};
mixer(…phone1); // 等于:一一对应,apple vivo oppo
mixer(…phone2); // 小于:缺少值为undefined,apple vivo undefined
mixer(…phone3); // 多于:多的参数值被忽略,apple vivo oppo

###### (6)将类数组转换为数组
const argsTransformArr = (…args) => console.log(args);
argsTransformArr(“pineapple”); // [“pineapple”]
argsTransformArr(66, 88, 99); // [66, 88, 99]

##### 2. 对象
###### (1)复制对象
const student = {
name: “Jack”,
school: {
class: “Software Engineering Class 2”
}
};
const studentCopy = { …student };
console.log(studentCopy); // {name: “Jack”,school:{class: “Software Engineering Class 3”}}

###### (2)合并对象——属性各不相同
* 新对象包含了合并的对象的`所有属性`
const personName = { name: “nina” };
const personSex = { sex: “female” };
const personAge = { age: 18 };
const person = { …personName, …personSex, …personAge };
console.log(person); // {name: “nina”, sex: “female”, age: 18}

###### (3)合并对象——包含相同属性
* 合并的对象中包含有`同名的属性`,则后面对象中的属性值`覆盖`前面的同名的属性值
const fruit1 = {
name: “apple”,
color: “red”
};
const fruit2 = {
name: “strawberry”,
weight: “20g”
};
const fruit = { …fruit1, …fruit2 };
console.log(fruit); // {name: “strawberry”, color: “red”, weight: “20g”}

###### (4)内部属性为对象进行展开——内部对象不会展开
const fruit3 = {
detail: {
name: “apple”,
size: “big”,
weight: “300g”
}
};
console.log({ …fruit3 });

###### (5)非对象展开
* 展开的是`空对象`,则仍然是空对象
* 展开的`不是对象`,则会自动将其转为对象,但是新创建的对象由于并`不包含任何属性`,故为空对象
console.log({ …{} }); // {}
console.log({ …1 }); // {}
console.log({ …undefined }); // {}
console.log({ …null }); // {}
console.log({ …true }); // {}
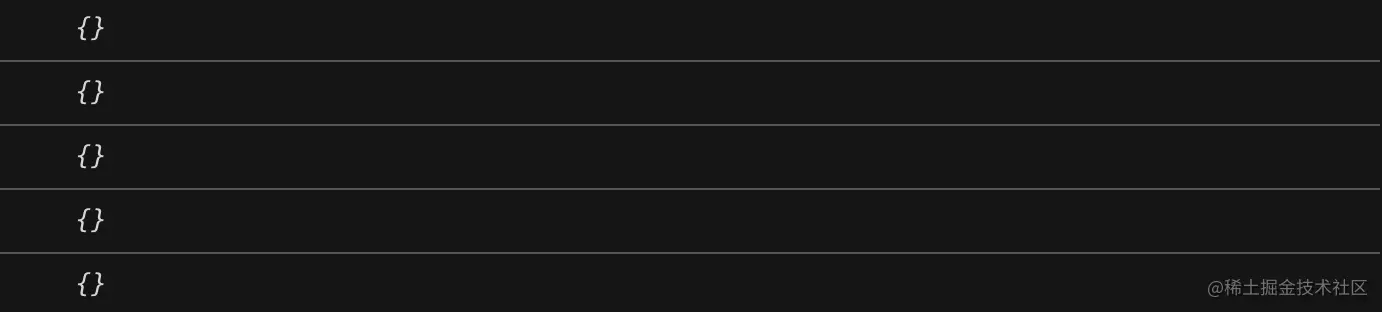
##### 3. 数组与对象合并使用
const tableData1 = {
list: [
{ name: “张三”, address: “南京” },
{ name: “李四”, address: “北京” }
],
pageSize: 10,
pageNum: 1
};
const tableData2 = {
list: [
{ name: “王五”, address: “深圳” },
{ name: “赵六”, address: “上海” }
],
pageSize: 20,
pageNum: 2
};
const allTableDate = {
…tableData1,
list: […tableData1.list, …tableData2.list]
};
console.log(allTableDate);
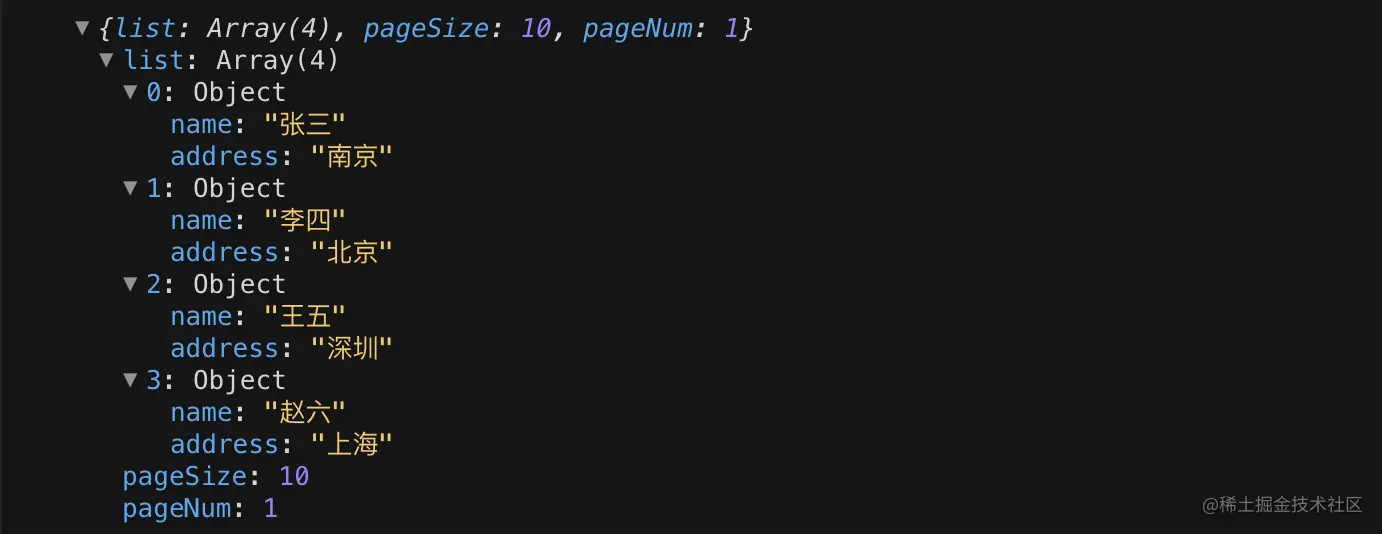
##### 4. 字符串
const name = “Song”;
console.log(…name); // 直接展开,S o n g
console.log([…name]); // 数组形式展开,[“S”, “o”, “n”, “g”]
console.log({ …name }); // 对象形式展开,{0: “S”, 1: “o”, 2: “n”, 3: “g”}
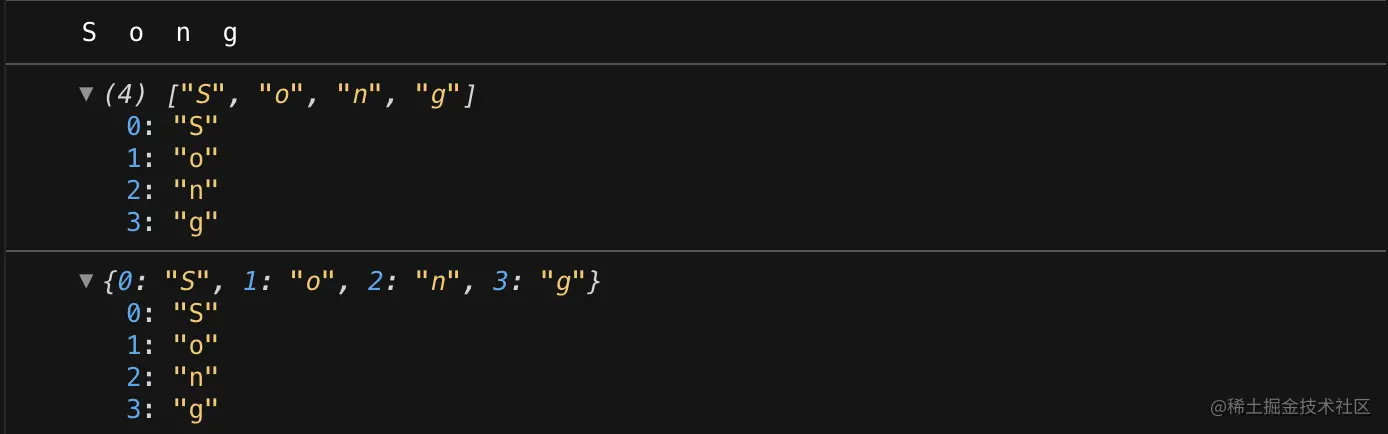
#### 三、 注意点
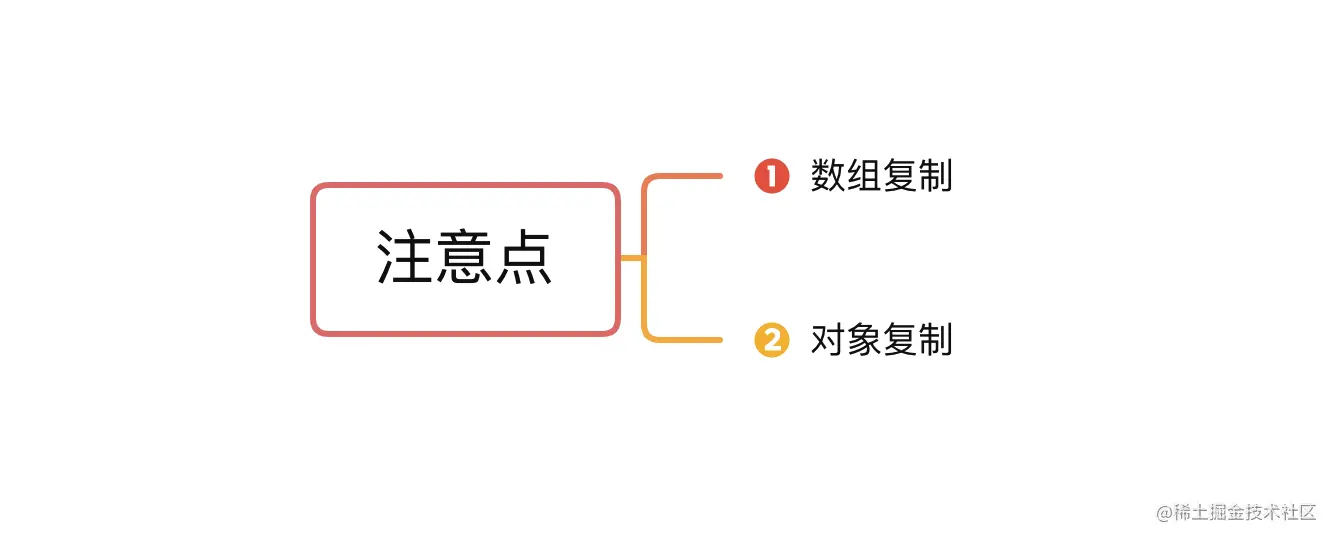
##### 1. 数组复制
* 通过运算符(...)拷贝`数组`,`值类型`的属性被`深度拷贝`了(用的`不同对象`),而引用类型的属性只是做了`浅拷贝`(复制的为`引用地址`,引用对象并未复制,即用的是`同一个对象`)
// 值类型
const citys = [“南京”, “北京”, “上海”, “成都”, “杭州”];
const citysCopy = […citys];
citys[0] = “深圳”;
console.log(citysCopy); // [“南京”, “北京”, “上海”, “成都”, “杭州”]

// 引用类型
let users = [
{
name: “小红”,
sex: “female”
},
{
name: “小明”,
sex: “male”
}
];
let usersCopy = […users];
users[0].name = “小花”;
console.info(usersCopy);

##### 2. 对象复制
* 通过运算符(...)拷贝`对象`,`值类型`的属性被`深度拷贝`了(用的`不同对象`),而引用类型的属性只是做了`浅拷贝`(复制的为`引用地址`,引用对象并未复制,即用的是`同一个对象`)
let user = {
name: “张三”,
sex: “男”,
address: {
city: “北京”
}
};
let clonedUser = { …user };
user.name = “李四”;
user.address.city = “四川”;
console.log(user);
console.log(clonedUser);
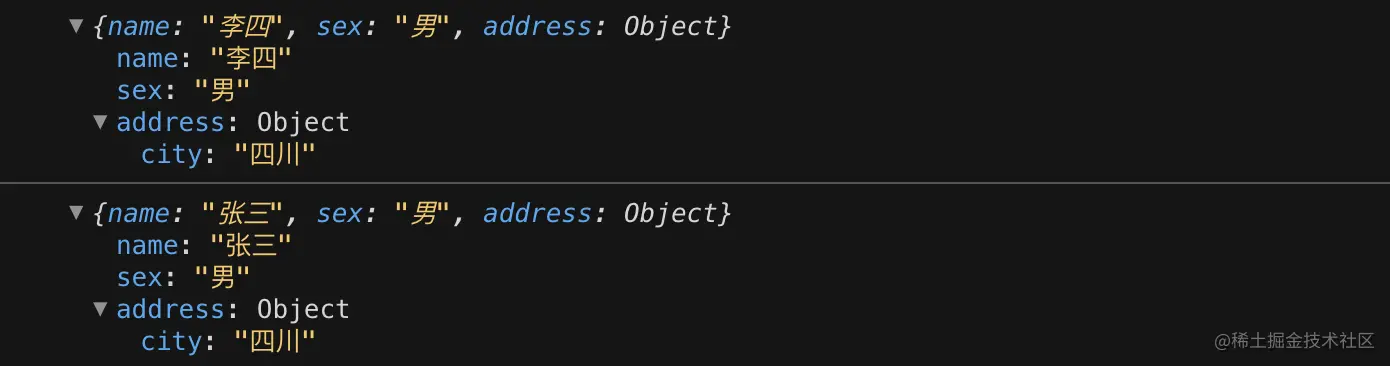
>
> 如有错误或遗漏,欢迎批评指正🌝
>
>
>
#### **前端面试题库 (**面试必备)****推荐:★★★★★****
地址:[前端面试题库](https://bbs.csdn.net/topics/618166371)
### 学习笔记
主要内容包括**html,css,html5,css3,JavaScript,正则表达式,函数,BOM,DOM,jQuery,AJAX,vue**等等
**[开源分享:【大厂前端面试题解析+核心总结学习笔记+真实项目实战+最新讲解视频】](https://bbs.csdn.net/topics/618166371)**
>**HTML/CSS**
**HTML:**HTML基本结构,标签属性,事件属性,文本标签,多媒体标签,列表 / 表格 / 表单标签,其他语义化标签,网页结构,模块划分
**CSS:**CSS代码语法,CSS 放置位置,CSS的继承,选择器的种类/优先级,背景样式,字体样式,文本属性,基本样式,样式重置,盒模型样式,浮动float,定位position,浏览器默认样式
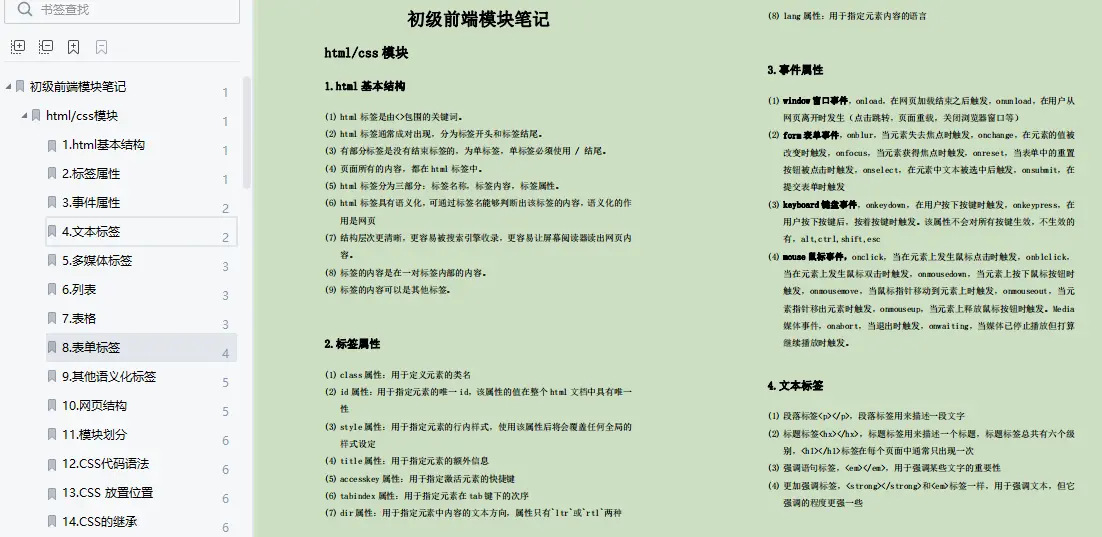
>**HTML5 /CSS3**
**HTML5:**HTML5 的优势,HTML5 废弃元素,HTML5 新增元素,HTML5 表单相关元素和属性
**CSS3:**CSS3 新增选择器,CSS3 新增属性,新增变形动画属性,3D变形属性,CSS3 的过渡属性,CSS3 的动画属性,CSS3 新增多列属性,CSS3新增单位,弹性盒模型
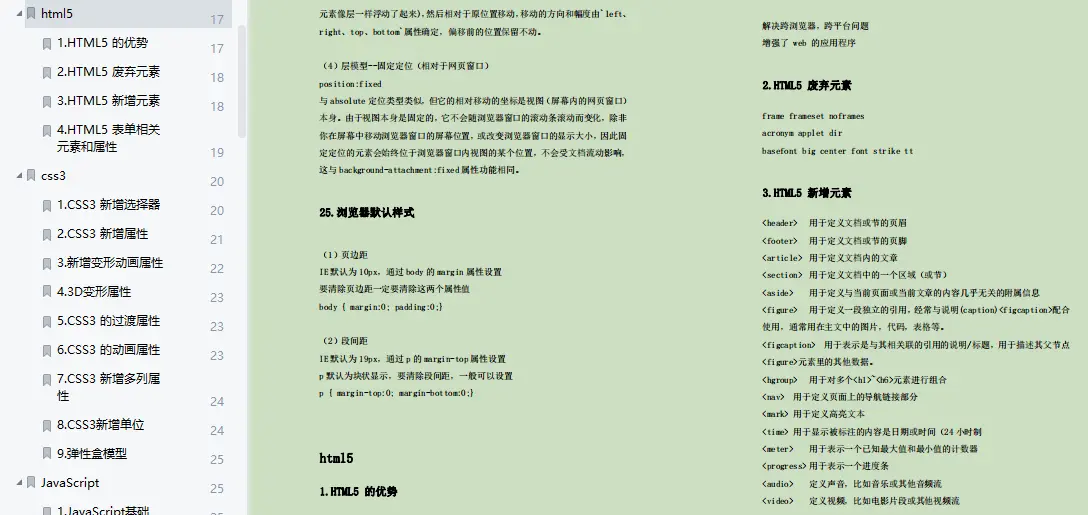
>**JavaScript**
**JavaScript:**JavaScript基础,JavaScript数据类型,算术运算,强制转换,赋值运算,关系运算,逻辑运算,三元运算,分支循环,switch,while,do-while,for,break,continue,数组,数组方法,二维数组,字符串
单相关元素和属性
**CSS3:**CSS3 新增选择器,CSS3 新增属性,新增变形动画属性,3D变形属性,CSS3 的过渡属性,CSS3 的动画属性,CSS3 新增多列属性,CSS3新增单位,弹性盒模型
[外链图片转存中...(img-1xLPGvRO-1714511906044)]
>**JavaScript**
**JavaScript:**JavaScript基础,JavaScript数据类型,算术运算,强制转换,赋值运算,关系运算,逻辑运算,三元运算,分支循环,switch,while,do-while,for,break,continue,数组,数组方法,二维数组,字符串
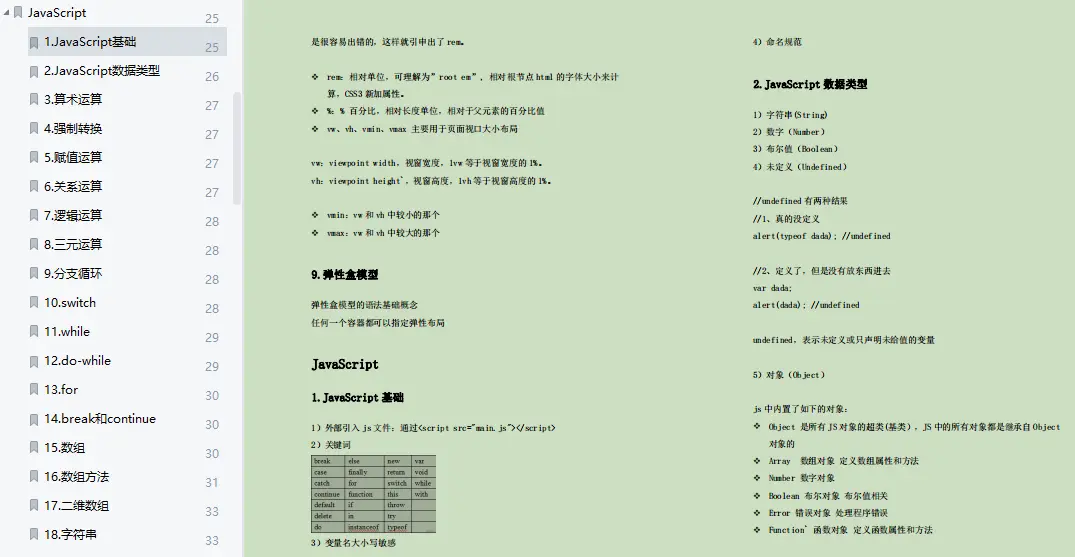