* 退出
*/
@GetMapping(value = "logout")
public R logout(HttpServletRequest request) {
request.getSession().invalidate();
return R.ok("退出成功");
}
/**
* 密码重置
*/
@IgnoreAuth
@RequestMapping(value = "/resetPass")
public R resetPass(String username, HttpServletRequest request){
UserEntity user = userService.selectOne(new EntityWrapper<UserEntity>().eq("username", username));
if(user==null) {
return R.error("账号不存在");
}
user.setPassword("123456");
userService.update(user,null);
return R.ok("密码已重置为:123456");
}
/**
* 列表
*/
@RequestMapping("/page")
public R page(@RequestParam Map<String, Object> params,UserEntity user){
EntityWrapper<UserEntity> ew = new EntityWrapper<UserEntity>();
PageUtils page = userService.queryPage(params, MPUtil.sort(MPUtil.between(MPUtil.allLike(ew, user), params), params));
return R.ok().put("data", page);
}
/**
* 列表
*/
@RequestMapping("/list")
public R list( UserEntity user){
EntityWrapper<UserEntity> ew = new EntityWrapper<UserEntity>();
ew.allEq(MPUtil.allEQMapPre( user, "user"));
return R.ok().put("data", userService.selectListView(ew));
}
/**
* 信息
*/
@RequestMapping("/info/{id}")
public R info(@PathVariable("id") String id){
UserEntity user = userService.selectById(id);
return R.ok().put("data", user);
}
/**
* 获取用户的session用户信息
*/
@RequestMapping("/session")
public R getCurrUser(HttpServletRequest request){
Long id = (Long)request.getSession().getAttribute("userId");
UserEntity user = userService.selectById(id);
return R.ok().put("data", user);
}
/**
* 保存
*/
@PostMapping("/save")
public R save(@RequestBody UserEntity user){
// ValidatorUtils.validateEntity(user);
if(userService.selectOne(new EntityWrapper().eq(“username”, user.getUsername())) !=null) {
return R.error(“用户已存在”);
}
userService.insert(user);
return R.ok();
}
/**
* 修改
*/
@RequestMapping("/update")
public R update(@RequestBody UserEntity user){
// ValidatorUtils.validateEntity(user);
userService.updateById(user);//全部更新
return R.ok();
}
/**
* 删除
*/
@RequestMapping("/delete")
public R delete(@RequestBody Long[] ids){
userService.deleteBatchIds(Arrays.asList(ids));
return R.ok();
}
}
/**
-
上传文件映射表
*/
@RestController
@RequestMapping(“file”)
@SuppressWarnings({“unchecked”,“rawtypes”})
public class FileController{
@Autowired
private ConfigService configService;
@Async
@RequestMapping(“/upload”)
public R upload(@RequestParam(“file”) MultipartFile file,String type) throws Exception {
if (file.isEmpty()) {
throw new EIException(“上传文件不能为空”);
}
String fileExt = file.getOriginalFilename().substring(file.getOriginalFilename().lastIndexOf(“.”)+1);
File upload = new File(“D:/work/”);
if(!upload.exists()) {
upload.mkdirs();
}
String fileName = new Date().getTime()+“.”+fileExt;
File dest = new File(upload+“/”+fileName);file.transferTo(dest); if(StringUtils.isNotBlank(type) && type.equals("1")) { ConfigEntity configEntity = configService.selectOne(new EntityWrapper<ConfigEntity>().eq("name", "faceFile")); if(configEntity==null) { configEntity = new ConfigEntity(); configEntity.setName("faceFile"); configEntity.setValue(fileName); } else { configEntity.setValue(fileName); } configService.insertOrUpdate(configEntity); } return R.ok().put("file", fileName);
}
/**
- 下载文件
*/
@IgnoreAuth
@RequestMapping(“/download”)
public ResponseEntity<byte[]> download(@RequestParam String fileName) {
try {
File path = new File(ResourceUtils.getURL(“classpath:static”).getPath());
if(!path.exists()) {
path = new File(“”);
}
File upload = new File(path.getAbsolutePath(),“/upload/”);
if(!upload.exists()) {
upload.mkdirs();
}
File file = new File(upload.getAbsolutePath()+“/”+fileName);
if(file.exists()){
/if(!fileService.canRead(file, SessionManager.getSessionUser())){
getResponse().sendError(403);
}/
HttpHeaders headers = new HttpHeaders();
headers.setContentType(MediaType.APPLICATION_OCTET_STREAM);
headers.setContentDispositionFormData(“attachment”, fileName);
return new ResponseEntity<byte[]>(FileUtils.readFileToByteArray(file),headers, HttpStatus.CREATED);
}
} catch (IOException e) {
e.printStackTrace();
}
return new ResponseEntity<byte[]>(HttpStatus.INTERNAL_SERVER_ERROR);
}
- 下载文件
}
>
> ### 数据库设计:
>
>
>
checixinxi表:
| | | | | | | |
| --- | --- | --- | --- | --- | --- | --- |
| 序号 | 字段名称 | 字段类型 | 大小 | 允许为空 | 最大长度 | 备注 |
| 1 | id | Int | 4 | | 10 | |
| 2 | addtime | | 150 | | 255 | |
| 3 | checimingcheng | | 150 | | 255 | |
| 4 | huochemingcheng | DateTime | 8 | | 255 | |
| 5 | chepai | | 150 | | 255 | |
| 6 | tupian | DateTime | 8 | | 255 | |
| 7 | qidianzhan | | 150 | | 255 | |
| 8 | zhongdianzhan | DateTime | 8 | | 255 | |
| 9 | tujing | | 150 | | 255 | |
| 10 | riqi | DateTime | 8 | | 255 | |
| 11 | chufashijian | | 150 | | 255 | |
| 12 | shizhang | DateTime | 8 | | 255 | |
| 13 | zuoweileixing | | 150 | | 255 | |
| 14 | jiage | DateTime | 8 | | 255 | |
| 15 | piaoshu | | 150 | | 255 | |
chexingxinxi表:
| | | | | | | |
| --- | --- | --- | --- | --- | --- | --- |
| 序号 | 字段名称 | 字段类型 | 大小 | 允许为空 | 最大长度 | 备注 |
| 1 | id | Int | 4 | | 10 | |
| 2 | addtime | | 150 | | 255 | |
| 3 | huochebianhao | | 150 | | 255 | |
| 4 | huochemingcheng | DateTime | 8 | | 255 | |
| 5 | shisu | | 150 | | 255 | |
| 6 | zuoweishu | DateTime | 8 | | 255 | |
| 7 | chepai | | 150 | | 255 | |
gaiqiandingdan表:
| | | | | | | |
| --- | --- | --- | --- | --- | --- | --- |
| 序号 | 字段名称 | 字段类型 | 大小 | 允许为空 | 最大长度 | 备注 |
| 1 | id | Int | 4 | | 10 | |
| 2 | addtime | | 150 | | 255 | |
| 3 | dingdanbianhao | | 150 | | 255 | |
| 4 | checimingcheng | | 150 | | 255 | |
| 5 | chepai | DateTime | 8 | | 255 | |
| 6 | qidianzhan | shangpinleixing | DateTime | 8 | 255 | |
| 7 | zhongdianzhan | | | | 255 | |
| 8 | zongjiage | DateTime | | | 255 | |
| 9 | gaiqianriqi | DateTime | | | 255 | |
| 10 | yonghuming | DateTime | | | 255 | |
| 11 | xingming | DateTime | | | 255 | |
| 12 | shouji | DateTime | | | 255 | |
goupiaodingdan表:
| | | | | | | |
| --- | --- | --- | --- | --- | --- | --- |
| 序号 | 字段名称 | 字段类型 | 大小 | 允许为空 | 最大长度 | 备注 |
| 1 | id | Int | 4 | | 10 | |
| 2 | addtime | | 150 | | 255 | |
| 3 | dingdanbianhao | | 150 | | 255 | |
| 4 | checimingcheng | | 150 | | 255 | |
| 5 | chepai | DateTime | 8 | | 255 | |
| 6 | qidianzhan | DateTime | | | 255 | |
| 7 | zhongdianzhan | | | | 255 | |
| 8 | chufashijian | shangpinleixing | DateTime | 8 | 255 | |
| 9 | zuoweileixing | shangpinleixing | DateTime | 8 | 255 | |
| 10 | jiage | shangpinleixing | DateTime | 8 | 255 | |
| 11 | piaoshu | shangpinleixing | DateTime | 8 | 255 | |
| 12 | zongjiage | shangpinleixing | DateTime | 8 | 255 | |
| 13 | zongjiage | shangpinleixing | DateTime | 8 | 255 | |
| 14 | goumairiqi | shangpinleixing | DateTime | 8 | 255 | |
| 15 | yonghuming | shangpinleixing | DateTime | 8 | 255 | |
| 16 | xingming | shangpinleixing | DateTime | 8 | 255 | |
| 17 | shouji | shangpinleixing | DateTime | 8 | 255 | |
| 18 | shenfenzheng | shangpinleixing | DateTime | 8 | 255 | |
>
> ### 论文报告:
>
>
>
[摘 要](#_Toc56689507)
[1 绪论](#_Toc56689508)
[1.1研究背景](#_Toc56689509)
[1.2研究现状](#_Toc56689510)
[1.3研究内容](#_Toc56689511)
[2 系统关键技术](#_Toc56689512)
[2.1 Spring Boot框架](#_Toc56689513)
[2.2 JAVA技术](#_Toc56689514)
[2.3 MYSQL数据库](#_Toc56689515)
[2.4 B/S结构](#_Toc56689516)
[3 系统分析](#_Toc56689517)
[3.1 可行性分析](#_Toc56689518)
[3.1.1 技术可行性](#_Toc56689519)
[3.1.2经济可行性](#_Toc56689520)
[3.1.3操作可行性](#_Toc56689521)
[3.2 系统性能分析](#_Toc56689522)
[3.3 系统功能分析](#_Toc56689523)
[3.4系统流程分析](#_Toc56689524)
[3.4.1登录流程](#_Toc56689525)
[3.4.2注册流程](#_Toc56689526)
[3.4.3添加信息流程](#_Toc56689527)
[3.4.4删除信息流程](#_Toc56689528)
[4 系统设计](#_Toc56689529)
[4.1系统概要设计](#_Toc56689530)
[4.2系统结构设计](#_Toc56689531)
[4.3系统顺序图设计](#_Toc56689532)
[4.3.1登录模块顺序图](#_Toc56689533)
[4.3.2添加信息模块顺序图](#_Toc56689534)
[4.4数据库设计](#_Toc56689535)
[4.4.1数据库E-R图设计](#_Toc56689536)
[4.4.2数据库表设计](#_Toc56689537)
[第5章 系统详细设计](#_Toc56689538)
[5.1前台首页功能模块](#_Toc56689539)
[5.2管理员功能模块](#_Toc56689540)
**自我介绍一下,小编13年上海交大毕业,曾经在小公司待过,也去过华为、OPPO等大厂,18年进入阿里一直到现在。**
**深知大多数同学面临毕业设计项目选题时,很多人都会感到无从下手,尤其是对于计算机专业的学生来说,选择一个合适的题目尤为重要。因为毕业设计不仅是我们在大学四年学习的一个总结,更是展示自己能力的重要机会。**
**因此收集整理了一份《2024年计算机毕业设计项目大全》,初衷也很简单,就是希望能够帮助提高效率,同时减轻大家的负担。**
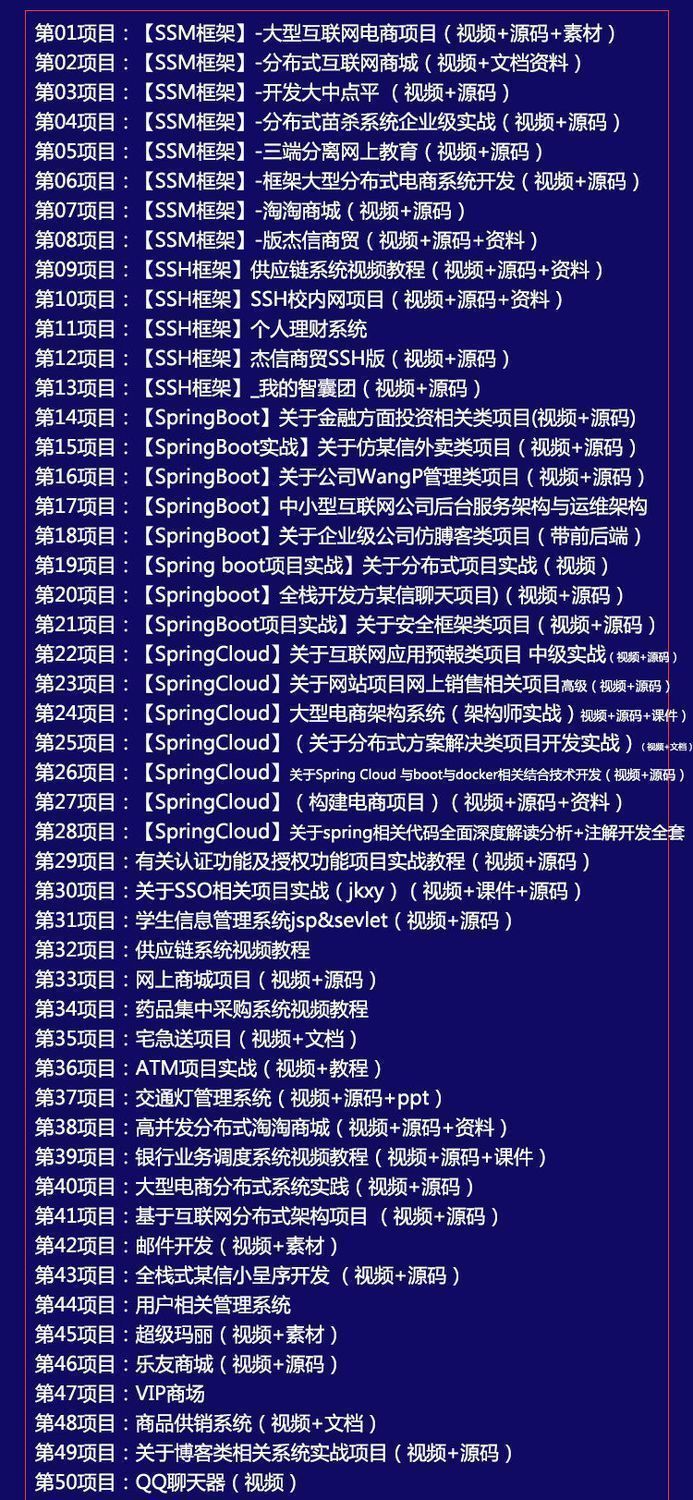
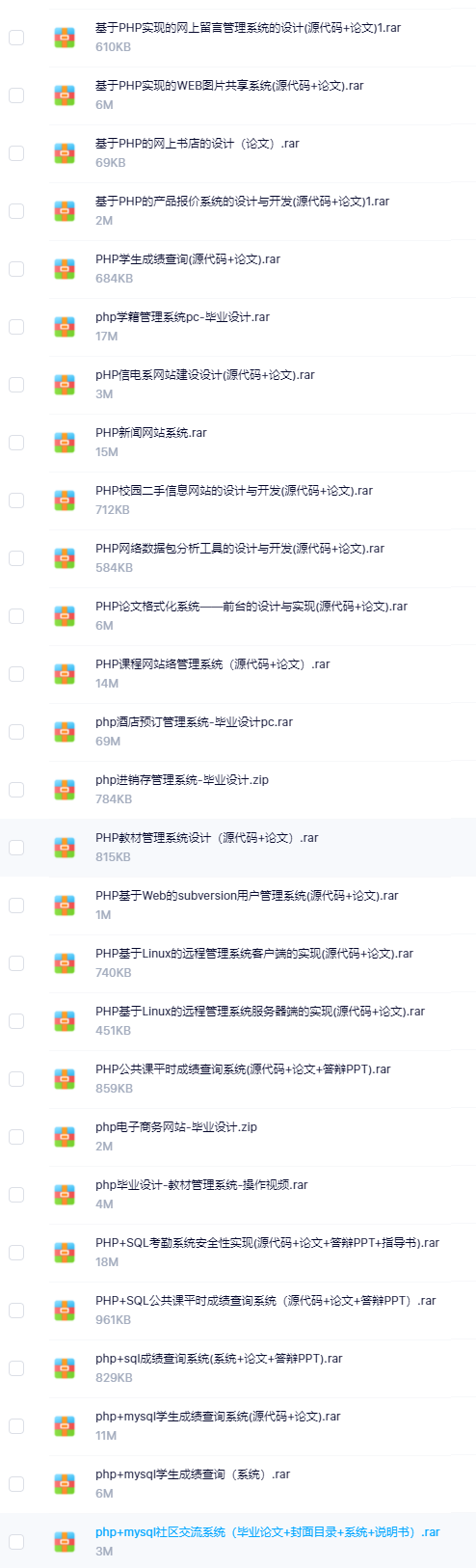
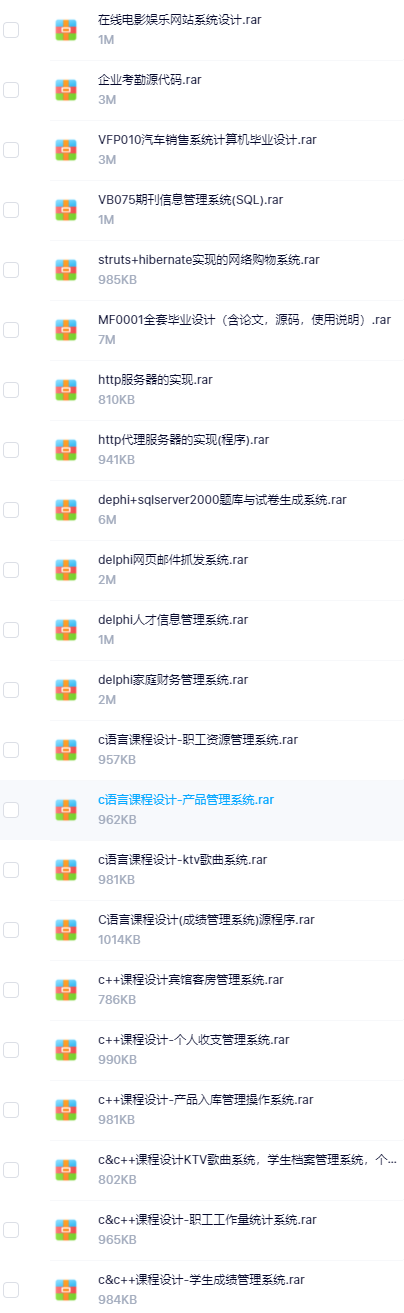
**既有Java、Web、PHP、也有C、小程序、Python等项目供你选择,真正体系化!**
**由于项目比较多,这里只是将部分目录截图出来,每个节点里面都包含素材文档、项目源码、讲解视频**
**如果你觉得这些内容对你有帮助,可以添加VX:vip1024c (备注项目大全获取)**
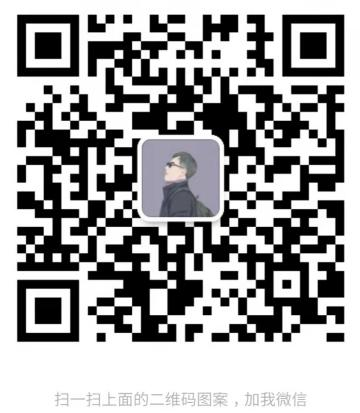
能力的重要机会。**
**因此收集整理了一份《2024年计算机毕业设计项目大全》,初衷也很简单,就是希望能够帮助提高效率,同时减轻大家的负担。**
[外链图片转存中...(img-yIeYCD0d-1712560672395)]
[外链图片转存中...(img-gh2b6HVY-1712560672395)]
[外链图片转存中...(img-Nhx3d956-1712560672395)]
**既有Java、Web、PHP、也有C、小程序、Python等项目供你选择,真正体系化!**
**由于项目比较多,这里只是将部分目录截图出来,每个节点里面都包含素材文档、项目源码、讲解视频**
**如果你觉得这些内容对你有帮助,可以添加VX:vip1024c (备注项目大全获取)**
[外链图片转存中...(img-KwjecE4L-1712560672396)]