console.log('start 1')
const res = await 2
console.log(res)
console.log('end')
}
run()
console.log('3')
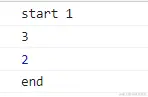
* **await+函数**
**一个最简单的场景:**
function fn() {
console.log('fn start')
console.log('fn end')
}
async function run() {
console.log('start 1')
const res = await fn()
console.log(res)
console.log('end')
}
run()
console.log('3')
运行结果为:
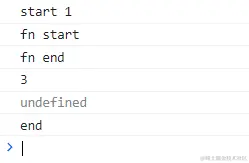
>
> 结论:如果await 右边是一个`函数`,它会立刻执行这个函数,**`而且只有当这个函数执行结束后(即函数完成)!才会将async剩余任务推入微任务队列`**
>
>
>
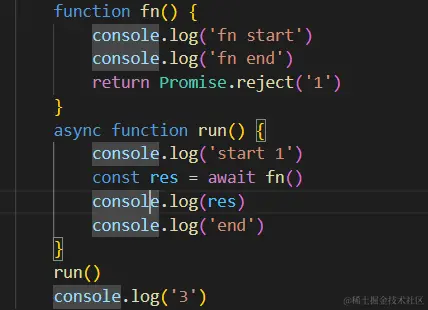
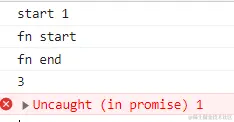
\*\*这是因为await等待的永远是promise,如果函数返回undefined,那么`await会等待函数执行完成`,将返回值转化为`Promise.resolve(undefined)` \*\*(即第二种情况 await + 普通值)。**如果函数执行完成后,返回一个失败的promise,那么它将不会再把剩余任务推入到微任务队列。**
复杂点的案例,**如果fn函数里面嵌套了await**
### 案例:
async function async1() {
console.log(1)
await async2()
console.log(2)
}
const async2 = async () => {
await setTimeout(() => {
Promise.resolve().then(() => {
console.log(3)
})
console.log(4)
}, 0)
}
const async3 = async () => {
Promise.resolve().then(() => {
console.log(6)
})
}
async1()
console.log(7)
async3()
**分析:**
* 首先调用async1 **输出1** ,await async2()**立刻调用async2()**,直至async2函数完成后,才会将cl(2)推入微任务队列
* 调用async2(), await 定时器,(**定时器本身也是个函数**),因此先将定时器的回调函数推入宏任务队列,定时器本身返回一个定时器ID
因此,async2可以转化为:
async ()=>{
await 数值; //即第二种情况
}
**重点:await 数值会转化为await Promise.resolve(数值),再将`async函数中剩余任务推入到微任务队列执行`。这时候,`async2函数中的剩余任务还有个return undefined`,这代表`async2函数并不能立刻执行完毕`,会将`return undefined推入到微任务队列中`(这才代表着`async2函数真正执行结束`)**
目前:宏任务队列:定时器回调函数任务
微任务队列:**return undefined(async2函数执行完毕)**
* 回到开始,**await async2(),目前async2还没有执行结束**,因此调用cl(7)
* 调用async3(),微任务队列推入 cl(6)
目前:宏任务队列:定时器回调函数任务
微任务队列:**return undefined(async2函数执行完毕)** cl(6)
* 从微任务队列中取出第一个任务,**return undefined**,`async2()函数执行完毕,await async2()` 转化为 Promise.resolve(undefined),因此将cl(2) 推入微任务队列
所以真正的结果是:1 7 6 2 4 3
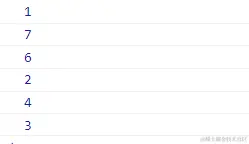
案例2:
async function async1() {
console.log(1)
await async2()
console.log(2)
}
const async2 = async () => {
await (async () => {
await (() => {
console.log(3)
})()
console.log(4)
})()
}
const async3 = async () => {
Promise.resolve().then(() => {
console.log(6)
})
}
React
-
介绍一下react
-
React单项数据流
-
react生命周期函数和react组件的生命周期
-
react和Vue的原理,区别,亮点,作用
-
reactJs的组件交流
-
有了解过react的虚拟DOM吗,虚拟DOM是怎么对比的呢
-
项目里用到了react,为什么要选择react,react有哪些好处
-
怎么获取真正的dom
-
选择react的原因
-
react的生命周期函数
-
setState之后的流程
-
react高阶组件知道吗?
-
React的jsx,函数式编程
-
react的组件是通过什么去判断是否刷新的
-
如何配置React-Router
-
路由的动态加载模块
-
Redux中间件是什么东西,接受几个参数
-
redux请求中间件如何处理并发
开源分享:【大厂前端面试题解析+核心总结学习笔记+真实项目实战+最新讲解视频】