最后
总的来说,面试官要是考察思路就会从你实际做过的项目入手,考察你实际编码能力,就会让你在电脑敲代码,看你用什么编辑器、插件、编码习惯等。所以我们在回答面试官问题时,有一个清晰的逻辑思路,清楚知道自己在和面试官说项目说技术时的话就好了
开源分享:【大厂前端面试题解析+核心总结学习笔记+真实项目实战+最新讲解视频】
注意:vscode编译器中,js文件使用装饰器会报红。解决方式:
在根目录编写jsconfig.json
{
"compilerOptions": {
"module": "commonjs",
"target": "es6",
"experimentalDecorators": true
},
"include": ["src/\*\*/\*"]
}
二、对 observables 作出响应
1. computed
计算值是可以根据现有的状态或其它计算值衍生出的值, 跟vue中的computed非常相似。
import {observable,computed} from 'mobx'
class Store{
@observable arr = [];
@observable obj = {a: 1};
@observable map = new Map();
@observable str = 'hello';
@observable num = 123;
@observable bool = false;
}
const store = new Store();
const result = computed(()=>{
console.log(1)//初始化会执行一次 修改也会执行
store.str + store.num
})
console.log(result.get());
// 监听数据的变化
result.observe((change)=>{
console.log('result:', change);//打印两次 因为store修改了两次
})
//两次对store属性的修改都会引起result的变化
store.str = 'world';
store.num = 220;
computed可作为装饰器, 将result的计算添加到类中:
class Store{
@observable arr = [];
@observable obj = {a: 1};
@observable map = new Map();
@observable str = 'hello';
@observable num = 123;
@observable bool = false;
@computed get result(){
return this.str + this.num;
}
}
2. autorun
当你想创建一个响应式函数,而该函数本身永远不会有观察者时,可以使用 mobx.autorun
所提供的函数总是立即被触发一次,然后每次它的依赖关系改变时会再次被触发。
经验法则:如果你有一个函数应该自动运行,但不会产生一个新的值,请使用autorun。 其余情况都应该使用 computed。
//aotu会立即触发一次
autorun(()=>{
console.log(store.str + store.num);
})
autorun(()=>{
console.log(store.result);
})
//两次修改都会引起autorun执行
store.num = 220;
store.str = 'world';
3. when
when(predicate: () => boolean, effect?: () => void, options?)
when 观察并运行给定的 predicate,直到返回true。 一旦返回 true,给定的 effect 就会被执行,然后 autorunner(自动运行程序) 会被清理。 该函数返回一个清理器以提前取消自动运行程序。
对于以响应式方式来进行处理或者取消,此函数非常有用。
when(()=>store.bool, ()=>{
console.log('when function run.....');
})
store.bool = true;
4. reaction
用法:reaction(() => data, (data, reaction) => { sideEffect }, options?)
。
autorun 的变种,对于如何追踪 observable 赋予了更细粒度的控制。
它接收两个函数参数,第一个(数据 函数)是用来追踪并返回数据作为第二个函数(效果 函数)的输入。
不同于 autorun 的是当创建时效果 函数不会直接运行,只有在数据表达式首次返回一个新值后才会运行。 在执行 效果 函数时访问的任何 observable 都不会被追踪。
// reaction 第一个参数的返回值作为第二个参数的输入
reaction(()=>[store.str, store.num], (arr)=>{
console.log(arr.join('/')); // world/220
})
//只要[store.str, store.num]中任意一值发生变化,reaction第二个函数都会执行
store.num = 220;
store.str = 'world';
三、改变 observables状态
1. action
接上面案例,添加action到类中:
class Store{
@observable arr = [];
@observable obj = {a: 1};
@observable map = new Map();
@observable str = 'hello';
@observable num = 123;
@observable bool = false;
//获取数据@computed
@computed get result(){
return this.str + this.num;
}
//修改数据@action
@action bar(){
this.str = 'world';
this.num = 40;
}
}
const store = new Store();
//调用action,只会执行一次
store.bar();
2. action.bound
action.bound
可以用来自动地将动作绑定到目标对象。
class Store{
@observable arr = [];
@observable obj = {a: 1};
@observable map = new Map();
@observable str = 'hello';
@observable num = 123;
@observable bool = false;
@computed get result(){
return this.str + this.num;
}
@action bar(){
this.str = 'world';
this.num = 40;
}
//this 永远都是正确的
@action.bound foo(){
this.str = 'world';
this.num = 40;
}
}
const store = new Store();
setInterval(store.foo, 1000)
3. runInAction
action
只能影响正在运行的函数,而无法影响当前函数调用的异步操作。如果你使用async function来处理业务,那么我们可以使用 runInAction
这个API来解决这个问题。
@action async fzz() {
await new Promise((resolve) => {
setTimeout(() => {
resolve(0)
}, 1000)
})
runInAction(()=>{
store.num = 220
store.str = 'world'
})
}
四、react-native + mobx案例 todolist
实现效果
1、 安装mobx
、 mobx-react
(将react和mobx衔接)以及配置装饰器
npm install mobx mobx-react --save-dev
配置装饰器具体步骤https://blog.csdn.net/weixin_44157964/article/details/108292457
2、创建一个store/index.js
3、App.js
中导入store/index.js
, 并且导入mobx-react
, 使用Provider
包裹组件
4、页面中使用mobx
inject
把store
注入到当前类组件的属性中
observer
观测render
函数中用了哪些可观察状态 , 如果store
中的值有变化 , render
函数就会重新渲染
二、webpack + mobx 环境配置
mkdir mobx-demo
cd mobx-demo
npm init -y
npm i webpack webpack-cli webpack-dev-server -D
npm i html-webpack-plugin -D
npm i babel-loader @babel/core @babel/preset-env -D
npm i @babel/plugin-proposal-decorators @babel/plugin-proposal-class-properties -D
npm i @babel/plugin-transform-runtime -D
npm i @babel/runtime -S
npm i mobx -S
### 结尾
学习html5、css、javascript这些基础知识,学习的渠道很多,就不多说了,例如,一些其他的优秀博客。但是本人觉得看书也很必要,可以节省很多时间,常见的javascript的书,例如:javascript的高级程序设计,是每位前端工程师必不可少的一本书,边看边用,了解js的一些基本知识,基本上很全面了,如果有时间可以读一些,js性能相关的书籍,以及设计者模式,在实践中都会用的到。
**[开源分享:【大厂前端面试题解析+核心总结学习笔记+真实项目实战+最新讲解视频】](https://bbs.csdn.net/forums/4304bb5a486d4c3ab8389e65ecb71ac0)**
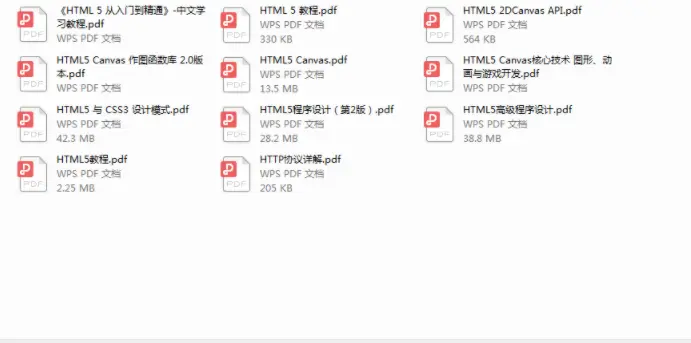
学习的渠道很多,就不多说了,例如,一些其他的优秀博客。但是本人觉得看书也很必要,可以节省很多时间,常见的javascript的书,例如:javascript的高级程序设计,是每位前端工程师必不可少的一本书,边看边用,了解js的一些基本知识,基本上很全面了,如果有时间可以读一些,js性能相关的书籍,以及设计者模式,在实践中都会用的到。
**[开源分享:【大厂前端面试题解析+核心总结学习笔记+真实项目实战+最新讲解视频】](https://bbs.csdn.net/forums/4304bb5a486d4c3ab8389e65ecb71ac0)**
[外链图片转存中...(img-2zQlWbzR-1715789870599)]