类的适配器模式
- 源(Adapee)角色:现在需要适配的接口。
- 目标(Target)角色:这就是所期待得到的接口。注意:由于这里讨论的是类适配器模式,因此目标不可以是类。
- 适配器(Adaper)角色:适配器类是本模式的核心。适配器把源接口转换成目标接口。显然,这一角色不可以是接口,而必须是具体类。
源(Adapee)角色
二当家喜欢狗狗,所以养了一只狗狗,他有时候会发出叫声。
package com.secondgod.adapter;
/\*\*
\* 狗狗
\*
\* @author 二当家的白帽子 https://le-yi.blog.csdn.net/
\*/
public class Dog {
/\*\*
\* 发出声音
\*/
public void makeSound() {
System.out.println("狗狗:汪汪汪。。。。。。");
}
}
目标(Target)角色
我们会和朋友聊天说话。
package com.secondgod.adapter;
/\*\*
\* 朋友
\*
\* @author 二当家的白帽子 https://le-yi.blog.csdn.net/
\*/
public interface IFriend {
/\*\*
\* 说话
\*/
void speak();
}
适配器(Adaper)角色
过了一段时间,二当家把狗狗当成了朋友,觉得它不是在叫,而是在说话。
package com.secondgod.adapter;
/\*\*
\* 狗狗朋友
\*
\* @author 二当家的白帽子 https://le-yi.blog.csdn.net/
\*/
public class DogFriend extends Dog implements IFriend {
/\*\*
\* 说话了
\*/
@Override
public void speak() {
super.makeSound();
}
}
我们测试一下和狗狗朋友的说话。
package com.secondgod.adapter;
/\*\*
\* 人
\*
\* @author 二当家的白帽子 https://le-yi.blog.csdn.net/
\*/
public class Person {
/\*\*
\* 和朋友聊天
\*
\* @param friend
\*/
public void speakTo(IFriend friend) {
System.out.println("人:朋友,你干什么呢?");
friend.speak();
}
public static void main(String[] args) {
Person person = new Person();
IFriend friend = new DogFriend();
person.speakTo(friend);
}
}
二当家的说一句,狗狗叫一声,我们真的像是在聊天。
增加源(Adapee)角色的后果
有一天,二当家的又养了一只猫猫。
package com.secondgod.adapter;
/\*\*
\* 猫猫
\*
\* @author 二当家的白帽子 https://le-yi.blog.csdn.net/
\*/
public class Cat {
/\*\*
\* 发出声音
\*/
public void makeSound() {
System.out.println("猫猫:喵喵喵。。。。。。");
}
}
过了几天,二当家的和猫猫也成了朋友。这时候只好再多增加一个猫朋友类。
package com.secondgod.adapter;
/\*\*
\* 猫猫朋友
\*
\* @author 二当家的白帽子 https://le-yi.blog.csdn.net/
\*/
public class CatFriend extends Cat implements IFriend {
/\*\*
\* 说话了
\*/
@Override
public void speak() {
super.makeSound();
}
}
二当家的和狗朋友,猫朋友聊天。
package com.secondgod.adapter;
/\*\*
\* 人
\*
\* @author 二当家的白帽子 https://le-yi.blog.csdn.net/
\*/
public class Person {
/\*\*
\* 和朋友聊天
\*
\* @param friend
\*/
public void speakTo(IFriend friend) {
System.out.println("人:朋友,你干什么呢?");
friend.speak();
}
public static void main(String[] args) {
Person person = new Person();
IFriend dogFriend = new DogFriend();
IFriend catFriend = new CatFriend();
person.speakTo(dogFriend);
person.speakTo(catFriend);
}
}
以后要是二当家的再有其他动物朋友,就需要再去增加适配器类。有没有办法通用一点呢?
对象的适配器模式
二当家的希望可以有一个和各种动物做朋友的办法,而不是每次有了新的动物朋友都需要增加一个适配器。
增加一个动物接口
package com.secondgod.adapter;
/\*\*
\* 动物
\*
\* @author 二当家的白帽子 https://le-yi.blog.csdn.net/
\*/
public interface IAnimal {
/\*\*
\* 发出声音
\*/
void makeSound();
}
让源(Adapee)角色的猫猫和狗狗实现动物接口
package com.secondgod.adapter;
/\*\*
\* 狗狗
\*
\* @author 二当家的白帽子 https://le-yi.blog.csdn.net/
\*/
public class Dog implements IAnimal {
/\*\*
\* 发出声音
\*/
public void makeSound() {
System.out.println("狗狗:汪汪汪。。。。。。");
}
}
package com.secondgod.adapter;
/\*\*
\* 猫猫
\*
\* @author 二当家的白帽子 https://le-yi.blog.csdn.net/
\*/
public class Cat implements IAnimal {
/\*\*
\* 发出声音
\*/
public void makeSound() {
System.out.println("猫猫:喵喵喵。。。。。。");
}
}
万物拟人适配器(Adaper)角色
package com.secondgod.adapter;
/\*\*
\* 万物拟人适配器
\*
\* @author 二当家的白帽子 https://le-yi.blog.csdn.net/
\*/
public class AnimalFriendAdaper implements IFriend {
/\*\*
\* 被拟人化的动物朋友
\*/
private IAnimal animal;
public AnimalFriendAdaper(IAnimal animal) {
this.animal = animal;
}
@Override
public void speak() {
animal.makeSound();
}
}
测试我们的万物拟人适配器。
package com.secondgod.adapter;
/\*\*
\* 人
\*
\* @author 二当家的白帽子 https://le-yi.blog.csdn.net/
\*/
public class Person {
/\*\*
\* 和朋友聊天
\*
\* @param friend
\*/
public void speakTo(IFriend friend) {
System.out.println("人:朋友,你干什么呢?");
friend.speak();
}
public static void main(String[] args) {
// 一个人
Person person = new Person();
// 一只狗
IAnimal dog = new Dog();
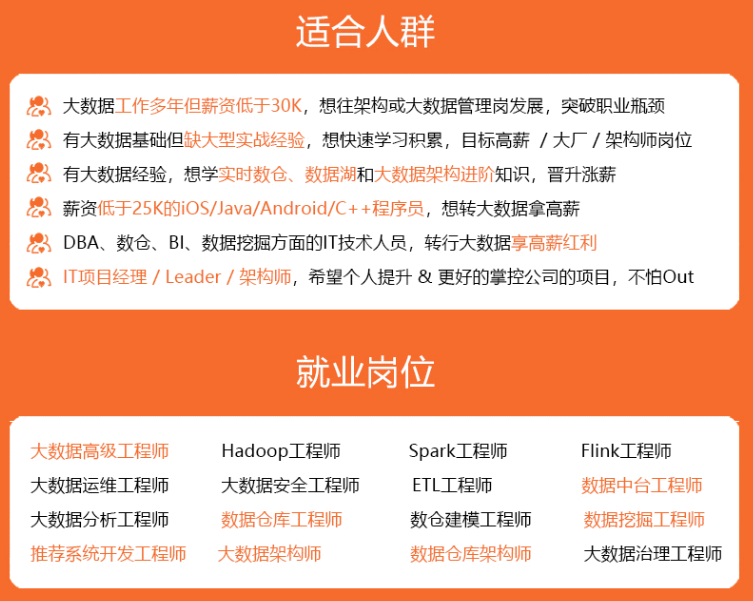
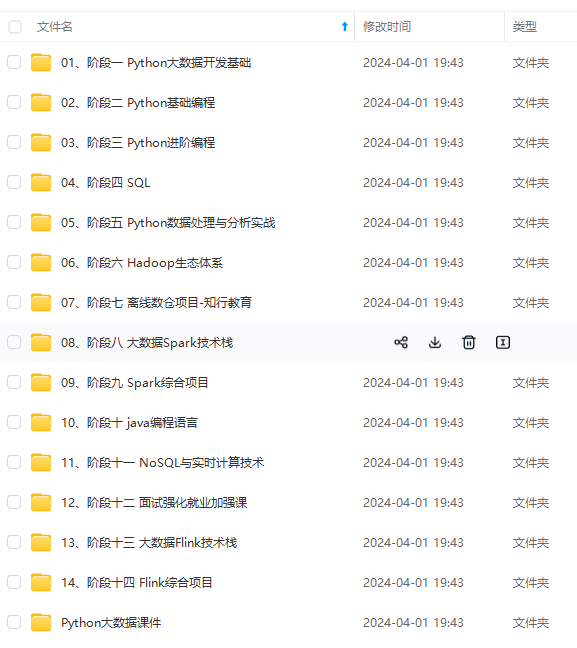
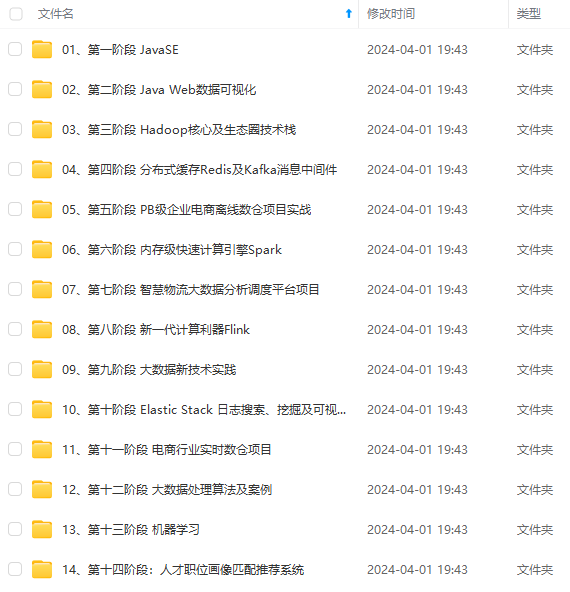
**既有适合小白学习的零基础资料,也有适合3年以上经验的小伙伴深入学习提升的进阶课程,涵盖了95%以上大数据知识点,真正体系化!**
**由于文件比较多,这里只是将部分目录截图出来,全套包含大厂面经、学习笔记、源码讲义、实战项目、大纲路线、讲解视频,并且后续会持续更新**
**[需要这份系统化资料的朋友,可以戳这里获取](https://bbs.csdn.net/topics/618545628)**
= new Person();
// 一只狗
IAnimal dog = new Dog();
[外链图片转存中...(img-2P1pNn8R-1714154440763)]
[外链图片转存中...(img-XE2EbdUD-1714154440763)]
[外链图片转存中...(img-vOrwa3pl-1714154440764)]
**既有适合小白学习的零基础资料,也有适合3年以上经验的小伙伴深入学习提升的进阶课程,涵盖了95%以上大数据知识点,真正体系化!**
**由于文件比较多,这里只是将部分目录截图出来,全套包含大厂面经、学习笔记、源码讲义、实战项目、大纲路线、讲解视频,并且后续会持续更新**
**[需要这份系统化资料的朋友,可以戳这里获取](https://bbs.csdn.net/topics/618545628)**