import com.mhys.crm.entity.TbEmp;
import com.mhys.crm.entity.Tb_emp_dept;
import java.util.List;
public interface TbEmpMapper {
int deleteByPrimaryKey(Integer empId);
int insert(TbEmp record);
TbEmp selectByPrimaryKey(Integer empId);
List selectAll();
int updateByPrimaryKey(TbEmp record);
//多表连查
List<Tb_emp_dept> selectEmp();
//模糊查询
List<Tb_emp_dept> selectEmpLike(Tb_emp_dept tb_emp_dept);
}
TbDeptMapper.xml
<?xml version="1.0" encoding="UTF-8" ?>delete from tb_dept
where dept_id = #{deptId,jdbcType=INTEGER}
insert into tb_dept (dept_id, dept_name, dept_address
)
values (#{deptId,jdbcType=INTEGER}, #{deptName,jdbcType=VARCHAR}, #{deptAddress,jdbcType=VARCHAR}
)
update tb_dept
set dept_name = #{deptName,jdbcType=VARCHAR},
dept_address = #{deptAddress,jdbcType=VARCHAR}
where dept_id = #{deptId,jdbcType=INTEGER}
select dept_id, dept_name, dept_address
from tb_dept
where dept_id = #{deptId,jdbcType=INTEGER}
select dept_id, dept_name, dept_address
from tb_dept
TbEmpMapper.xml
<?xml version="1.0" encoding="UTF-8" ?>delete from tb_emp
where emp_id = #{empId,jdbcType=INTEGER}
insert into tb_emp (emp_id, emp_name, emp_position,
emp_in_date, emp_salary, dept_id
)
values (#{empId,jdbcType=INTEGER}, #{empName,jdbcType=VARCHAR}, #{empPosition,jdbcType=VARCHAR},
#{empInDate,jdbcType=DATE}, #{empSalary,jdbcType=REAL}, #{deptId,jdbcType=INTEGER}
)
update tb_emp
set emp_name = #{empName,jdbcType=VARCHAR},
emp_position = #{empPosition,jdbcType=VARCHAR},
emp_in_date = #{empInDate,jdbcType=DATE},
emp_salary = #{empSalary,jdbcType=REAL},
dept_id = #{deptId,jdbcType=INTEGER}
where emp_id = #{empId,jdbcType=INTEGER}
select emp_id, emp_name, emp_position, emp_in_date, emp_salary, dept_id
from tb_emp
where emp_id = #{empId,jdbcType=INTEGER}
select emp_id, emp_name, emp_position, emp_in_date, emp_salary, dept_id
from tb_emp
SELECT emp_id, emp_name, emp_position, emp_in_date, emp_salary,dept_name,
dept_address FROM tb_emp e,tb_dept d WHERE e.dept_id=d.dept_id
SELECT emp_id, emp_name, emp_position, emp_in_date, emp_salary,dept_name,
dept_address FROM tb_emp e LEFT JOIN tb_dept d on e.dept_id=d.dept_id WHERE emp_position LIKE “%”#{empPosition}“%”
and emp_name like “%”#{empName}“%”
com.mhys.crm.entity【实体的包】
Tb_emp_dept.java
package com.mhys.crm.entity;
import java.util.Date;
public class Tb_emp_dept {
private Integer empId;
private String empName;
private String empPosition;
private Date empInDate;
private Float empSalary;
private Integer deptId;
private String deptName;
private String deptAddress;
public Integer getEmpId() {
return empId;
}
public void setEmpId(Integer empId) {
this.empId = empId;
}
public String getEmpName() {
return empName;
}
public void setEmpName(String empName) {
this.empName = empName;
}
public String getEmpPosition() {
return empPosition;
}
public void setEmpPosition(String empPosition) {
this.empPosition = empPosition;
}
public Date getEmpInDate() {
return empInDate;
}
public void setEmpInDate(Date empInDate) {
this.empInDate = empInDate;
}
public Float getEmpSalary() {
return empSalary;
}
public void setEmpSalary(Float empSalary) {
this.empSalary = empSalary;
}
public Integer getDeptId() {
return deptId;
}
public void setDeptId(Integer deptId) {
this.deptId = deptId;
}
public String getDeptName() {
return deptName;
}
public void setDeptName(String deptName) {
this.deptName = deptName;
}
public String getDeptAddress() {
return deptAddress;
}
public void setDeptAddress(String deptAddress) {
this.deptAddress = deptAddress;
}
public Tb_emp_dept(Integer empId, String empName, String empPosition, Date empInDate, Float empSalary,
Integer deptId, String deptName, String deptAddress) {
super();
this.empId = empId;
this.empName = empName;
this.empPosition = empPosition;
this.empInDate = empInDate;
this.empSalary = empSalary;
this.deptId = deptId;
this.deptName = deptName;
this.deptAddress = deptAddress;
}
public Tb_emp_dept() {
super();
}
@Override
public String toString() {
return “Tb_emp_dept [empId=” + empId + “, empName=” + empName + “, empPosition=” + empPosition + “, empInDate=”
-
empInDate + “, empSalary=” + empSalary + “, deptId=” + deptId + “, deptName=” + deptName
-
“, deptAddress=” + deptAddress + “]”;
}
}
TbDept.java
package com.mhys.crm.entity;
public class TbDept {
private Integer deptId;
private String deptName;
private String deptAddress;
public Integer getDeptId() {
return deptId;
}
public void setDeptId(Integer deptId) {
this.deptId = deptId;
}
public String getDeptName() {
return deptName;
}
public void setDeptName(String deptName) {
this.deptName = deptName == null ? null : deptName.trim();
}
public String getDeptAddress() {
return deptAddress;
}
public void setDeptAddress(String deptAddress) {
this.deptAddress = deptAddress == null ? null : deptAddress.trim();
}
}
TbEmp.java
package com.mhys.crm.entity;
import java.util.Date;
public class TbEmp {
private Integer empId;
private String empName;
private String empPosition;
private String empInDate;
private Float empSalary;
private Integer deptId;
public Integer getEmpId() {
return empId;
}
public void setEmpId(Integer empId) {
this.empId = empId;
}
public String getEmpName() {
return empName;
}
public void setEmpName(String empName) {
this.empName = empName == null ? null : empName.trim();
}
public String getEmpPosition() {
return empPosition;
}
public void setEmpPosition(String empPosition) {
this.empPosition = empPosition == null ? null : empPosition.trim();
}
public String getEmpInDate() {
return empInDate;
}
public void setEmpInDate(String empInDate) {
this.empInDate = empInDate;
}
public Float getEmpSalary() {
return empSalary;
}
public void setEmpSalary(Float empSalary) {
this.empSalary = empSalary;
}
public Integer getDeptId() {
return deptId;
}
public void setDeptId(Integer deptId) {
this.deptId = deptId;
}
}
com.mhys.crm.service【业务处理类】
Tb_empService.java
package com.mhys.crm.service;
import java.util.List;
import com.mhys.crm.entity.TbEmp;
import com.mhys.crm.entity.Tb_emp_dept;
public interface Tb_empService {
//查询
List<Tb_emp_dept> selectAll(Tb_emp_dept tb_emp_dept);
//添加
int add(TbEmp tbEmp);
int delete(int id);
}
com.mhys.crm.service.impl【业务处理的实现类】
Tb_empServiceImpl.java
package com.mhys.crm.service.impl;
import java.util.List;
import javax.annotation.Resource;
import org.springframework.stereotype.Service;
import com.mhys.crm.dao.TbEmpMapper;
import com.mhys.crm.entity.TbEmp;
import com.mhys.crm.entity.Tb_emp_dept;
import com.mhys.crm.service.Tb_empService;
@Service
public class Tb_empServiceImpl implements Tb_empService {
@Resource
private TbEmpMapper mapper;
@Override
public List<Tb_emp_dept> selectAll(Tb_emp_dept tb_emp_dept) {
// TODO Auto-generated method stub
System.out.println(tb_emp_dept.getEmpName());
System.out.println(tb_emp_dept.getEmpPosition());
if (tb_emp_dept.getEmpName().equals(“”)&&tb_emp_dept.getEmpPosition().equals(“”)) {
List<Tb_emp_dept> list=mapper.selectEmp();
return list;
}else {
List<Tb_emp_dept> list=mapper.selectEmpLike(tb_emp_dept);
return list;
}
}
@Override
public int add(TbEmp tbEmp) {
// TODO Auto-generated method stub
int rows=mapper.insert(tbEmp);
return rows;
}
@Override
public int delete(int id) {
// TODO Auto-generated method stub
return mapper.deleteByPrimaryKey(id);
}
}
genter【实体类自动生成包】
Tb_empController.java
package genter;
import java.io.IOException;
import java.io.InputStream;
import java.sql.SQLException;
import java.util.ArrayList;
import java.util.List;
import org.mybatis.generator.api.MyBatisGenerator;
import org.mybatis.generator.config.Configuration;
import org.mybatis.generator.config.xml.ConfigurationParser;
import org.mybatis.generator.exception.InvalidConfigurationException;
import org.mybatis.generator.exception.XMLParserException;
import org.mybatis.generator.internal.DefaultShellCallback;
public class Generator {
/*
- targetRuntime=“MyBatis3Simple”, 不生成Example
*/
public void generateMyBatis() {
//MBG执行过程中的警告信息
List warnings = new ArrayList();
//当生成的代码重复时,覆盖原代码
boolean overwrite = true ;
String generatorFile = “/generatorConfig.xml”;
//String generatorFile = “/generator/generatorConfigExample.xml”;
//读取MBG配置文件
InputStream is = Generator.class.getResourceAsStream(generatorFile);
ConfigurationParser cp = new ConfigurationParser(warnings);
Configuration config;
try {
config = cp.parseConfiguration(is);
DefaultShellCallback callback = new DefaultShellCallback(overwrite);
//创建MBG
MyBatisGenerator myBatisGenerator = new MyBatisGenerator(config, callback, warnings);
//执行生成代码
myBatisGenerator.generate(null);
} catch (IOException e) {
e.printStackTrace();
} catch (XMLParserException e) {
e.printStackTrace();
} catch (InvalidConfigurationException e) {
e.printStackTrace();
} catch (SQLException e) {
e.printStackTrace();
} catch (InterruptedException e) {
e.printStackTrace();
}
for (String warning : warnings) {
System.out.println(warning);
}
}
public static void main(String[] args) {
Generator generator = new Generator();
generator.generateMyBatis();
}
}
generatorConfig.xml
<?xml version="1.0" encoding="UTF-8"?><jdbcConnection driverClass=“com.mysql.jdbc.Driver”
connectionURL=“jdbc:mysql://localhost:3306/cqsw”
userId=“root” password=“123456”>
<javaClientGenerator targetPackage=“com.mhys.crm.dao” type=“XMLMAPPER”
targetProject=“src”/>
resource
mybatis
SqlMapConfig.xml
<?xml version="1.0" encoding="UTF-8" ?>spring
applicationContext-dao.xml
<?xml version="1.0" encoding="UTF-8"?><beans xmlns=“http://www.springframework.org/schema/beans”
xmlns:context=“http://www.springframework.org/schema/context” xmlns:p=“http://www.springframework.org/schema/p”
xmlns:aop=“http://www.springframework.org/schema/aop” xmlns:tx=“http://www.springframework.org/schema/tx”
xmlns:xsi=“http://www.w3.org/2001/XMLSchema-instance”
xsi:schemaLocation="http://www.springframework.org/schema/beans http://www.springframework.org/schema/beans/spring-beans-4.0.xsd
http://www.springframework.org/schema/context http://www.springframework.org/schema/context/spring-context-4.0.xsd
http://www.springframework.org/schema/aop http://www.springframework.org/schema/aop/spring-aop-4.0.xsd http://www.springframework.org/schema/tx http://www.springframework.org/schema/tx/spring-tx-4.0.xsd
http://www.springframework.org/schema/util http://www.springframework.org/schema/util/spring-util-4.0.xsd">
<context:property-placeholder location=“classpath:database.properties” />
applicationContext-service.xml
<?xml version="1.0" encoding="UTF-8"?><beans xmlns=“http://www.springframework.org/schema/beans”
xmlns:context=“http://www.springframework.org/schema/context” xmlns:p=“http://www.springframework.org/schema/p”
xmlns:aop=“http://www.springframework.org/schema/aop” xmlns:tx=“http://www.springframework.org/schema/tx”
xmlns:xsi=“http://www.w3.org/2001/XMLSchema-instance”
xsi:schemaLocation="http://www.springframework.org/schema/beans http://www.springframework.org/schema/beans/spring-beans-4.0.xsd
http://www.springframework.org/schema/context http://www.springframework.org/schema/context/spring-context-4.0.xsd
http://www.springframework.org/schema/aop http://www.springframework.org/schema/aop/spring-aop-4.0.xsd http://www.springframework.org/schema/tx http://www.springframework.org/schema/tx/spring-tx-4.0.xsd
http://www.springframework.org/schema/util http://www.springframework.org/schema/util/spring-util-4.0.xsd">
<context:component-scan base-package=“com.mhys.crm.service” />
<tx:annotation-driven transaction-manager=“transactionManager” />
spring-mvc.xml
<?xml version="1.0" encoding="UTF-8"?><beans xmlns=“http://www.springframework.org/schema/beans”
xmlns:xsi=“http://www.w3.org/2001/XMLSchema-instance” xmlns:p=“http://www.springframework.org/schema/p”
xmlns:context=“http://www.springframework.org/schema/context”
xmlns:mvc=“http://www.springframework.org/schema/mvc”
xsi:schemaLocation="http://www.springframework.org/schema/beans http://www.springframework.org/schema/beans/spring-beans-4.0.xsd
http://www.springframework.org/schema/mvc http://www.springframework.org/schema/mvc/spring-mvc-4.0.xsd
http://www.springframework.org/schema/context http://www.springframework.org/schema/context/spring-context-4.0.xsd">
<context:component-scan base-package=“com.mhys.crm.controller” />
<mvc:annotation-driven />
WebContent
jsp
index.jsp
<%@ page language=“java” contentType=“text/html; charset=utf-8”
pageEncoding=“utf-8”%>
account.jsp
<%@ page language=“java” contentType=“text/html; charset=utf-8”
pageEncoding=“utf-8”%>
<%@ taglib prefix=“c” uri=“http://java.sun.com/jsp/jstl/core” %>
<%@ taglib prefix=“fmt” uri=“http://java.sun.com/jsp/jstl/fmt”%>
人事管理系统
搜索
深知大多数Java工程师,想要提升技能,往往是自己摸索成长或者是报班学习,但对于培训机构动则几千的学费,着实压力不小。自己不成体系的自学效果低效又漫长,而且极易碰到天花板技术停滞不前!
因此收集整理了一份《2024年Java开发全套学习资料》,初衷也很简单,就是希望能够帮助到想自学提升又不知道该从何学起的朋友,同时减轻大家的负担。
既有适合小白学习的零基础资料,也有适合3年以上经验的小伙伴深入学习提升的进阶课程,基本涵盖了95%以上Java开发知识点,真正体系化!
由于文件比较大,这里只是将部分目录截图出来,每个节点里面都包含大厂面经、学习笔记、源码讲义、实战项目、讲解视频,并且会持续更新!
如果你觉得这些内容对你有帮助,可以扫码获取!!(备注Java获取)
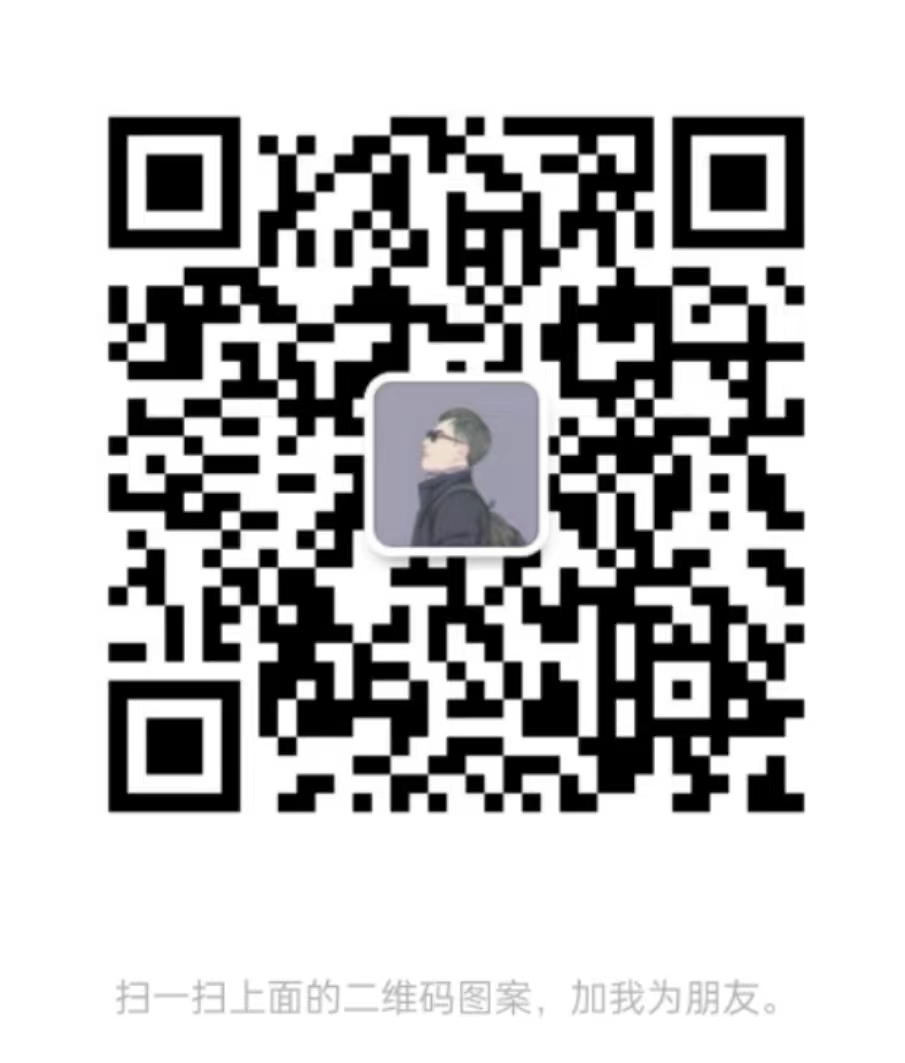
最后
整理的这些资料希望对Java开发的朋友们有所参考以及少走弯路,本文的重点是你有没有收获与成长,其余的都不重要,希望读者们能谨记这一点。
其实面试这一块早在第一个说的25大面试专题就全都有的。以上提及的这些全部的面试+学习的各种笔记资料,我这差不多来回搞了三个多月,收集整理真的很不容易,其中还有很多自己的一些知识总结。正是因为很麻烦,所以对以上这些学习复习资料感兴趣
《互联网大厂面试真题解析、进阶开发核心学习笔记、全套讲解视频、实战项目源码讲义》点击传送门即可获取!
m{
width:90%;
padding:10px;
margin: 0 auto;
}
.bottom{
width:90%;
padding:10px;
margin: 0 auto;
}
h1{
text-align:center;
}
tr:hover{
box-shadow: #8f8fff 10px 10px 30px 5px ;
background: #a1a4b5;
}
.form-group{
padding: 20px;
}
人事管理系统
搜索
深知大多数Java工程师,想要提升技能,往往是自己摸索成长或者是报班学习,但对于培训机构动则几千的学费,着实压力不小。自己不成体系的自学效果低效又漫长,而且极易碰到天花板技术停滞不前!
因此收集整理了一份《2024年Java开发全套学习资料》,初衷也很简单,就是希望能够帮助到想自学提升又不知道该从何学起的朋友,同时减轻大家的负担。[外链图片转存中…(img-RLcCACaa-1713609491008)]
[外链图片转存中…(img-8t4kZFvb-1713609491009)]
[外链图片转存中…(img-34LwaTVw-1713609491009)]
既有适合小白学习的零基础资料,也有适合3年以上经验的小伙伴深入学习提升的进阶课程,基本涵盖了95%以上Java开发知识点,真正体系化!
由于文件比较大,这里只是将部分目录截图出来,每个节点里面都包含大厂面经、学习笔记、源码讲义、实战项目、讲解视频,并且会持续更新!
如果你觉得这些内容对你有帮助,可以扫码获取!!(备注Java获取)
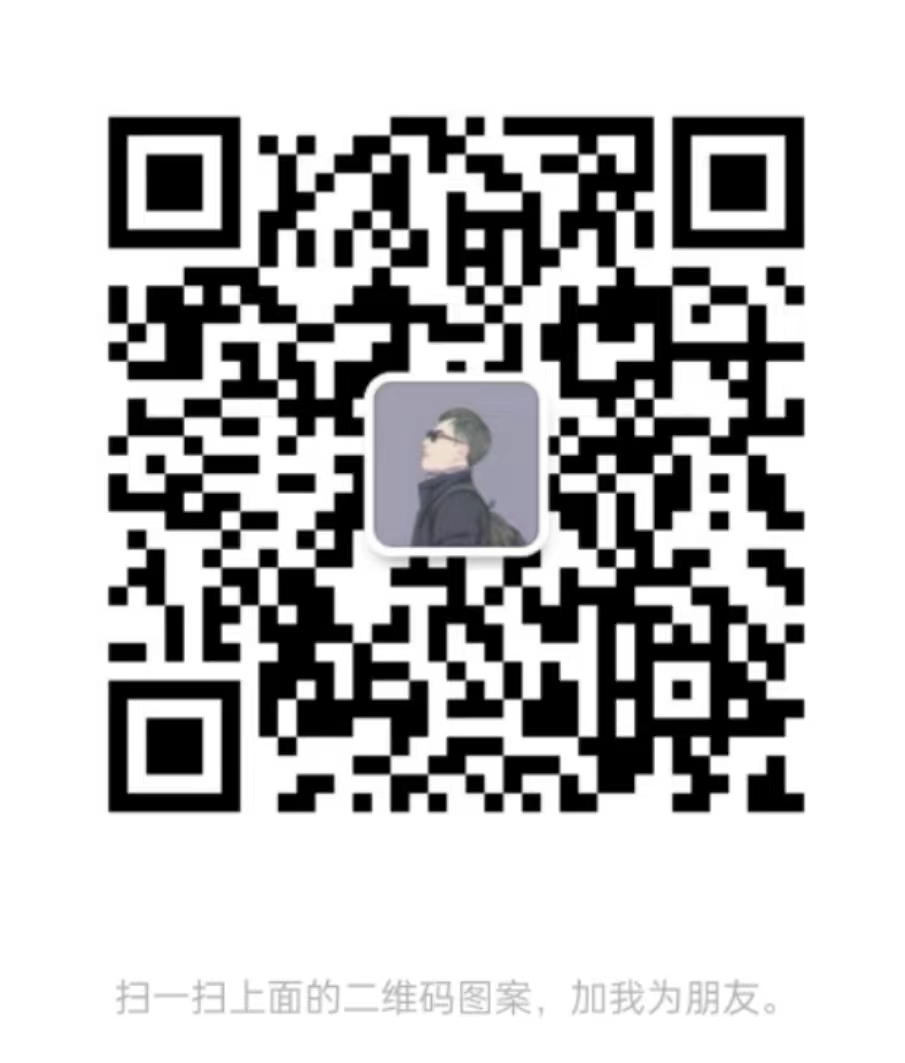
最后
整理的这些资料希望对Java开发的朋友们有所参考以及少走弯路,本文的重点是你有没有收获与成长,其余的都不重要,希望读者们能谨记这一点。
[外链图片转存中…(img-HbAeWXLZ-1713609491010)]
[外链图片转存中…(img-0RVT1MUq-1713609491010)]
其实面试这一块早在第一个说的25大面试专题就全都有的。以上提及的这些全部的面试+学习的各种笔记资料,我这差不多来回搞了三个多月,收集整理真的很不容易,其中还有很多自己的一些知识总结。正是因为很麻烦,所以对以上这些学习复习资料感兴趣
《互联网大厂面试真题解析、进阶开发核心学习笔记、全套讲解视频、实战项目源码讲义》点击传送门即可获取!