsTable.setModel(new DefaultTableModel(new Object[][] {},
new String[] { “货物编号”, “货物名称”,“供货商”, “性别”,
“货物价格”, “货物描述”, “货物类别” }) {
boolean[] columnEditables = new boolean[] { false, false, false, false, false, false, false };
public boolean isCellEditable(int row, int column) {
return columnEditables[column];
}
});
scrollPane.setViewportView(goodsTable);
getContentPane().setLayout(groupLayout);
// 填充表单
this.fillGoodsTable(new Goods());
this.fillGoodsTypeNameItem(“search”);
this.fillGoodsTypeNameItem(“modify”);
}
/**
-
货物删除事件
-
@param arg0
*/
private void deleteGoodsActionPerformed(ActionEvent arg0) {
String id = this.goodsIdTxt.getText();
if (StringUtil.isEmpty(id)) {
JOptionPane.showMessageDialog(null, “请选择要删除的货物”);
return;
}
int n = JOptionPane.showConfirmDialog(null, “确定要删除此货物?”);
Goods goods = new Goods(Integer.parseInt(id));
if (n == 0) {
Connection conn = null;
try {
conn = dbUtil.getCon();
int result = goodsDao.deleteGoods(conn, goods);
if (result == 1) {
JOptionPane.showMessageDialog(null, “货物删除成功!”);
this.resetValue();
this.fillGoodsTable(new Goods());
} else {
JOptionPane.showMessageDialog(null, “货物删除失败!”);
}
} catch (Exception e) {
e.printStackTrace();
JOptionPane.showMessageDialog(null, “货物删除失败!”);
} finally {
try {
dbUtil.close(conn);
} catch (SQLException e) {
e.printStackTrace();
}
}
}
}
/**
-
货物修改事件
-
@param a
*/
private void modifyGoodsActionPerformed(Object a) {
String id = this.goodsIdTxt.getText();
String goodsName = this.goodsNameTxt.getText();
String price = this.priceTxt.getText();
String goodsSupplier = this.goodsSupplierTxt.getText();
String goodsDesc = this.goodsDescTxt.getText();
if (StringUtil.isEmpty(id)) {
JOptionPane.showMessageDialog(null, “请选择要修改的货物”);
return;
}
if (StringUtil.isEmpty(goodsName)) {
JOptionPane.showMessageDialog(null, “货物名称不能为空!”);
return;
}
if (StringUtil.isEmpty(price)) {
JOptionPane.showMessageDialog(null, “货物价格不能为空!”);
return;
}
if (StringUtil.isEmpty(goodsSupplier)) {
JOptionPane.showMessageDialog(null, “供货商名称不能为空!”);
return;
}
if (StringUtil.isEmpty(goodsDesc)) {
JOptionPane.showMessageDialog(null, “货物描述不能为空!”);
return;
}
String sex = “”;
if (manJrb.isSelected()) {
sex = “男”;
} else if (femaleJrb.isSelected()) {
sex = “女”;
}
GoodsType goodsType = (GoodsType) this.goodsTypeNameJcb.getSelectedItem();
int goodsTypeId = goodsType.getId();
Goods goods = new Goods(Integer.parseInt(id), goodsName, goodsSupplier, sex, Double.parseDouble(price),
goodsDesc, goodsTypeId);
Connection conn = null;
try {
conn = DbUtil.getCon();
int result = GoodsDao.updateGoods(conn, goods);
if (result == 1) {
JOptionPane.showMessageDialog(null, “货物修改成功!”);
this.fillGoodsTable(new Goods());
} else {
JOptionPane.showMessageDialog(null, “货物修改失败!”);
}
} catch (Exception e) {
e.printStackTrace();
JOptionPane.showMessageDialog(null, “货物修改失败!”);
} finally {
try {
dbUtil.close(conn);
} catch (SQLException e) {
e.printStackTrace();
}
}
}
/**
-
货物搜索事件
-
@param arg0
*/
private void searchGoodsActionPerformed(ActionEvent arg0) {
String goodsName = this.s_goodsNameTxt.getText();
String goodsSupplier = this.s_goodsSupplierTxt.getText();
GoodsType goodsType = (GoodsType) this.s_goodsTypeNameJcbTxt.getSelectedItem();
int goodsTypeId = goodsType.getId();
Goods goods = new Goods(goodsName, goodsSupplier, goodsTypeId);
this.fillGoodsTable(goods);
}
/**
-
填充下拉列表菜单
-
@param type
*/
private void fillGoodsTypeNameItem(String type) {
Connection conn = null;
GoodsType goodsType = null;
ResultSet rs = null;
try {
conn = dbUtil.getCon();
rs = goodsTypeDao.listGoodsType(conn, new GoodsType());
if (“search”.equals(type)) {
goodsType = new GoodsType();
goodsType.setGoodsTypeName(“请选择…”);
goodsType.setId(-1);
this.s_goodsTypeNameJcbTxt.addItem(goodsType);
}
while (rs.next()) {
goodsType = new GoodsType();
goodsType.setId(rs.getInt(“id”));
goodsType.setGoodsTypeName(rs.getString(“goodsTypeName”));
if (“search”.equals(type)) {
this.s_goodsTypeNameJcbTxt.addItem(goodsType);
} else if (“modify”.equals(type)) {
this.goodsTypeNameJcb.addItem(goodsType);
}
}
} catch (Exception e) {
e.printStackTrace();
} finally {
try {
dbUtil.close(conn, rs);
} catch (SQLException e) {
e.printStackTrace();
}
}
}
/**
-
填充表单事件
-
@param goods
*/
private void fillGoodsTable(Goods goods) {
DefaultTableModel dtm = (DefaultTableModel) goodsTable.getModel();
dtm.setRowCount(0);
Connection conn = null;
ResultSet rs = null;
try {
conn = DbUtil.getCon();
自我介绍一下,小编13年上海交大毕业,曾经在小公司待过,也去过华为、OPPO等大厂,18年进入阿里一直到现在。
深知大多数Java工程师,想要提升技能,往往是自己摸索成长或者是报班学习,但对于培训机构动则几千的学费,着实压力不小。自己不成体系的自学效果低效又漫长,而且极易碰到天花板技术停滞不前!
因此收集整理了一份《2024年Java开发全套学习资料》,初衷也很简单,就是希望能够帮助到想自学提升又不知道该从何学起的朋友,同时减轻大家的负担。
既有适合小白学习的零基础资料,也有适合3年以上经验的小伙伴深入学习提升的进阶课程,基本涵盖了95%以上Java开发知识点,真正体系化!
由于文件比较大,这里只是将部分目录截图出来,每个节点里面都包含大厂面经、学习笔记、源码讲义、实战项目、讲解视频,并且会持续更新!
如果你觉得这些内容对你有帮助,可以扫码获取!!(备注Java获取)
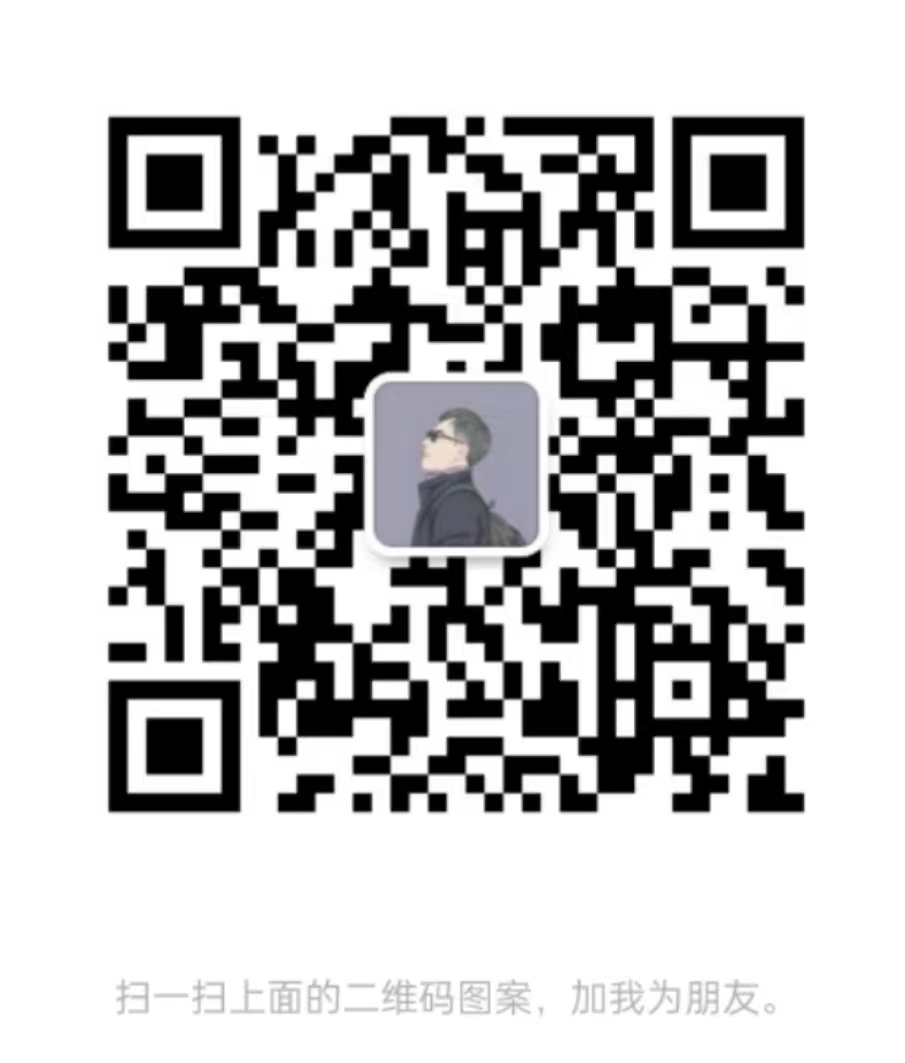
最后
ActiveMQ消息中间件面试专题
- 什么是ActiveMQ?
- ActiveMQ服务器宕机怎么办?
- 丢消息怎么办?
- 持久化消息非常慢怎么办?
- 消息的不均匀消费怎么办?
- 死信队列怎么办?
- ActiveMQ中的消息重发时间间隔和重发次数吗?
ActiveMQ消息中间件面试专题解析拓展:
redis面试专题及答案
- 支持一致性哈希的客户端有哪些?
- Redis与其他key-value存储有什么不同?
- Redis的内存占用情况怎么样?
- 都有哪些办法可以降低Redis的内存使用情况呢?
- 查看Redis使用情况及状态信息用什么命令?
- Redis的内存用完了会发生什么?
- Redis是单线程的,如何提高多核CPU的利用率?
Spring面试专题及答案
- 谈谈你对 Spring 的理解
- Spring 有哪些优点?
- Spring 中的设计模式
- 怎样开启注解装配以及常用注解
- 简单介绍下 Spring bean 的生命周期
Spring面试答案解析拓展
高并发多线程面试专题
- 现在有线程 T1、T2 和 T3。你如何确保 T2 线程在 T1 之后执行,并且 T3 线程在 T2 之后执行?
- Java 中新的 Lock 接口相对于同步代码块(synchronized block)有什么优势?如果让你实现一个高性能缓存,支持并发读取和单一写入,你如何保证数据完整性。
- Java 中 wait 和 sleep 方法有什么区别?
- 如何在 Java 中实现一个阻塞队列?
- 如何在 Java 中编写代码解决生产者消费者问题?
- 写一段死锁代码。你在 Java 中如何解决死锁?
高并发多线程面试解析与拓展
jvm面试专题与解析
- JVM 由哪些部分组成?
- JVM 内存划分?
- Java 的内存模型?
- 引用的分类?
- GC什么时候开始?
JVM面试专题解析与拓展!
《互联网大厂面试真题解析、进阶开发核心学习笔记、全套讲解视频、实战项目源码讲义》点击传送门即可获取!
;
}
/**
-
填充下拉列表菜单
-
@param type
*/
private void fillGoodsTypeNameItem(String type) {
Connection conn = null;
GoodsType goodsType = null;
ResultSet rs = null;
try {
conn = dbUtil.getCon();
rs = goodsTypeDao.listGoodsType(conn, new GoodsType());
if (“search”.equals(type)) {
goodsType = new GoodsType();
goodsType.setGoodsTypeName(“请选择…”);
goodsType.setId(-1);
this.s_goodsTypeNameJcbTxt.addItem(goodsType);
}
while (rs.next()) {
goodsType = new GoodsType();
goodsType.setId(rs.getInt(“id”));
goodsType.setGoodsTypeName(rs.getString(“goodsTypeName”));
if (“search”.equals(type)) {
this.s_goodsTypeNameJcbTxt.addItem(goodsType);
} else if (“modify”.equals(type)) {
this.goodsTypeNameJcb.addItem(goodsType);
}
}
} catch (Exception e) {
e.printStackTrace();
} finally {
try {
dbUtil.close(conn, rs);
} catch (SQLException e) {
e.printStackTrace();
}
}
}
/**
-
填充表单事件
-
@param goods
*/
private void fillGoodsTable(Goods goods) {
DefaultTableModel dtm = (DefaultTableModel) goodsTable.getModel();
dtm.setRowCount(0);
Connection conn = null;
ResultSet rs = null;
try {
conn = DbUtil.getCon();
自我介绍一下,小编13年上海交大毕业,曾经在小公司待过,也去过华为、OPPO等大厂,18年进入阿里一直到现在。
深知大多数Java工程师,想要提升技能,往往是自己摸索成长或者是报班学习,但对于培训机构动则几千的学费,着实压力不小。自己不成体系的自学效果低效又漫长,而且极易碰到天花板技术停滞不前!
因此收集整理了一份《2024年Java开发全套学习资料》,初衷也很简单,就是希望能够帮助到想自学提升又不知道该从何学起的朋友,同时减轻大家的负担。[外链图片转存中…(img-tkKXgiCX-1713081966752)]
[外链图片转存中…(img-eSHBxjhp-1713081966752)]
[外链图片转存中…(img-PAz8m0kP-1713081966753)]
既有适合小白学习的零基础资料,也有适合3年以上经验的小伙伴深入学习提升的进阶课程,基本涵盖了95%以上Java开发知识点,真正体系化!
由于文件比较大,这里只是将部分目录截图出来,每个节点里面都包含大厂面经、学习笔记、源码讲义、实战项目、讲解视频,并且会持续更新!
如果你觉得这些内容对你有帮助,可以扫码获取!!(备注Java获取)
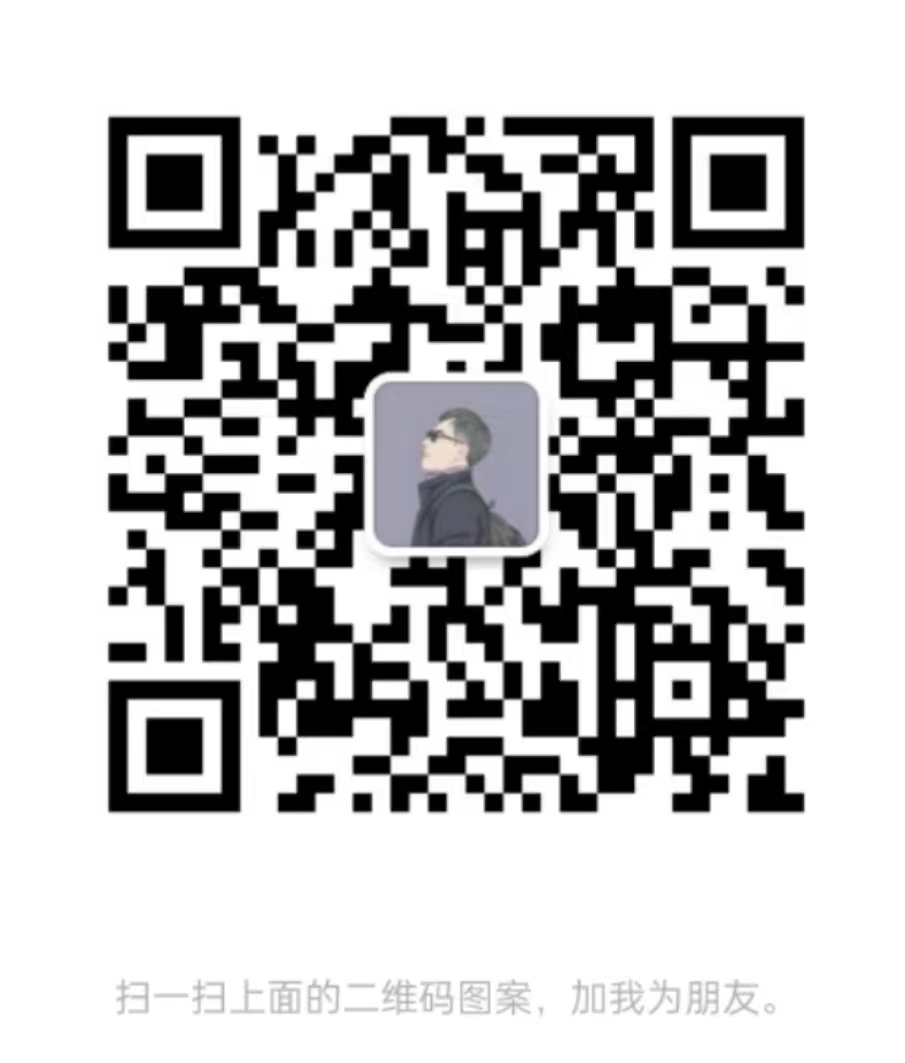
最后
ActiveMQ消息中间件面试专题
- 什么是ActiveMQ?
- ActiveMQ服务器宕机怎么办?
- 丢消息怎么办?
- 持久化消息非常慢怎么办?
- 消息的不均匀消费怎么办?
- 死信队列怎么办?
- ActiveMQ中的消息重发时间间隔和重发次数吗?
ActiveMQ消息中间件面试专题解析拓展:
[外链图片转存中…(img-wT6D6GIN-1713081966753)]
redis面试专题及答案
- 支持一致性哈希的客户端有哪些?
- Redis与其他key-value存储有什么不同?
- Redis的内存占用情况怎么样?
- 都有哪些办法可以降低Redis的内存使用情况呢?
- 查看Redis使用情况及状态信息用什么命令?
- Redis的内存用完了会发生什么?
- Redis是单线程的,如何提高多核CPU的利用率?
[外链图片转存中…(img-mbbp9R9Y-1713081966753)]
Spring面试专题及答案
- 谈谈你对 Spring 的理解
- Spring 有哪些优点?
- Spring 中的设计模式
- 怎样开启注解装配以及常用注解
- 简单介绍下 Spring bean 的生命周期
Spring面试答案解析拓展
[外链图片转存中…(img-5dj8BgfB-1713081966753)]
高并发多线程面试专题
- 现在有线程 T1、T2 和 T3。你如何确保 T2 线程在 T1 之后执行,并且 T3 线程在 T2 之后执行?
- Java 中新的 Lock 接口相对于同步代码块(synchronized block)有什么优势?如果让你实现一个高性能缓存,支持并发读取和单一写入,你如何保证数据完整性。
- Java 中 wait 和 sleep 方法有什么区别?
- 如何在 Java 中实现一个阻塞队列?
- 如何在 Java 中编写代码解决生产者消费者问题?
- 写一段死锁代码。你在 Java 中如何解决死锁?
高并发多线程面试解析与拓展
[外链图片转存中…(img-Kpz41zaS-1713081966754)]
jvm面试专题与解析
- JVM 由哪些部分组成?
- JVM 内存划分?
- Java 的内存模型?
- 引用的分类?
- GC什么时候开始?
JVM面试专题解析与拓展!
[外链图片转存中…(img-XPZ2HYFj-1713081966754)]
《互联网大厂面试真题解析、进阶开发核心学习笔记、全套讲解视频、实战项目源码讲义》点击传送门即可获取!