if ( !fileExists)
{//文件不存在,则上传
HDFSApi.copyFromLocalFile(conf, localFilePath, remoteFilePath);
System.out.println(localFilePath + " 已上传至 " + remoteFilePath);
}
else if (a2)
{//选择覆盖
HDFSApi.copyFromLocalFile(conf, localFilePath, remoteFilePath);
System.out.println(localFilePath + " 已覆盖 " + remoteFilePath);
}
else if(a1)
{//选择追加
HDFSApi.appendToFile(conf, localFilePath, remoteFilePath);
System.out.println(localFilePath + " 已追加至 " + remoteFilePath);
}
}
catch (Exception e)
{
e.printStackTrace();
}
}
}
---
### ⭐️HDFSApi2
**2)从 HDFS 中下载指定文件。如果本地文件与要下载的文件名称相同,则自动对下载的文件重命名;**
**Shell命令**
if $(./bin/hdfs dfs -test -e file:///usr/local/hadoop/text.txt);
then $(./bin/hdfs dfs -copyToLocal text.txt ./text2.txt);
else $(./bin/hdfs dfs -copyToLocal text.txt ./text.txt);
fi
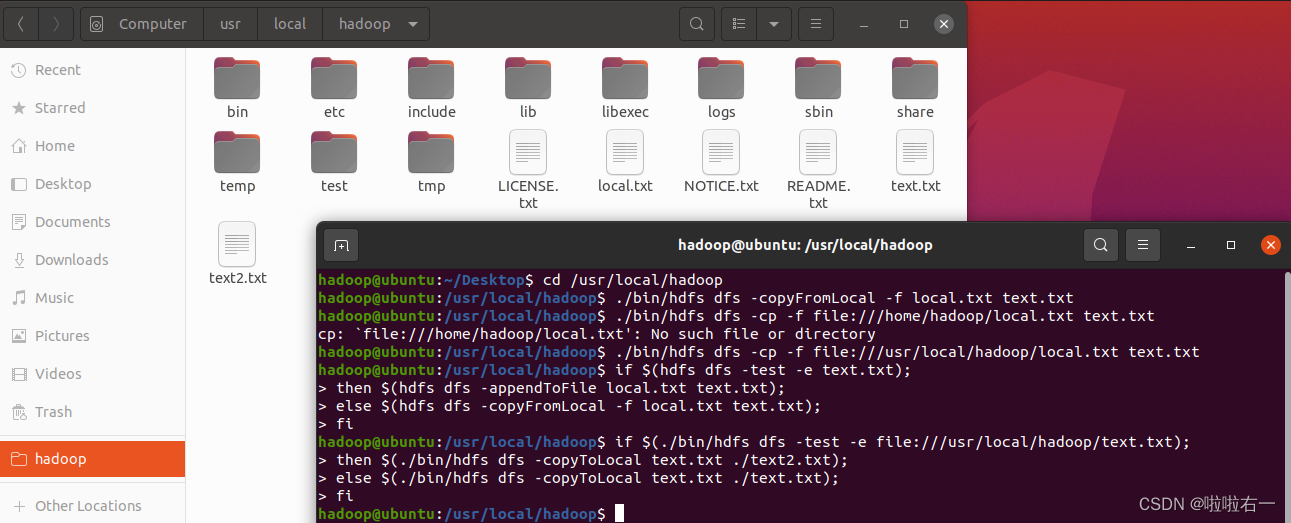
---
**编程实现**

package HDFSApi;
import org.apache.hadoop.conf.Configuration;
import org.apache.hadoop.fs.*;
import java.io.*;
public class HDFSApi2
{
/*
下载文件到本地
判断本地路径是否已存在,若已存在,则自动进行重命名
*/
public static void copyToLocal(Configuration conf, String remoteFilePath, String localFilePath) throws IOException
{
FileSystem fs = FileSystem.get(conf);
Path remotePath = new Path(remoteFilePath);
File f = new File(localFilePath);
if(f.exists())
{ //如果文件名存在,自动重命名(在文件名后面加上 _0, _1 …)
System.out.println(localFilePath + " 已存在.");
Integer i = 0;
while (true)
{
f = new File(localFilePath + “_” + i.toString());
if (!f.exists())
{
localFilePath = localFilePath + “_” + i.toString();
break;
}
}
System.out.println("将重新命名为: " + localFilePath);
}
// 下载文件到本地
Path localPath = new Path(localFilePath);
fs.copyToLocalFile(remotePath, localPath);
fs.close();
}
/*
主函数
*/
public static void main(String[] args)
{
Configuration conf = new Configuration();
conf.set(“fs.default.name”,“hdfs://localhost:9000”);
String localFilePath = “/usr/local/hadoop/local.txt”;
String remoteFilePath = “/usr/local/hadoop/text.txt”;
try
{
HDFSApi2.copyToLocal(conf, remoteFilePath, localFilePath);
System.out.println(“下载完成”);
}
catch (Exception e)
{
e.printStackTrace();
}
}
}
---
### ⭐️HDFSApi3
**3)将 HDFS 中指定文件的内容输出到终端中;**
**Shell命令**
hdfs dfs -cat text.txt

---
>
> 刚开始先跑shell,运行不报错,但无内容输出(但txt里是有内容的)。编程实现跑了一遍,再回去跑shell就有输出了(?
>
>
>
**编程实现**

package HDFSApi;
import org.apache.hadoop.conf.Configuration;
import org.apache.hadoop.fs.*;
import java.io.*;
public class HDFSApi3
{
public static void cat(Configuration conf, String remoteFilePath) throws IOException
{
/*
读取文件内容
*/
FileSystem fs = FileSystem.get(conf);
Path remotePath = new Path(remoteFilePath);
FSDataInputStream in = fs.open(remotePath);
BufferedReader d = new BufferedReader(new InputStreamReader(in));
String line = null;
while ( (line = d.readLine()) != null )
{
System.out.println(line);
}
d.close();
in.close();
fs.close();
}
/\*
主函数
*/
public static void main(String[] args)
{
Configuration conf = new Configuration();
conf.set(“fs.default.name”,“hdfs://localhost:9000”);
String remoteFilePath = “/usr/local/hadoop/text.txt”; // HDFS 路径
try
{
System.out.println(“读取文件: " + remoteFilePath);
HDFSApi3.cat(conf, remoteFilePath);
System.out.println(”\n 读取完成");
}
catch (Exception e)
{
e.printStackTrace();
}
}
}
### ⭐️HDFSApi4
**4)显示 HDFS 中指定的文件的读写权限、大小、创建时间、路径等信息;**
**Shell命令**
hdfs dfs -ls -h text.txt

---
**编程实现**
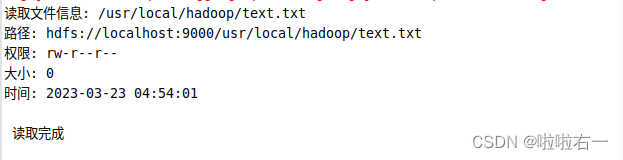
package HDFSApi;
import org.apache.hadoop.conf.Configuration;
import org.apache.hadoop.fs.*;
import java.io.*;
import java.text.SimpleDateFormat;
public class HDFSApi4
{
/*
显示指定文件的信息
*/
public static void ls(Configuration conf, String remoteFilePath) throws IOException
{
FileSystem fs = FileSystem.get(conf);
Path remotePath = new Path(remoteFilePath);
FileStatus[] fileStatuses = fs.listStatus(remotePath);
for (FileStatus s : fileStatuses)
{
System.out.println("路径: " + s.getPath().toString());
System.out.println("权限: " + s.getPermission().toString());
System.out.println("大小: " + s.getLen());
/* 返回的是时间戳,转化为时间日期格式 */
Long timeStamp = s.getModificationTime();
SimpleDateFormat format = new SimpleDateFormat(“yyyy-MM-dd HH:mm:ss”);
String date = format.format(timeStamp);
System.out.println("时间: " + date);
}
fs.close();
}
/\*\*
* 主函数
*/
public static void main(String[] args)
{
Configuration conf = new Configuration();
conf.set(“fs.default.name”,“hdfs://localhost:9000”);
String remoteFilePath = “/usr/local/hadoop/text.txt”; // HDFS 路径
try
{
System.out.println(“读取文件信息: " + remoteFilePath);
HDFSApi4.ls(conf, remoteFilePath);
System.out.println(”\n 读取完成");
}
catch (Exception e)
{
e.printStackTrace();
}
}
}
### ⭐️HDFSApi5
**5)给定 HDFS 中某一个目录,递归输出该目录下的所有文件的读写权限、大小、创建时间、路径等信息;**
**Shell命令**
cd /usr/local/hadoop
./bin/hdfs dfs -ls -R -h /usr/hadoop

---
**编程实现**
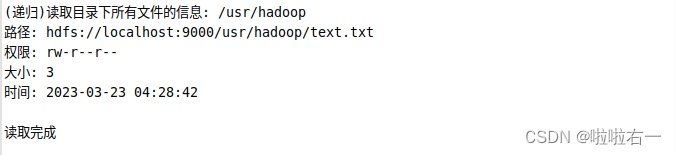
package HDFSApi;
import org.apache.hadoop.conf.Configuration;
import org.apache.hadoop.fs.*;
import java.io.*;
import java.text.SimpleDateFormat;
public class HDFSApi5
{
/*
显示指定文件夹下所有文件的信息(递归)
*/
public static void lsDir(Configuration conf, String remoteDir) throws IOException
{
FileSystem fs = FileSystem.get(conf);
Path dirPath = new Path(remoteDir);
/* 递归获取目录下的所有文件 */
RemoteIterator remoteIterator = fs.listFiles(dirPath, true);
/* 输出每个文件的信息 */
while (remoteIterator.hasNext())
{
FileStatus s = remoteIterator.next();
System.out.println("路径: " + s.getPath().toString());
System.out.println("权限: " + s.getPermission().toString());
System.out.println("大小: " + s.getLen());
/* 返回的是时间戳,转化为时间日期格式 */
Long timeStamp = s.getModificationTime();
SimpleDateFormat format = new SimpleDateFormat(“yyyy-MM-dd HH:mm:ss”);
String date = format.format(timeStamp);
System.out.println("时间: " + date);
System.out.println();
}
fs.close();
}
/\*
主函数
*/
public static void main(String[] args)
{
Configuration conf = new Configuration();
conf.set(“fs.default.name”,“hdfs://localhost:9000”);
String remoteDir = “/usr/hadoop”; // HDFS 路径
try
{
System.out.println("(递归)读取目录下所有文件的信息: " + remoteDir);
HDFSApi5.lsDir(conf, remoteDir);
System.out.println(“读取完成”);
}
catch (Exception e)
{
e.printStackTrace();
}
}
}
### ⭐️HDFSApi6
**6)提供一个 HDFS 内的文件的路径,对该文件进行创建和删除操作。如果文件所在目录不存在,则自动创建目录;**
**Shell命令**
if $(hdfs dfs -test -d dir1/dir2);
then $(hdfs dfs -touchz dir1/dir2/filename);
else $(hdfs dfs -mkdir -p dir1/dir2 && hdfs dfs -touchz dir1/dir2/filename);
fi
hdfs dfs -rm dir1/dir2/filename #删除文件

---
**编程实现**
路径存在的情况(以下代码是路径存在的情况)

路径不存在的情况

目录不存在的情况

package HDFSApi;
import org.apache.hadoop.conf.Configuration;
import org.apache.hadoop.fs.*;
import java.io.*;
public class HDFSApi6
{
/*
判断路径是否存在
*/
public static boolean test(Configuration conf, String path) throws IOException
{
FileSystem fs = FileSystem.get(conf);
return fs.exists(new Path(path));
}
/*
创建目录
*/
public static boolean mkdir(Configuration conf, String remoteDir) throws IOException
{
FileSystem fs = FileSystem.get(conf);
Path dirPath = new Path(remoteDir);
boolean result = fs.mkdirs(dirPath);
fs.close();
return result;
}
/*
创建文件
*/
public static void touchz(Configuration conf, String remoteFilePath) throws IOException
{
FileSystem fs = FileSystem.get(conf);
Path remotePath = new Path(remoteFilePath);
FSDataOutputStream outputStream = fs.create(remotePath);
outputStream.close();
fs.close();
}
/*
删除文件
*/
public static boolean rm(Configuration conf, String remoteFilePath) throws IOException
{
FileSystem fs = FileSystem.get(conf);
Path remotePath = new Path(remoteFilePath);
boolean result = fs.delete(remotePath, false);
fs.close();
return result;
}
/*
主函数
*/
public static void main(String[] args)
{
Configuration conf = new Configuration();
conf.set(“fs.default.name”,“hdfs://localhost:9000”);
String remoteFilePath = “/usr/local/hadoop/text.txt”; // HDFS 路径
String remoteDir = “/usr/hadoop/input”; // HDFS 路径对应的目录
try {
/* 判断路径是否存在,存在则删除,否则进行创建 */
if ( HDFSApi6.test(conf, remoteFilePath) )
{
HDFSApi6.rm(conf, remoteFilePath); // 删除
System.out.println("删除路径: " + remoteFilePath);
}
else
{
if ( !HDFSApi6.test(conf, remoteDir) )
{ // 若目录不存在,则进行创建
HDFSApi6.mkdir(conf, remoteDir);
System.out.println("创建文件夹: " + remoteDir);
}
HDFSApi6.touchz(conf, remoteFilePath);
System.out.println("创建路径: " + remoteFilePath);
}
}
catch (Exception e)
{
e.printStackTrace();
}
}
}
### ⭐️HDFSApi7
**7)提供一个 HDFS 的目录的路径,对该目录进行创建和删除操作。创建目录时,如果目录文件所在目录不存在,则自动创建相应目录;删除目录时,当该目录为空时删除,当该目录不为空时不删除该目录;**
**Shell命令**
cd /usr/local/hadoop
./bin/hdfs dfs -mkdir -p dir1/dir2
./bin/hdfs dfs -rmdir dir1/dir2
#若为非空目录,强制删除语句如下
./bin/hdfs dfs -rm -R dir1/dir2

---
**编程实现**
目录不存在于是创建

目录存在且为空,删除

package HDFSApi;
import org.apache.hadoop.conf.Configuration;
import org.apache.hadoop.fs.*;
import java.io.*;
public class HDFSApi7
{
/*
判断路径是否存在
*/
public static boolean test(Configuration conf, String path) throws IOException
{
//访问获取hdfs文件系统数据
FileSystem fs = FileSystem.get(conf);
return fs.exists(new Path(path));
}
/*
判断目录是否为空
true: 空,false: 非空
*/
public static boolean isDirEmpty(Configuration conf, String remoteDir) throws IOException
{
FileSystem fs = FileSystem.get(conf);
Path dirPath = new Path(remoteDir);//获取hdfs文件路径
RemoteIterator remoteIterator = fs.listFiles(dirPath, true);
//获取当前路径下的文件,得到file类型的数组
return !remoteIterator.hasNext();//hasNext表示文件有内容,用这个判断目录是否为空
}
/*
创建目录
*/
public static boolean mkdir(Configuration conf, String remoteDir) throws IOException
{
FileSystem fs = FileSystem.get(conf);
Path dirPath = new Path(remoteDir);
boolean result = fs.mkdirs(dirPath);//mkdirs,创建
fs.close();
return result;
}
/*
删除目录
*/
public static boolean rmDir(Configuration conf, String remoteDir) throws IOException
{
FileSystem fs = FileSystem.get(conf);//访问获取hdfs文件系统数据
Path dirPath = new Path(remoteDir);//获取hdfs文件路径
/* 第二个参数表示是否递归删除所有文件 */
boolean result = fs.delete(dirPath, true);//delete,删除
fs.close();
return result;
}
/*
主函数
*/
public static void main(String[] args)
{
Configuration conf = new Configuration();
conf.set(“fs.default.name”,“hdfs://localhost:9000”);
String remoteDir = “/usr/hadoop/test/dir”;
Boolean forceDelete = false;
try
{
/* 判断目录是否存在,不存在则创建,存在则删除 */
if ( !HDFSApi7.test(conf, remoteDir) )
{
HDFSApi7.mkdir(conf, remoteDir); //创建
System.out.println("创建目录: " + remoteDir);
}
else
{
if ( HDFSApi7.isDirEmpty(conf, remoteDir) || forceDelete )
{ // 目录为空或强制删除
HDFSApi7.rmDir(conf, remoteDir);
System.out.println("删除目录: " + remoteDir);
}
else
{ // 目录不为空
System.out.println("目录不为空,不删除: " + remoteDir);
}
}
}
catch (Exception e)
{
e.printStackTrace();
}
}
}
### ⭐️HDFSApi8
**8)向 HDFS 中指定的文件追加内容,由用户指定内容追加到原有文件的开头或结尾;**
**Shell命令**
#追加到原文件末尾
cd /usr/local/hadoop
hdfs dfs -appendToFile local.txt text.txt
#追加到原文件开头
hdfs dfs -get text.txt
cat text.txt >> local.txt
hdfs dfs -copyFromLocal -f local.txt text.txt
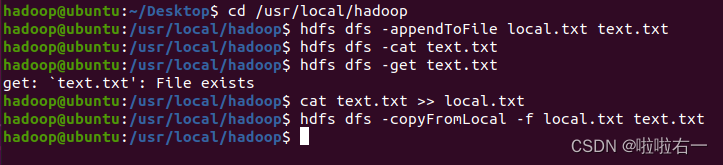
---
**编程实现**


package HDFSApi;
import org.apache.hadoop.conf.Configuration;
import org.apache.hadoop.fs.*;
import java.io.*;
import java.util.Scanner;
public class HDFSApi8
{
/*
判断路径是否存在
*/
public static boolean test(Configuration conf, String path) throws IOException
{
FileSystem fs = FileSystem.get(conf);
return fs.exists(new Path(path));
}
/*
追加文本内容
*/
public static void appendContentToFile(Configuration conf, String content, String remoteFilePath) throws IOException
{
FileSystem fs = FileSystem.get(conf);//获取数据
Path remotePath = new Path(remoteFilePath);//获取路径
/* 创建一个文件输出流,输出的内容将追加到文件末尾 */
FSDataOutputStream out = fs.append(remotePath);//追加文件内容
out.write(content.getBytes());//把字符串转化为字节数组
out.close();
fs.close();
}
/*
追加文件内容
*/
public static void appendToFile(Configuration conf, String localFilePath, String remoteFilePath) throws IOException
{
FileSystem fs = FileSystem.get(conf);//获取数据
Path remotePath = new Path(remoteFilePath);//获取路径
/* 创建一个文件读入流 */
FileInputStream in = new FileInputStream(localFilePath);
/* 创建一个文件输出流,输出的内容将追加到文件末尾 */
FSDataOutputStream out = fs.append(remotePath);
/* 读写文件内容 */
byte[] data = new byte[1024];
int read = -1;
if(in!=null)
{
while ( (read = in.read(data)) > 0 )
{
out.write(data, 0, read);
}
}
out.close();
in.close();
fs.close();
}
/*
移动文件到本地
移动后,删除源文件
*/
public static void moveToLocalFile(Configuration conf, String remoteFilePath, String localFilePath) throws IOException
{
FileSystem fs = FileSystem.get(conf);//获取数据
Path remotePath = new Path(remoteFilePath);//获取路径
自我介绍一下,小编13年上海交大毕业,曾经在小公司待过,也去过华为、OPPO等大厂,18年进入阿里一直到现在。
深知大多数大数据工程师,想要提升技能,往往是自己摸索成长或者是报班学习,但对于培训机构动则几千的学费,着实压力不小。自己不成体系的自学效果低效又漫长,而且极易碰到天花板技术停滞不前!
因此收集整理了一份《2024年大数据全套学习资料》,初衷也很简单,就是希望能够帮助到想自学提升又不知道该从何学起的朋友。
既有适合小白学习的零基础资料,也有适合3年以上经验的小伙伴深入学习提升的进阶课程,基本涵盖了95%以上大数据开发知识点,真正体系化!
由于文件比较大,这里只是将部分目录大纲截图出来,每个节点里面都包含大厂面经、学习笔记、源码讲义、实战项目、讲解视频,并且后续会持续更新
如果你觉得这些内容对你有帮助,可以添加VX:vip204888 (备注大数据获取)
一个人可以走的很快,但一群人才能走的更远。不论你是正从事IT行业的老鸟或是对IT行业感兴趣的新人,都欢迎扫码加入我们的的圈子(技术交流、学习资源、职场吐槽、大厂内推、面试辅导),让我们一起学习成长!
3年上海交大毕业,曾经在小公司待过,也去过华为、OPPO等大厂,18年进入阿里一直到现在。**
深知大多数大数据工程师,想要提升技能,往往是自己摸索成长或者是报班学习,但对于培训机构动则几千的学费,着实压力不小。自己不成体系的自学效果低效又漫长,而且极易碰到天花板技术停滞不前!
因此收集整理了一份《2024年大数据全套学习资料》,初衷也很简单,就是希望能够帮助到想自学提升又不知道该从何学起的朋友。
[外链图片转存中…(img-heB1hfQh-1713019498543)]
[外链图片转存中…(img-i4fZfwpL-1713019498544)]
[外链图片转存中…(img-1hPKPYDd-1713019498545)]
[外链图片转存中…(img-uwqHt2Kk-1713019498545)]
[外链图片转存中…(img-leoVXWJN-1713019498545)]
既有适合小白学习的零基础资料,也有适合3年以上经验的小伙伴深入学习提升的进阶课程,基本涵盖了95%以上大数据开发知识点,真正体系化!
由于文件比较大,这里只是将部分目录大纲截图出来,每个节点里面都包含大厂面经、学习笔记、源码讲义、实战项目、讲解视频,并且后续会持续更新
如果你觉得这些内容对你有帮助,可以添加VX:vip204888 (备注大数据获取)
[外链图片转存中…(img-Hl7mJxo7-1713019498546)]
一个人可以走的很快,但一群人才能走的更远。不论你是正从事IT行业的老鸟或是对IT行业感兴趣的新人,都欢迎扫码加入我们的的圈子(技术交流、学习资源、职场吐槽、大厂内推、面试辅导),让我们一起学习成长!