# 1. 得到幻灯片对象
prs = Presentation(“demo.pptx”)
# 2. 获取prs对象中的每一页PPT
for slide in prs.slides:
# 3. 遍历获取每页PPT中的内容
for shape in slide.shapes:
# 4. 打印具体的Shape
print(shape)
# 5. 我是一条华丽的分割线,划分不同的slide
print(“——————————————————————————”)
**输出结果:**
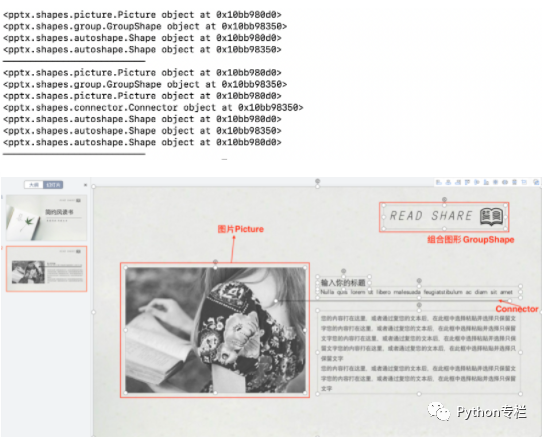
如果想获取每个Shape里面的文字也可以:
>
> shape.has\_text\_frame ----->是否有文字 shape.text\_frame ----->获取文字框
>
>
>
from pptx import Presentation
from pptx.util import Inches
# 1. 得到幻灯片对象
prs = Presentation(“demo.pptx”)
# 2. 获取prs对象中的每一页PPT
for slide in prs.slides:
# 3. 遍历获取每页PPT中的内容
for shape in slide.shapes:
# 4. 判断是否存在文本
if shape.has_text_frame:
# 5. 如果存在则获取内容
text_frame = shape.text_frame
print(text_frame.text)
# 6. 我是一条华丽的分割线,划分不同的slide
print(“——————————————————————————”)
输出的结果(对比前面的幻灯片):
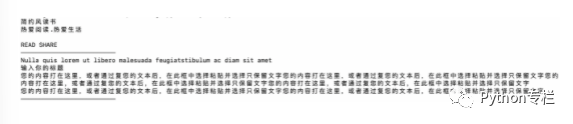
**从shape中找paragraph**
from pptx import Presentation
from pptx.util import Inches
# 1. 得到幻灯片对象
prs = Presentation(“demo.pptx”)
# 2. 获取prs对象中的每一页PPT
for slide in prs.slides:
# 3. 遍历获取每页PPT中的内容
for shape in slide.shapes:
# 4. 判断是否存在文本
if shape.has_text_frame:
# 5. 获取shape中的文本
text_frame = shape.text_frame
# 6. 获取text_frame中的段落内容
for paragraph in text_frame.paragraphs:
# 7. 打印段落内容
print(paragraph.text)
print(‘----------paragraph------------’)
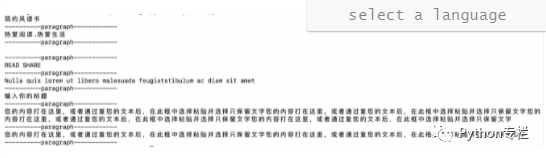
### **综合应用**
编写一个Python程序,要求
(1)打开demo.pptx
(2)按照paragraph分段,转换成为word文档
(3)保存为demo.docx
from pptx import Presentation
from docx import Document
doc = Document()
prs = Presentation(“婚礼策划师的最爱.pptx”)
ls = []
for slide in prs.slides:
for shape in slide.shapes:
if shape.has_text_frame:
text_frame = shape.text_frame
for paragraph in text_frame.paragraphs:
if paragraph.text != ‘’:
doc.add_paragraph(paragraph.text)
doc.save(“demo.docx”)
### **向PPT写入内容**
首先了解模板和占位符
Slides\_layouts:版式,一个幻灯片母版由多个版式组成,索引从0开始。
Placeholder:占位符:存在PPT母版里面的幻灯片的某一部件:Placeholder
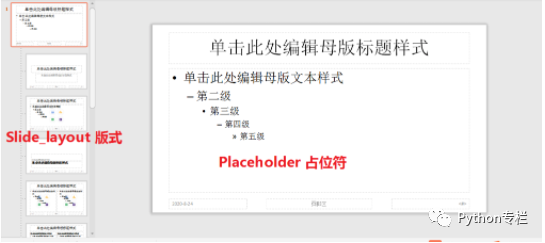
在创建一页ppt时,需要指定对应的布局,在该模块中, 内置了以下9种布局
1. Title
2. Title and Content
3. Section Header
4. Two Content
5. Comparison
6. Title Only
7. Blank
8. Content with Caption
9. Picture with Caption
通过数字下标0到9来访问,指定布局添加一页ppt的用法如下:
### **创建placeholders**
from pptx import Presentation
prs = Presentation() #初始化一个空pptx文档
slide = prs.slides.add_slide(prs.slide_layouts[0]) # 用第一个母版生成一页ppt
for shape in slide.placeholders: # 获取这一页所有的占位符
phf = shape.placeholder_format
print(f’{phf.idx}–{shape.name}–{phf.type}‘) # id号–占位符形状名称-占位符的类型
shape.text = f’{phf.idx}–{shape.name}–{phf.type}’
prs.save(“new_template.pptx”)
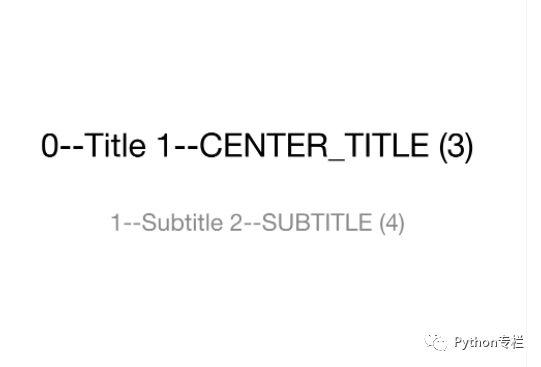
也可以一次添加多个
from pptx import Presentation
prs = Presentation() #初始化一个空pptx文档
i = 0
while i <= 10:
slide = prs.slides.add_slide(prs.slide_layouts[i])
i = i+1
for shape in slide.placeholders: # 获取这一页所有的占位符
phf = shape.placeholder_format
print(f’{phf.idx}–{shape.name}–{phf.type}‘) # id号–占位符形状名称-占位符的类型
shape.text = f’{phf.idx}–{shape.name}–{phf.type}’
prs.save(“new_template.pptx”)
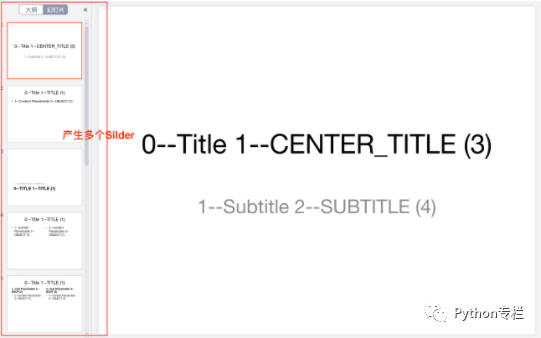
**向占位符中添加内容**
主要使用:
>
> ###### shape.text = 字符串
>
>
> ###### prs.save(文件路径)
>
>
>
from pptx import Presentation
prs = Presentation()
# 用第一个母版生成一页ppt
slide = prs.slides.add_slide(prs.slide_layouts[1])
# 获取当前页的标题
title_shape = slide.shapes.title
# 向标题中添加文本
title_shape.text = ‘宋宋的Python专栏’
# 获取副标题
subtitle = slide.shapes.placeholders[1]
# 副标题中添加文本
subtitle.text = ‘宋宋是一个爱美的大女生’
# 副标题中添加新段落
new_paragraph1 = subtitle.text_frame.add_paragraph()
# 向段落中添加文本
new_paragraph1.text = ‘我是一个专注学习技术的大女生’
new_paragraph1.level = 1
new_paragraph2 = subtitle.text_frame.add_paragraph()
new_paragraph2.text = ‘我是一个认真写文章的大女生哈哈哈’
new_paragraph2.level = 2
# 保存内容
prs.save(“写入内容1.pptx”)
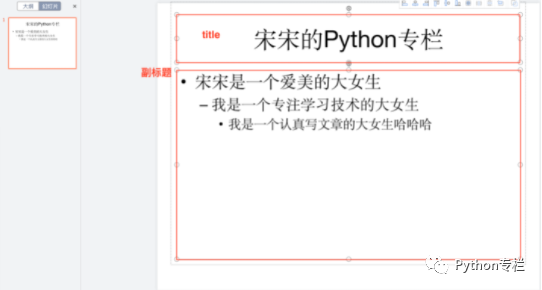
空白的PPT模板中添加文本框和图形
from pptx import Presentation
from pptx.util import Cm,Pt,Inches
from pptx.enum.shapes import MSO_SHAPE
prs = Presentation(‘写入内容1.pptx’)
slide = prs.slides.add_slide(prs.slide_layouts[6]) # 6的layout中是一个空白的Slider,里面没有占位符
# 单独向里面添加文本框和图片
left = top = width = height =Cm(3) # # left,top为相对位置,width,height为文本框大小。满足条件顺序是左>上>右>下
text_box = slide.shapes.add_textbox(left,top,width,height)
tf = text_box.text_frame
网上学习资料一大堆,但如果学到的知识不成体系,遇到问题时只是浅尝辄止,不再深入研究,那么很难做到真正的技术提升。
一个人可以走的很快,但一群人才能走的更远!不论你是正从事IT行业的老鸟或是对IT行业感兴趣的新人,都欢迎加入我们的的圈子(技术交流、学习资源、职场吐槽、大厂内推、面试辅导),让我们一起学习成长!