安装RabbitMQ要注意,安装路径不能有中文,你的电脑,用户,起名千万不要起中文名.不然就会和我一样,重装.或者永远不装RabbitMQ.
还有就是安装RabbitMQ要装erlang,要注意好erlang和RabbitMQ的版本对应.
启动就是打开文件夹->sbin->server.bat就启动了
使用方法
当你需要异步执行一个任务时:
可以初始化一个tasks
app = Celery('tasks', broker='amqp://guest@localhost//') # 指定broker为rabbitmq
@app.task()
def print_task(x, y): # 一个任务
print(x + y)
启动命令:(打在terminal)
celery -A tasks worker -l INFO
celery -A tasks worker -l INFO -P solo -c 2
# 这个的意思是启动tasks示例 打印info日志 -P solo是pool solo执行池指定为solo -c 2是2个进程
# -P / --pool prefork(默认使用)、solo、eventlet、gevent
# 参数
# -A / --app 要使用的应用程序实例
# -n / --hostname 设置自定义主机名
# -Q / --queues 指定一个消息队列,该进程只接受此队列的任务
# –max-tasks-per-child 配置工作单元子进程在被一个新进程取代之前可以执行的最大任务数量
# –max-memory-per-child 设置工作单元子进程被替换之前可以使用的最大内存
# -l / --loglevel 定义打印log的等级 DEBUG, INFO, WARNING, ERROR, CRITICAL, FATAL
# –autoscale 池的进程的最大数量和最小数量
# -c / --concurrency 同时处理任务的工作进程数量,默认值是系统上可用的cpu数量
# -B / --beat 定义运行celery打周期任务调度程序
# -h / --help help!help!help!
# 也就是说这一行的意思是 启动tasks这个实例,打印info日志
# control + c可以退出
此时就可以异步执行print_task这个方法了,只需要
print_task.delay(x, y)
# 或者
print_task.apply_async([x, y])
delay和apply_async的应用差不多,是apply_async的简便版.
# .delay(_args, *_kwargs)等价于调用 .apply_async(args, kwargs)
task.delay(arg1, arg2, kwarg1='x', kwarg2='y')
# 等同于
task.apply_async(args=[arg1, arg2], kwargs={'kwarg1': 'x', 'kwarg2': 'y'})
# 但是delay不支持其他参数,apply_async支持很多
task.apply_async(countdown=10) # 现在开始10s后执行
task.apply_async(eta=now + timedelta(seconds=10)) # 10s后 指明eta
task.apply_async(countdown=60, expires=120) # 60s后, 120s后过期
task.apply_async(expires=now + timedelta(days=2)) # 指定2天后过期
定时任务
要启用beat
**celery -A tasks beat**
代码:
@app.on_after_configure.connect
def setup_periodic_tasks(sender, **kwargs):
# 每天早上7点00 执行print_task 参数为1, 2
sender.add_periodic_task(crontab(hour="7", minute="0"), print_task.s(1, 2),
name='print_task')
# minute="*/5" 每五分钟
感受
用下来感觉功能非常全面,但是启动真的很麻烦.
我要先打开rabbitmq
再打开监听
再打开beat
再写好任务
如果定时任务要等待时间
如果是异步我要delay
而且要装一堆东西
所以我查到了下面这个
flask-apscheduler
安装
**pip install flask-apscheduler**
介绍
适配于Flask框架 是APScheduler扩展 支持flask配置类加载定时任务调度器配置和任务配置明细 提供内部restful风格API监控管理定时任务、认证机制、host白名单访问机制 高度与flask蓝图集成
"""add_job(func, trigger=None, args=None, kwargs=None, id=None, \
name=None, misfire_grace_time=undefined, coalesce=undefined, \
max_instances=undefined, next_run_time=undefined, \
jobstore='default', executor='default', \
replace_existing=False, **trigger_args)
Adds the given job to the job list and wakes up the scheduler if it's already running.
Any option that defaults to ``undefined`` will be replaced with the corresponding default
value when the job is scheduled (which happens when the scheduler is started, or
immediately if the scheduler is already running).
The ``func`` argument can be given either as a callable object or a textual reference in
the ``package.module:some.object`` format, where the first half (separated by ``:``) is an
importable module and the second half is a reference to the callable object, relative to
the module.
The ``trigger`` argument can either be:
#. the alias name of the trigger (e.g. ``date``, ``interval`` or ``cron``), in which case
any extra keyword arguments to this method are passed on to the trigger's constructor
#. an instance of a trigger class
:param func: callable (or a textual reference to one) to run at the given time
:param str|apscheduler.triggers.base.BaseTrigger trigger: trigger that determines when
``func`` is called
:param list|tuple args: list of positional arguments to call func with
:param dict kwargs: dict of keyword arguments to call func with
:param str|unicode id: explicit identifier for the job (for modifying it later)
:param str|unicode name: textual description of the job
:param int misfire_grace_time: seconds after the designated runtime that the job is still
allowed to be run (or ``None`` to allow the job to run no matter how late it is)
:param bool coalesce: run once instead of many times if the scheduler determines that the
job should be run more than once in succession
:param int max_instances: maximum number of concurrently running instances allowed for this
job
:param datetime next_run_time: when to first run the job, regardless of the trigger (pass
``None`` to add the job as paused)
:param str|unicode jobstore: alias of the job store to store the job in
:param str|unicode executor: alias of the executor to run the job with
:param bool replace_existing: ``True`` to replace an existing job with the same ``id``
(but retain the number of runs from the existing one)
:rtype: Job
"""
"""
将给定的任务添加到任务列表中,并在调度程序已在运行时唤醒它。
当调度作业时,任何默认为“未定义”的选项都将替换为相应的默认值(当调度程序启动时,或当调度程序已在运行时立即发生)。
“func”参数可以作为中的可调用对象或文本引用给出
`package.module:some.object`格式,其中前半部分(用`:``分隔)是可导入模块,后半部分是对可调用对象的引用,相对于模块。
“触发器”参数可以是:
#.触发器的别名(例如“date”、“interval”或“cron”),在这种情况下
该方法的任何额外关键字参数都会传递给触发器的构造函数
#.触发器类的实例
:param func:可调用(或对函数的文本引用)以在给定时间运行
:param str | apscheduler.triggers.base.BaseTrigger trigger:确定何时的触发器
### 一、Python所有方向的学习路线
Python所有方向路线就是把Python常用的技术点做整理,形成各个领域的知识点汇总,它的用处就在于,你可以按照上面的知识点去找对应的学习资源,保证自己学得较为全面。
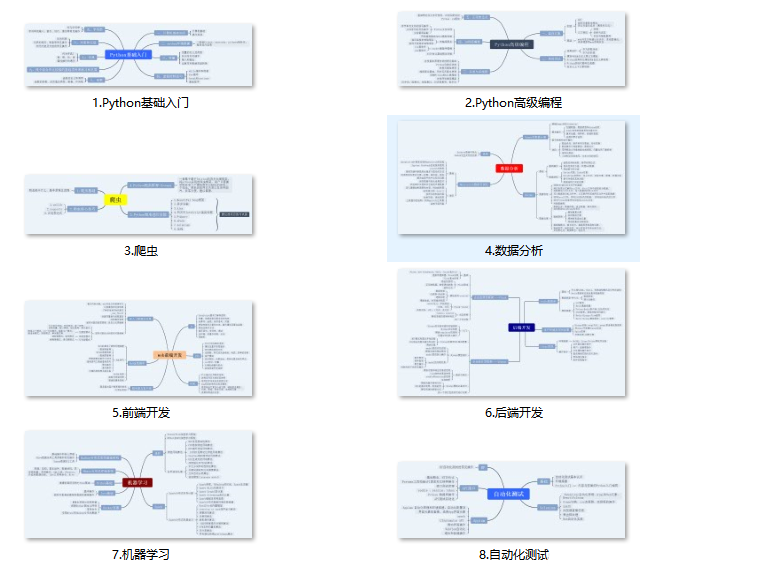
### 二、学习软件
工欲善其事必先利其器。学习Python常用的开发软件都在这里了,给大家节省了很多时间。
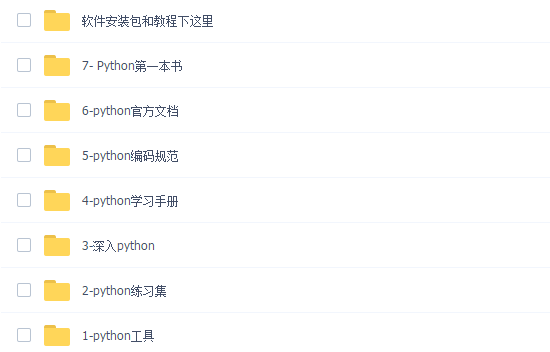
### 三、入门学习视频
我们在看视频学习的时候,不能光动眼动脑不动手,比较科学的学习方法是在理解之后运用它们,这时候练手项目就很适合了。
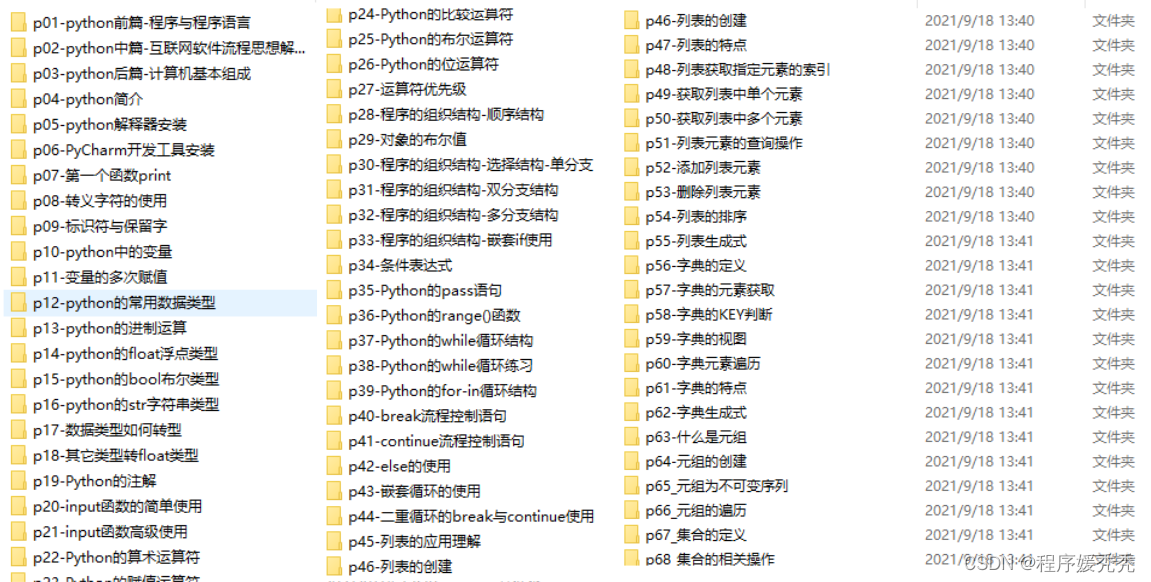
**网上学习资料一大堆,但如果学到的知识不成体系,遇到问题时只是浅尝辄止,不再深入研究,那么很难做到真正的技术提升。**
**[需要这份系统化学习资料的朋友,可以戳这里无偿获取](https://bbs.csdn.net/topics/618317507)**
**一个人可以走的很快,但一群人才能走的更远!不论你是正从事IT行业的老鸟或是对IT行业感兴趣的新人,都欢迎加入我们的的圈子(技术交流、学习资源、职场吐槽、大厂内推、面试辅导),让我们一起学习成长!**