if (spacePressed && timer > 5) {
initialiseGame();
setGameMode(gameModes.TITLE);
}
}
function processGifts() {
gifts.forEach((g) => {
if (g && g.alive) {
// draw gift
drawGift(g);
if (g.y > canvas.height) {
g.alive = false;
if (!g.bomb) score–;
}
// move gift
g.y+=g.speed;
// rotate gift
g.rotation+=5;
if ( g.rotation > 359) g.rotation = 0;
// check for collision
if ((g.y + (giftHeight/2)) >= ((canvas.height - elfHeight - snowHeight) + 20)
&& (g.y<canvas.height-snowHeight+20)) {
if ((elfX + 25) <= (g.x + (giftWidth/2)) && ((elfX+20) + (elfWidth)) >= g.x )
{
g.alive = false;
if (!g.bomb) {
score+=5;
} else {
doBombCollision();
}
}
}
}
});
}
function drawGift(g) {
switch (g.colour) {
case 1:
drawColouredGift(greenGiftImage, g);
break;
case 2:
drawColouredGift(redGiftImage, g);
break;
case 3:
drawColouredGift(blueGiftImage, g);
break;
case 4:
drawRotatedImage(bombImage, g.x, g.y, 180, 45);
break;
}
}
function drawColouredGift(colourImage, g) {
drawRotatedImage(colourImage, g.x, g.y, g.rotation, 35);
}
function doBombCollision() {
health–;
bangX=elfX;
bangTime = 5;
if (health == 0) {
setHighScore();
setGameMode(gameModes.GAMEOVER);
}
}
function drawBang() {
if (bangTime > 0) {
bangTime–;
ctx.drawImage(bangImage, bangX, (canvas.height-75)-snowHeight, 75,75);
}
}
function drawElf() {
ctx.drawImage(elfImage, elfX,(canvas.height - elfHeight) - (snowHeight - 2),80,80);
}
function spawn() {
var newX = 5 + (Math.random() * (canvas.width - 5));
var colour;
var bomb = false;
if (randomNumber(1,bombChance) == bombChance) {
colour = 4;
bomb = true;
} else {
colour = randomNumber(1,3);
}
var newGift = {
x: newX,
y: 0,
speed: randomNumber(2,6),
alive: true,
rotation: 0,
colour: colour,
bomb: bomb,
};
gifts[maxGift] = newGift;
maxGift++;
if (maxGift > 75) {
maxGift = 0;
}
}
function spawnGifts() {
if (timer > spawnTimer) {
spawn();
timer = 0;
}
}
function drawRotatedImage(image, x, y, angle, scale)
{
ctx.save();
ctx.translate(x, y);
ctx.rotate(angle * TO_RADIANS);
ctx.drawImage(image, -(image.width/2), -(image.height/2), scale, scale);
ctx.restore();
}
function drawHUD() {
ctx.font = “20px Arial”;
ctx.fillStyle = “yellow”;
ctx.fillText(Score: ${score}
, 0, 25);
var heart = ‘❤’;
var hearts = health > 0 ? heart.repeat(health) : " ";
ctx.fillText(“Helf:”, canvas.width - 120, 25);
ctx.fillStyle = “red”;
ctx.fillText(${hearts}
, canvas.width - 60, 26);
}
function initialiseGame() {
health = 3;
elfX = (canvas.width-elfWidth)/2;
bangTime = 0;
score = 0;
snowHeight = 6;
timer = 0;
spawnTimer = 50;
gifts = [];
}
function initialiseSnow() {
for (i=0; i<maxSnowflakes; i++) {
var startY = -randomNumber(0, canvas.height);
snowflakes[i] = {
x: randomNumber(0, canvas.width-snowflakeSize),
y: startY,
startY: startY,
colour: snowflakeColours[randomNumber(0,3)],
radius: (Math.random() * 3 + 1),
speed: randomNumber(snowflakeMinSpeed, snowflakeMaxSpeed)
};
}
}
function drawSnow() {
for (i=0; i<maxSnowflakes; i++) {
snowflakes[i].y+=snowflakes[i].speed;
if (snowflakes[i].y>canvas.height) snowflakes[i].y = snowflakes[i].startY;
ctx.beginPath();
ctx.arc(snowflakes[i].x, snowflakes[i].y, snowflakes[i].radius, 0, 2 * Math.PI, false);
ctx.fillStyle = snowflakes[i].colour;
ctx.fill();
}
}
function drawFloor() {
var snowTopY = canvas.height - snowHeight;
ctx.fillStyle = ‘#fff’;
ctx.beginPath();
ctx.moveTo(0, snowTopY);
ctx.lineTo(canvas.width, snowTopY);
ctx.lineTo(canvas.width, canvas.height);
ctx.lineTo(0, canvas.height);
ctx.closePath();
ctx.fill();
}
function drawSnowPerson() {
var snowTopY = canvas.height - snowHeight;
drawCircle(“#fff”, 100, snowTopY-20, 40);
drawCircle(“#fff”, 100, snowTopY-70, 20);
drawRectangle(“#835C3B”, 85, snowTopY-105, 30, 20);
drawRectangle(“#835C3B”, 75, snowTopY-90, 50, 6);
drawTriangle(“#ffa500”, 100, snowTopY-64, 7);
drawCircle(“#000”, 93, snowTopY-76, 3);
drawCircle(“#000”, 108, snowTopY-76, 3);
drawCircle(“#000”, 100, snowTopY-40, 2);
drawCircle(“#000”, 100, snowTopY-30, 2);
drawCircle(“#000”, 100, snowTopY-20, 2);
}
function drawTriangle(color, x, y, height) {
ctx.strokeStyle = ctx.fillStyle = color;
ctx.beginPath();
ctx.moveTo(x, y);
ctx.lineTo(x - height, y - height);
ctx.lineTo(x + height, y - height);
ctx.fill();
}
function drawCircle(color, x, y, radius) {
ctx.strokeStyle = ctx.fillStyle = color;
ctx.beginPath();
ctx.arc(x, y, radius, 0, Math.PI * 2, true);
ctx.closePath();
ctx.stroke();
ctx.fill();
}
function drawRectangle(color, x, y, width, height) {
ctx.strokeStyle = ctx.fillStyle = color;
ctx.fillRect(x, y, width, height);
}
function randomNumber(low, high) {
return Math.floor(Math.random() * high) + low;
}
function makeColorGradient(frequency1, frequency2, frequency3,
phase1, phase2, phase3,
center, width, len) {
var colours = [];
for (var i = 0; i < len; ++i)
{
var r = Math.sin(frequency1*i + phase1) * width + center;
var g = Math.sin(frequency2*i + phase2) * width + center;
var b = Math.sin(frequency3*i + phase3) * width + center;
colours.push(RGB2Color(r,g,b));
}
return colours;
}
function RGB2Color(r,g,b) {
return ‘#’ + byte2Hex® + byte2Hex(g) + byte2Hex(b);
}
function byte2Hex(n) {
var nybHexString = “0123456789ABCDEF”;
return String(nybHexString.substr((n >> 4) & 0x0F,1)) + nybHexString.substr(n & 0x0F,1);
}
function setColourGradient() {
center = 128;
width = 127;
steps = 6;
frequency = 2*Math.PI/steps;
return makeColorGradient(frequency,frequency,frequency,0,2,4,center,width,50);
}
function initialiseSpawnInterval() {
if (gameMode === gameModes.PLAYING && spawnTimer>2) {
spawnTimer–;
spawnTimeChangeInterval-=50;
}
}
function setGameMode(mode) {
gameMode = mode;
timer=0;
}
function raiseSnow() {
if (gameMode === gameModes.PLAYING && snowHeight < canvas.height) {
snowHeight++;
}
}
function setHighScore() {
var currentHighScore = getHighScore();
if (currentHighScore !=-1 && score > currentHighScore) {
localStorage.setItem(“highScore”, score);
}
}
function getHighScore() {
if (!localStorage) return -1;
var highScore = localStorage.getItem(“highScore”);
return highScore || 0;
}
titleColours = setColourGradient();
initialiseSnow();
setInterval(draw, 30);
setInterval(initialiseSpawnInterval, spawnTimeChangeInterval);
setInterval(raiseSnow, 666);
**html代码**
<canvas id="canvas" width="450" height="540"></canvas>
### 演示流程
打包的文件就三个,一个css的代码,一个JS的代码,还有一个html的文件,打包好之后,点击html的文件就能直接运行了呢。
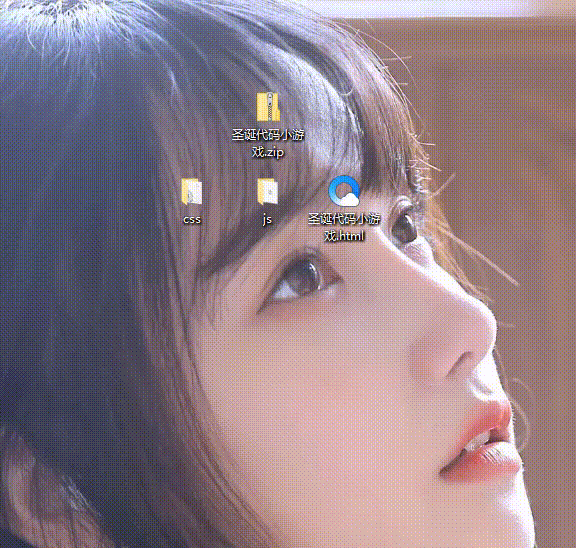
### 最后
不知道你们用的什么环境,我一般都是用的Python3.6环境和pycharm解释器,没有软件,或者没有资料,没人解答问题,都可以免费领取(包括今天的代码),过几天我还会做个视频教程出来,有需要也可以领取~
给大家准备的学习资料包括但不限于:
Python 环境、pycharm编辑器/永久激活/翻译插件
python 零基础视频教程
Python 界面开发实战教程
Python 爬虫实战教程
Python 数据分析实战教程
python 游戏开发实战教程
Python 电子书100本
Python 学习路线规划
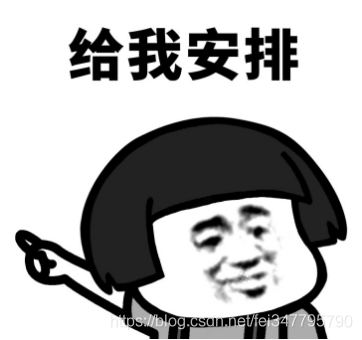
**网上学习资料一大堆,但如果学到的知识不成体系,遇到问题时只是浅尝辄止,不再深入研究,那么很难做到真正的技术提升。**
**[需要这份系统化学习资料的朋友,可以戳这里无偿获取](https://bbs.csdn.net/topics/618317507)**
**一个人可以走的很快,但一群人才能走的更远!不论你是正从事IT行业的老鸟或是对IT行业感兴趣的新人,都欢迎加入我们的的圈子(技术交流、学习资源、职场吐槽、大厂内推、面试辅导),让我们一起学习成长!**