#### 2.2.2 合并两个排序的链表
/\*\*
*
* @param ListNode
* 链表1
* @param ListNode
* 链表2
* @return ListNode
* 合并后的链表
*/
private static ListNode Merge(ListNode list1,ListNode list2) {
// 其中之一为空或均为空
if(list1 == null || list2 == null){
return list1 == null?(list2 == null?list1:list2):list1;
}else{
ListNode headNode = null;
if(list1.val < list2.val){
headNode = list1;
headNode.next = Merge(list1.next, list2);
}else{
headNode = list2;
headNode.next = Merge(list1, list2.next);
}
return headNode;
}
}
#### 2.2.3 求两个链表的第一个公共节点问题
private static ListNode FindFirstCommonNode2(ListNode pHead1, ListNode pHead2) {
// 第一步首先是特殊输入测试,即为空或长度为0的情况,切记!
if(pHead1 == null || pHead2 == null){
return null;
}
int len1 = getLength(pHead1);
int len2 = getLength(pHead2);
int difLen = 0;
// pHeadTmp1指向较长链表
ListNode pHeadLong = pHead1;
// pHeadTmp2指向较短链表
ListNode pHeadShort = pHead2;
if(len1 > len2){
difLen = len1 - len2;
}else{
pHeadLong = pHead2;
pHeadShort = pHead1;
difLen = len2 - len1;
}
// 1.先让长的链表走difLen步
for(int i = 0; i < difLen; i++){
pHeadLong = pHeadLong.next;
}
// 2.对齐后,两个链表同时走
while(pHeadLong != null && pHeadShort != null && pHeadLong != pHeadShort){
pHeadLong = pHeadLong.next;
pHeadShort = pHeadShort.next;
}
return pHeadLong;
}
private static int getLength(ListNode pHead){
int len = 0;
while(pHead != null){
len++;
pHead = pHead.next;
}
return len;
}
### 三、多线程
#### 3.1 [线程与进程的区别](https://bbs.csdn.net/topics/618166371)
#### 3.2 实现生产者、消费者
package cn.edu.ujn.producer_customer;
// 公共资源类
class PublicResource {
private static final int MAX\_RESOURCE\_NUMBER = 10;
private int number = 0;
/\*\*
* 增加公共资源
*/
public synchronized void increace() {
while (number > MAX_RESOURCE_NUMBER) {
try {
wait();
} catch (InterruptedException e) {
e.printStackTrace();
}
}
// 添加资源
number++;
System.out.println(number);
// 进行通知
notify();
}
/\*\*
* 减少公共资源
*/
public synchronized void decreace() {
while (number == 0) {
try {
wait();
} catch (InterruptedException e) {
e.printStackTrace();
}
}
number–;
System.out.println(number);
notify();
}
}
// 生产者线程,负责生产公共资源
class ProducerThread implements Runnable {
private PublicResource resource;
public ProducerThread(PublicResource resource) {
this.resource = resource;
}
@Override
public void run() {
for (int i = 0; i < 10; i++) {
try {
Thread.sleep((long) (Math.random() \* 1000));
} catch (InterruptedException e) {
e.printStackTrace();
}
resource.increace();
}
}
}
// 消费者线程,负责消费公共资源
class ConsumerThread implements Runnable {
private PublicResource resource;
public ConsumerThread(PublicResource resource) {
this.resource = resource;
}
@Override
public void run() {
for (int i = 0; i < 10; i++) {
try {
Thread.sleep((long) (Math.random() \* 1000));
} catch (InterruptedException e) {
e.printStackTrace();
}
resource.decreace();
}
}
}
public class ProducerConsumerTest {
public static void main(String[] args) {
PublicResource resource = new PublicResource();
new Thread(new ProducerThread(resource)).start();
new Thread(new ConsumerThread(resource)).start();
new Thread(new ProducerThread(resource)).start();
new Thread(new ConsumerThread(resource)).start();
new Thread(new ProducerThread(resource)).start();
new Thread(new ConsumerThread(resource)).start();
}
}
#### 3.3 多线程发短信
### 四、设计模式
* [观察者设计模式](https://bbs.csdn.net/topics/618166371)
* [单例模式](https://bbs.csdn.net/topics/618166371)
* [简单工厂](https://bbs.csdn.net/topics/618166371)
* [工厂方法](https://bbs.csdn.net/topics/618166371)
* [抽象工厂设计模式](https://bbs.csdn.net/topics/618166371)
* [代理设计模式](https://bbs.csdn.net/topics/618166371)
* [动态代理设计模式](https://bbs.csdn.net/topics/618166371)
### 五、排序
* [快排](https://bbs.csdn.net/topics/618166371)
* [归并排序](https://bbs.csdn.net/topics/618166371)
### 六、查找
* [二分查找](https://bbs.csdn.net/topics/618166371)
### 七、存储
* [堆栈区别](https://bbs.csdn.net/topics/618166371)
### 总结
为了帮助大家更好温习重点知识、更高效的准备面试,特别整理了《前端工程师面试手册》电子稿文件。
内容包括html,css,JavaScript,ES6,计算机网络,浏览器,工程化,模块化,Node.js,框架,数据结构,性能优化,项目等等。
包含了腾讯、字节跳动、小米、阿里、滴滴、美团、58、拼多多、360、新浪、搜狐等一线互联网公司面试被问到的题目,涵盖了初中级前端技术点。
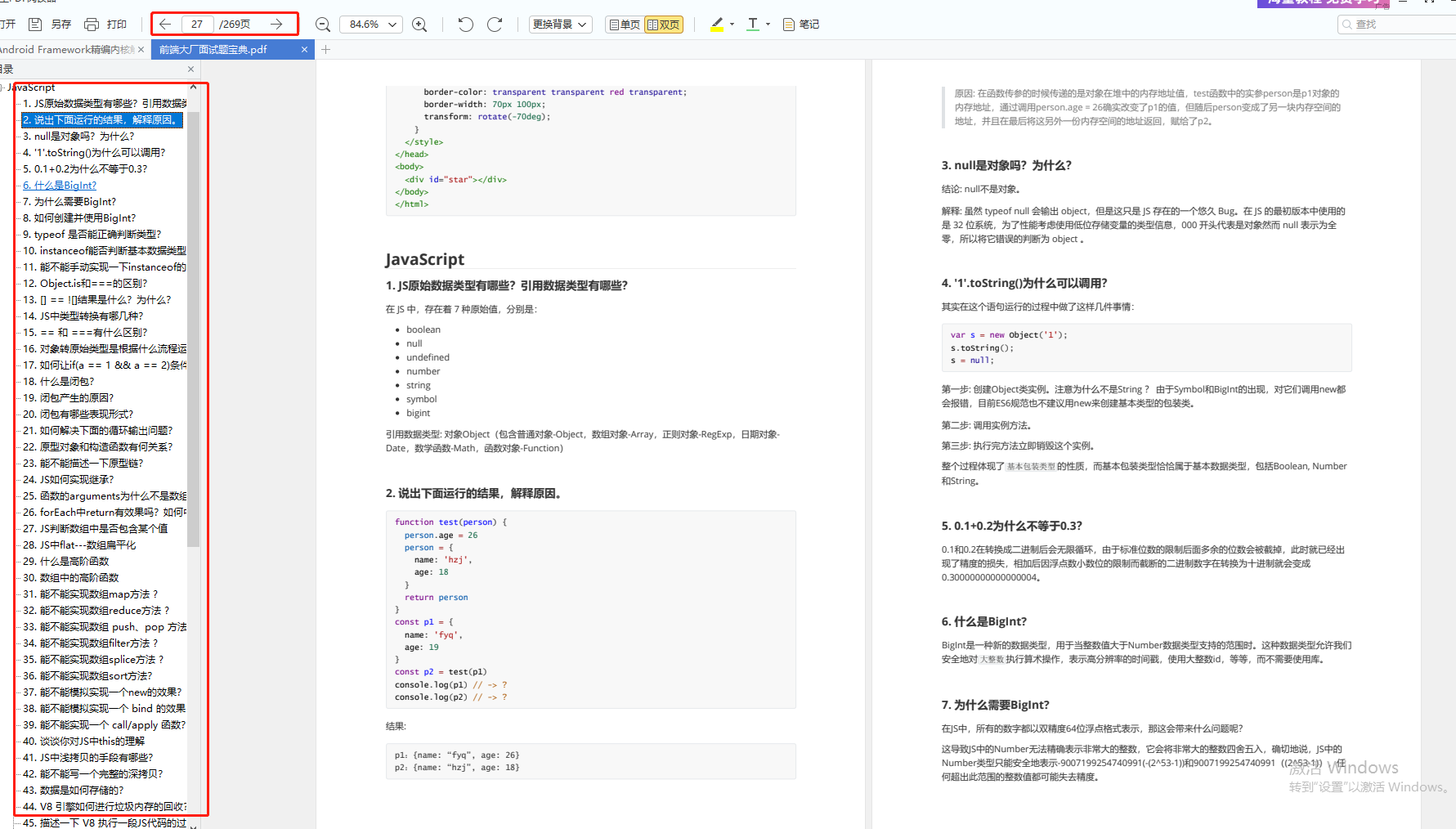
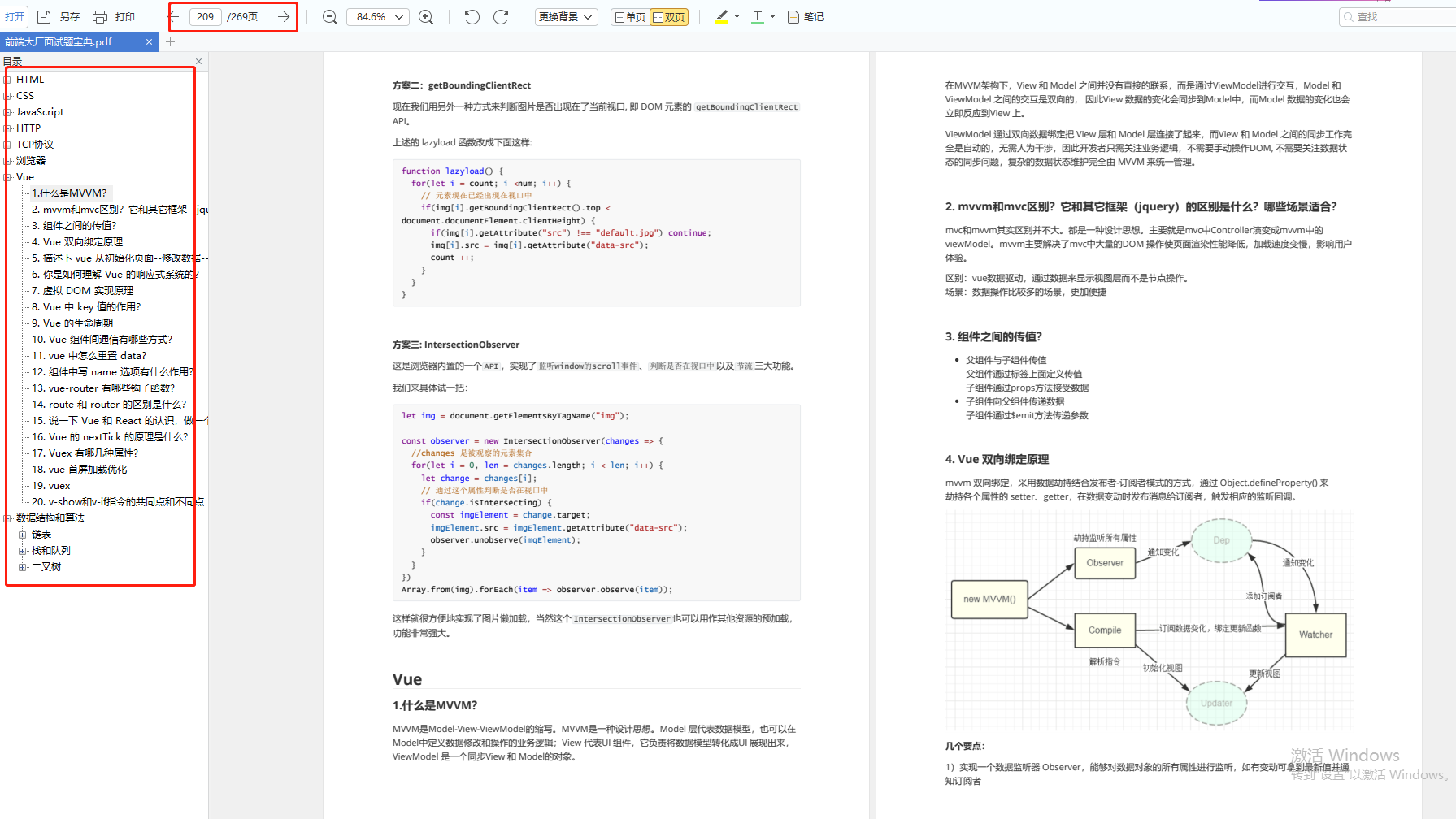
**前端面试题汇总**
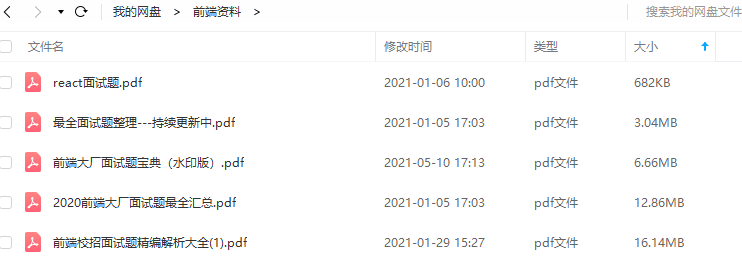
**[开源分享:【大厂前端面试题解析+核心总结学习笔记+真实项目实战+最新讲解视频】](https://bbs.csdn.net/topics/618166371)**
**JavaScript**
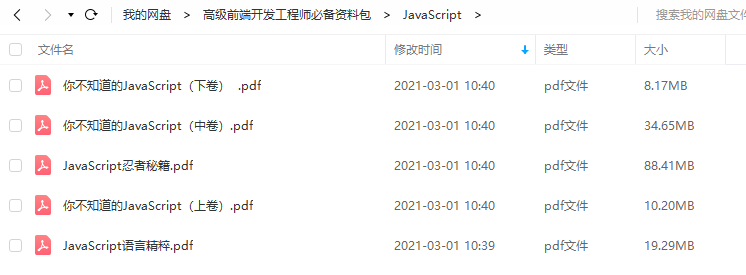
**性能**
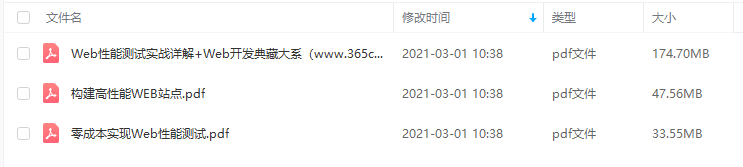
**linux**
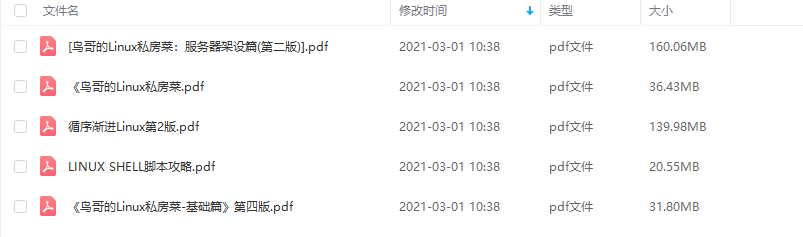