class-validator可以说是一个简化验证的依赖库 (采用注释的方式进行校验)
但是缺少中文文档和过程,以自己的理解和对官网文档的阅读进行整理输出。
它的好兄弟class-transformer
class-validator官方提供的方式还不能直接对一个请求的返回值进行校验,它要求必须要是类的一个对象,所以需要做一些处理。
可以将一个json转成指定的类的对象
import { Type, plainToClass } from 'class-transformer';
//plainToClass json转class
//type 指定隐式类型
let users = plainToClass(User, userJson);
1.引入
import {
validate,
validateOrReject,
Contains,
IsInt,
Length,
IsEmail,
IsFQDN,
IsDate,
Min,
Max,
} from 'class-validator'; //引入
2.输出类
export class Post {
@Length(10, 20)
title: string;
@Contains('hello')
text: string;
@IsInt()
@Min(0)
@Max(10)
rating: number;
@IsEmail()
email: string;
@IsFQDN()
site: string;
@IsDate()
createDate: Date;
} //导出类
3.声明类并调用类中的方法进行校验
let post = new Post(); //声明一个post
post.title = 'Hello'; // 不通过
post.text = 'this is a great post about hell world'; // 不通过
post.rating = 11; // 不通过
post.email = 'google.com'; // 不通过
post.site = 'googlecom'; // 不通过
4.验证监听
validate(post).then(errors => {
// errors is an array of validation errors
if (errors.length > 0) {
console.log('validation failed. errors: ', errors);
} else {
console.log('validation succeed');
}
});
5.错误输出
此方法返回一个validationError 对象 每一项如下
{
target: Object; // 已经验证的对象.
property: string; // 未通过验证的对象属性.
value: any; // 未通过验证的值.
constraints?: { // 验证失败并带有错误消息.
[type: string]: string;
};
children?: ValidationError[]; // Contains all nested validation errors of the property
}
6.消息
在装饰器下提示指定信息
import { MinLength, MaxLength } from 'class-validator';
export class Post {
@MinLength(10, {
message: 'Title is too short',
})
@MaxLength(50, {
message: 'Title is too long',
})
title: string;
}
在消息中可以使用特殊的$符号
$value - 正在验证的值
$property - 要验证的对象属性的名称
$target - 要验证的对象的类的名称
$constraint1, $constraint2, ... $constraintN - 由特定验证类型定义的约束
举个栗子 》》》》》》》》》
import { MinLength, MaxLength } from 'class-validator';
export class Post {
@MinLength(10, {
**前端面试题汇总**
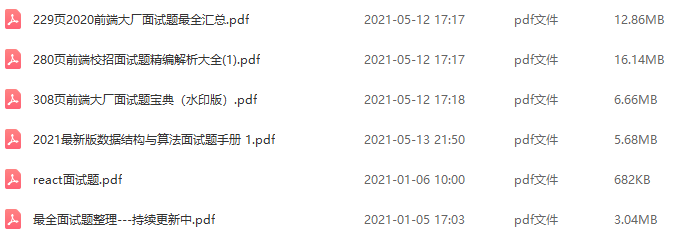
**JavaScript**
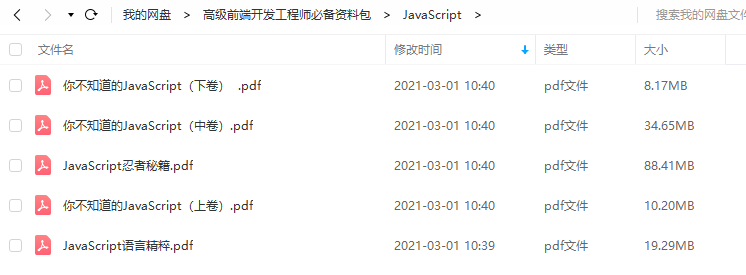
**性能**
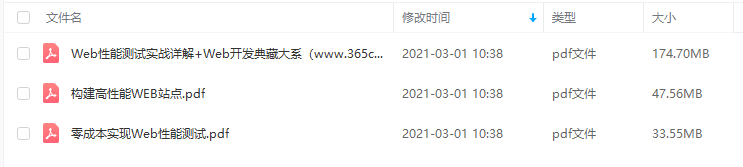
**linux**
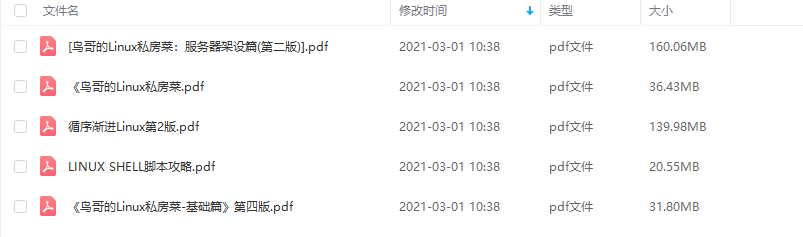
**前端资料汇总**
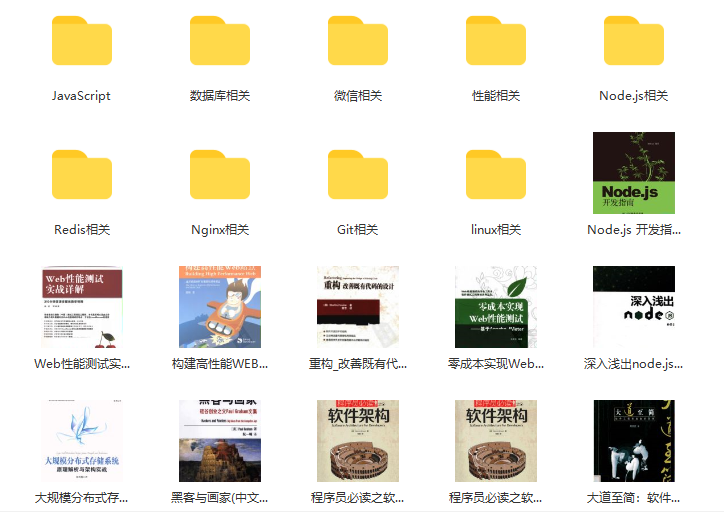