bank
目录结构
Bank
JAR包:
src
com.controller
BankController.java
package com.controller;
import java.util.List;
import javax.annotation.Resource;
import org.springframework.stereotype.Controller;
import org.springframework.ui.Model;
import org.springframework.web.bind.annotation.RequestMapping;
import org.springframework.web.servlet.ModelAndView;
import com.dao.TAccountMapper;
import com.entity.TAccount;
@Controller
public class BankController {
@Resource
TAccountMapper tAccountMapper;
//主界面表格显示
@RequestMapping(“/BankList”)
public ModelAndView MainList(String name) {
List selectAll = tAccountMapper.selectAll(name);
ModelAndView modelAndView = new ModelAndView();
modelAndView.addObject(“selectAll”, selectAll);
modelAndView.setViewName(“bankList”);
return modelAndView;
}
//删除
@RequestMapping(“/delList”)
public String delList(int id) {
int deleteByPrimaryKey = tAccountMapper.deleteByPrimaryKey(id);
if (deleteByPrimaryKey>0) {
return “redirect:/BankList.do”;
}
return “redirect:/BankList.do”;
}
//跳转修改界面的方法
@RequestMapping(“/updListT”)
public String updListT(Model model,int id) {
List select = tAccountMapper.selectByPrimaryKey(id);
model.addAttribute(“select”, select);
return “updList”;
}
//修改
@RequestMapping(“/updList”)
public String updList(TAccount account,Model model) {
int updateByPrimaryKey = tAccountMapper.updateByPrimaryKey(account);
if (updateByPrimaryKey>0) {
return “redirect:/BankList.do”;
}
return “redirect:/BankList.do”;
}
//跳转添加界面
@RequestMapping(“/addListT”)
public String addList() {
return “addList”;
}
//添加
@RequestMapping(“/addList”)
public String addList(TAccount account,Model model) {
int insert = tAccountMapper.insert(account);
if (insert>0) {
return “redirect:/BankList.do”;
}
return “redirect:/BankList.do”;
}
}
com.dao
TAccountMapper.java
package com.dao;
import java.util.List;
import org.apache.ibatis.annotations.Param;
import com.entity.TAccount;
public interface TAccountMapper {
int deleteByPrimaryKey(Integer id);
int insert(TAccount record);
List selectByPrimaryKey(Integer id);
List selectAll(@Param(“name”)String name);
int updateByPrimaryKey(TAccount record);
}
TAccountMapper.xml
<?xml version="1.0" encoding="UTF-8" ?>delete from t_account
where id = #{id,jdbcType=INTEGER}
insert into t_account (id, number, name,
money)
values (#{id,jdbcType=INTEGER}, #{number,jdbcType=VARCHAR}, #{name,jdbcType=VARCHAR},
#{money,jdbcType=DECIMAL})
update t_account
set number = #{number,jdbcType=VARCHAR},
name = #{name,jdbcType=VARCHAR},
money = #{money,jdbcType=DECIMAL}
where id = #{id,jdbcType=INTEGER}
select id, number, name, money
from t_account
where id = #{id}
select id, number, name, money
from t_account
name like “%”#{name}“%”
com.entity
TAccount.java
package com.entity;
import java.math.BigDecimal;
public class TAccount {
private Integer id;
private String number;
private String name;
private BigDecimal money;
public Integer getId() {
return id;
}
public void setId(Integer id) {
this.id = id;
}
public String getNumber() {
return number;
}
public void setNumber(String number) {
this.number = number == null ? null : number.trim();
}
public String getName() {
return name;
}
public void setName(String name) {
this.name = name == null ? null : name.trim();
}
public BigDecimal getMoney() {
return money;
}
public void setMoney(BigDecimal money) {
this.money = money;
}
}
mybatis
sqlMapConfig.xml
<?xml version="1.0" encoding="UTF-8"?>spring
applicationContext-dao.xml
<?xml version="1.0" encoding="UTF-8"?><beans xmlns:xsi=“http://www.w3.org/2001/XMLSchema-instance”
xmlns=“http://www.springframework.org/schema/beans”
xmlns:p=“http://www.springframework.org/schema/p”
xmlns:context=“http://www.springframework.org/schema/context”
xmlns:aop=“http://www.springframework.org/schema/aop”
xmlns:tx=“http://www.springframework.org/schema/tx”
xsi:schemaLocation="http://www.springframework.org/schema/beans
http://www.springframework.org/schema/beans/spring-beans-4.2.xsd
http://www.springframework.org/schema/context
http://www.springframework.org/schema/context/spring-context-4.2.xsd
http://www.springframework.org/schema/aop
http://www.springframework.org/schema/aop/spring-aop-4.2.xsd
http://www.springframework.org/schema/tx
http://www.springframework.org/schema/tx/spring-tx.xsd
http://www.springframework.org/schema/mvc
http://www.springframework.org/schema/mvc/spring-mvc-4.2.xsd ">
<context:property-placeholder location=“classpath:jdbc.properties”></context:property-placeholder>
<tx:annotation-driven transaction-manager=“transactionManager” />
spring-mvc.xml
<?xml version="1.0" encoding="UTF-8"?><beans xmlns:xsi=“http://www.w3.org/2001/XMLSchema-instance”
xmlns=“http://www.springframework.org/schema/beans”
xmlns:context=“http://www.springframework.org/schema/context”
xmlns:aop=“http://www.springframework.org/schema/aop”
xmlns:tx=“http://www.springframework.org/schema/tx”
xmlns:mvc=“http://www.springframework.org/schema/mvc”
xsi:schemaLocation="http://www.springframework.org/schema/beans
http://www.springframework.org/schema/beans/spring-beans-4.2.xsd
http://www.springframework.org/schema/context
http://www.springframework.org/schema/context/spring-context-4.2.xsd
http://www.springframework.org/schema/aop
http://www.springframework.org/schema/aop/spring-aop-4.2.xsd
http://www.springframework.org/schema/tx
http://www.springframework.org/schema/tx/spring-tx-4.2.xsd
http://www.springframework.org/schema/mvc
http://www.springframework.org/schema/mvc/spring-mvc-4.2.xsd ">
<context:component-scan base-package=“com.controller” />
<mvc:annotation-driven />
jdbc.properties
jdbc.url=jdbc:mysql://localhost:3306/bank?useUnicode=true&characterEncoding=UTF-8&useSSL=false
jdbc.username=root
jdbc.password=123456
jdbc.driver=com.mysql.jdbc.Driver
WebContent
web.xml
<?xml version="1.0" encoding="UTF-8"?>Bank
index.html
index.htm
index.jsp
default.html
default.htm
default.jsp
contextConfigLocation
classpath:spring/applicationContext-*.xml
org.springframework.web.context.ContextLoaderListener
springmvc
org.springframework.web.servlet.DispatcherServlet
contextConfigLocation
classpath:spring/spring-mvc.xml
1
springmvc
*.do
CharacterEncodingFilter
org.springframework.web.filter.CharacterEncodingFilter
encoding
UTF-8
CharacterEncodingFilter
/*
JSP
index.jsp
<%@ page language=“java” import=“java.util.*” pageEncoding=“UTF-8”%>
<%
String path = request.getContextPath();
String basePath = request.getScheme()+“😕/”+request.getServerName()+“:”+request.getServerPort()+path;
%>
addList.jsp
<%@ page language=“java” contentType=“text/html; charset=utf-8”
pageEncoding=“utf-8”%>
添加信息
placeholder=“请输入姓名” name=“name” id=“” value=“” />
卡号:placeholder=“请输入卡号” name=“number” id=“” value=“” />
余额:placeholder=“请输入余额” name=“money” id=“” value=“” />
<input
style=“width: 100px; margin-top: 10px; padding: 5px; background-color: #EFEFEF;”
type=“submit” value=“添加” /><input
style=“width: 100px; margin-top: 10px; padding: 5px; background-color: #EFEFEF; margin-left: 10%”
type=“reset” value=“重置” />
bankList.jsp
自我介绍一下,小编13年上海交大毕业,曾经在小公司待过,也去过华为、OPPO等大厂,18年进入阿里一直到现在。
深知大多数Java工程师,想要提升技能,往往是自己摸索成长或者是报班学习,但对于培训机构动则几千的学费,着实压力不小。自己不成体系的自学效果低效又漫长,而且极易碰到天花板技术停滞不前!
因此收集整理了一份《2024年Java开发全套学习资料》,初衷也很简单,就是希望能够帮助到想自学提升又不知道该从何学起的朋友,同时减轻大家的负担。
既有适合小白学习的零基础资料,也有适合3年以上经验的小伙伴深入学习提升的进阶课程,基本涵盖了95%以上Java开发知识点,真正体系化!
由于文件比较大,这里只是将部分目录截图出来,每个节点里面都包含大厂面经、学习笔记、源码讲义、实战项目、讲解视频,并且会持续更新!
如果你觉得这些内容对你有帮助,可以扫码获取!!(备注Java获取)
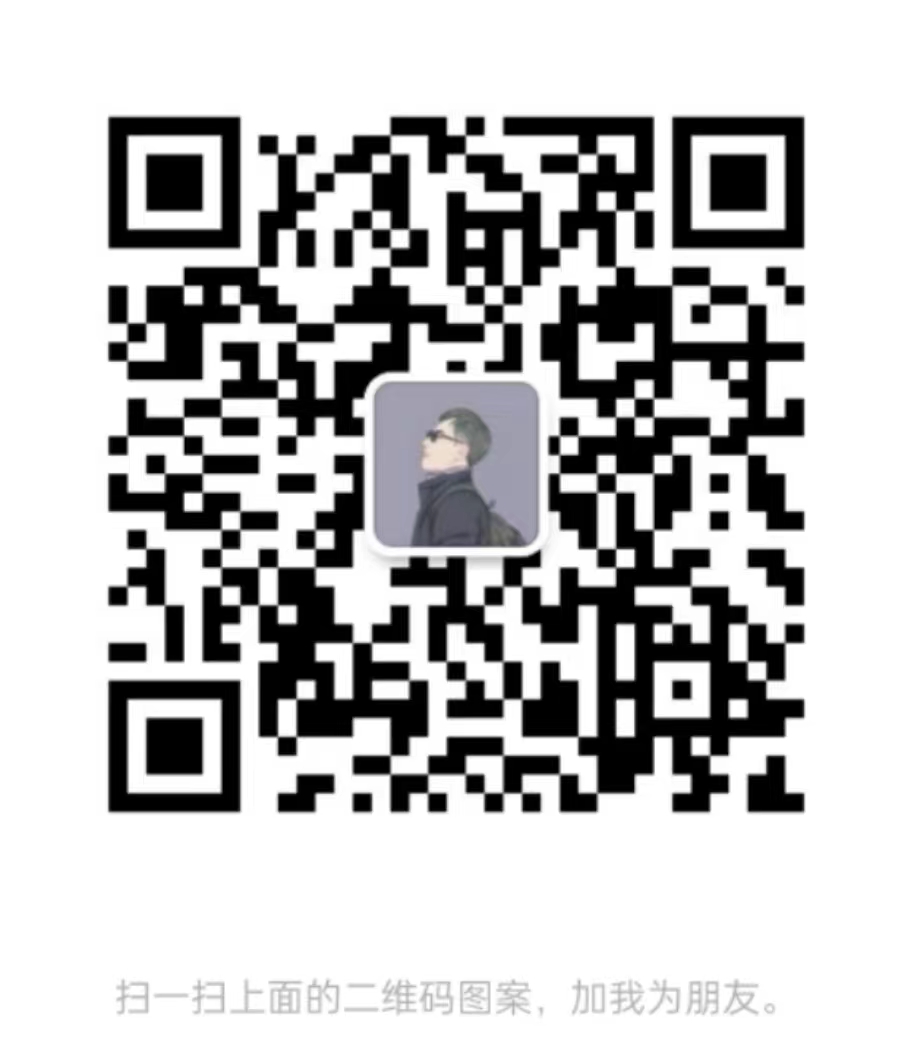
独家面经总结,超级精彩
本人面试腾讯,阿里,百度等企业总结下来的面试经历,都是真实的,分享给大家!
Java面试准备
准确的说这里又分为两部分:
- Java刷题
- 算法刷题
Java刷题:此份文档详细记录了千道面试题与详解;
《互联网大厂面试真题解析、进阶开发核心学习笔记、全套讲解视频、实战项目源码讲义》点击传送门即可获取!
理了一份《2024年Java开发全套学习资料》,初衷也很简单,就是希望能够帮助到想自学提升又不知道该从何学起的朋友,同时减轻大家的负担。**[外链图片转存中…(img-SIBjorpu-1713609694315)]
[外链图片转存中…(img-4PjmLbnw-1713609694315)]
[外链图片转存中…(img-fTfSl0AD-1713609694316)]
既有适合小白学习的零基础资料,也有适合3年以上经验的小伙伴深入学习提升的进阶课程,基本涵盖了95%以上Java开发知识点,真正体系化!
由于文件比较大,这里只是将部分目录截图出来,每个节点里面都包含大厂面经、学习笔记、源码讲义、实战项目、讲解视频,并且会持续更新!
如果你觉得这些内容对你有帮助,可以扫码获取!!(备注Java获取)
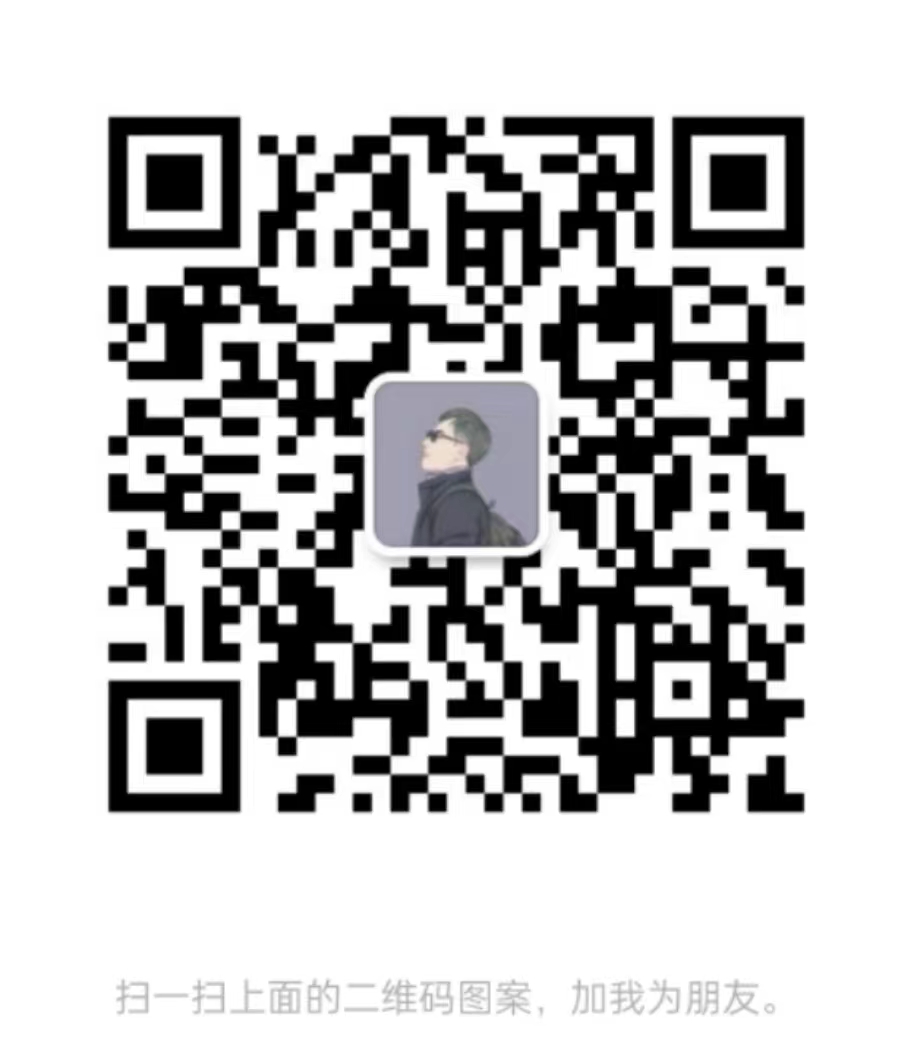
独家面经总结,超级精彩
本人面试腾讯,阿里,百度等企业总结下来的面试经历,都是真实的,分享给大家!
[外链图片转存中…(img-yWOPyT1I-1713609694316)]
[外链图片转存中…(img-imy67PCD-1713609694316)]
[外链图片转存中…(img-OzdUAi60-1713609694316)]
[外链图片转存中…(img-xV4ajXiX-1713609694316)]
Java面试准备
准确的说这里又分为两部分:
- Java刷题
- 算法刷题
Java刷题:此份文档详细记录了千道面试题与详解;
[外链图片转存中…(img-NeCodbDH-1713609694317)]
[外链图片转存中…(img-detE6eEk-1713609694317)]
《互联网大厂面试真题解析、进阶开发核心学习笔记、全套讲解视频、实战项目源码讲义》点击传送门即可获取!